adding functions to a knex/bookshelf model in node/express
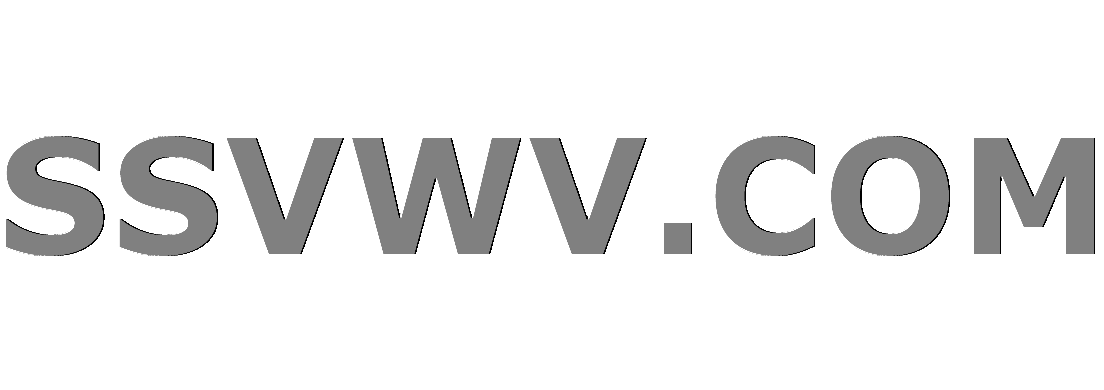
Multi tool use
up vote
0
down vote
favorite
I have a model like this:
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
module.exports = User;
With this I can do things like
const User = require("../models/user");
User.fetchAll({columns:['id','email']}).then((data) => {
res.json(data);
}).catch(err => {
res.json(err);
});
What if I want to add costume functions to my model such as:
var connection = require('./dbconnection.js');
var User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
User.getUsers = (callback) => {
if (connection) {
const newLocal = "select * FROM users";
connection.query(newLocal, (err,rows,fields) => {
if (err) {
return res.sendStatus(500);
} else {
callback(null,rows);
}
});
}
};
module.exports = User;
And then do something like:
const User = require("../models/user");
User.getUsers((err,data) => {
res.status(200).json(data);
});
Is this posible? Or should I just conform with the bookshelf functions?
Right now the error I get is connection.query is not a function
And models/dbconnection.js is:
const mysql = require('mysql');
port = process.env.PORT || 3333;
if (port == 3333) {
var connection = mysql.createConnection({
host: process.env.DB_HOST,
port: process.env.DB_PORT,
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
database: process.env.DB_NAME,
insecureAuth: true
});
} else {
console.log("Error");
}
connection.connect();
module.exports.connection = connection;
node.js express knex.js bookshelf.js
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I have a model like this:
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
module.exports = User;
With this I can do things like
const User = require("../models/user");
User.fetchAll({columns:['id','email']}).then((data) => {
res.json(data);
}).catch(err => {
res.json(err);
});
What if I want to add costume functions to my model such as:
var connection = require('./dbconnection.js');
var User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
User.getUsers = (callback) => {
if (connection) {
const newLocal = "select * FROM users";
connection.query(newLocal, (err,rows,fields) => {
if (err) {
return res.sendStatus(500);
} else {
callback(null,rows);
}
});
}
};
module.exports = User;
And then do something like:
const User = require("../models/user");
User.getUsers((err,data) => {
res.status(200).json(data);
});
Is this posible? Or should I just conform with the bookshelf functions?
Right now the error I get is connection.query is not a function
And models/dbconnection.js is:
const mysql = require('mysql');
port = process.env.PORT || 3333;
if (port == 3333) {
var connection = mysql.createConnection({
host: process.env.DB_HOST,
port: process.env.DB_PORT,
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
database: process.env.DB_NAME,
insecureAuth: true
});
} else {
console.log("Error");
}
connection.connect();
module.exports.connection = connection;
node.js express knex.js bookshelf.js
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a model like this:
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
module.exports = User;
With this I can do things like
const User = require("../models/user");
User.fetchAll({columns:['id','email']}).then((data) => {
res.json(data);
}).catch(err => {
res.json(err);
});
What if I want to add costume functions to my model such as:
var connection = require('./dbconnection.js');
var User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
User.getUsers = (callback) => {
if (connection) {
const newLocal = "select * FROM users";
connection.query(newLocal, (err,rows,fields) => {
if (err) {
return res.sendStatus(500);
} else {
callback(null,rows);
}
});
}
};
module.exports = User;
And then do something like:
const User = require("../models/user");
User.getUsers((err,data) => {
res.status(200).json(data);
});
Is this posible? Or should I just conform with the bookshelf functions?
Right now the error I get is connection.query is not a function
And models/dbconnection.js is:
const mysql = require('mysql');
port = process.env.PORT || 3333;
if (port == 3333) {
var connection = mysql.createConnection({
host: process.env.DB_HOST,
port: process.env.DB_PORT,
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
database: process.env.DB_NAME,
insecureAuth: true
});
} else {
console.log("Error");
}
connection.connect();
module.exports.connection = connection;
node.js express knex.js bookshelf.js
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I have a model like this:
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
module.exports = User;
With this I can do things like
const User = require("../models/user");
User.fetchAll({columns:['id','email']}).then((data) => {
res.json(data);
}).catch(err => {
res.json(err);
});
What if I want to add costume functions to my model such as:
var connection = require('./dbconnection.js');
var User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
});
User.getUsers = (callback) => {
if (connection) {
const newLocal = "select * FROM users";
connection.query(newLocal, (err,rows,fields) => {
if (err) {
return res.sendStatus(500);
} else {
callback(null,rows);
}
});
}
};
module.exports = User;
And then do something like:
const User = require("../models/user");
User.getUsers((err,data) => {
res.status(200).json(data);
});
Is this posible? Or should I just conform with the bookshelf functions?
Right now the error I get is connection.query is not a function
And models/dbconnection.js is:
const mysql = require('mysql');
port = process.env.PORT || 3333;
if (port == 3333) {
var connection = mysql.createConnection({
host: process.env.DB_HOST,
port: process.env.DB_PORT,
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
database: process.env.DB_NAME,
insecureAuth: true
});
} else {
console.log("Error");
}
connection.connect();
module.exports.connection = connection;
node.js express knex.js bookshelf.js
node.js express knex.js bookshelf.js
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Nov 5 at 18:00
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 5 at 17:46


daniel gon
264
264
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
daniel gon is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Yes, you can add your custom functions to bookshelf models in two different ways.
- Instance methods
For example, you want to return user's full name, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true,
//be careful here, if you use arrow function `this` context will change
//and your function won't work as expected
returnFullName: function() {
return this.get('firstname') + this.get('lastname');
}
});
module.exports = User;
then you will call this function like this
User.forge().where({id: SOME_ID}).fetch()
.then(function(user) {
var fullName = user.returnFullName();
});
- Class methods
For example, you want to return user's email based on username, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
}, {
getUserEmail: function(SOME_USERNAME) {
return User.forge().where({username: SOME_USERNAME}).fetch()
.then(function (user) {
if(user) {
return Promise.resolve(user.get('email'));
} else {
return Promise.resolve(null);
}
});
}
});
module.exports = User;
then you can call this function like
User.getUserEmail(SOME_USERNAME)
.then(function(email) {
console.log(email);
});
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Yes, you can add your custom functions to bookshelf models in two different ways.
- Instance methods
For example, you want to return user's full name, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true,
//be careful here, if you use arrow function `this` context will change
//and your function won't work as expected
returnFullName: function() {
return this.get('firstname') + this.get('lastname');
}
});
module.exports = User;
then you will call this function like this
User.forge().where({id: SOME_ID}).fetch()
.then(function(user) {
var fullName = user.returnFullName();
});
- Class methods
For example, you want to return user's email based on username, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
}, {
getUserEmail: function(SOME_USERNAME) {
return User.forge().where({username: SOME_USERNAME}).fetch()
.then(function (user) {
if(user) {
return Promise.resolve(user.get('email'));
} else {
return Promise.resolve(null);
}
});
}
});
module.exports = User;
then you can call this function like
User.getUserEmail(SOME_USERNAME)
.then(function(email) {
console.log(email);
});
add a comment |
up vote
0
down vote
accepted
Yes, you can add your custom functions to bookshelf models in two different ways.
- Instance methods
For example, you want to return user's full name, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true,
//be careful here, if you use arrow function `this` context will change
//and your function won't work as expected
returnFullName: function() {
return this.get('firstname') + this.get('lastname');
}
});
module.exports = User;
then you will call this function like this
User.forge().where({id: SOME_ID}).fetch()
.then(function(user) {
var fullName = user.returnFullName();
});
- Class methods
For example, you want to return user's email based on username, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
}, {
getUserEmail: function(SOME_USERNAME) {
return User.forge().where({username: SOME_USERNAME}).fetch()
.then(function (user) {
if(user) {
return Promise.resolve(user.get('email'));
} else {
return Promise.resolve(null);
}
});
}
});
module.exports = User;
then you can call this function like
User.getUserEmail(SOME_USERNAME)
.then(function(email) {
console.log(email);
});
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Yes, you can add your custom functions to bookshelf models in two different ways.
- Instance methods
For example, you want to return user's full name, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true,
//be careful here, if you use arrow function `this` context will change
//and your function won't work as expected
returnFullName: function() {
return this.get('firstname') + this.get('lastname');
}
});
module.exports = User;
then you will call this function like this
User.forge().where({id: SOME_ID}).fetch()
.then(function(user) {
var fullName = user.returnFullName();
});
- Class methods
For example, you want to return user's email based on username, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
}, {
getUserEmail: function(SOME_USERNAME) {
return User.forge().where({username: SOME_USERNAME}).fetch()
.then(function (user) {
if(user) {
return Promise.resolve(user.get('email'));
} else {
return Promise.resolve(null);
}
});
}
});
module.exports = User;
then you can call this function like
User.getUserEmail(SOME_USERNAME)
.then(function(email) {
console.log(email);
});
Yes, you can add your custom functions to bookshelf models in two different ways.
- Instance methods
For example, you want to return user's full name, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true,
//be careful here, if you use arrow function `this` context will change
//and your function won't work as expected
returnFullName: function() {
return this.get('firstname') + this.get('lastname');
}
});
module.exports = User;
then you will call this function like this
User.forge().where({id: SOME_ID}).fetch()
.then(function(user) {
var fullName = user.returnFullName();
});
- Class methods
For example, you want to return user's email based on username, your User model will look something like this
const User = db.Model.extend({
tableName: 'users',
hasSecurePassword: true
}, {
getUserEmail: function(SOME_USERNAME) {
return User.forge().where({username: SOME_USERNAME}).fetch()
.then(function (user) {
if(user) {
return Promise.resolve(user.get('email'));
} else {
return Promise.resolve(null);
}
});
}
});
module.exports = User;
then you can call this function like
User.getUserEmail(SOME_USERNAME)
.then(function(email) {
console.log(email);
});
answered Nov 7 at 0:09
zerosand1s
455313
455313
add a comment |
add a comment |
daniel gon is a new contributor. Be nice, and check out our Code of Conduct.
daniel gon is a new contributor. Be nice, and check out our Code of Conduct.
daniel gon is a new contributor. Be nice, and check out our Code of Conduct.
daniel gon is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53159554%2fadding-functions-to-a-knex-bookshelf-model-in-node-express%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
v,uXJUhlYccLRnLqTRgpVS2NPHGG