Can withStyles be used on the result of a function?
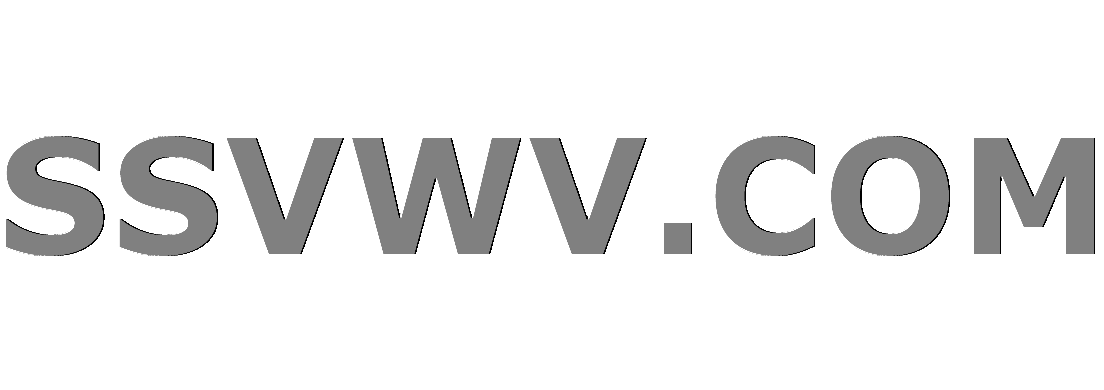
Multi tool use
up vote
1
down vote
favorite
I'm currently trying to apply additional styling to the result of a function that returns one of two components depending on the presence of a specific set of props, as such:
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const DefaultImage = withStyles({
root: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
})(AccountCircle)
export default function UserAvatar(props) {
return props.userImage ? <Avatar src={props.userImage} /> : <DefaultImage />
}
However, when I apply withStyles to the result, none of the additional styling appears. Am I approaching this the right way?
import React from 'react'
import UserAvatar from './avatar.js'
import { withStyles } from '@material-ui/core/styles'
const UserAvatarHeader = withStyles({
root: {
margin: '2vh',
float: 'right'
}
})(UserAvatar)
export default function PageHeader(props) {
return (
...
<UserAvatarHeader userImage={props.userImage} />
...
)
}
javascript css reactjs material-ui
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
favorite
I'm currently trying to apply additional styling to the result of a function that returns one of two components depending on the presence of a specific set of props, as such:
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const DefaultImage = withStyles({
root: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
})(AccountCircle)
export default function UserAvatar(props) {
return props.userImage ? <Avatar src={props.userImage} /> : <DefaultImage />
}
However, when I apply withStyles to the result, none of the additional styling appears. Am I approaching this the right way?
import React from 'react'
import UserAvatar from './avatar.js'
import { withStyles } from '@material-ui/core/styles'
const UserAvatarHeader = withStyles({
root: {
margin: '2vh',
float: 'right'
}
})(UserAvatar)
export default function PageHeader(props) {
return (
...
<UserAvatarHeader userImage={props.userImage} />
...
)
}
javascript css reactjs material-ui
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm currently trying to apply additional styling to the result of a function that returns one of two components depending on the presence of a specific set of props, as such:
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const DefaultImage = withStyles({
root: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
})(AccountCircle)
export default function UserAvatar(props) {
return props.userImage ? <Avatar src={props.userImage} /> : <DefaultImage />
}
However, when I apply withStyles to the result, none of the additional styling appears. Am I approaching this the right way?
import React from 'react'
import UserAvatar from './avatar.js'
import { withStyles } from '@material-ui/core/styles'
const UserAvatarHeader = withStyles({
root: {
margin: '2vh',
float: 'right'
}
})(UserAvatar)
export default function PageHeader(props) {
return (
...
<UserAvatarHeader userImage={props.userImage} />
...
)
}
javascript css reactjs material-ui
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I'm currently trying to apply additional styling to the result of a function that returns one of two components depending on the presence of a specific set of props, as such:
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const DefaultImage = withStyles({
root: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
})(AccountCircle)
export default function UserAvatar(props) {
return props.userImage ? <Avatar src={props.userImage} /> : <DefaultImage />
}
However, when I apply withStyles to the result, none of the additional styling appears. Am I approaching this the right way?
import React from 'react'
import UserAvatar from './avatar.js'
import { withStyles } from '@material-ui/core/styles'
const UserAvatarHeader = withStyles({
root: {
margin: '2vh',
float: 'right'
}
})(UserAvatar)
export default function PageHeader(props) {
return (
...
<UserAvatarHeader userImage={props.userImage} />
...
)
}
javascript css reactjs material-ui
javascript css reactjs material-ui
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 5 at 2:06
jjnotjayjay
61
61
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
jjnotjayjay is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
try this way
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const styles = {
default: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
}
function UserAvatar(props) {
const { classes, userImage } = props;
return userImage ? <Avatar src={userImage} /> : <AccountCircle className={classes.default} />
}
export default withStyles(styles)(UserAvatar)
I found this from the documentation: https://material-ui.com/style/icons/
please let me know if you have any further questions
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
try this way
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const styles = {
default: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
}
function UserAvatar(props) {
const { classes, userImage } = props;
return userImage ? <Avatar src={userImage} /> : <AccountCircle className={classes.default} />
}
export default withStyles(styles)(UserAvatar)
I found this from the documentation: https://material-ui.com/style/icons/
please let me know if you have any further questions
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
add a comment |
up vote
0
down vote
try this way
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const styles = {
default: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
}
function UserAvatar(props) {
const { classes, userImage } = props;
return userImage ? <Avatar src={userImage} /> : <AccountCircle className={classes.default} />
}
export default withStyles(styles)(UserAvatar)
I found this from the documentation: https://material-ui.com/style/icons/
please let me know if you have any further questions
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
add a comment |
up vote
0
down vote
up vote
0
down vote
try this way
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const styles = {
default: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
}
function UserAvatar(props) {
const { classes, userImage } = props;
return userImage ? <Avatar src={userImage} /> : <AccountCircle className={classes.default} />
}
export default withStyles(styles)(UserAvatar)
I found this from the documentation: https://material-ui.com/style/icons/
please let me know if you have any further questions
try this way
import React from 'react'
import { withStyles } from '@material-ui/core/styles'
import Avatar from '@material-ui/core/Avatar'
import AccountCircle from '@material-ui/icons/AccountCircle'
const styles = {
default: {
backgroundColor: 'rgba(255, 255, 255, 0.5)',
width: '40px',
height: '40px',
borderRadius: '50%'
}
}
function UserAvatar(props) {
const { classes, userImage } = props;
return userImage ? <Avatar src={userImage} /> : <AccountCircle className={classes.default} />
}
export default withStyles(styles)(UserAvatar)
I found this from the documentation: https://material-ui.com/style/icons/
please let me know if you have any further questions
answered Nov 5 at 13:19
Nadun
978610
978610
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
add a comment |
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
Thanks for responding. However, I'm trying to use the result of UserAvatar without additional margin styling in some situations, and only apply the addtl. margin styling in others (UserAvatarHeader). It appears your solution would apply the styling every time UserAvatar is rendered.
– jjnotjayjay
Nov 5 at 18:17
add a comment |
jjnotjayjay is a new contributor. Be nice, and check out our Code of Conduct.
jjnotjayjay is a new contributor. Be nice, and check out our Code of Conduct.
jjnotjayjay is a new contributor. Be nice, and check out our Code of Conduct.
jjnotjayjay is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53147407%2fcan-withstyles-be-used-on-the-result-of-a-function%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
peJoHC,Dys,hj6h66W7A6ux3FidUD