Post-Redirect-Get with Spring WebFlux and Thymeleaf
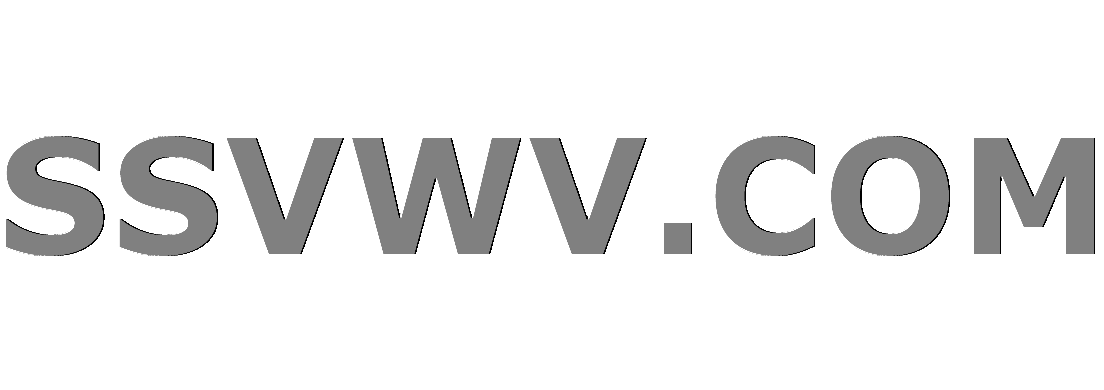
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Can someone explain to me how to implement the post-redirect-get pattern in Spring WebFlux and Thymeleaf? What subscribes on the database save method?
@GetMapping("/register")
public String showRegisterForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@PostMapping
public String processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return "register";
} else {
userRepository.save(user); //what subscribes on this?
//how to redirect on e.g. "/login"?
}
}
thymeleaf spring-webflux
add a comment |
Can someone explain to me how to implement the post-redirect-get pattern in Spring WebFlux and Thymeleaf? What subscribes on the database save method?
@GetMapping("/register")
public String showRegisterForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@PostMapping
public String processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return "register";
} else {
userRepository.save(user); //what subscribes on this?
//how to redirect on e.g. "/login"?
}
}
thymeleaf spring-webflux
add a comment |
Can someone explain to me how to implement the post-redirect-get pattern in Spring WebFlux and Thymeleaf? What subscribes on the database save method?
@GetMapping("/register")
public String showRegisterForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@PostMapping
public String processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return "register";
} else {
userRepository.save(user); //what subscribes on this?
//how to redirect on e.g. "/login"?
}
}
thymeleaf spring-webflux
Can someone explain to me how to implement the post-redirect-get pattern in Spring WebFlux and Thymeleaf? What subscribes on the database save method?
@GetMapping("/register")
public String showRegisterForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@PostMapping
public String processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return "register";
} else {
userRepository.save(user); //what subscribes on this?
//how to redirect on e.g. "/login"?
}
}
thymeleaf spring-webflux
thymeleaf spring-webflux
asked Nov 25 '18 at 10:54


PeterPeter
40219
40219
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can make your controller method return a reactive type like this:
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return Mono.just("register");
} else {
return userRepository.save(user).thenReturn("redirect:/login");
}
}
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
add a comment |
Just for the record I recommend the solution that Brian wrote as is express the intent much better. However if you want to impress your friends. The here is some without the if statement.
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
return Mono
.just(bindingResult.hasErrors())
.filter(t -> t)
.flatMap( t-> Mono.just("register"))
.switchIfEmpty(userRepository.save(user).thenReturn("redirect:/login"));
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53466751%2fpost-redirect-get-with-spring-webflux-and-thymeleaf%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can make your controller method return a reactive type like this:
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return Mono.just("register");
} else {
return userRepository.save(user).thenReturn("redirect:/login");
}
}
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
add a comment |
You can make your controller method return a reactive type like this:
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return Mono.just("register");
} else {
return userRepository.save(user).thenReturn("redirect:/login");
}
}
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
add a comment |
You can make your controller method return a reactive type like this:
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return Mono.just("register");
} else {
return userRepository.save(user).thenReturn("redirect:/login");
}
}
You can make your controller method return a reactive type like this:
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
if (bindingResult.hasErrors()) {
return Mono.just("register");
} else {
return userRepository.save(user).thenReturn("redirect:/login");
}
}
answered Nov 25 '18 at 12:27
Brian ClozelBrian Clozel
32.7k880106
32.7k880106
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
add a comment |
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
I don't know why I didn't try this at the first place. Thank you very much. :)
– Peter
Nov 25 '18 at 12:34
add a comment |
Just for the record I recommend the solution that Brian wrote as is express the intent much better. However if you want to impress your friends. The here is some without the if statement.
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
return Mono
.just(bindingResult.hasErrors())
.filter(t -> t)
.flatMap( t-> Mono.just("register"))
.switchIfEmpty(userRepository.save(user).thenReturn("redirect:/login"));
}
add a comment |
Just for the record I recommend the solution that Brian wrote as is express the intent much better. However if you want to impress your friends. The here is some without the if statement.
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
return Mono
.just(bindingResult.hasErrors())
.filter(t -> t)
.flatMap( t-> Mono.just("register"))
.switchIfEmpty(userRepository.save(user).thenReturn("redirect:/login"));
}
add a comment |
Just for the record I recommend the solution that Brian wrote as is express the intent much better. However if you want to impress your friends. The here is some without the if statement.
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
return Mono
.just(bindingResult.hasErrors())
.filter(t -> t)
.flatMap( t-> Mono.just("register"))
.switchIfEmpty(userRepository.save(user).thenReturn("redirect:/login"));
}
Just for the record I recommend the solution that Brian wrote as is express the intent much better. However if you want to impress your friends. The here is some without the if statement.
@PostMapping
public Mono<String> processRegisterForm(@Valid User user, BindingResult bindingResult) {
return Mono
.just(bindingResult.hasErrors())
.filter(t -> t)
.flatMap( t-> Mono.just("register"))
.switchIfEmpty(userRepository.save(user).thenReturn("redirect:/login"));
}
answered Nov 25 '18 at 21:29


piotr szybickipiotr szybicki
7211511
7211511
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53466751%2fpost-redirect-get-with-spring-webflux-and-thymeleaf%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3j BxV0zjT9Ayptly Z6uNwhxI6tE6tl6vTRGhbkfcvuskUdE2svaCSB gZtCV0bbwp5poDZSA oBHUZg4QP2RWHx9d