Android: GetApplication in RecyclerViewAdapter
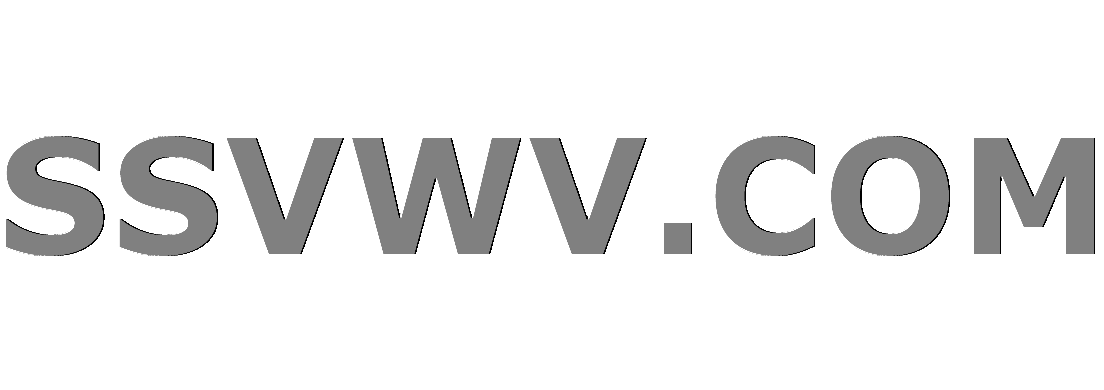
Multi tool use
I would like to inflate a Recyclerview within a onClickListener Method from a Recyclerview.
For that I know that I have to get the Context from the correspoding activity to set the LinearLayoutManager.
Problem:
Since I am using a Recyclerview in Fragment to try to create another Recyclerview I do not know how to get the correct context.
What I tried:
Use FragmentActivity mContext in my constructor to later on try to get the context via "mContext.getApplicationContext()" (got the FragmentActivity from another post, used Context mContext beforehand)
Issue in the Code:
I will break down my Code as good as possible:
- Find the Code from my first Recyclerview from where I try to initialize the LinearLayoutManager
public class deckbuilder_RViewAdapter_Card extends RecyclerView.Adapter {
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData) {
this.mData = mData;
this.mContext = mContext;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(mContext.getApplicationContext(), LinearLayoutManager.VERTICAL, false);
rvList.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_List addCardAdapter = new deckbuilder_RViewAdapter_List(mContext, listCards);
rvList.setAdapter(addCardAdapter);
}
});
}
public int getItemCount() {
return mData.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCardImage;
TextView tvCardName;
public ViewHolder(@NonNull View introListView) {
super(introListView);
fab_deckbuilder_add = introListView.findViewById(R.id.fab_deckbuilder_add);
fab_deckbuilder_remove = introListView.findViewById(R.id.fab_deckbuilder_remove);
rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
}
}
Code from the Recyclerview I want to initialize:
public class deckbuilder_RViewAdapter_List extends RecyclerView.Adapter {
private List<Decklist> mDecklist;
private Context mContext;
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.layout_cardview_deckbuilder_list, viewGroup, false);
return new deckbuilder_RViewAdapter_List.ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, int position) {
viewHolder.ivCard.setImageResource(mDecklist.get(position).getCardImage());
viewHolder.ivType.setImageResource(mDecklist.get(position).getTypeImage());
viewHolder.tvName.setText(mDecklist.get(position).getName());
viewHolder.tvCost.setText(mDecklist.get(position).getCost());
viewHolder.tvNumber.setText(mDecklist.get(position).getNumber());
}
public int getItemCount() {
return mDecklist.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCard, ivType;
TextView tvName, tvCost, tvNumber;
public ViewHolder(@NonNull View itemView) {
super(itemView);
ivCard = itemView.findViewById(R.id.ivDecklistCardImage);
ivType = itemView.findViewById(R.id.ivDecklistTypeImage);
tvName = itemView.findViewById(R.id.tvDecklistName);
tvCost = itemView.findViewById(R.id.tvDecklistCost);
tvNumber = itemView.findViewById(R.id.tvDecklistNumber);
}
}
And Lastly please See my Logcat:
2018-11-11 17:46:15.589 21538-21538/com.example.chris.projectartifact E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.chris.projectartifact, PID: 21538
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.support.v7.widget.RecyclerView.setLayoutManager(android.support.v7.widget.RecyclerView$LayoutManager)' on a null object reference
at com.example.chris.projectartifact.b_deckbuilderTap.deckbuilder_RViewAdapter_Card$3.onClick(deckbuilder_RViewAdapter_Card.java:116)
at android.view.View.performClick(View.java:6291)
at android.view.View$PerformClick.run(View.java:24931)
at android.os.Handler.handleCallback(Handler.java:808)
at android.os.Handler.dispatchMessage(Handler.java:101)
at android.os.Looper.loop(Looper.java:166)
at android.app.ActivityThread.main(ActivityThread.java:7425)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:245)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:921)
Requested edits:
Layout Cardview Decklist:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/cardView_deckbuilder_list_id"
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:cardview= "http://schemas.android.com/apk/res-auto"
android:layout_width="150dp"
android:layout_height="20dp"
android:layout_marginTop="5dp"
cardview:cardCornerRadius="5dp"
android:layout_marginStart="5dp"
android:clickable="true"
android:foreground="?android:attr/selectableItemBackground"
>
<android.support.constraint.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
android:orientation="vertical">
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/axe"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<ImageView
android:id="@+id/ivDecklistCardImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:scrollY="-7dp"
android:src="@drawable/thunder_gods_wrath"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/ivDecklistTypeImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/introspells"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list_secound"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list" />
<TextView
android:id="@+id/tvDecklistCost"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_secound"
android:text="20"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_third"/>
<TextView
android:id="@+id/tvDecklistName"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_third"
android:text="Thunder"
android:autoSizeMaxTextSize="20sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_last"/>
<TextView
android:id="@+id/tvDecklistNumber"
android:layout_width="0dp"
android:layout_height="match_parent"
android:text="x3"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffff"
cardview:layout_constraintEnd_toEndOf="parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_last" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="20dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_secound"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="40dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_third"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="55dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_last"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_end="15dp" />
</android.support.constraint.ConstraintLayout>
</android.support.v7.widget.CardView>
and furthermore please find rv_deckbuilder_list at the end of the following layout:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:background="@drawable/background"
>
<LinearLayout
android:id="@+id/llCarddeck"
android:layout_width="0dp"
android:layout_height="60dp"
android:orientation="vertical"
android:layout_marginEnd="10dp"
android:weightSum="6"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintTop_toTopOf="parent"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Rarity"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Category"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1.5"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Order"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="4"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4">
<ToggleButton
android:id="@+id/btnDeckbuilderBasic"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_basic_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderCommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_common_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderUncommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_uncommon_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderRare"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="2.5dp"
android:layout_marginTop="2.5dp"
android:layout_marginEnd="3dp"
android:layout_marginBottom="2.5dp"
android:layout_weight="1"
android:background="@drawable/y_rare_layout" />
<!--<TextView-->
<!--android:id="@+id/textView"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="wrap_content"-->
<!--android:layout_weight="1"-->
<!--android:text="android:layout_weight="4.25"
-->
<!--android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"" />-->
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4"
android:orientation="horizontal"
>
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilderCategory"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilder"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="1.5"
android:background="@drawable/x_linearlayoutcontainer_blank">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center">
<android.support.v7.widget.SwitchCompat
android:id="@+id/switchDeckbuilders"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:checked="false"
android:gravity="center|fill_horizontal"
android:showText="false" />
</FrameLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<!--<android.support.v7.widget.RecyclerView-->
<!--android:id="@+id/rv_deckbuilder_cards"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="0dp"-->
<!--/>-->
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_cards"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginEnd="10dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/llCarddeck"
/>
<LinearLayout
android:id="@+id/llDecklist"
android:layout_width="0dp"
android:layout_height="30dp"
android:background="@drawable/background"
android:orientation="horizontal"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintEnd_toEndOf="parent"/>
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_list"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintTop_toBottomOf="@+id/llDecklist" />
<!--</android.support.v7.widget.RecyclerView>-->
<android.support.constraint.Guideline
android:id="@+id/gl_v_66"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintGuide_percent="0.66"
android:orientation="vertical" />
</android.support.constraint.ConstraintLayout>
Requested Update 2.0:
The first RV which is called rvCards (via deckbuilder_RViewAdapter_Cards) is getting inflated in the following fragment:
public class deckbuilder_fragment extends Fragment {
//..
List<Cards> listCards;
DBHelper dbHelper;
RecyclerView rvCards;
//..
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// return super.onCreateView(inflater, container, savedInstanceState);
View view = inflater.inflate(R.layout.deckbuilder_fragment, container, false);
//..
//...
dbHelper = new DBHelper(getActivity().getApplicationContext());
dbHelper.createDataBase();
rvCards = view.findViewById(R.id.rv_deckbuilder_cards);
updateAdapter();
return view;
}}
Whereas the Adapter is updated in:
public void updateAdapter() {
listCards = dbHelper.getDeckbuilderCards(spinnerDeckbuilderType.getSelectedItem().toString(), true, btnDeckbuilderBasic.isChecked(), btnDeckbuilderCommon.isChecked(), btnDeckbuilderUncommon.isChecked(),btnDeckbuilderRare.isChecked(), spinnerDeckbuilderCategory.getSelectedItem().toString());
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(getActivity(), LinearLayoutManager.HORIZONTAL, false);
rvCards.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_Card newAdapterCards = new deckbuilder_RViewAdapter_Card(getActivity(), listCards);
rvCards.setAdapter(newAdapterCards);
}
java

add a comment |
I would like to inflate a Recyclerview within a onClickListener Method from a Recyclerview.
For that I know that I have to get the Context from the correspoding activity to set the LinearLayoutManager.
Problem:
Since I am using a Recyclerview in Fragment to try to create another Recyclerview I do not know how to get the correct context.
What I tried:
Use FragmentActivity mContext in my constructor to later on try to get the context via "mContext.getApplicationContext()" (got the FragmentActivity from another post, used Context mContext beforehand)
Issue in the Code:
I will break down my Code as good as possible:
- Find the Code from my first Recyclerview from where I try to initialize the LinearLayoutManager
public class deckbuilder_RViewAdapter_Card extends RecyclerView.Adapter {
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData) {
this.mData = mData;
this.mContext = mContext;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(mContext.getApplicationContext(), LinearLayoutManager.VERTICAL, false);
rvList.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_List addCardAdapter = new deckbuilder_RViewAdapter_List(mContext, listCards);
rvList.setAdapter(addCardAdapter);
}
});
}
public int getItemCount() {
return mData.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCardImage;
TextView tvCardName;
public ViewHolder(@NonNull View introListView) {
super(introListView);
fab_deckbuilder_add = introListView.findViewById(R.id.fab_deckbuilder_add);
fab_deckbuilder_remove = introListView.findViewById(R.id.fab_deckbuilder_remove);
rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
}
}
Code from the Recyclerview I want to initialize:
public class deckbuilder_RViewAdapter_List extends RecyclerView.Adapter {
private List<Decklist> mDecklist;
private Context mContext;
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.layout_cardview_deckbuilder_list, viewGroup, false);
return new deckbuilder_RViewAdapter_List.ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, int position) {
viewHolder.ivCard.setImageResource(mDecklist.get(position).getCardImage());
viewHolder.ivType.setImageResource(mDecklist.get(position).getTypeImage());
viewHolder.tvName.setText(mDecklist.get(position).getName());
viewHolder.tvCost.setText(mDecklist.get(position).getCost());
viewHolder.tvNumber.setText(mDecklist.get(position).getNumber());
}
public int getItemCount() {
return mDecklist.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCard, ivType;
TextView tvName, tvCost, tvNumber;
public ViewHolder(@NonNull View itemView) {
super(itemView);
ivCard = itemView.findViewById(R.id.ivDecklistCardImage);
ivType = itemView.findViewById(R.id.ivDecklistTypeImage);
tvName = itemView.findViewById(R.id.tvDecklistName);
tvCost = itemView.findViewById(R.id.tvDecklistCost);
tvNumber = itemView.findViewById(R.id.tvDecklistNumber);
}
}
And Lastly please See my Logcat:
2018-11-11 17:46:15.589 21538-21538/com.example.chris.projectartifact E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.chris.projectartifact, PID: 21538
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.support.v7.widget.RecyclerView.setLayoutManager(android.support.v7.widget.RecyclerView$LayoutManager)' on a null object reference
at com.example.chris.projectartifact.b_deckbuilderTap.deckbuilder_RViewAdapter_Card$3.onClick(deckbuilder_RViewAdapter_Card.java:116)
at android.view.View.performClick(View.java:6291)
at android.view.View$PerformClick.run(View.java:24931)
at android.os.Handler.handleCallback(Handler.java:808)
at android.os.Handler.dispatchMessage(Handler.java:101)
at android.os.Looper.loop(Looper.java:166)
at android.app.ActivityThread.main(ActivityThread.java:7425)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:245)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:921)
Requested edits:
Layout Cardview Decklist:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/cardView_deckbuilder_list_id"
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:cardview= "http://schemas.android.com/apk/res-auto"
android:layout_width="150dp"
android:layout_height="20dp"
android:layout_marginTop="5dp"
cardview:cardCornerRadius="5dp"
android:layout_marginStart="5dp"
android:clickable="true"
android:foreground="?android:attr/selectableItemBackground"
>
<android.support.constraint.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
android:orientation="vertical">
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/axe"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<ImageView
android:id="@+id/ivDecklistCardImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:scrollY="-7dp"
android:src="@drawable/thunder_gods_wrath"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/ivDecklistTypeImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/introspells"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list_secound"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list" />
<TextView
android:id="@+id/tvDecklistCost"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_secound"
android:text="20"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_third"/>
<TextView
android:id="@+id/tvDecklistName"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_third"
android:text="Thunder"
android:autoSizeMaxTextSize="20sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_last"/>
<TextView
android:id="@+id/tvDecklistNumber"
android:layout_width="0dp"
android:layout_height="match_parent"
android:text="x3"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffff"
cardview:layout_constraintEnd_toEndOf="parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_last" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="20dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_secound"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="40dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_third"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="55dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_last"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_end="15dp" />
</android.support.constraint.ConstraintLayout>
</android.support.v7.widget.CardView>
and furthermore please find rv_deckbuilder_list at the end of the following layout:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:background="@drawable/background"
>
<LinearLayout
android:id="@+id/llCarddeck"
android:layout_width="0dp"
android:layout_height="60dp"
android:orientation="vertical"
android:layout_marginEnd="10dp"
android:weightSum="6"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintTop_toTopOf="parent"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Rarity"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Category"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1.5"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Order"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="4"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4">
<ToggleButton
android:id="@+id/btnDeckbuilderBasic"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_basic_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderCommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_common_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderUncommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_uncommon_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderRare"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="2.5dp"
android:layout_marginTop="2.5dp"
android:layout_marginEnd="3dp"
android:layout_marginBottom="2.5dp"
android:layout_weight="1"
android:background="@drawable/y_rare_layout" />
<!--<TextView-->
<!--android:id="@+id/textView"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="wrap_content"-->
<!--android:layout_weight="1"-->
<!--android:text="android:layout_weight="4.25"
-->
<!--android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"" />-->
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4"
android:orientation="horizontal"
>
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilderCategory"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilder"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="1.5"
android:background="@drawable/x_linearlayoutcontainer_blank">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center">
<android.support.v7.widget.SwitchCompat
android:id="@+id/switchDeckbuilders"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:checked="false"
android:gravity="center|fill_horizontal"
android:showText="false" />
</FrameLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<!--<android.support.v7.widget.RecyclerView-->
<!--android:id="@+id/rv_deckbuilder_cards"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="0dp"-->
<!--/>-->
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_cards"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginEnd="10dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/llCarddeck"
/>
<LinearLayout
android:id="@+id/llDecklist"
android:layout_width="0dp"
android:layout_height="30dp"
android:background="@drawable/background"
android:orientation="horizontal"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintEnd_toEndOf="parent"/>
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_list"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintTop_toBottomOf="@+id/llDecklist" />
<!--</android.support.v7.widget.RecyclerView>-->
<android.support.constraint.Guideline
android:id="@+id/gl_v_66"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintGuide_percent="0.66"
android:orientation="vertical" />
</android.support.constraint.ConstraintLayout>
Requested Update 2.0:
The first RV which is called rvCards (via deckbuilder_RViewAdapter_Cards) is getting inflated in the following fragment:
public class deckbuilder_fragment extends Fragment {
//..
List<Cards> listCards;
DBHelper dbHelper;
RecyclerView rvCards;
//..
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// return super.onCreateView(inflater, container, savedInstanceState);
View view = inflater.inflate(R.layout.deckbuilder_fragment, container, false);
//..
//...
dbHelper = new DBHelper(getActivity().getApplicationContext());
dbHelper.createDataBase();
rvCards = view.findViewById(R.id.rv_deckbuilder_cards);
updateAdapter();
return view;
}}
Whereas the Adapter is updated in:
public void updateAdapter() {
listCards = dbHelper.getDeckbuilderCards(spinnerDeckbuilderType.getSelectedItem().toString(), true, btnDeckbuilderBasic.isChecked(), btnDeckbuilderCommon.isChecked(), btnDeckbuilderUncommon.isChecked(),btnDeckbuilderRare.isChecked(), spinnerDeckbuilderCategory.getSelectedItem().toString());
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(getActivity(), LinearLayoutManager.HORIZONTAL, false);
rvCards.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_Card newAdapterCards = new deckbuilder_RViewAdapter_Card(getActivity(), listCards);
rvCards.setAdapter(newAdapterCards);
}
java

2
It appears that the problem is with "rvList". What is it and where is it given a value?
– theblitz
Nov 11 at 10:45
@theblitz In my public class Viewholder it is getting initialized.
– Christian.gruener
Nov 11 at 11:08
@theblitz it is getting initalized as RecyclerView rvList; aswell.
– Christian.gruener
Nov 11 at 11:43
add a comment |
I would like to inflate a Recyclerview within a onClickListener Method from a Recyclerview.
For that I know that I have to get the Context from the correspoding activity to set the LinearLayoutManager.
Problem:
Since I am using a Recyclerview in Fragment to try to create another Recyclerview I do not know how to get the correct context.
What I tried:
Use FragmentActivity mContext in my constructor to later on try to get the context via "mContext.getApplicationContext()" (got the FragmentActivity from another post, used Context mContext beforehand)
Issue in the Code:
I will break down my Code as good as possible:
- Find the Code from my first Recyclerview from where I try to initialize the LinearLayoutManager
public class deckbuilder_RViewAdapter_Card extends RecyclerView.Adapter {
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData) {
this.mData = mData;
this.mContext = mContext;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(mContext.getApplicationContext(), LinearLayoutManager.VERTICAL, false);
rvList.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_List addCardAdapter = new deckbuilder_RViewAdapter_List(mContext, listCards);
rvList.setAdapter(addCardAdapter);
}
});
}
public int getItemCount() {
return mData.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCardImage;
TextView tvCardName;
public ViewHolder(@NonNull View introListView) {
super(introListView);
fab_deckbuilder_add = introListView.findViewById(R.id.fab_deckbuilder_add);
fab_deckbuilder_remove = introListView.findViewById(R.id.fab_deckbuilder_remove);
rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
}
}
Code from the Recyclerview I want to initialize:
public class deckbuilder_RViewAdapter_List extends RecyclerView.Adapter {
private List<Decklist> mDecklist;
private Context mContext;
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.layout_cardview_deckbuilder_list, viewGroup, false);
return new deckbuilder_RViewAdapter_List.ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, int position) {
viewHolder.ivCard.setImageResource(mDecklist.get(position).getCardImage());
viewHolder.ivType.setImageResource(mDecklist.get(position).getTypeImage());
viewHolder.tvName.setText(mDecklist.get(position).getName());
viewHolder.tvCost.setText(mDecklist.get(position).getCost());
viewHolder.tvNumber.setText(mDecklist.get(position).getNumber());
}
public int getItemCount() {
return mDecklist.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCard, ivType;
TextView tvName, tvCost, tvNumber;
public ViewHolder(@NonNull View itemView) {
super(itemView);
ivCard = itemView.findViewById(R.id.ivDecklistCardImage);
ivType = itemView.findViewById(R.id.ivDecklistTypeImage);
tvName = itemView.findViewById(R.id.tvDecklistName);
tvCost = itemView.findViewById(R.id.tvDecklistCost);
tvNumber = itemView.findViewById(R.id.tvDecklistNumber);
}
}
And Lastly please See my Logcat:
2018-11-11 17:46:15.589 21538-21538/com.example.chris.projectartifact E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.chris.projectartifact, PID: 21538
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.support.v7.widget.RecyclerView.setLayoutManager(android.support.v7.widget.RecyclerView$LayoutManager)' on a null object reference
at com.example.chris.projectartifact.b_deckbuilderTap.deckbuilder_RViewAdapter_Card$3.onClick(deckbuilder_RViewAdapter_Card.java:116)
at android.view.View.performClick(View.java:6291)
at android.view.View$PerformClick.run(View.java:24931)
at android.os.Handler.handleCallback(Handler.java:808)
at android.os.Handler.dispatchMessage(Handler.java:101)
at android.os.Looper.loop(Looper.java:166)
at android.app.ActivityThread.main(ActivityThread.java:7425)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:245)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:921)
Requested edits:
Layout Cardview Decklist:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/cardView_deckbuilder_list_id"
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:cardview= "http://schemas.android.com/apk/res-auto"
android:layout_width="150dp"
android:layout_height="20dp"
android:layout_marginTop="5dp"
cardview:cardCornerRadius="5dp"
android:layout_marginStart="5dp"
android:clickable="true"
android:foreground="?android:attr/selectableItemBackground"
>
<android.support.constraint.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
android:orientation="vertical">
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/axe"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<ImageView
android:id="@+id/ivDecklistCardImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:scrollY="-7dp"
android:src="@drawable/thunder_gods_wrath"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/ivDecklistTypeImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/introspells"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list_secound"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list" />
<TextView
android:id="@+id/tvDecklistCost"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_secound"
android:text="20"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_third"/>
<TextView
android:id="@+id/tvDecklistName"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_third"
android:text="Thunder"
android:autoSizeMaxTextSize="20sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_last"/>
<TextView
android:id="@+id/tvDecklistNumber"
android:layout_width="0dp"
android:layout_height="match_parent"
android:text="x3"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffff"
cardview:layout_constraintEnd_toEndOf="parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_last" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="20dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_secound"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="40dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_third"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="55dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_last"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_end="15dp" />
</android.support.constraint.ConstraintLayout>
</android.support.v7.widget.CardView>
and furthermore please find rv_deckbuilder_list at the end of the following layout:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:background="@drawable/background"
>
<LinearLayout
android:id="@+id/llCarddeck"
android:layout_width="0dp"
android:layout_height="60dp"
android:orientation="vertical"
android:layout_marginEnd="10dp"
android:weightSum="6"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintTop_toTopOf="parent"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Rarity"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Category"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1.5"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Order"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="4"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4">
<ToggleButton
android:id="@+id/btnDeckbuilderBasic"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_basic_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderCommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_common_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderUncommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_uncommon_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderRare"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="2.5dp"
android:layout_marginTop="2.5dp"
android:layout_marginEnd="3dp"
android:layout_marginBottom="2.5dp"
android:layout_weight="1"
android:background="@drawable/y_rare_layout" />
<!--<TextView-->
<!--android:id="@+id/textView"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="wrap_content"-->
<!--android:layout_weight="1"-->
<!--android:text="android:layout_weight="4.25"
-->
<!--android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"" />-->
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4"
android:orientation="horizontal"
>
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilderCategory"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilder"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="1.5"
android:background="@drawable/x_linearlayoutcontainer_blank">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center">
<android.support.v7.widget.SwitchCompat
android:id="@+id/switchDeckbuilders"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:checked="false"
android:gravity="center|fill_horizontal"
android:showText="false" />
</FrameLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<!--<android.support.v7.widget.RecyclerView-->
<!--android:id="@+id/rv_deckbuilder_cards"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="0dp"-->
<!--/>-->
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_cards"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginEnd="10dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/llCarddeck"
/>
<LinearLayout
android:id="@+id/llDecklist"
android:layout_width="0dp"
android:layout_height="30dp"
android:background="@drawable/background"
android:orientation="horizontal"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintEnd_toEndOf="parent"/>
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_list"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintTop_toBottomOf="@+id/llDecklist" />
<!--</android.support.v7.widget.RecyclerView>-->
<android.support.constraint.Guideline
android:id="@+id/gl_v_66"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintGuide_percent="0.66"
android:orientation="vertical" />
</android.support.constraint.ConstraintLayout>
Requested Update 2.0:
The first RV which is called rvCards (via deckbuilder_RViewAdapter_Cards) is getting inflated in the following fragment:
public class deckbuilder_fragment extends Fragment {
//..
List<Cards> listCards;
DBHelper dbHelper;
RecyclerView rvCards;
//..
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// return super.onCreateView(inflater, container, savedInstanceState);
View view = inflater.inflate(R.layout.deckbuilder_fragment, container, false);
//..
//...
dbHelper = new DBHelper(getActivity().getApplicationContext());
dbHelper.createDataBase();
rvCards = view.findViewById(R.id.rv_deckbuilder_cards);
updateAdapter();
return view;
}}
Whereas the Adapter is updated in:
public void updateAdapter() {
listCards = dbHelper.getDeckbuilderCards(spinnerDeckbuilderType.getSelectedItem().toString(), true, btnDeckbuilderBasic.isChecked(), btnDeckbuilderCommon.isChecked(), btnDeckbuilderUncommon.isChecked(),btnDeckbuilderRare.isChecked(), spinnerDeckbuilderCategory.getSelectedItem().toString());
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(getActivity(), LinearLayoutManager.HORIZONTAL, false);
rvCards.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_Card newAdapterCards = new deckbuilder_RViewAdapter_Card(getActivity(), listCards);
rvCards.setAdapter(newAdapterCards);
}
java

I would like to inflate a Recyclerview within a onClickListener Method from a Recyclerview.
For that I know that I have to get the Context from the correspoding activity to set the LinearLayoutManager.
Problem:
Since I am using a Recyclerview in Fragment to try to create another Recyclerview I do not know how to get the correct context.
What I tried:
Use FragmentActivity mContext in my constructor to later on try to get the context via "mContext.getApplicationContext()" (got the FragmentActivity from another post, used Context mContext beforehand)
Issue in the Code:
I will break down my Code as good as possible:
- Find the Code from my first Recyclerview from where I try to initialize the LinearLayoutManager
public class deckbuilder_RViewAdapter_Card extends RecyclerView.Adapter {
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData) {
this.mData = mData;
this.mContext = mContext;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(mContext.getApplicationContext(), LinearLayoutManager.VERTICAL, false);
rvList.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_List addCardAdapter = new deckbuilder_RViewAdapter_List(mContext, listCards);
rvList.setAdapter(addCardAdapter);
}
});
}
public int getItemCount() {
return mData.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCardImage;
TextView tvCardName;
public ViewHolder(@NonNull View introListView) {
super(introListView);
fab_deckbuilder_add = introListView.findViewById(R.id.fab_deckbuilder_add);
fab_deckbuilder_remove = introListView.findViewById(R.id.fab_deckbuilder_remove);
rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
}
}
Code from the Recyclerview I want to initialize:
public class deckbuilder_RViewAdapter_List extends RecyclerView.Adapter {
private List<Decklist> mDecklist;
private Context mContext;
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.layout_cardview_deckbuilder_list, viewGroup, false);
return new deckbuilder_RViewAdapter_List.ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, int position) {
viewHolder.ivCard.setImageResource(mDecklist.get(position).getCardImage());
viewHolder.ivType.setImageResource(mDecklist.get(position).getTypeImage());
viewHolder.tvName.setText(mDecklist.get(position).getName());
viewHolder.tvCost.setText(mDecklist.get(position).getCost());
viewHolder.tvNumber.setText(mDecklist.get(position).getNumber());
}
public int getItemCount() {
return mDecklist.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
ImageView ivCard, ivType;
TextView tvName, tvCost, tvNumber;
public ViewHolder(@NonNull View itemView) {
super(itemView);
ivCard = itemView.findViewById(R.id.ivDecklistCardImage);
ivType = itemView.findViewById(R.id.ivDecklistTypeImage);
tvName = itemView.findViewById(R.id.tvDecklistName);
tvCost = itemView.findViewById(R.id.tvDecklistCost);
tvNumber = itemView.findViewById(R.id.tvDecklistNumber);
}
}
And Lastly please See my Logcat:
2018-11-11 17:46:15.589 21538-21538/com.example.chris.projectartifact E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.chris.projectartifact, PID: 21538
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.support.v7.widget.RecyclerView.setLayoutManager(android.support.v7.widget.RecyclerView$LayoutManager)' on a null object reference
at com.example.chris.projectartifact.b_deckbuilderTap.deckbuilder_RViewAdapter_Card$3.onClick(deckbuilder_RViewAdapter_Card.java:116)
at android.view.View.performClick(View.java:6291)
at android.view.View$PerformClick.run(View.java:24931)
at android.os.Handler.handleCallback(Handler.java:808)
at android.os.Handler.dispatchMessage(Handler.java:101)
at android.os.Looper.loop(Looper.java:166)
at android.app.ActivityThread.main(ActivityThread.java:7425)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:245)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:921)
Requested edits:
Layout Cardview Decklist:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/cardView_deckbuilder_list_id"
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:cardview= "http://schemas.android.com/apk/res-auto"
android:layout_width="150dp"
android:layout_height="20dp"
android:layout_marginTop="5dp"
cardview:cardCornerRadius="5dp"
android:layout_marginStart="5dp"
android:clickable="true"
android:foreground="?android:attr/selectableItemBackground"
>
<android.support.constraint.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
android:orientation="vertical">
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/axe"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<ImageView
android:id="@+id/ivDecklistCardImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:scrollY="-7dp"
android:src="@drawable/thunder_gods_wrath"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list"
cardview:layout_constraintStart_toStartOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/ivDecklistTypeImage"
android:layout_width="0dp"
android:layout_height="match_parent"
android:src="@drawable/introspells"
cardview:layout_constraintEnd_toStartOf="@id/gl_v_db_list_secound"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list" />
<TextView
android:id="@+id/tvDecklistCost"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_secound"
android:text="20"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_third"/>
<TextView
android:id="@+id/tvDecklistName"
android:layout_width="0dp"
android:layout_height="match_parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_third"
android:text="Thunder"
android:autoSizeMaxTextSize="20sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffffff"
cardview:layout_constraintEnd_toEndOf="@id/gl_v_db_list_last"/>
<TextView
android:id="@+id/tvDecklistNumber"
android:layout_width="0dp"
android:layout_height="match_parent"
android:text="x3"
android:autoSizeMaxTextSize="16sp"
android:autoSizeMinTextSize="8sp"
android:autoSizeTextType="uniform"
android:gravity="center"
android:textColor="#ffff"
cardview:layout_constraintEnd_toEndOf="parent"
cardview:layout_constraintStart_toStartOf="@id/gl_v_db_list_last" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="20dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_secound"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="40dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_third"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_begin="55dp" />
<android.support.constraint.Guideline
android:id="@+id/gl_v_db_list_last"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
cardview:layout_constraintGuide_end="15dp" />
</android.support.constraint.ConstraintLayout>
</android.support.v7.widget.CardView>
and furthermore please find rv_deckbuilder_list at the end of the following layout:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:background="@drawable/background"
>
<LinearLayout
android:id="@+id/llCarddeck"
android:layout_width="0dp"
android:layout_height="60dp"
android:orientation="vertical"
android:layout_marginEnd="10dp"
android:weightSum="6"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintTop_toTopOf="parent"
>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Rarity"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="4.25">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Category"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<TextView
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1.5"
android:gravity="center_horizontal"
android:paddingTop="1dp"
android:text="Order"
android:textColor="#fff"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="4"
android:orientation="horizontal"
android:weightSum="10">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4">
<ToggleButton
android:id="@+id/btnDeckbuilderBasic"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_basic_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderCommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_common_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderUncommon"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:layout_marginTop="3dp"
android:layout_marginBottom="3dp"
android:layout_weight="1"
android:background="@drawable/y_uncommon_layout" />
<ToggleButton
android:id="@+id/btnDeckbuilderRare"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="2.5dp"
android:layout_marginTop="2.5dp"
android:layout_marginEnd="3dp"
android:layout_marginBottom="2.5dp"
android:layout_weight="1"
android:background="@drawable/y_rare_layout" />
<!--<TextView-->
<!--android:id="@+id/textView"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="wrap_content"-->
<!--android:layout_weight="1"-->
<!--android:text="android:layout_weight="4.25"
-->
<!--android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"" />-->
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="4.25"
android:background="@drawable/x_linearlayoutcontainer_blank"
android:weightSum="4"
android:orientation="horizontal"
>
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilderCategory"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="3dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
<android.support.v7.widget.AppCompatSpinner
android:id="@+id/spinnerDeckbuilder"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:autoSizeMaxTextSize="15sp"
android:autoSizeMinTextSize="10sp"
android:autoSizeTextType="uniform"
android:backgroundTint="@color/white"
android:dropDownWidth="match_parent"
android:popupBackground="#8A8A8A"
android:spinnerMode="dropdown"
android:textSize="15sp" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="1dp"
android:layout_marginEnd="1dp"
android:layout_weight="1.5"
android:background="@drawable/x_linearlayoutcontainer_blank">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center">
<android.support.v7.widget.SwitchCompat
android:id="@+id/switchDeckbuilders"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:checked="false"
android:gravity="center|fill_horizontal"
android:showText="false" />
</FrameLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<!--<android.support.v7.widget.RecyclerView-->
<!--android:id="@+id/rv_deckbuilder_cards"-->
<!--android:layout_width="0dp"-->
<!--android:layout_height="0dp"-->
<!--/>-->
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_cards"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginEnd="10dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="@id/gl_v_66"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/llCarddeck"
/>
<LinearLayout
android:id="@+id/llDecklist"
android:layout_width="0dp"
android:layout_height="30dp"
android:background="@drawable/background"
android:orientation="horizontal"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintEnd_toEndOf="parent"/>
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_deckbuilder_list"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@id/gl_v_66"
app:layout_constraintTop_toBottomOf="@+id/llDecklist" />
<!--</android.support.v7.widget.RecyclerView>-->
<android.support.constraint.Guideline
android:id="@+id/gl_v_66"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintGuide_percent="0.66"
android:orientation="vertical" />
</android.support.constraint.ConstraintLayout>
Requested Update 2.0:
The first RV which is called rvCards (via deckbuilder_RViewAdapter_Cards) is getting inflated in the following fragment:
public class deckbuilder_fragment extends Fragment {
//..
List<Cards> listCards;
DBHelper dbHelper;
RecyclerView rvCards;
//..
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// return super.onCreateView(inflater, container, savedInstanceState);
View view = inflater.inflate(R.layout.deckbuilder_fragment, container, false);
//..
//...
dbHelper = new DBHelper(getActivity().getApplicationContext());
dbHelper.createDataBase();
rvCards = view.findViewById(R.id.rv_deckbuilder_cards);
updateAdapter();
return view;
}}
Whereas the Adapter is updated in:
public void updateAdapter() {
listCards = dbHelper.getDeckbuilderCards(spinnerDeckbuilderType.getSelectedItem().toString(), true, btnDeckbuilderBasic.isChecked(), btnDeckbuilderCommon.isChecked(), btnDeckbuilderUncommon.isChecked(),btnDeckbuilderRare.isChecked(), spinnerDeckbuilderCategory.getSelectedItem().toString());
LinearLayoutManager layoutManagerCards = new LinearLayoutManager(getActivity(), LinearLayoutManager.HORIZONTAL, false);
rvCards.setLayoutManager(layoutManagerCards);
deckbuilder_RViewAdapter_Card newAdapterCards = new deckbuilder_RViewAdapter_Card(getActivity(), listCards);
rvCards.setAdapter(newAdapterCards);
}
java

java

edited Nov 12 at 6:11
asked Nov 11 at 10:33
Christian.gruener
258
258
2
It appears that the problem is with "rvList". What is it and where is it given a value?
– theblitz
Nov 11 at 10:45
@theblitz In my public class Viewholder it is getting initialized.
– Christian.gruener
Nov 11 at 11:08
@theblitz it is getting initalized as RecyclerView rvList; aswell.
– Christian.gruener
Nov 11 at 11:43
add a comment |
2
It appears that the problem is with "rvList". What is it and where is it given a value?
– theblitz
Nov 11 at 10:45
@theblitz In my public class Viewholder it is getting initialized.
– Christian.gruener
Nov 11 at 11:08
@theblitz it is getting initalized as RecyclerView rvList; aswell.
– Christian.gruener
Nov 11 at 11:43
2
2
It appears that the problem is with "rvList". What is it and where is it given a value?
– theblitz
Nov 11 at 10:45
It appears that the problem is with "rvList". What is it and where is it given a value?
– theblitz
Nov 11 at 10:45
@theblitz In my public class Viewholder it is getting initialized.
– Christian.gruener
Nov 11 at 11:08
@theblitz In my public class Viewholder it is getting initialized.
– Christian.gruener
Nov 11 at 11:08
@theblitz it is getting initalized as RecyclerView rvList; aswell.
– Christian.gruener
Nov 11 at 11:43
@theblitz it is getting initalized as RecyclerView rvList; aswell.
– Christian.gruener
Nov 11 at 11:43
add a comment |
1 Answer
1
active
oldest
votes
I think you're just getting rvList
from the wrong place, it shouldn't be in your view holder, it should be in your fragment, that's why it's throwing NullPointerException when you click on it.
Firstly, remove rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
from your view holder, because it will only return null.
Next, update your deckbuilder_RViewAdapter_List
adapter so it can support data changes:
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
public void setCards(List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
notifyDataSetChanged();
}
And also update your deckbuilder_RViewAdapter_Card
adapter so it can take in a reference of deckbuilder_RViewAdapter_List
:
private deckbuilder_RViewAdapter_List mCardListAdapter;
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData, deckbuilder_RViewAdapter_List mCardListAdapter) {
this.mData = mData;
this.mContext = mContext;
this.mCardListAdapter = mCardListAdapter;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
mCardListAdapter.setCards(listCards);
}
});
}
And finally, find the RecyclerView
s in your fragment, then attach them with appropriate adapters:
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// ... after inflate view
rvCards = view.findViewById(R.id.rv_deckbuilder_cards)
rvList = view.findViewById(R.id.rv_deckbuilder_list)
deckbuilder_RViewAdapter_List listAdapter = new deckbuilder_RViewAdapter_List(..)
deckbuilder_RViewAdapter_Card cardAdapter = new deckbuilder_RViewAdapter_Card(.., listAdapter)
rvCards.setAdapter(cardAdapter);
rvList.setAdapter(listAdapter);
// ...
}
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I think I know what you're trying to do.. Firstly you certainly do not need these linervList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to passrvList
to the adapter.
– Aaron
Nov 12 at 4:46
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
|
show 11 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53247870%2fandroid-getapplication-in-recyclerviewadapter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you're just getting rvList
from the wrong place, it shouldn't be in your view holder, it should be in your fragment, that's why it's throwing NullPointerException when you click on it.
Firstly, remove rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
from your view holder, because it will only return null.
Next, update your deckbuilder_RViewAdapter_List
adapter so it can support data changes:
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
public void setCards(List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
notifyDataSetChanged();
}
And also update your deckbuilder_RViewAdapter_Card
adapter so it can take in a reference of deckbuilder_RViewAdapter_List
:
private deckbuilder_RViewAdapter_List mCardListAdapter;
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData, deckbuilder_RViewAdapter_List mCardListAdapter) {
this.mData = mData;
this.mContext = mContext;
this.mCardListAdapter = mCardListAdapter;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
mCardListAdapter.setCards(listCards);
}
});
}
And finally, find the RecyclerView
s in your fragment, then attach them with appropriate adapters:
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// ... after inflate view
rvCards = view.findViewById(R.id.rv_deckbuilder_cards)
rvList = view.findViewById(R.id.rv_deckbuilder_list)
deckbuilder_RViewAdapter_List listAdapter = new deckbuilder_RViewAdapter_List(..)
deckbuilder_RViewAdapter_Card cardAdapter = new deckbuilder_RViewAdapter_Card(.., listAdapter)
rvCards.setAdapter(cardAdapter);
rvList.setAdapter(listAdapter);
// ...
}
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I think I know what you're trying to do.. Firstly you certainly do not need these linervList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to passrvList
to the adapter.
– Aaron
Nov 12 at 4:46
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
|
show 11 more comments
I think you're just getting rvList
from the wrong place, it shouldn't be in your view holder, it should be in your fragment, that's why it's throwing NullPointerException when you click on it.
Firstly, remove rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
from your view holder, because it will only return null.
Next, update your deckbuilder_RViewAdapter_List
adapter so it can support data changes:
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
public void setCards(List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
notifyDataSetChanged();
}
And also update your deckbuilder_RViewAdapter_Card
adapter so it can take in a reference of deckbuilder_RViewAdapter_List
:
private deckbuilder_RViewAdapter_List mCardListAdapter;
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData, deckbuilder_RViewAdapter_List mCardListAdapter) {
this.mData = mData;
this.mContext = mContext;
this.mCardListAdapter = mCardListAdapter;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
mCardListAdapter.setCards(listCards);
}
});
}
And finally, find the RecyclerView
s in your fragment, then attach them with appropriate adapters:
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// ... after inflate view
rvCards = view.findViewById(R.id.rv_deckbuilder_cards)
rvList = view.findViewById(R.id.rv_deckbuilder_list)
deckbuilder_RViewAdapter_List listAdapter = new deckbuilder_RViewAdapter_List(..)
deckbuilder_RViewAdapter_Card cardAdapter = new deckbuilder_RViewAdapter_Card(.., listAdapter)
rvCards.setAdapter(cardAdapter);
rvList.setAdapter(listAdapter);
// ...
}
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I think I know what you're trying to do.. Firstly you certainly do not need these linervList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to passrvList
to the adapter.
– Aaron
Nov 12 at 4:46
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
|
show 11 more comments
I think you're just getting rvList
from the wrong place, it shouldn't be in your view holder, it should be in your fragment, that's why it's throwing NullPointerException when you click on it.
Firstly, remove rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
from your view holder, because it will only return null.
Next, update your deckbuilder_RViewAdapter_List
adapter so it can support data changes:
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
public void setCards(List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
notifyDataSetChanged();
}
And also update your deckbuilder_RViewAdapter_Card
adapter so it can take in a reference of deckbuilder_RViewAdapter_List
:
private deckbuilder_RViewAdapter_List mCardListAdapter;
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData, deckbuilder_RViewAdapter_List mCardListAdapter) {
this.mData = mData;
this.mContext = mContext;
this.mCardListAdapter = mCardListAdapter;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
mCardListAdapter.setCards(listCards);
}
});
}
And finally, find the RecyclerView
s in your fragment, then attach them with appropriate adapters:
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// ... after inflate view
rvCards = view.findViewById(R.id.rv_deckbuilder_cards)
rvList = view.findViewById(R.id.rv_deckbuilder_list)
deckbuilder_RViewAdapter_List listAdapter = new deckbuilder_RViewAdapter_List(..)
deckbuilder_RViewAdapter_Card cardAdapter = new deckbuilder_RViewAdapter_Card(.., listAdapter)
rvCards.setAdapter(cardAdapter);
rvList.setAdapter(listAdapter);
// ...
}
I think you're just getting rvList
from the wrong place, it shouldn't be in your view holder, it should be in your fragment, that's why it's throwing NullPointerException when you click on it.
Firstly, remove rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
from your view holder, because it will only return null.
Next, update your deckbuilder_RViewAdapter_List
adapter so it can support data changes:
public deckbuilder_RViewAdapter_List (Context mContext, List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
this.mContext = mContext;
}
public void setCards(List<Decklist> mDecklist) {
this.mDecklist = mDecklist;
notifyDataSetChanged();
}
And also update your deckbuilder_RViewAdapter_Card
adapter so it can take in a reference of deckbuilder_RViewAdapter_List
:
private deckbuilder_RViewAdapter_List mCardListAdapter;
public deckbuilder_RViewAdapter_Card(FragmentActivity mContext, List<Cards> mData, deckbuilder_RViewAdapter_List mCardListAdapter) {
this.mData = mData;
this.mContext = mContext;
this.mCardListAdapter = mCardListAdapter;
}
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, final int position) {
fab_deckbuilder_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Decklist> listCards = new ArrayList<>();
listCards.add(new Decklist( ... );
mCardListAdapter.setCards(listCards);
}
});
}
And finally, find the RecyclerView
s in your fragment, then attach them with appropriate adapters:
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
// ... after inflate view
rvCards = view.findViewById(R.id.rv_deckbuilder_cards)
rvList = view.findViewById(R.id.rv_deckbuilder_list)
deckbuilder_RViewAdapter_List listAdapter = new deckbuilder_RViewAdapter_List(..)
deckbuilder_RViewAdapter_Card cardAdapter = new deckbuilder_RViewAdapter_Card(.., listAdapter)
rvCards.setAdapter(cardAdapter);
rvList.setAdapter(listAdapter);
// ...
}
edited Nov 12 at 7:38
answered Nov 11 at 12:05


Aaron
1,7051212
1,7051212
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I think I know what you're trying to do.. Firstly you certainly do not need these linervList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to passrvList
to the adapter.
– Aaron
Nov 12 at 4:46
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
|
show 11 more comments
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I think I know what you're trying to do.. Firstly you certainly do not need these linervList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to passrvList
to the adapter.
– Aaron
Nov 12 at 4:46
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Hey @Aaron I implemented your suggestion and I still have to same error. I would like to set the LinearLayoutManager onto a new Recyclerview. I thought the place to use findViewById is in the Viewholder of the first Recyclerview, since I am using a Button in the first Recyclerview to call the new Recyclerview... But I still have the same error.. Any other suggestions? Can I help you with something? And anyway, I am new to the concept of a context. Since I thought my View is inside a fragment I need to get the context of the Application. Am I wrong with that?Thank you for your help so far.
– Christian.gruener
Nov 11 at 13:40
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
Okay, could you share you layout_cardview_deckbuilder_list, and also the layout that includes rv_deckbuilder_list?
– Aaron
Nov 11 at 18:30
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I did that. Hopefully that is of help @Aaron. :)
– Christian.gruener
Nov 12 at 4:33
I think I know what you're trying to do.. Firstly you certainly do not need these line
rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to pass rvList
to the adapter.– Aaron
Nov 12 at 4:46
I think I know what you're trying to do.. Firstly you certainly do not need these line
rvList = introListView.findViewById(R.id.rv_deckbuilder_list);
because I don't think it's in the item view. And next, you probably want to pass rvList
to the adapter.– Aaron
Nov 12 at 4:46
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
@Christian.gruener I've updated my answer, hope it works for you.
– Aaron
Nov 12 at 5:06
|
show 11 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53247870%2fandroid-getapplication-in-recyclerviewadapter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eeUvzi,h,Ybxkm7tp RIW6AnLu8BnC6dcgJz0Fx,w,4FEU228YDBuCL2WjwgvFf,EAk
2
It appears that the problem is with "rvList". What is it and where is it given a value?
– theblitz
Nov 11 at 10:45
@theblitz In my public class Viewholder it is getting initialized.
– Christian.gruener
Nov 11 at 11:08
@theblitz it is getting initalized as RecyclerView rvList; aswell.
– Christian.gruener
Nov 11 at 11:43