Setting text of UILabel from notification works on simulator but not physical device
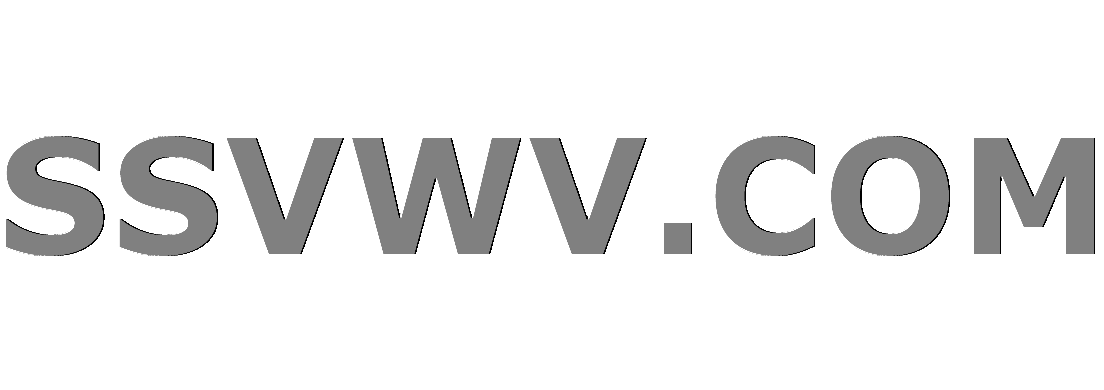
Multi tool use
I have a simple app that updates a UILabel when the user taps on a local push notification. In the simulator when I tap on the notification, applicationDidBecomeActive gets called and in that, I have a shared object referring to the view controller class. Then I call the function in that class that get a new string and sets the UILabel to said string.
This works all the time in the simulator but when I load the app onto my device it works the first couple of times and then stops.
I know the device is updating the string value because when I swap to another view in the app and switch back the UILabel now shows the correctly updated value but not when coming back from a notification.
Has anyone else had this problem where the simulator and physical device behave differently?
As requested here is some code that hopefully helps:
This is from the appDelagate.swift file
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
fetchCurrentUnQuoteFromViewController()
print("applicationDidBecomeActive has finished running.")
}
//Custom functions for the delagate
func setDefaultToTrue() {
readyForNewUnQuote = true
defaults.setValue(readyForNewUnQuote, forKey: "readyForNewUnQuote")
}
func fetchCurrentUnQuoteFromViewController() {
let quoteViewController:QuoteViewController = window!.rootViewController as! QuoteViewController
quoteViewController.getRandomGradient()
quoteViewController.fetchRandomUnQuote()
}
In my view controller here are the functions that are called from the appDelagate
func getRandomGradient() {
let randomNumber: Int = Int.random(in: 0 ... gradientArray.count - 1)
QuoteGradient.image = UIImage(named: gradientArray[randomNumber])
}
func updateUI() {
unQuoteLabel.text = defaults.string(forKey: "currentQuote")
}
Edit: I have basically added viewController.viewDidLoad() as an extra call and that seems to be working. Is this a bad call to make? It seems like a hackish way to fix things.
Ok, I think I've narrowed it down even further. I have a second view which I am trying to use for a settings page. If I just keep on the first view with the quote text then when a notification pops up I can tap it and it will update to the new text that is set.
However, if I go to the settings view and then back to the quote view then when I tap on a notification it doesn't update the text until I go back to the settings page and then back to the quote page again.
I am pretty new to iOS development and don't really know if going to the new view does anything to the shared object I am creating or if it does something else I am not aware of.
Edit 2: Here is the function that fires when a user taps on a notification:
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
setDefaultToTrue()
print(defaults.value(forKey: "readyForNewUnQuote") ?? "Error")
fetchCurrentUnQuoteFromViewController()
print("response from notification was fired.")
completionHandler()
}
ios swift ios-simulator
|
show 3 more comments
I have a simple app that updates a UILabel when the user taps on a local push notification. In the simulator when I tap on the notification, applicationDidBecomeActive gets called and in that, I have a shared object referring to the view controller class. Then I call the function in that class that get a new string and sets the UILabel to said string.
This works all the time in the simulator but when I load the app onto my device it works the first couple of times and then stops.
I know the device is updating the string value because when I swap to another view in the app and switch back the UILabel now shows the correctly updated value but not when coming back from a notification.
Has anyone else had this problem where the simulator and physical device behave differently?
As requested here is some code that hopefully helps:
This is from the appDelagate.swift file
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
fetchCurrentUnQuoteFromViewController()
print("applicationDidBecomeActive has finished running.")
}
//Custom functions for the delagate
func setDefaultToTrue() {
readyForNewUnQuote = true
defaults.setValue(readyForNewUnQuote, forKey: "readyForNewUnQuote")
}
func fetchCurrentUnQuoteFromViewController() {
let quoteViewController:QuoteViewController = window!.rootViewController as! QuoteViewController
quoteViewController.getRandomGradient()
quoteViewController.fetchRandomUnQuote()
}
In my view controller here are the functions that are called from the appDelagate
func getRandomGradient() {
let randomNumber: Int = Int.random(in: 0 ... gradientArray.count - 1)
QuoteGradient.image = UIImage(named: gradientArray[randomNumber])
}
func updateUI() {
unQuoteLabel.text = defaults.string(forKey: "currentQuote")
}
Edit: I have basically added viewController.viewDidLoad() as an extra call and that seems to be working. Is this a bad call to make? It seems like a hackish way to fix things.
Ok, I think I've narrowed it down even further. I have a second view which I am trying to use for a settings page. If I just keep on the first view with the quote text then when a notification pops up I can tap it and it will update to the new text that is set.
However, if I go to the settings view and then back to the quote view then when I tap on a notification it doesn't update the text until I go back to the settings page and then back to the quote page again.
I am pretty new to iOS development and don't really know if going to the new view does anything to the shared object I am creating or if it does something else I am not aware of.
Edit 2: Here is the function that fires when a user taps on a notification:
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
setDefaultToTrue()
print(defaults.value(forKey: "readyForNewUnQuote") ?? "Error")
fetchCurrentUnQuoteFromViewController()
print("response from notification was fired.")
completionHandler()
}
ios swift ios-simulator
You'll have to provide some code. Yes, simulator and physical device do behave differently in some respects.
– Mike Taverne
Nov 16 '18 at 3:18
@MikeTaverne I have updated my question with some code that I hope will help with this investigation.
– Puddinglord
Nov 16 '18 at 3:26
If the app is killed then you might need to check in didFinishLaunching. You can check for the payload there. I am sure there are questions you can check
– agibson007
Nov 16 '18 at 3:32
@agibson007 The app isn't being killed when this is happening. It's just in the background in an inactive state.
– Puddinglord
Nov 16 '18 at 3:33
@Puddinglord where is didReceiveLocalNotification function. You should also handle things there as well.
– agibson007
Nov 16 '18 at 3:43
|
show 3 more comments
I have a simple app that updates a UILabel when the user taps on a local push notification. In the simulator when I tap on the notification, applicationDidBecomeActive gets called and in that, I have a shared object referring to the view controller class. Then I call the function in that class that get a new string and sets the UILabel to said string.
This works all the time in the simulator but when I load the app onto my device it works the first couple of times and then stops.
I know the device is updating the string value because when I swap to another view in the app and switch back the UILabel now shows the correctly updated value but not when coming back from a notification.
Has anyone else had this problem where the simulator and physical device behave differently?
As requested here is some code that hopefully helps:
This is from the appDelagate.swift file
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
fetchCurrentUnQuoteFromViewController()
print("applicationDidBecomeActive has finished running.")
}
//Custom functions for the delagate
func setDefaultToTrue() {
readyForNewUnQuote = true
defaults.setValue(readyForNewUnQuote, forKey: "readyForNewUnQuote")
}
func fetchCurrentUnQuoteFromViewController() {
let quoteViewController:QuoteViewController = window!.rootViewController as! QuoteViewController
quoteViewController.getRandomGradient()
quoteViewController.fetchRandomUnQuote()
}
In my view controller here are the functions that are called from the appDelagate
func getRandomGradient() {
let randomNumber: Int = Int.random(in: 0 ... gradientArray.count - 1)
QuoteGradient.image = UIImage(named: gradientArray[randomNumber])
}
func updateUI() {
unQuoteLabel.text = defaults.string(forKey: "currentQuote")
}
Edit: I have basically added viewController.viewDidLoad() as an extra call and that seems to be working. Is this a bad call to make? It seems like a hackish way to fix things.
Ok, I think I've narrowed it down even further. I have a second view which I am trying to use for a settings page. If I just keep on the first view with the quote text then when a notification pops up I can tap it and it will update to the new text that is set.
However, if I go to the settings view and then back to the quote view then when I tap on a notification it doesn't update the text until I go back to the settings page and then back to the quote page again.
I am pretty new to iOS development and don't really know if going to the new view does anything to the shared object I am creating or if it does something else I am not aware of.
Edit 2: Here is the function that fires when a user taps on a notification:
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
setDefaultToTrue()
print(defaults.value(forKey: "readyForNewUnQuote") ?? "Error")
fetchCurrentUnQuoteFromViewController()
print("response from notification was fired.")
completionHandler()
}
ios swift ios-simulator
I have a simple app that updates a UILabel when the user taps on a local push notification. In the simulator when I tap on the notification, applicationDidBecomeActive gets called and in that, I have a shared object referring to the view controller class. Then I call the function in that class that get a new string and sets the UILabel to said string.
This works all the time in the simulator but when I load the app onto my device it works the first couple of times and then stops.
I know the device is updating the string value because when I swap to another view in the app and switch back the UILabel now shows the correctly updated value but not when coming back from a notification.
Has anyone else had this problem where the simulator and physical device behave differently?
As requested here is some code that hopefully helps:
This is from the appDelagate.swift file
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
fetchCurrentUnQuoteFromViewController()
print("applicationDidBecomeActive has finished running.")
}
//Custom functions for the delagate
func setDefaultToTrue() {
readyForNewUnQuote = true
defaults.setValue(readyForNewUnQuote, forKey: "readyForNewUnQuote")
}
func fetchCurrentUnQuoteFromViewController() {
let quoteViewController:QuoteViewController = window!.rootViewController as! QuoteViewController
quoteViewController.getRandomGradient()
quoteViewController.fetchRandomUnQuote()
}
In my view controller here are the functions that are called from the appDelagate
func getRandomGradient() {
let randomNumber: Int = Int.random(in: 0 ... gradientArray.count - 1)
QuoteGradient.image = UIImage(named: gradientArray[randomNumber])
}
func updateUI() {
unQuoteLabel.text = defaults.string(forKey: "currentQuote")
}
Edit: I have basically added viewController.viewDidLoad() as an extra call and that seems to be working. Is this a bad call to make? It seems like a hackish way to fix things.
Ok, I think I've narrowed it down even further. I have a second view which I am trying to use for a settings page. If I just keep on the first view with the quote text then when a notification pops up I can tap it and it will update to the new text that is set.
However, if I go to the settings view and then back to the quote view then when I tap on a notification it doesn't update the text until I go back to the settings page and then back to the quote page again.
I am pretty new to iOS development and don't really know if going to the new view does anything to the shared object I am creating or if it does something else I am not aware of.
Edit 2: Here is the function that fires when a user taps on a notification:
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
setDefaultToTrue()
print(defaults.value(forKey: "readyForNewUnQuote") ?? "Error")
fetchCurrentUnQuoteFromViewController()
print("response from notification was fired.")
completionHandler()
}
ios swift ios-simulator
ios swift ios-simulator
edited Nov 16 '18 at 13:09
Puddinglord
asked Nov 16 '18 at 3:03
PuddinglordPuddinglord
4810
4810
You'll have to provide some code. Yes, simulator and physical device do behave differently in some respects.
– Mike Taverne
Nov 16 '18 at 3:18
@MikeTaverne I have updated my question with some code that I hope will help with this investigation.
– Puddinglord
Nov 16 '18 at 3:26
If the app is killed then you might need to check in didFinishLaunching. You can check for the payload there. I am sure there are questions you can check
– agibson007
Nov 16 '18 at 3:32
@agibson007 The app isn't being killed when this is happening. It's just in the background in an inactive state.
– Puddinglord
Nov 16 '18 at 3:33
@Puddinglord where is didReceiveLocalNotification function. You should also handle things there as well.
– agibson007
Nov 16 '18 at 3:43
|
show 3 more comments
You'll have to provide some code. Yes, simulator and physical device do behave differently in some respects.
– Mike Taverne
Nov 16 '18 at 3:18
@MikeTaverne I have updated my question with some code that I hope will help with this investigation.
– Puddinglord
Nov 16 '18 at 3:26
If the app is killed then you might need to check in didFinishLaunching. You can check for the payload there. I am sure there are questions you can check
– agibson007
Nov 16 '18 at 3:32
@agibson007 The app isn't being killed when this is happening. It's just in the background in an inactive state.
– Puddinglord
Nov 16 '18 at 3:33
@Puddinglord where is didReceiveLocalNotification function. You should also handle things there as well.
– agibson007
Nov 16 '18 at 3:43
You'll have to provide some code. Yes, simulator and physical device do behave differently in some respects.
– Mike Taverne
Nov 16 '18 at 3:18
You'll have to provide some code. Yes, simulator and physical device do behave differently in some respects.
– Mike Taverne
Nov 16 '18 at 3:18
@MikeTaverne I have updated my question with some code that I hope will help with this investigation.
– Puddinglord
Nov 16 '18 at 3:26
@MikeTaverne I have updated my question with some code that I hope will help with this investigation.
– Puddinglord
Nov 16 '18 at 3:26
If the app is killed then you might need to check in didFinishLaunching. You can check for the payload there. I am sure there are questions you can check
– agibson007
Nov 16 '18 at 3:32
If the app is killed then you might need to check in didFinishLaunching. You can check for the payload there. I am sure there are questions you can check
– agibson007
Nov 16 '18 at 3:32
@agibson007 The app isn't being killed when this is happening. It's just in the background in an inactive state.
– Puddinglord
Nov 16 '18 at 3:33
@agibson007 The app isn't being killed when this is happening. It's just in the background in an inactive state.
– Puddinglord
Nov 16 '18 at 3:33
@Puddinglord where is didReceiveLocalNotification function. You should also handle things there as well.
– agibson007
Nov 16 '18 at 3:43
@Puddinglord where is didReceiveLocalNotification function. You should also handle things there as well.
– agibson007
Nov 16 '18 at 3:43
|
show 3 more comments
2 Answers
2
active
oldest
votes
Give this a go. I have not used local notifications in a while but it appears you can check the delegate for the notification center for a acceptance of the alert.
import UIKit
import UserNotifications
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
let handler = NotificationHandler()
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
handler.appDelegate = self
let center = UNUserNotificationCenter.current()
center.delegate = handler
return true
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
}
class NotificationHandler: NSObject, UNUserNotificationCenterDelegate {
var appDelegate : AppDelegate?
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// Play sound and show alert to the user
completionHandler([.alert,.sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter,
didReceive response: UNNotificationResponse,
withCompletionHandler completionHandler: @escaping () -> Void) {
switch response.actionIdentifier {
case UNNotificationDismissActionIdentifier:
print("Dismiss Action")
case UNNotificationDefaultActionIdentifier:
print("we are launching")
if let app = appDelegate,
let root = app.window?.rootViewController as? ViewController{
print("we have the info")
root.titleLabel.text = response.notification.request.content.title
root.bodyLabel.text = response.notification.request.content.body
}
case "Snooze":
print("Snooze")
case "Delete":
print("Delete")
default:
print("Unknown action")
}
completionHandler()
}
}
import UIKit
import UserNotifications
class ViewController: UIViewController {
let center = UNUserNotificationCenter.current()
lazy var titleLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: 0, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
lazy var bodyLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: titleLabel.frame.maxY, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.addSubview(titleLabel)
titleLabel.center = self.view.center
titleLabel.text = "We are just getting started"
self.view.addSubview(bodyLabel)
let options: UNAuthorizationOptions = [.alert, .sound]
center.requestAuthorization(options: options) {
(granted, error) in
if !granted {
print("Something went wrong")
}
}
DispatchQueue.main.asyncAfter(deadline: DispatchTime.now() + 2.0) {
self.center.removeAllDeliveredNotifications()
self.center.removeAllPendingNotificationRequests()
self.setNotificationWith(title: "Party", contentBody: "Party like it's 1999")
}
}
func setNotificationWith(title:String,contentBody:String){
let content = UNMutableNotificationContent()
content.title = title
content.body = contentBody
content.sound = UNNotificationSound.default
let date = Date(timeIntervalSinceNow: 60)
let triggerDate = Calendar.current.dateComponents([.year,.month,.day,.hour,.minute,.second,], from: date)
let trigger = UNCalendarNotificationTrigger(dateMatching: triggerDate,
repeats: false)
let identifier = "NotificationTest"
let request = UNNotificationRequest(identifier: identifier,
content: content, trigger: trigger)
center.add(request, withCompletionHandler: { (error) in
if let error = error {
// Something went wrong
print("we have an error (error)")
}
})
}
}
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
add a comment |
I have solved my problem! The issue was with the segues I was using in the storyboard.
Instead of using visual segues I changed it so that when I press the settings button I run this function here which loads in a view from the storyboard and then presents it to the user. Then I have another button on the settings page that dismisses the page.
@IBAction func settingsButtonAction(_ sender: UIButton) {
let controller = storyboard?.instantiateViewController(withIdentifier: "SettingsViewController") as! SettingsViewController
present(controller, animated: true, completion: nil)
}
I have no idea why this was causing the issue as I am still new to swift and iOS development but from now on I won't be using the storyboard to present different views to the user. I will only be using the present and dismiss functions for now.
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53330823%2fsetting-text-of-uilabel-from-notification-works-on-simulator-but-not-physical-de%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Give this a go. I have not used local notifications in a while but it appears you can check the delegate for the notification center for a acceptance of the alert.
import UIKit
import UserNotifications
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
let handler = NotificationHandler()
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
handler.appDelegate = self
let center = UNUserNotificationCenter.current()
center.delegate = handler
return true
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
}
class NotificationHandler: NSObject, UNUserNotificationCenterDelegate {
var appDelegate : AppDelegate?
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// Play sound and show alert to the user
completionHandler([.alert,.sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter,
didReceive response: UNNotificationResponse,
withCompletionHandler completionHandler: @escaping () -> Void) {
switch response.actionIdentifier {
case UNNotificationDismissActionIdentifier:
print("Dismiss Action")
case UNNotificationDefaultActionIdentifier:
print("we are launching")
if let app = appDelegate,
let root = app.window?.rootViewController as? ViewController{
print("we have the info")
root.titleLabel.text = response.notification.request.content.title
root.bodyLabel.text = response.notification.request.content.body
}
case "Snooze":
print("Snooze")
case "Delete":
print("Delete")
default:
print("Unknown action")
}
completionHandler()
}
}
import UIKit
import UserNotifications
class ViewController: UIViewController {
let center = UNUserNotificationCenter.current()
lazy var titleLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: 0, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
lazy var bodyLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: titleLabel.frame.maxY, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.addSubview(titleLabel)
titleLabel.center = self.view.center
titleLabel.text = "We are just getting started"
self.view.addSubview(bodyLabel)
let options: UNAuthorizationOptions = [.alert, .sound]
center.requestAuthorization(options: options) {
(granted, error) in
if !granted {
print("Something went wrong")
}
}
DispatchQueue.main.asyncAfter(deadline: DispatchTime.now() + 2.0) {
self.center.removeAllDeliveredNotifications()
self.center.removeAllPendingNotificationRequests()
self.setNotificationWith(title: "Party", contentBody: "Party like it's 1999")
}
}
func setNotificationWith(title:String,contentBody:String){
let content = UNMutableNotificationContent()
content.title = title
content.body = contentBody
content.sound = UNNotificationSound.default
let date = Date(timeIntervalSinceNow: 60)
let triggerDate = Calendar.current.dateComponents([.year,.month,.day,.hour,.minute,.second,], from: date)
let trigger = UNCalendarNotificationTrigger(dateMatching: triggerDate,
repeats: false)
let identifier = "NotificationTest"
let request = UNNotificationRequest(identifier: identifier,
content: content, trigger: trigger)
center.add(request, withCompletionHandler: { (error) in
if let error = error {
// Something went wrong
print("we have an error (error)")
}
})
}
}
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
add a comment |
Give this a go. I have not used local notifications in a while but it appears you can check the delegate for the notification center for a acceptance of the alert.
import UIKit
import UserNotifications
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
let handler = NotificationHandler()
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
handler.appDelegate = self
let center = UNUserNotificationCenter.current()
center.delegate = handler
return true
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
}
class NotificationHandler: NSObject, UNUserNotificationCenterDelegate {
var appDelegate : AppDelegate?
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// Play sound and show alert to the user
completionHandler([.alert,.sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter,
didReceive response: UNNotificationResponse,
withCompletionHandler completionHandler: @escaping () -> Void) {
switch response.actionIdentifier {
case UNNotificationDismissActionIdentifier:
print("Dismiss Action")
case UNNotificationDefaultActionIdentifier:
print("we are launching")
if let app = appDelegate,
let root = app.window?.rootViewController as? ViewController{
print("we have the info")
root.titleLabel.text = response.notification.request.content.title
root.bodyLabel.text = response.notification.request.content.body
}
case "Snooze":
print("Snooze")
case "Delete":
print("Delete")
default:
print("Unknown action")
}
completionHandler()
}
}
import UIKit
import UserNotifications
class ViewController: UIViewController {
let center = UNUserNotificationCenter.current()
lazy var titleLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: 0, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
lazy var bodyLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: titleLabel.frame.maxY, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.addSubview(titleLabel)
titleLabel.center = self.view.center
titleLabel.text = "We are just getting started"
self.view.addSubview(bodyLabel)
let options: UNAuthorizationOptions = [.alert, .sound]
center.requestAuthorization(options: options) {
(granted, error) in
if !granted {
print("Something went wrong")
}
}
DispatchQueue.main.asyncAfter(deadline: DispatchTime.now() + 2.0) {
self.center.removeAllDeliveredNotifications()
self.center.removeAllPendingNotificationRequests()
self.setNotificationWith(title: "Party", contentBody: "Party like it's 1999")
}
}
func setNotificationWith(title:String,contentBody:String){
let content = UNMutableNotificationContent()
content.title = title
content.body = contentBody
content.sound = UNNotificationSound.default
let date = Date(timeIntervalSinceNow: 60)
let triggerDate = Calendar.current.dateComponents([.year,.month,.day,.hour,.minute,.second,], from: date)
let trigger = UNCalendarNotificationTrigger(dateMatching: triggerDate,
repeats: false)
let identifier = "NotificationTest"
let request = UNNotificationRequest(identifier: identifier,
content: content, trigger: trigger)
center.add(request, withCompletionHandler: { (error) in
if let error = error {
// Something went wrong
print("we have an error (error)")
}
})
}
}
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
add a comment |
Give this a go. I have not used local notifications in a while but it appears you can check the delegate for the notification center for a acceptance of the alert.
import UIKit
import UserNotifications
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
let handler = NotificationHandler()
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
handler.appDelegate = self
let center = UNUserNotificationCenter.current()
center.delegate = handler
return true
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
}
class NotificationHandler: NSObject, UNUserNotificationCenterDelegate {
var appDelegate : AppDelegate?
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// Play sound and show alert to the user
completionHandler([.alert,.sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter,
didReceive response: UNNotificationResponse,
withCompletionHandler completionHandler: @escaping () -> Void) {
switch response.actionIdentifier {
case UNNotificationDismissActionIdentifier:
print("Dismiss Action")
case UNNotificationDefaultActionIdentifier:
print("we are launching")
if let app = appDelegate,
let root = app.window?.rootViewController as? ViewController{
print("we have the info")
root.titleLabel.text = response.notification.request.content.title
root.bodyLabel.text = response.notification.request.content.body
}
case "Snooze":
print("Snooze")
case "Delete":
print("Delete")
default:
print("Unknown action")
}
completionHandler()
}
}
import UIKit
import UserNotifications
class ViewController: UIViewController {
let center = UNUserNotificationCenter.current()
lazy var titleLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: 0, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
lazy var bodyLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: titleLabel.frame.maxY, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.addSubview(titleLabel)
titleLabel.center = self.view.center
titleLabel.text = "We are just getting started"
self.view.addSubview(bodyLabel)
let options: UNAuthorizationOptions = [.alert, .sound]
center.requestAuthorization(options: options) {
(granted, error) in
if !granted {
print("Something went wrong")
}
}
DispatchQueue.main.asyncAfter(deadline: DispatchTime.now() + 2.0) {
self.center.removeAllDeliveredNotifications()
self.center.removeAllPendingNotificationRequests()
self.setNotificationWith(title: "Party", contentBody: "Party like it's 1999")
}
}
func setNotificationWith(title:String,contentBody:String){
let content = UNMutableNotificationContent()
content.title = title
content.body = contentBody
content.sound = UNNotificationSound.default
let date = Date(timeIntervalSinceNow: 60)
let triggerDate = Calendar.current.dateComponents([.year,.month,.day,.hour,.minute,.second,], from: date)
let trigger = UNCalendarNotificationTrigger(dateMatching: triggerDate,
repeats: false)
let identifier = "NotificationTest"
let request = UNNotificationRequest(identifier: identifier,
content: content, trigger: trigger)
center.add(request, withCompletionHandler: { (error) in
if let error = error {
// Something went wrong
print("we have an error (error)")
}
})
}
}
Give this a go. I have not used local notifications in a while but it appears you can check the delegate for the notification center for a acceptance of the alert.
import UIKit
import UserNotifications
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
let handler = NotificationHandler()
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
handler.appDelegate = self
let center = UNUserNotificationCenter.current()
center.delegate = handler
return true
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
}
class NotificationHandler: NSObject, UNUserNotificationCenterDelegate {
var appDelegate : AppDelegate?
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// Play sound and show alert to the user
completionHandler([.alert,.sound])
}
func userNotificationCenter(_ center: UNUserNotificationCenter,
didReceive response: UNNotificationResponse,
withCompletionHandler completionHandler: @escaping () -> Void) {
switch response.actionIdentifier {
case UNNotificationDismissActionIdentifier:
print("Dismiss Action")
case UNNotificationDefaultActionIdentifier:
print("we are launching")
if let app = appDelegate,
let root = app.window?.rootViewController as? ViewController{
print("we have the info")
root.titleLabel.text = response.notification.request.content.title
root.bodyLabel.text = response.notification.request.content.body
}
case "Snooze":
print("Snooze")
case "Delete":
print("Delete")
default:
print("Unknown action")
}
completionHandler()
}
}
import UIKit
import UserNotifications
class ViewController: UIViewController {
let center = UNUserNotificationCenter.current()
lazy var titleLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: 0, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
lazy var bodyLabel : UILabel = {
let lbl = UILabel(frame: CGRect(x: 20, y: titleLabel.frame.maxY, width: self.view.bounds.width - 40, height: 50))
lbl.textColor = .black
lbl.font = UIFont.systemFont(ofSize: 20)
lbl.numberOfLines = 0
lbl.textAlignment = .center
return lbl
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.addSubview(titleLabel)
titleLabel.center = self.view.center
titleLabel.text = "We are just getting started"
self.view.addSubview(bodyLabel)
let options: UNAuthorizationOptions = [.alert, .sound]
center.requestAuthorization(options: options) {
(granted, error) in
if !granted {
print("Something went wrong")
}
}
DispatchQueue.main.asyncAfter(deadline: DispatchTime.now() + 2.0) {
self.center.removeAllDeliveredNotifications()
self.center.removeAllPendingNotificationRequests()
self.setNotificationWith(title: "Party", contentBody: "Party like it's 1999")
}
}
func setNotificationWith(title:String,contentBody:String){
let content = UNMutableNotificationContent()
content.title = title
content.body = contentBody
content.sound = UNNotificationSound.default
let date = Date(timeIntervalSinceNow: 60)
let triggerDate = Calendar.current.dateComponents([.year,.month,.day,.hour,.minute,.second,], from: date)
let trigger = UNCalendarNotificationTrigger(dateMatching: triggerDate,
repeats: false)
let identifier = "NotificationTest"
let request = UNNotificationRequest(identifier: identifier,
content: content, trigger: trigger)
center.add(request, withCompletionHandler: { (error) in
if let error = error {
// Something went wrong
print("we have an error (error)")
}
})
}
}
answered Nov 16 '18 at 17:21
agibson007agibson007
2,5332714
2,5332714
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
add a comment |
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I have added the delegate you have provided and now I can see when a user taps on the notification. The problem now is when I swap to a different view which is the settings page and then switch back, now notifications don't update the UILabel in real time anymore. If I switch the view after I tap the notification the UILabel updates but not when the notification is tapped.
– Puddinglord
Nov 16 '18 at 17:36
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I need more info. Are you saying you are looking at the screen and tap on the notification you want to switch the info in the previous screen?
– agibson007
Nov 16 '18 at 17:39
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
I have two views. The main page and the settings page. They are connected by segues. When I start the app I can go home and get a notification and when I tap it the main page will update with a new quote as I want it to. If I start the app and go to the settings page and then go back to the main page, go home and then get a notification, the app will resume to the main page but the UILabel won't b updated. It will now only update if I tap the settings button and then go back to the main view where the quote was.
– Puddinglord
Nov 16 '18 at 17:45
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
Can you show me or tell me exactly where you call the update to the UI?
– agibson007
Nov 16 '18 at 18:04
add a comment |
I have solved my problem! The issue was with the segues I was using in the storyboard.
Instead of using visual segues I changed it so that when I press the settings button I run this function here which loads in a view from the storyboard and then presents it to the user. Then I have another button on the settings page that dismisses the page.
@IBAction func settingsButtonAction(_ sender: UIButton) {
let controller = storyboard?.instantiateViewController(withIdentifier: "SettingsViewController") as! SettingsViewController
present(controller, animated: true, completion: nil)
}
I have no idea why this was causing the issue as I am still new to swift and iOS development but from now on I won't be using the storyboard to present different views to the user. I will only be using the present and dismiss functions for now.
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
add a comment |
I have solved my problem! The issue was with the segues I was using in the storyboard.
Instead of using visual segues I changed it so that when I press the settings button I run this function here which loads in a view from the storyboard and then presents it to the user. Then I have another button on the settings page that dismisses the page.
@IBAction func settingsButtonAction(_ sender: UIButton) {
let controller = storyboard?.instantiateViewController(withIdentifier: "SettingsViewController") as! SettingsViewController
present(controller, animated: true, completion: nil)
}
I have no idea why this was causing the issue as I am still new to swift and iOS development but from now on I won't be using the storyboard to present different views to the user. I will only be using the present and dismiss functions for now.
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
add a comment |
I have solved my problem! The issue was with the segues I was using in the storyboard.
Instead of using visual segues I changed it so that when I press the settings button I run this function here which loads in a view from the storyboard and then presents it to the user. Then I have another button on the settings page that dismisses the page.
@IBAction func settingsButtonAction(_ sender: UIButton) {
let controller = storyboard?.instantiateViewController(withIdentifier: "SettingsViewController") as! SettingsViewController
present(controller, animated: true, completion: nil)
}
I have no idea why this was causing the issue as I am still new to swift and iOS development but from now on I won't be using the storyboard to present different views to the user. I will only be using the present and dismiss functions for now.
I have solved my problem! The issue was with the segues I was using in the storyboard.
Instead of using visual segues I changed it so that when I press the settings button I run this function here which loads in a view from the storyboard and then presents it to the user. Then I have another button on the settings page that dismisses the page.
@IBAction func settingsButtonAction(_ sender: UIButton) {
let controller = storyboard?.instantiateViewController(withIdentifier: "SettingsViewController") as! SettingsViewController
present(controller, animated: true, completion: nil)
}
I have no idea why this was causing the issue as I am still new to swift and iOS development but from now on I won't be using the storyboard to present different views to the user. I will only be using the present and dismiss functions for now.
edited Nov 17 '18 at 1:33
answered Nov 16 '18 at 18:19
PuddinglordPuddinglord
4810
4810
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
add a comment |
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Honestly? This is a public place to ask questions, which I did. Also for people to help answer which you did. It's not my fault that you didn't completely solve my problem. Sorry I didn't give you that green checkmark you so desperately need. I specifically said that I was new to swift and iOS development so yes, my philosophy is bad. But instead of helping me with my philosophy you decided to just be rude about it. I guess thanking you means nothing. Noted pal.
– Puddinglord
Nov 17 '18 at 1:29
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
Well it’s just the question has nothing to do with the answer. No green check mark should be on here. I felt shortness from you in earlier comments. Maybe I read that wrong and text is hard to interpret. I am glad you fixed it. Good luck learning. I can be short when I have been into it for a while so sorry about that. I have been there as well. Storyboards are fine though. You just need to pass the data to a property and update in viewWillAppear or somewhere.
– agibson007
Nov 17 '18 at 1:37
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
I appreciate this I really do thanks. I do agree that the answer is not related to the question. Have a good one!
– Puddinglord
Nov 17 '18 at 2:11
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
Just another tip. I bet you kept using perform segue even for dismissing. When you dismiss you can dismiss a storyboard Vc using a segue the same way as in code. dismissVC or popView depending on how it was presented
– agibson007
Nov 17 '18 at 2:20
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
I was indeed using preform segue for dismissing the settings page as I was unaware of doing it a different way. Thanks for the tip!
– Puddinglord
Nov 17 '18 at 21:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53330823%2fsetting-text-of-uilabel-from-notification-works-on-simulator-but-not-physical-de%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KM3djPEeSEe8qjWx8fwXjPuGl3F nI5z z3XVPU 1vpUa l9tbDm939IilhHz TaC9EX4
You'll have to provide some code. Yes, simulator and physical device do behave differently in some respects.
– Mike Taverne
Nov 16 '18 at 3:18
@MikeTaverne I have updated my question with some code that I hope will help with this investigation.
– Puddinglord
Nov 16 '18 at 3:26
If the app is killed then you might need to check in didFinishLaunching. You can check for the payload there. I am sure there are questions you can check
– agibson007
Nov 16 '18 at 3:32
@agibson007 The app isn't being killed when this is happening. It's just in the background in an inactive state.
– Puddinglord
Nov 16 '18 at 3:33
@Puddinglord where is didReceiveLocalNotification function. You should also handle things there as well.
– agibson007
Nov 16 '18 at 3:43