How to use variables in a GraphQL query?
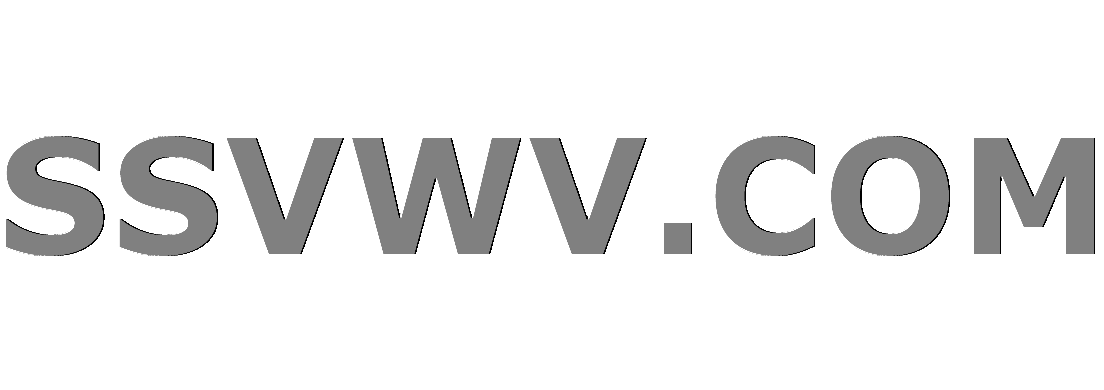
Multi tool use
up vote
1
down vote
favorite
So I have to make two queries. First will return an array with multiple objects and I want to get the ID of the first object to use it in my second query.
The problem is that I can't use b_id: props.getBusiness.business[0]._id
Any idea how can I work with this?
const GET_USER_BUSINESS = gql`
query getUserBusiness {
getUserBusiness {
_id
}
}
`;
const GET_BUSINESS_JOBS = gql`
query getBusinessJobs($b_id: ID!) {
getBusinessJobs(b_id: $b_id ) {
_id
name
}
}
`;
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
options: (props) => (
{
variables:
{
b_id: props.getUserBusiness.business[0].b_id
}
}
)
})
)(ProposalContainer);
reactjs react-native graphql
add a comment |
up vote
1
down vote
favorite
So I have to make two queries. First will return an array with multiple objects and I want to get the ID of the first object to use it in my second query.
The problem is that I can't use b_id: props.getBusiness.business[0]._id
Any idea how can I work with this?
const GET_USER_BUSINESS = gql`
query getUserBusiness {
getUserBusiness {
_id
}
}
`;
const GET_BUSINESS_JOBS = gql`
query getBusinessJobs($b_id: ID!) {
getBusinessJobs(b_id: $b_id ) {
_id
name
}
}
`;
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
options: (props) => (
{
variables:
{
b_id: props.getUserBusiness.business[0].b_id
}
}
)
})
)(ProposalContainer);
reactjs react-native graphql
Do you control the server end of this too?
– David Maze
Nov 7 at 18:52
@DavidMaze no it is just a query
– Markus Hayner
Nov 7 at 19:04
getUserBusiness
sounds like it would return a single object and not an array... does it?
– Daniel Rearden
Nov 8 at 5:32
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
So I have to make two queries. First will return an array with multiple objects and I want to get the ID of the first object to use it in my second query.
The problem is that I can't use b_id: props.getBusiness.business[0]._id
Any idea how can I work with this?
const GET_USER_BUSINESS = gql`
query getUserBusiness {
getUserBusiness {
_id
}
}
`;
const GET_BUSINESS_JOBS = gql`
query getBusinessJobs($b_id: ID!) {
getBusinessJobs(b_id: $b_id ) {
_id
name
}
}
`;
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
options: (props) => (
{
variables:
{
b_id: props.getUserBusiness.business[0].b_id
}
}
)
})
)(ProposalContainer);
reactjs react-native graphql
So I have to make two queries. First will return an array with multiple objects and I want to get the ID of the first object to use it in my second query.
The problem is that I can't use b_id: props.getBusiness.business[0]._id
Any idea how can I work with this?
const GET_USER_BUSINESS = gql`
query getUserBusiness {
getUserBusiness {
_id
}
}
`;
const GET_BUSINESS_JOBS = gql`
query getBusinessJobs($b_id: ID!) {
getBusinessJobs(b_id: $b_id ) {
_id
name
}
}
`;
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
options: (props) => (
{
variables:
{
b_id: props.getUserBusiness.business[0].b_id
}
}
)
})
)(ProposalContainer);
reactjs react-native graphql
reactjs react-native graphql
asked Nov 7 at 17:28
Markus Hayner
549211
549211
Do you control the server end of this too?
– David Maze
Nov 7 at 18:52
@DavidMaze no it is just a query
– Markus Hayner
Nov 7 at 19:04
getUserBusiness
sounds like it would return a single object and not an array... does it?
– Daniel Rearden
Nov 8 at 5:32
add a comment |
Do you control the server end of this too?
– David Maze
Nov 7 at 18:52
@DavidMaze no it is just a query
– Markus Hayner
Nov 7 at 19:04
getUserBusiness
sounds like it would return a single object and not an array... does it?
– Daniel Rearden
Nov 8 at 5:32
Do you control the server end of this too?
– David Maze
Nov 7 at 18:52
Do you control the server end of this too?
– David Maze
Nov 7 at 18:52
@DavidMaze no it is just a query
– Markus Hayner
Nov 7 at 19:04
@DavidMaze no it is just a query
– Markus Hayner
Nov 7 at 19:04
getUserBusiness
sounds like it would return a single object and not an array... does it?– Daniel Rearden
Nov 8 at 5:32
getUserBusiness
sounds like it would return a single object and not an array... does it?– Daniel Rearden
Nov 8 at 5:32
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Couple of things. One, by default, the graphql
HOC passes a prop called data
down to the wrapped component with the query data. However, because you specify a name
in the HOC's config, the prop will actually be called whatever name you pass it. In other words, you can access the _id
this way:
props.business.getUserBusiness[0]._id
...if getUserBusiness returns an array. Otherwise:
props.business.getUserBusiness._id
Secondly, props.business
will be undefined until the query completes. We probably don't want to send the GET_BUSINESS_JOBS
query until we have an _id
to work with. So we want to pass a skip
function to the HOC's config:
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
skip: (props) => !props.business || !props.business.getUserBusiness[0]
options: (props) => (
{
variables:
{
b_id: props.business.getUserBusiness[0]._id
}
}
)
})
)(ProposalContainer)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Couple of things. One, by default, the graphql
HOC passes a prop called data
down to the wrapped component with the query data. However, because you specify a name
in the HOC's config, the prop will actually be called whatever name you pass it. In other words, you can access the _id
this way:
props.business.getUserBusiness[0]._id
...if getUserBusiness returns an array. Otherwise:
props.business.getUserBusiness._id
Secondly, props.business
will be undefined until the query completes. We probably don't want to send the GET_BUSINESS_JOBS
query until we have an _id
to work with. So we want to pass a skip
function to the HOC's config:
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
skip: (props) => !props.business || !props.business.getUserBusiness[0]
options: (props) => (
{
variables:
{
b_id: props.business.getUserBusiness[0]._id
}
}
)
})
)(ProposalContainer)
add a comment |
up vote
0
down vote
accepted
Couple of things. One, by default, the graphql
HOC passes a prop called data
down to the wrapped component with the query data. However, because you specify a name
in the HOC's config, the prop will actually be called whatever name you pass it. In other words, you can access the _id
this way:
props.business.getUserBusiness[0]._id
...if getUserBusiness returns an array. Otherwise:
props.business.getUserBusiness._id
Secondly, props.business
will be undefined until the query completes. We probably don't want to send the GET_BUSINESS_JOBS
query until we have an _id
to work with. So we want to pass a skip
function to the HOC's config:
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
skip: (props) => !props.business || !props.business.getUserBusiness[0]
options: (props) => (
{
variables:
{
b_id: props.business.getUserBusiness[0]._id
}
}
)
})
)(ProposalContainer)
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Couple of things. One, by default, the graphql
HOC passes a prop called data
down to the wrapped component with the query data. However, because you specify a name
in the HOC's config, the prop will actually be called whatever name you pass it. In other words, you can access the _id
this way:
props.business.getUserBusiness[0]._id
...if getUserBusiness returns an array. Otherwise:
props.business.getUserBusiness._id
Secondly, props.business
will be undefined until the query completes. We probably don't want to send the GET_BUSINESS_JOBS
query until we have an _id
to work with. So we want to pass a skip
function to the HOC's config:
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
skip: (props) => !props.business || !props.business.getUserBusiness[0]
options: (props) => (
{
variables:
{
b_id: props.business.getUserBusiness[0]._id
}
}
)
})
)(ProposalContainer)
Couple of things. One, by default, the graphql
HOC passes a prop called data
down to the wrapped component with the query data. However, because you specify a name
in the HOC's config, the prop will actually be called whatever name you pass it. In other words, you can access the _id
this way:
props.business.getUserBusiness[0]._id
...if getUserBusiness returns an array. Otherwise:
props.business.getUserBusiness._id
Secondly, props.business
will be undefined until the query completes. We probably don't want to send the GET_BUSINESS_JOBS
query until we have an _id
to work with. So we want to pass a skip
function to the HOC's config:
export default compose(
withApollo,
graphql(GET_USER_BUSINESS,
{
name: "business"
}),
graphql(GET_BUSINESS_JOBS,
{
name: "jobs",
skip: (props) => !props.business || !props.business.getUserBusiness[0]
options: (props) => (
{
variables:
{
b_id: props.business.getUserBusiness[0]._id
}
}
)
})
)(ProposalContainer)
answered Nov 8 at 5:35


Daniel Rearden
11.5k1935
11.5k1935
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53194708%2fhow-to-use-variables-in-a-graphql-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xWwqfIBOn7Vd wYFTJsN2pSOxG4h8n vJ,BbVRrtc,UYgJQA0EVn aME76etZyt7tK9nasOq,cUjqL
Do you control the server end of this too?
– David Maze
Nov 7 at 18:52
@DavidMaze no it is just a query
– Markus Hayner
Nov 7 at 19:04
getUserBusiness
sounds like it would return a single object and not an array... does it?– Daniel Rearden
Nov 8 at 5:32