Trouble using mysqli_stmt_bind_result
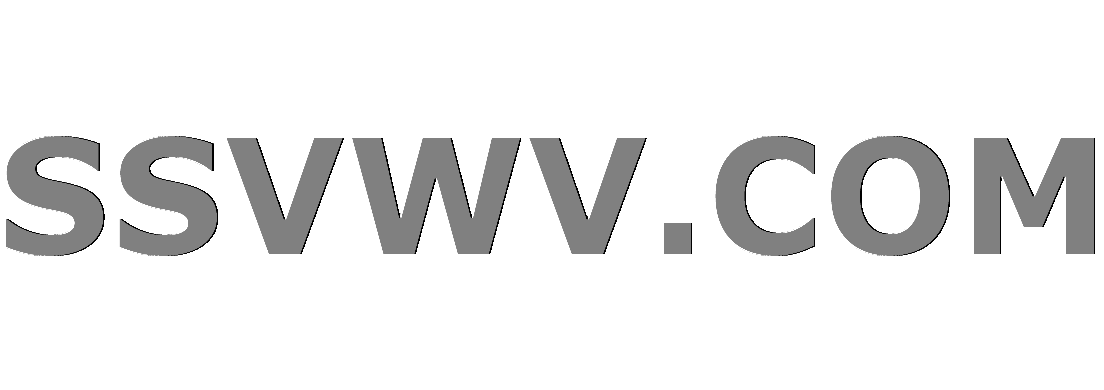
Multi tool use
up vote
1
down vote
favorite
I am trying to use mysqli_stmt_bind_result()
but I think I am not using it correctly. The code below grabs the username and password and runs a query in the database and if it matches it is meant to echo back success.
However, when I print out the row it is empty. What might I be doing wrong?
I am not planing to use mysqli_stmt_get_result()
I know how that works. I want to make use of mysqli_stmt_bind_result
in this case.
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST'){
require 'dbh.inc.php';
$email = $_POST['email'];
$pass = $_POST['pass'];
if (empty($email) || empty($pass)) {
header("Location: ../login.php?error=emptyfields");
exit();
}
else {
$sql = "SELECT idUsers, emailUsers, firstNameUsers, lastNameUsers, pwdUsers, bdayUsers FROM users WHERE emailUsers = ?";
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sql)) {
echo 'errorme';
exit();
}
else {
mysqli_stmt_bind_param($stmt ,"s", $email);
mysqli_stmt_execute($stmt);
mysqli_stmt_bind_result($stmt, $id_Users ,$email_Users, $first_NameUsers, $last_NameUsers, $pass_word, $bday_Users);
if ($row=mysqli_stmt_fetch($stmt)) {
$message = $row["$email"];
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $row['pwdUsers']);
if ($pwdCheck == false) {
echo 'wrongpass';
exit();
}
else if($pwdCheck == true) {
session_start();
$_SESSION['userId'] = $row['idUsers'];
$_SESSION['userName'] = $row['firstNameUsers'];
echo 'success';
exit();
}
else {
echo 'noresults';
exit();
}
}
else {
echo 'nouser';
exit();
}
}
}
}
else {
header("Location: ../login.php");
exit();
}
?>
php mysql mysqli
|
show 1 more comment
up vote
1
down vote
favorite
I am trying to use mysqli_stmt_bind_result()
but I think I am not using it correctly. The code below grabs the username and password and runs a query in the database and if it matches it is meant to echo back success.
However, when I print out the row it is empty. What might I be doing wrong?
I am not planing to use mysqli_stmt_get_result()
I know how that works. I want to make use of mysqli_stmt_bind_result
in this case.
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST'){
require 'dbh.inc.php';
$email = $_POST['email'];
$pass = $_POST['pass'];
if (empty($email) || empty($pass)) {
header("Location: ../login.php?error=emptyfields");
exit();
}
else {
$sql = "SELECT idUsers, emailUsers, firstNameUsers, lastNameUsers, pwdUsers, bdayUsers FROM users WHERE emailUsers = ?";
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sql)) {
echo 'errorme';
exit();
}
else {
mysqli_stmt_bind_param($stmt ,"s", $email);
mysqli_stmt_execute($stmt);
mysqli_stmt_bind_result($stmt, $id_Users ,$email_Users, $first_NameUsers, $last_NameUsers, $pass_word, $bday_Users);
if ($row=mysqli_stmt_fetch($stmt)) {
$message = $row["$email"];
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $row['pwdUsers']);
if ($pwdCheck == false) {
echo 'wrongpass';
exit();
}
else if($pwdCheck == true) {
session_start();
$_SESSION['userId'] = $row['idUsers'];
$_SESSION['userName'] = $row['firstNameUsers'];
echo 'success';
exit();
}
else {
echo 'noresults';
exit();
}
}
else {
echo 'nouser';
exit();
}
}
}
}
else {
header("Location: ../login.php");
exit();
}
?>
php mysql mysqli
You should really not be using that ancient procedural mysqli stuff for new code. mysqli has an object-oriented interface that is slightly more modern, though many (myself included) would recommend using PDO instead.
– miken32
Nov 7 at 18:24
Also please verify what output you're getting from your script with the code provided, e.g. "nouser", "noresults", etc.
– miken32
Nov 7 at 18:26
You made use of bind_result, yet, you are not using all the bound variables in your script. See example 2 in the documentation
– Ibu
Nov 7 at 18:28
I am getting wrongpass...even though the pass is 100% correct (this might be due to the row being empty..). I will take a look at PDO.
– Jack
Nov 7 at 18:28
Use these variables that you defined$id_Users ,$email_Users, $first_NameUsers,...
Not$row
– Ibu
Nov 7 at 18:30
|
show 1 more comment
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to use mysqli_stmt_bind_result()
but I think I am not using it correctly. The code below grabs the username and password and runs a query in the database and if it matches it is meant to echo back success.
However, when I print out the row it is empty. What might I be doing wrong?
I am not planing to use mysqli_stmt_get_result()
I know how that works. I want to make use of mysqli_stmt_bind_result
in this case.
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST'){
require 'dbh.inc.php';
$email = $_POST['email'];
$pass = $_POST['pass'];
if (empty($email) || empty($pass)) {
header("Location: ../login.php?error=emptyfields");
exit();
}
else {
$sql = "SELECT idUsers, emailUsers, firstNameUsers, lastNameUsers, pwdUsers, bdayUsers FROM users WHERE emailUsers = ?";
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sql)) {
echo 'errorme';
exit();
}
else {
mysqli_stmt_bind_param($stmt ,"s", $email);
mysqli_stmt_execute($stmt);
mysqli_stmt_bind_result($stmt, $id_Users ,$email_Users, $first_NameUsers, $last_NameUsers, $pass_word, $bday_Users);
if ($row=mysqli_stmt_fetch($stmt)) {
$message = $row["$email"];
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $row['pwdUsers']);
if ($pwdCheck == false) {
echo 'wrongpass';
exit();
}
else if($pwdCheck == true) {
session_start();
$_SESSION['userId'] = $row['idUsers'];
$_SESSION['userName'] = $row['firstNameUsers'];
echo 'success';
exit();
}
else {
echo 'noresults';
exit();
}
}
else {
echo 'nouser';
exit();
}
}
}
}
else {
header("Location: ../login.php");
exit();
}
?>
php mysql mysqli
I am trying to use mysqli_stmt_bind_result()
but I think I am not using it correctly. The code below grabs the username and password and runs a query in the database and if it matches it is meant to echo back success.
However, when I print out the row it is empty. What might I be doing wrong?
I am not planing to use mysqli_stmt_get_result()
I know how that works. I want to make use of mysqli_stmt_bind_result
in this case.
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST'){
require 'dbh.inc.php';
$email = $_POST['email'];
$pass = $_POST['pass'];
if (empty($email) || empty($pass)) {
header("Location: ../login.php?error=emptyfields");
exit();
}
else {
$sql = "SELECT idUsers, emailUsers, firstNameUsers, lastNameUsers, pwdUsers, bdayUsers FROM users WHERE emailUsers = ?";
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sql)) {
echo 'errorme';
exit();
}
else {
mysqli_stmt_bind_param($stmt ,"s", $email);
mysqli_stmt_execute($stmt);
mysqli_stmt_bind_result($stmt, $id_Users ,$email_Users, $first_NameUsers, $last_NameUsers, $pass_word, $bday_Users);
if ($row=mysqli_stmt_fetch($stmt)) {
$message = $row["$email"];
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $row['pwdUsers']);
if ($pwdCheck == false) {
echo 'wrongpass';
exit();
}
else if($pwdCheck == true) {
session_start();
$_SESSION['userId'] = $row['idUsers'];
$_SESSION['userName'] = $row['firstNameUsers'];
echo 'success';
exit();
}
else {
echo 'noresults';
exit();
}
}
else {
echo 'nouser';
exit();
}
}
}
}
else {
header("Location: ../login.php");
exit();
}
?>
php mysql mysqli
php mysql mysqli
edited Nov 7 at 18:36


Qirel
9,53862337
9,53862337
asked Nov 7 at 18:21
Jack
127114
127114
You should really not be using that ancient procedural mysqli stuff for new code. mysqli has an object-oriented interface that is slightly more modern, though many (myself included) would recommend using PDO instead.
– miken32
Nov 7 at 18:24
Also please verify what output you're getting from your script with the code provided, e.g. "nouser", "noresults", etc.
– miken32
Nov 7 at 18:26
You made use of bind_result, yet, you are not using all the bound variables in your script. See example 2 in the documentation
– Ibu
Nov 7 at 18:28
I am getting wrongpass...even though the pass is 100% correct (this might be due to the row being empty..). I will take a look at PDO.
– Jack
Nov 7 at 18:28
Use these variables that you defined$id_Users ,$email_Users, $first_NameUsers,...
Not$row
– Ibu
Nov 7 at 18:30
|
show 1 more comment
You should really not be using that ancient procedural mysqli stuff for new code. mysqli has an object-oriented interface that is slightly more modern, though many (myself included) would recommend using PDO instead.
– miken32
Nov 7 at 18:24
Also please verify what output you're getting from your script with the code provided, e.g. "nouser", "noresults", etc.
– miken32
Nov 7 at 18:26
You made use of bind_result, yet, you are not using all the bound variables in your script. See example 2 in the documentation
– Ibu
Nov 7 at 18:28
I am getting wrongpass...even though the pass is 100% correct (this might be due to the row being empty..). I will take a look at PDO.
– Jack
Nov 7 at 18:28
Use these variables that you defined$id_Users ,$email_Users, $first_NameUsers,...
Not$row
– Ibu
Nov 7 at 18:30
You should really not be using that ancient procedural mysqli stuff for new code. mysqli has an object-oriented interface that is slightly more modern, though many (myself included) would recommend using PDO instead.
– miken32
Nov 7 at 18:24
You should really not be using that ancient procedural mysqli stuff for new code. mysqli has an object-oriented interface that is slightly more modern, though many (myself included) would recommend using PDO instead.
– miken32
Nov 7 at 18:24
Also please verify what output you're getting from your script with the code provided, e.g. "nouser", "noresults", etc.
– miken32
Nov 7 at 18:26
Also please verify what output you're getting from your script with the code provided, e.g. "nouser", "noresults", etc.
– miken32
Nov 7 at 18:26
You made use of bind_result, yet, you are not using all the bound variables in your script. See example 2 in the documentation
– Ibu
Nov 7 at 18:28
You made use of bind_result, yet, you are not using all the bound variables in your script. See example 2 in the documentation
– Ibu
Nov 7 at 18:28
I am getting wrongpass...even though the pass is 100% correct (this might be due to the row being empty..). I will take a look at PDO.
– Jack
Nov 7 at 18:28
I am getting wrongpass...even though the pass is 100% correct (this might be due to the row being empty..). I will take a look at PDO.
– Jack
Nov 7 at 18:28
Use these variables that you defined
$id_Users ,$email_Users, $first_NameUsers,...
Not $row
– Ibu
Nov 7 at 18:30
Use these variables that you defined
$id_Users ,$email_Users, $first_NameUsers,...
Not $row
– Ibu
Nov 7 at 18:30
|
show 1 more comment
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You bind the results through mysqli_stmt_bind_result()
, and these are the variables which will hold the values once your results are fetched.
Your fetch()
function returns a boolean, and not a result (unlike the non-prepared queries).
if (mysqli_stmt_fetch($stmt)) {
$message = $email_Users;
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $pass_word);
The same goes for any place you attempt to use $row
- you have to use the variables that are assigned in mysqli_stmt_bind_result()
(so $id_Users
instead of $row['idUsers']
, $first_NameUsers
instead of $row['firstNameUsers']
and so on).
See the documentation for each method for more details.
mysqli_stmt::bind_result()
mysqli_stmt::fetch()
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You bind the results through mysqli_stmt_bind_result()
, and these are the variables which will hold the values once your results are fetched.
Your fetch()
function returns a boolean, and not a result (unlike the non-prepared queries).
if (mysqli_stmt_fetch($stmt)) {
$message = $email_Users;
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $pass_word);
The same goes for any place you attempt to use $row
- you have to use the variables that are assigned in mysqli_stmt_bind_result()
(so $id_Users
instead of $row['idUsers']
, $first_NameUsers
instead of $row['firstNameUsers']
and so on).
See the documentation for each method for more details.
mysqli_stmt::bind_result()
mysqli_stmt::fetch()
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
add a comment |
up vote
2
down vote
accepted
You bind the results through mysqli_stmt_bind_result()
, and these are the variables which will hold the values once your results are fetched.
Your fetch()
function returns a boolean, and not a result (unlike the non-prepared queries).
if (mysqli_stmt_fetch($stmt)) {
$message = $email_Users;
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $pass_word);
The same goes for any place you attempt to use $row
- you have to use the variables that are assigned in mysqli_stmt_bind_result()
(so $id_Users
instead of $row['idUsers']
, $first_NameUsers
instead of $row['firstNameUsers']
and so on).
See the documentation for each method for more details.
mysqli_stmt::bind_result()
mysqli_stmt::fetch()
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You bind the results through mysqli_stmt_bind_result()
, and these are the variables which will hold the values once your results are fetched.
Your fetch()
function returns a boolean, and not a result (unlike the non-prepared queries).
if (mysqli_stmt_fetch($stmt)) {
$message = $email_Users;
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $pass_word);
The same goes for any place you attempt to use $row
- you have to use the variables that are assigned in mysqli_stmt_bind_result()
(so $id_Users
instead of $row['idUsers']
, $first_NameUsers
instead of $row['firstNameUsers']
and so on).
See the documentation for each method for more details.
mysqli_stmt::bind_result()
mysqli_stmt::fetch()
You bind the results through mysqli_stmt_bind_result()
, and these are the variables which will hold the values once your results are fetched.
Your fetch()
function returns a boolean, and not a result (unlike the non-prepared queries).
if (mysqli_stmt_fetch($stmt)) {
$message = $email_Users;
echo "<script type='text/javascript'>alert('$message');</script>";
$pwdCheck = password_verify($pass, $pass_word);
The same goes for any place you attempt to use $row
- you have to use the variables that are assigned in mysqli_stmt_bind_result()
(so $id_Users
instead of $row['idUsers']
, $first_NameUsers
instead of $row['firstNameUsers']
and so on).
See the documentation for each method for more details.
mysqli_stmt::bind_result()
mysqli_stmt::fetch()
answered Nov 7 at 18:34


Qirel
9,53862337
9,53862337
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
add a comment |
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
Perfect makes complete sense thank you so much!
– Jack
Nov 7 at 18:40
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53195500%2ftrouble-using-mysqli-stmt-bind-result%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3dQWqxoh,nwWc 19A,tUVnXO,wP18ck,TwIemJwLI4PQHQVk,amixy,e,y XJ
You should really not be using that ancient procedural mysqli stuff for new code. mysqli has an object-oriented interface that is slightly more modern, though many (myself included) would recommend using PDO instead.
– miken32
Nov 7 at 18:24
Also please verify what output you're getting from your script with the code provided, e.g. "nouser", "noresults", etc.
– miken32
Nov 7 at 18:26
You made use of bind_result, yet, you are not using all the bound variables in your script. See example 2 in the documentation
– Ibu
Nov 7 at 18:28
I am getting wrongpass...even though the pass is 100% correct (this might be due to the row being empty..). I will take a look at PDO.
– Jack
Nov 7 at 18:28
Use these variables that you defined
$id_Users ,$email_Users, $first_NameUsers,...
Not$row
– Ibu
Nov 7 at 18:30