Delete all occurence of href tag and keep the textes inside
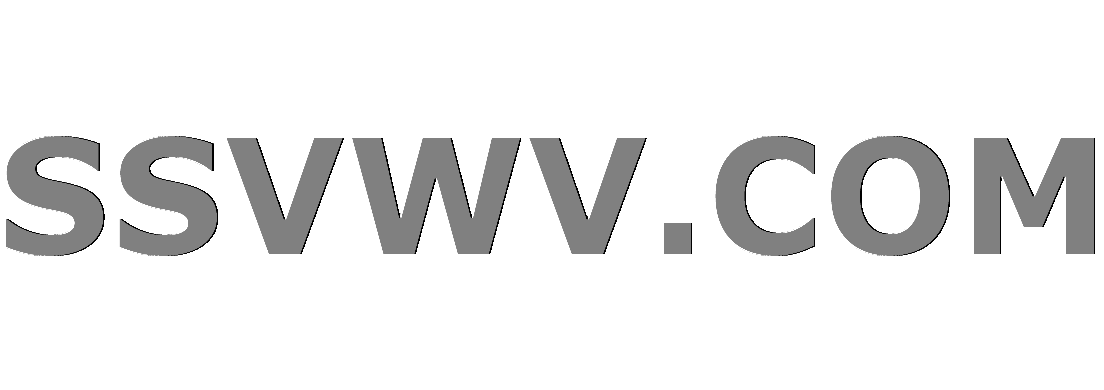
Multi tool use
I have a long String containing a HTML document. I want to delete all the href Tags but keep the text. The following example:
Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text
should become:
Some text example 1 </p> some example 2 text
The solution I found is to get all the textes, and then try to iterate again through the text and replace the tag number n with the text number n.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
var texts = .slice.call(a).map(function(val){
return val.innerHTML;
});
alert(texts);
// TODO ieterate and replace occurence n with texts[n]
Is there a besser way to do this?
javascript
add a comment |
I have a long String containing a HTML document. I want to delete all the href Tags but keep the text. The following example:
Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text
should become:
Some text example 1 </p> some example 2 text
The solution I found is to get all the textes, and then try to iterate again through the text and replace the tag number n with the text number n.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
var texts = .slice.call(a).map(function(val){
return val.innerHTML;
});
alert(texts);
// TODO ieterate and replace occurence n with texts[n]
Is there a besser way to do this?
javascript
Are there ever any tags nested inside the<a>
s? Eg<a href...><img src...>
?
– CertainPerformance
Nov 19 '18 at 10:55
No there is no nested Tags
– Ericc34
Nov 19 '18 at 10:56
1
"Is there a besser way to do this?" — Questions about improving working code belong on codereview.stackexchange.com (make sure you take their tour and learn how to write questions in the right format for that site).
– Quentin
Nov 19 '18 at 10:57
Why is this needed? Is it not just going to be put right back on the page?
– DarkHeart Productions
Nov 19 '18 at 10:58
add a comment |
I have a long String containing a HTML document. I want to delete all the href Tags but keep the text. The following example:
Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text
should become:
Some text example 1 </p> some example 2 text
The solution I found is to get all the textes, and then try to iterate again through the text and replace the tag number n with the text number n.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
var texts = .slice.call(a).map(function(val){
return val.innerHTML;
});
alert(texts);
// TODO ieterate and replace occurence n with texts[n]
Is there a besser way to do this?
javascript
I have a long String containing a HTML document. I want to delete all the href Tags but keep the text. The following example:
Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text
should become:
Some text example 1 </p> some example 2 text
The solution I found is to get all the textes, and then try to iterate again through the text and replace the tag number n with the text number n.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
var texts = .slice.call(a).map(function(val){
return val.innerHTML;
});
alert(texts);
// TODO ieterate and replace occurence n with texts[n]
Is there a besser way to do this?
javascript
javascript
asked Nov 19 '18 at 10:53
Ericc34Ericc34
82
82
Are there ever any tags nested inside the<a>
s? Eg<a href...><img src...>
?
– CertainPerformance
Nov 19 '18 at 10:55
No there is no nested Tags
– Ericc34
Nov 19 '18 at 10:56
1
"Is there a besser way to do this?" — Questions about improving working code belong on codereview.stackexchange.com (make sure you take their tour and learn how to write questions in the right format for that site).
– Quentin
Nov 19 '18 at 10:57
Why is this needed? Is it not just going to be put right back on the page?
– DarkHeart Productions
Nov 19 '18 at 10:58
add a comment |
Are there ever any tags nested inside the<a>
s? Eg<a href...><img src...>
?
– CertainPerformance
Nov 19 '18 at 10:55
No there is no nested Tags
– Ericc34
Nov 19 '18 at 10:56
1
"Is there a besser way to do this?" — Questions about improving working code belong on codereview.stackexchange.com (make sure you take their tour and learn how to write questions in the right format for that site).
– Quentin
Nov 19 '18 at 10:57
Why is this needed? Is it not just going to be put right back on the page?
– DarkHeart Productions
Nov 19 '18 at 10:58
Are there ever any tags nested inside the
<a>
s? Eg <a href...><img src...>
?– CertainPerformance
Nov 19 '18 at 10:55
Are there ever any tags nested inside the
<a>
s? Eg <a href...><img src...>
?– CertainPerformance
Nov 19 '18 at 10:55
No there is no nested Tags
– Ericc34
Nov 19 '18 at 10:56
No there is no nested Tags
– Ericc34
Nov 19 '18 at 10:56
1
1
"Is there a besser way to do this?" — Questions about improving working code belong on codereview.stackexchange.com (make sure you take their tour and learn how to write questions in the right format for that site).
– Quentin
Nov 19 '18 at 10:57
"Is there a besser way to do this?" — Questions about improving working code belong on codereview.stackexchange.com (make sure you take their tour and learn how to write questions in the right format for that site).
– Quentin
Nov 19 '18 at 10:57
Why is this needed? Is it not just going to be put right back on the page?
– DarkHeart Productions
Nov 19 '18 at 10:58
Why is this needed? Is it not just going to be put right back on the page?
– DarkHeart Productions
Nov 19 '18 at 10:58
add a comment |
5 Answers
5
active
oldest
votes
After first line write following code
a_string = a_string.replace(/(<a.*?>)/g,'').replace(/</a>/g,' ');
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This is not working
– Ericc34
Nov 19 '18 at 11:05
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
add a comment |
You can use the following Regex:
var regex = /(<s*a([^>]+)>|</s*as*>)/ig;
var str = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text';
str.replace(regex, ""); //Some text example 1</p> some example 2text
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
Try with.replace(regex, " ");
(space character instead of no character)
– Zim
Nov 19 '18 at 11:09
If you get such output, it can only be because you used an undefined 2nd argument forreplace
. This error is not due to the regular expression.
– Zim
Nov 19 '18 at 11:10
add a comment |
Try below regex:
var a_txt = a_string.replace(/<a[s]+[^>]*?href[s]?=[s"']*(.*?)["']*.*?>/g,"").replace(/</a>/g," ");
add a comment |
Your solution of query-selecting all a-tags isn't actually too bad. Instead of getting the text with map you could just iterate over the list and replace each element with its content. No need for regular expressions:
el.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
also, instead of "parsing" your html by putting it into a p-element, you could use a DomParser:
var parser = new DOMParser();
var doc = parser.parseFromString(a_string, "text/html");
doc.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
add a comment |
as stated in some answer above, your code isn't bad. I avoid the use of regular expression when not necessary.
To finish your code, you neeed to iterate through all A{ELEMENTS}. I am typing from mobile phone. Let me know if you hit an error. Thanks.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
for( var t = a.length - 1; t >=0 ; t-- ){
for(var c = a[t].childNodes.length - 1; c >= 0; c-- ){
if( a[t].nextSibling ){
document.insertBefore( a[t].childNodes[c], a[t].nextSibling );
} else {
a[t].parentNode.appendChild( a[t].childNodes[c]);
}
}
a[t].remove();
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53373064%2fdelete-all-occurence-of-href-tag-and-keep-the-textes-inside%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
After first line write following code
a_string = a_string.replace(/(<a.*?>)/g,'').replace(/</a>/g,' ');
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This is not working
– Ericc34
Nov 19 '18 at 11:05
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
add a comment |
After first line write following code
a_string = a_string.replace(/(<a.*?>)/g,'').replace(/</a>/g,' ');
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This is not working
– Ericc34
Nov 19 '18 at 11:05
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
add a comment |
After first line write following code
a_string = a_string.replace(/(<a.*?>)/g,'').replace(/</a>/g,' ');
After first line write following code
a_string = a_string.replace(/(<a.*?>)/g,'').replace(/</a>/g,' ');
edited Nov 19 '18 at 11:38
answered Nov 19 '18 at 10:59


Kamil KiełczewskiKamil Kiełczewski
11.3k86693
11.3k86693
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This is not working
– Ericc34
Nov 19 '18 at 11:05
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
add a comment |
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This is not working
– Ericc34
Nov 19 '18 at 11:05
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This regular expression does not fulfill the OP requirements as it excludes the <a> tag content.
– Zim
Nov 19 '18 at 11:03
This is not working
– Ericc34
Nov 19 '18 at 11:05
This is not working
– Ericc34
Nov 19 '18 at 11:05
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
If I'm not mistaken, it gives: "Some text </p> some text"
– Zim
Nov 19 '18 at 11:06
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Ericc34 I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:07
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
@Zim you have right, I did not notice it - I update my answer
– Kamil Kiełczewski
Nov 19 '18 at 11:08
add a comment |
You can use the following Regex:
var regex = /(<s*a([^>]+)>|</s*as*>)/ig;
var str = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text';
str.replace(regex, ""); //Some text example 1</p> some example 2text
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
Try with.replace(regex, " ");
(space character instead of no character)
– Zim
Nov 19 '18 at 11:09
If you get such output, it can only be because you used an undefined 2nd argument forreplace
. This error is not due to the regular expression.
– Zim
Nov 19 '18 at 11:10
add a comment |
You can use the following Regex:
var regex = /(<s*a([^>]+)>|</s*as*>)/ig;
var str = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text';
str.replace(regex, ""); //Some text example 1</p> some example 2text
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
Try with.replace(regex, " ");
(space character instead of no character)
– Zim
Nov 19 '18 at 11:09
If you get such output, it can only be because you used an undefined 2nd argument forreplace
. This error is not due to the regular expression.
– Zim
Nov 19 '18 at 11:10
add a comment |
You can use the following Regex:
var regex = /(<s*a([^>]+)>|</s*as*>)/ig;
var str = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text';
str.replace(regex, ""); //Some text example 1</p> some example 2text
You can use the following Regex:
var regex = /(<s*a([^>]+)>|</s*as*>)/ig;
var str = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text';
str.replace(regex, ""); //Some text example 1</p> some example 2text
answered Nov 19 '18 at 10:58


ZimZim
1,0741817
1,0741817
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
Try with.replace(regex, " ");
(space character instead of no character)
– Zim
Nov 19 '18 at 11:09
If you get such output, it can only be because you used an undefined 2nd argument forreplace
. This error is not due to the regular expression.
– Zim
Nov 19 '18 at 11:10
add a comment |
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
Try with.replace(regex, " ");
(space character instead of no character)
– Zim
Nov 19 '18 at 11:09
If you get such output, it can only be because you used an undefined 2nd argument forreplace
. This error is not due to the regular expression.
– Zim
Nov 19 '18 at 11:10
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
I get Some text undefinedexample 1undefined</p> some undefinedexampleundefined 2text as result
– Ericc34
Nov 19 '18 at 11:06
Try with
.replace(regex, " ");
(space character instead of no character)– Zim
Nov 19 '18 at 11:09
Try with
.replace(regex, " ");
(space character instead of no character)– Zim
Nov 19 '18 at 11:09
If you get such output, it can only be because you used an undefined 2nd argument for
replace
. This error is not due to the regular expression.– Zim
Nov 19 '18 at 11:10
If you get such output, it can only be because you used an undefined 2nd argument for
replace
. This error is not due to the regular expression.– Zim
Nov 19 '18 at 11:10
add a comment |
Try below regex:
var a_txt = a_string.replace(/<a[s]+[^>]*?href[s]?=[s"']*(.*?)["']*.*?>/g,"").replace(/</a>/g," ");
add a comment |
Try below regex:
var a_txt = a_string.replace(/<a[s]+[^>]*?href[s]?=[s"']*(.*?)["']*.*?>/g,"").replace(/</a>/g," ");
add a comment |
Try below regex:
var a_txt = a_string.replace(/<a[s]+[^>]*?href[s]?=[s"']*(.*?)["']*.*?>/g,"").replace(/</a>/g," ");
Try below regex:
var a_txt = a_string.replace(/<a[s]+[^>]*?href[s]?=[s"']*(.*?)["']*.*?>/g,"").replace(/</a>/g," ");
edited Nov 19 '18 at 11:59
answered Nov 19 '18 at 11:45
user3392782user3392782
1614
1614
add a comment |
add a comment |
Your solution of query-selecting all a-tags isn't actually too bad. Instead of getting the text with map you could just iterate over the list and replace each element with its content. No need for regular expressions:
el.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
also, instead of "parsing" your html by putting it into a p-element, you could use a DomParser:
var parser = new DOMParser();
var doc = parser.parseFromString(a_string, "text/html");
doc.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
add a comment |
Your solution of query-selecting all a-tags isn't actually too bad. Instead of getting the text with map you could just iterate over the list and replace each element with its content. No need for regular expressions:
el.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
also, instead of "parsing" your html by putting it into a p-element, you could use a DomParser:
var parser = new DOMParser();
var doc = parser.parseFromString(a_string, "text/html");
doc.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
add a comment |
Your solution of query-selecting all a-tags isn't actually too bad. Instead of getting the text with map you could just iterate over the list and replace each element with its content. No need for regular expressions:
el.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
also, instead of "parsing" your html by putting it into a p-element, you could use a DomParser:
var parser = new DOMParser();
var doc = parser.parseFromString(a_string, "text/html");
doc.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
Your solution of query-selecting all a-tags isn't actually too bad. Instead of getting the text with map you could just iterate over the list and replace each element with its content. No need for regular expressions:
el.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
also, instead of "parsing" your html by putting it into a p-element, you could use a DomParser:
var parser = new DOMParser();
var doc = parser.parseFromString(a_string, "text/html");
doc.querySelectorAll('a').forEach(function( a_el ){
var text = document.createTextNode(a_el.innerText);
a_el.parentNode.replaceChild(text, a_el);
});
answered Nov 19 '18 at 12:25


FitziFitzi
38029
38029
add a comment |
add a comment |
as stated in some answer above, your code isn't bad. I avoid the use of regular expression when not necessary.
To finish your code, you neeed to iterate through all A{ELEMENTS}. I am typing from mobile phone. Let me know if you hit an error. Thanks.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
for( var t = a.length - 1; t >=0 ; t-- ){
for(var c = a[t].childNodes.length - 1; c >= 0; c-- ){
if( a[t].nextSibling ){
document.insertBefore( a[t].childNodes[c], a[t].nextSibling );
} else {
a[t].parentNode.appendChild( a[t].childNodes[c]);
}
}
a[t].remove();
}
add a comment |
as stated in some answer above, your code isn't bad. I avoid the use of regular expression when not necessary.
To finish your code, you neeed to iterate through all A{ELEMENTS}. I am typing from mobile phone. Let me know if you hit an error. Thanks.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
for( var t = a.length - 1; t >=0 ; t-- ){
for(var c = a[t].childNodes.length - 1; c >= 0; c-- ){
if( a[t].nextSibling ){
document.insertBefore( a[t].childNodes[c], a[t].nextSibling );
} else {
a[t].parentNode.appendChild( a[t].childNodes[c]);
}
}
a[t].remove();
}
add a comment |
as stated in some answer above, your code isn't bad. I avoid the use of regular expression when not necessary.
To finish your code, you neeed to iterate through all A{ELEMENTS}. I am typing from mobile phone. Let me know if you hit an error. Thanks.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
for( var t = a.length - 1; t >=0 ; t-- ){
for(var c = a[t].childNodes.length - 1; c >= 0; c-- ){
if( a[t].nextSibling ){
document.insertBefore( a[t].childNodes[c], a[t].nextSibling );
} else {
a[t].parentNode.appendChild( a[t].childNodes[c]);
}
}
a[t].remove();
}
as stated in some answer above, your code isn't bad. I avoid the use of regular expression when not necessary.
To finish your code, you neeed to iterate through all A{ELEMENTS}. I am typing from mobile phone. Let me know if you hit an error. Thanks.
var a_string = 'Some text <a href="mailto:mail@example.com">example 1</a></p> some <a href="www.example2.com"> example 2</a>text',
el = document.createElement('p');
el.innerHTML = a_string;
var a = el.querySelectorAll('a');
for( var t = a.length - 1; t >=0 ; t-- ){
for(var c = a[t].childNodes.length - 1; c >= 0; c-- ){
if( a[t].nextSibling ){
document.insertBefore( a[t].childNodes[c], a[t].nextSibling );
} else {
a[t].parentNode.appendChild( a[t].childNodes[c]);
}
}
a[t].remove();
}
answered Nov 19 '18 at 13:36


Edwin Dijas ChiwonaEdwin Dijas Chiwona
35118
35118
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53373064%2fdelete-all-occurence-of-href-tag-and-keep-the-textes-inside%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ojkh1,8R n,qhNT6 NWUYQOGAIjVfxjWoqBvPN0iIZhxCq18DuB 8SlhQCgC,OqwI5nMmgoB,EkqjL Hj PhmqWYQS9LRqp8EE
Are there ever any tags nested inside the
<a>
s? Eg<a href...><img src...>
?– CertainPerformance
Nov 19 '18 at 10:55
No there is no nested Tags
– Ericc34
Nov 19 '18 at 10:56
1
"Is there a besser way to do this?" — Questions about improving working code belong on codereview.stackexchange.com (make sure you take their tour and learn how to write questions in the right format for that site).
– Quentin
Nov 19 '18 at 10:57
Why is this needed? Is it not just going to be put right back on the page?
– DarkHeart Productions
Nov 19 '18 at 10:58