Vector printing incorrect data
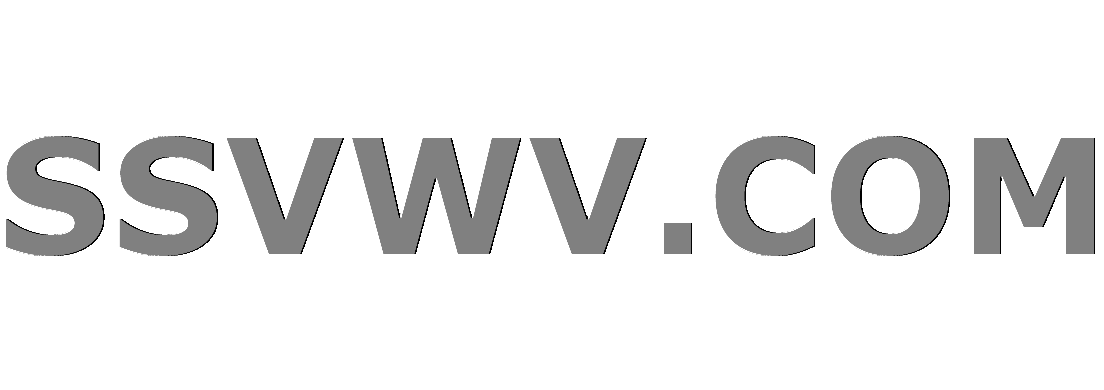
Multi tool use
Can someone tell my why my program prints wrong values?
I've mostly followed an youtube video how to use vectors. And the program which was written, i was able to run it. I tried to implement the same method for my program which is for cars (video was for students). But i'm having problems. Funny part is, that the student program ran well, i don't remember changing anything to it, and now it also doesn't print anything at all.
So i'm trying to make a program that inputs car brand, year and price into a vector of a class and then print out all the stored information. But i'm currently getting mixed results.
I'm either getting blank spaces for results or some huge numbers and blank space for the brand (string)
When i'm not using references & i get blank results, with & i get what i've written below.
Does anyone have an idea?
When i input:
brand - Lambo
year - 1997
price 25000
I get:
Car brand:
Car year: 2686588
Car price: 6.95144e-308
main.cpp
#include <iostream>
#include <string>
#include "Car.h"
#include <vector>
using namespace std;
Car::Car(){
}
Car::Car(string brand, int year, double price){
brand;
year;
price;
}
Car::~Car(){
}
string Car::getBrand() const{
return brand;
}
int Car::getYear() const{
return year;
}
double Car::getPrice()const{
return price;
}
void Car::setBrand(string brand){
brand;
}
void Car::setYear(int year){
year;
}
void Car::setPrice(double price){
price;
}
void enterData(vector<Car>&);
void showData(const vector<Car>&);
int main(){
vector<Car> myGarage;
int chc;
cout << "-- M A I N M E N U --nn";
do{
cout << "Please make a choicenn";
cout << "1. Enter car details" << endl;
cout << "2. Show car details" << endl;
cout << "3. Exit" << endl;
cin >> chc;
switch(chc){
case 1:
enterData(myGarage);
break;
case 2:
showData(myGarage);
break;
case 3:
cout << "Have a nice day!";
break;
}
}
while(chc != 3);
}
void enterData(vector<Car>& newMyGarage){
string brand;
int year;
double price;
cout << "How many cars are in your garage?";
int garageSize;
cin >> garageSize;
for(int i = 0; i < garageSize; i++){
cout << "Car brand: ";
cin >> brand;
cout << "Car year: ";
cin >> year;
cout << "Car price: ";
cin >> price;
Car newCar(brand, year, price);
newMyGarage.push_back(newCar);
cout << endl;
}
}
void showData(const vector<Car>& newMyGarage){
unsigned int size = newMyGarage.size();
for(unsigned int s = 0; s < size; s++){
cout << "Car brand: " << newMyGarage[s].getBrand() << endl;
cout << "Car year: " << newMyGarage[s].getYear() << endl;
cout << "Car price: " << newMyGarage[s].getPrice() << endl;
cout << endl;
}
}
header file Car.h
#ifndef CAR_H_INCLUDED
#define CAR_H_INCLUDED
#include <iostream>
#include <string>
using namespace std;
class Car{
public:
string brand;
int year;
double price;
Car();
Car(string, int, double);
~Car();
string getBrand() const;
int getYear() const;
double getPrice() const;
void setBrand(string);
void setYear(int);
void setPrice(double);
};
#endif // CAR_H_INCLUDED
class vector printing result
add a comment |
Can someone tell my why my program prints wrong values?
I've mostly followed an youtube video how to use vectors. And the program which was written, i was able to run it. I tried to implement the same method for my program which is for cars (video was for students). But i'm having problems. Funny part is, that the student program ran well, i don't remember changing anything to it, and now it also doesn't print anything at all.
So i'm trying to make a program that inputs car brand, year and price into a vector of a class and then print out all the stored information. But i'm currently getting mixed results.
I'm either getting blank spaces for results or some huge numbers and blank space for the brand (string)
When i'm not using references & i get blank results, with & i get what i've written below.
Does anyone have an idea?
When i input:
brand - Lambo
year - 1997
price 25000
I get:
Car brand:
Car year: 2686588
Car price: 6.95144e-308
main.cpp
#include <iostream>
#include <string>
#include "Car.h"
#include <vector>
using namespace std;
Car::Car(){
}
Car::Car(string brand, int year, double price){
brand;
year;
price;
}
Car::~Car(){
}
string Car::getBrand() const{
return brand;
}
int Car::getYear() const{
return year;
}
double Car::getPrice()const{
return price;
}
void Car::setBrand(string brand){
brand;
}
void Car::setYear(int year){
year;
}
void Car::setPrice(double price){
price;
}
void enterData(vector<Car>&);
void showData(const vector<Car>&);
int main(){
vector<Car> myGarage;
int chc;
cout << "-- M A I N M E N U --nn";
do{
cout << "Please make a choicenn";
cout << "1. Enter car details" << endl;
cout << "2. Show car details" << endl;
cout << "3. Exit" << endl;
cin >> chc;
switch(chc){
case 1:
enterData(myGarage);
break;
case 2:
showData(myGarage);
break;
case 3:
cout << "Have a nice day!";
break;
}
}
while(chc != 3);
}
void enterData(vector<Car>& newMyGarage){
string brand;
int year;
double price;
cout << "How many cars are in your garage?";
int garageSize;
cin >> garageSize;
for(int i = 0; i < garageSize; i++){
cout << "Car brand: ";
cin >> brand;
cout << "Car year: ";
cin >> year;
cout << "Car price: ";
cin >> price;
Car newCar(brand, year, price);
newMyGarage.push_back(newCar);
cout << endl;
}
}
void showData(const vector<Car>& newMyGarage){
unsigned int size = newMyGarage.size();
for(unsigned int s = 0; s < size; s++){
cout << "Car brand: " << newMyGarage[s].getBrand() << endl;
cout << "Car year: " << newMyGarage[s].getYear() << endl;
cout << "Car price: " << newMyGarage[s].getPrice() << endl;
cout << endl;
}
}
header file Car.h
#ifndef CAR_H_INCLUDED
#define CAR_H_INCLUDED
#include <iostream>
#include <string>
using namespace std;
class Car{
public:
string brand;
int year;
double price;
Car();
Car(string, int, double);
~Car();
string getBrand() const;
int getYear() const;
double getPrice() const;
void setBrand(string);
void setYear(int);
void setPrice(double);
};
#endif // CAR_H_INCLUDED
class vector printing result
In the overloaded constructor, you do not assign any values to the class variables. (the same for the set functions as well) ... so the output is uninitialized garbage values for year and price, the brand is likely an empty string so it appears to be blank.
– Paul T.
Nov 23 '18 at 2:42
add a comment |
Can someone tell my why my program prints wrong values?
I've mostly followed an youtube video how to use vectors. And the program which was written, i was able to run it. I tried to implement the same method for my program which is for cars (video was for students). But i'm having problems. Funny part is, that the student program ran well, i don't remember changing anything to it, and now it also doesn't print anything at all.
So i'm trying to make a program that inputs car brand, year and price into a vector of a class and then print out all the stored information. But i'm currently getting mixed results.
I'm either getting blank spaces for results or some huge numbers and blank space for the brand (string)
When i'm not using references & i get blank results, with & i get what i've written below.
Does anyone have an idea?
When i input:
brand - Lambo
year - 1997
price 25000
I get:
Car brand:
Car year: 2686588
Car price: 6.95144e-308
main.cpp
#include <iostream>
#include <string>
#include "Car.h"
#include <vector>
using namespace std;
Car::Car(){
}
Car::Car(string brand, int year, double price){
brand;
year;
price;
}
Car::~Car(){
}
string Car::getBrand() const{
return brand;
}
int Car::getYear() const{
return year;
}
double Car::getPrice()const{
return price;
}
void Car::setBrand(string brand){
brand;
}
void Car::setYear(int year){
year;
}
void Car::setPrice(double price){
price;
}
void enterData(vector<Car>&);
void showData(const vector<Car>&);
int main(){
vector<Car> myGarage;
int chc;
cout << "-- M A I N M E N U --nn";
do{
cout << "Please make a choicenn";
cout << "1. Enter car details" << endl;
cout << "2. Show car details" << endl;
cout << "3. Exit" << endl;
cin >> chc;
switch(chc){
case 1:
enterData(myGarage);
break;
case 2:
showData(myGarage);
break;
case 3:
cout << "Have a nice day!";
break;
}
}
while(chc != 3);
}
void enterData(vector<Car>& newMyGarage){
string brand;
int year;
double price;
cout << "How many cars are in your garage?";
int garageSize;
cin >> garageSize;
for(int i = 0; i < garageSize; i++){
cout << "Car brand: ";
cin >> brand;
cout << "Car year: ";
cin >> year;
cout << "Car price: ";
cin >> price;
Car newCar(brand, year, price);
newMyGarage.push_back(newCar);
cout << endl;
}
}
void showData(const vector<Car>& newMyGarage){
unsigned int size = newMyGarage.size();
for(unsigned int s = 0; s < size; s++){
cout << "Car brand: " << newMyGarage[s].getBrand() << endl;
cout << "Car year: " << newMyGarage[s].getYear() << endl;
cout << "Car price: " << newMyGarage[s].getPrice() << endl;
cout << endl;
}
}
header file Car.h
#ifndef CAR_H_INCLUDED
#define CAR_H_INCLUDED
#include <iostream>
#include <string>
using namespace std;
class Car{
public:
string brand;
int year;
double price;
Car();
Car(string, int, double);
~Car();
string getBrand() const;
int getYear() const;
double getPrice() const;
void setBrand(string);
void setYear(int);
void setPrice(double);
};
#endif // CAR_H_INCLUDED
class vector printing result
Can someone tell my why my program prints wrong values?
I've mostly followed an youtube video how to use vectors. And the program which was written, i was able to run it. I tried to implement the same method for my program which is for cars (video was for students). But i'm having problems. Funny part is, that the student program ran well, i don't remember changing anything to it, and now it also doesn't print anything at all.
So i'm trying to make a program that inputs car brand, year and price into a vector of a class and then print out all the stored information. But i'm currently getting mixed results.
I'm either getting blank spaces for results or some huge numbers and blank space for the brand (string)
When i'm not using references & i get blank results, with & i get what i've written below.
Does anyone have an idea?
When i input:
brand - Lambo
year - 1997
price 25000
I get:
Car brand:
Car year: 2686588
Car price: 6.95144e-308
main.cpp
#include <iostream>
#include <string>
#include "Car.h"
#include <vector>
using namespace std;
Car::Car(){
}
Car::Car(string brand, int year, double price){
brand;
year;
price;
}
Car::~Car(){
}
string Car::getBrand() const{
return brand;
}
int Car::getYear() const{
return year;
}
double Car::getPrice()const{
return price;
}
void Car::setBrand(string brand){
brand;
}
void Car::setYear(int year){
year;
}
void Car::setPrice(double price){
price;
}
void enterData(vector<Car>&);
void showData(const vector<Car>&);
int main(){
vector<Car> myGarage;
int chc;
cout << "-- M A I N M E N U --nn";
do{
cout << "Please make a choicenn";
cout << "1. Enter car details" << endl;
cout << "2. Show car details" << endl;
cout << "3. Exit" << endl;
cin >> chc;
switch(chc){
case 1:
enterData(myGarage);
break;
case 2:
showData(myGarage);
break;
case 3:
cout << "Have a nice day!";
break;
}
}
while(chc != 3);
}
void enterData(vector<Car>& newMyGarage){
string brand;
int year;
double price;
cout << "How many cars are in your garage?";
int garageSize;
cin >> garageSize;
for(int i = 0; i < garageSize; i++){
cout << "Car brand: ";
cin >> brand;
cout << "Car year: ";
cin >> year;
cout << "Car price: ";
cin >> price;
Car newCar(brand, year, price);
newMyGarage.push_back(newCar);
cout << endl;
}
}
void showData(const vector<Car>& newMyGarage){
unsigned int size = newMyGarage.size();
for(unsigned int s = 0; s < size; s++){
cout << "Car brand: " << newMyGarage[s].getBrand() << endl;
cout << "Car year: " << newMyGarage[s].getYear() << endl;
cout << "Car price: " << newMyGarage[s].getPrice() << endl;
cout << endl;
}
}
header file Car.h
#ifndef CAR_H_INCLUDED
#define CAR_H_INCLUDED
#include <iostream>
#include <string>
using namespace std;
class Car{
public:
string brand;
int year;
double price;
Car();
Car(string, int, double);
~Car();
string getBrand() const;
int getYear() const;
double getPrice() const;
void setBrand(string);
void setYear(int);
void setPrice(double);
};
#endif // CAR_H_INCLUDED
class vector printing result
class vector printing result
asked Nov 23 '18 at 2:23


StenliNSStenliNS
61
61
In the overloaded constructor, you do not assign any values to the class variables. (the same for the set functions as well) ... so the output is uninitialized garbage values for year and price, the brand is likely an empty string so it appears to be blank.
– Paul T.
Nov 23 '18 at 2:42
add a comment |
In the overloaded constructor, you do not assign any values to the class variables. (the same for the set functions as well) ... so the output is uninitialized garbage values for year and price, the brand is likely an empty string so it appears to be blank.
– Paul T.
Nov 23 '18 at 2:42
In the overloaded constructor, you do not assign any values to the class variables. (the same for the set functions as well) ... so the output is uninitialized garbage values for year and price, the brand is likely an empty string so it appears to be blank.
– Paul T.
Nov 23 '18 at 2:42
In the overloaded constructor, you do not assign any values to the class variables. (the same for the set functions as well) ... so the output is uninitialized garbage values for year and price, the brand is likely an empty string so it appears to be blank.
– Paul T.
Nov 23 '18 at 2:42
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439948%2fvector-printing-incorrect-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439948%2fvector-printing-incorrect-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FKOBLPLK
In the overloaded constructor, you do not assign any values to the class variables. (the same for the set functions as well) ... so the output is uninitialized garbage values for year and price, the brand is likely an empty string so it appears to be blank.
– Paul T.
Nov 23 '18 at 2:42