Keras variable() memory leak
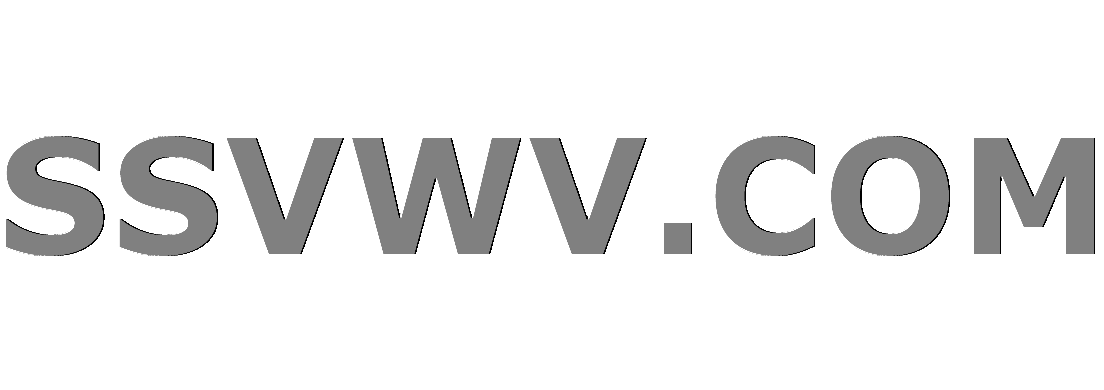
Multi tool use
up vote
0
down vote
favorite
I am new to Keras, and tensorflow in general, and have a problem. I am using some of the loss functions (binary_crossentropy and mean_squared_error mainly) to calculate the loss after prediction. Since Keras only accepts it's own variable type, I am creating one and supply it as an argument. This scenario is executed in a loop (with sleep) as such:
Get appropriate data -> predict -> calculate the lost -> return it.
Since I have multiple models that follow this pattern I created tensorflow graphs and sessions to prevent collision (also when exporting the models' weights I had problem with single graph and session so I had to create distinct ones for every single model).
However, now the memory is rising uncontrollably, from couple of MiB to 700MiB in couple of iterations. I am aware of Keras's clear_session() and gc.collect(), and I use them at the end of every iteration, but the problem is still present. Here I provide a code snippet, which is not the actual code, from the project. I've created separate script in order to isolate the problem:
import tensorflow as tf
from keras import backend as K
from keras.losses import binary_crossentropy, mean_squared_error
from time import time, sleep
import gc
from numpy.random import rand
from os import getpid
from psutil import Process
from csv import DictWriter
from keras import backend as K
this_process = Process(getpid())
graph = tf.Graph()
sess = tf.Session(graph=graph)
cnt = 0
max_c = 500
with open('/home/quark/Desktop/python-test/leak-7.csv', 'a') as file:
writer = DictWriter(file, fieldnames=['time', 'mem'])
writer.writeheader()
while cnt < max_c:
with graph.as_default(), sess.as_default():
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
rec_loss = K.eval(binary_crossentropy(y_true, y_pred))
val_loss = K.eval(mean_squared_error(y_true, y_pred))
writer.writerow({
'time': int(time()),
'mem': this_process.memory_info().rss
})
K.clear_session()
gc.collect()
cnt += 1
print(max_c - cnt)
sleep(0.1)
Additionally, I've added the plot of the memory usage:
Keras memory leak
Any help is appreciated.
python tensorflow memory-leaks keras
add a comment |
up vote
0
down vote
favorite
I am new to Keras, and tensorflow in general, and have a problem. I am using some of the loss functions (binary_crossentropy and mean_squared_error mainly) to calculate the loss after prediction. Since Keras only accepts it's own variable type, I am creating one and supply it as an argument. This scenario is executed in a loop (with sleep) as such:
Get appropriate data -> predict -> calculate the lost -> return it.
Since I have multiple models that follow this pattern I created tensorflow graphs and sessions to prevent collision (also when exporting the models' weights I had problem with single graph and session so I had to create distinct ones for every single model).
However, now the memory is rising uncontrollably, from couple of MiB to 700MiB in couple of iterations. I am aware of Keras's clear_session() and gc.collect(), and I use them at the end of every iteration, but the problem is still present. Here I provide a code snippet, which is not the actual code, from the project. I've created separate script in order to isolate the problem:
import tensorflow as tf
from keras import backend as K
from keras.losses import binary_crossentropy, mean_squared_error
from time import time, sleep
import gc
from numpy.random import rand
from os import getpid
from psutil import Process
from csv import DictWriter
from keras import backend as K
this_process = Process(getpid())
graph = tf.Graph()
sess = tf.Session(graph=graph)
cnt = 0
max_c = 500
with open('/home/quark/Desktop/python-test/leak-7.csv', 'a') as file:
writer = DictWriter(file, fieldnames=['time', 'mem'])
writer.writeheader()
while cnt < max_c:
with graph.as_default(), sess.as_default():
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
rec_loss = K.eval(binary_crossentropy(y_true, y_pred))
val_loss = K.eval(mean_squared_error(y_true, y_pred))
writer.writerow({
'time': int(time()),
'mem': this_process.memory_info().rss
})
K.clear_session()
gc.collect()
cnt += 1
print(max_c - cnt)
sleep(0.1)
Additionally, I've added the plot of the memory usage:
Keras memory leak
Any help is appreciated.
python tensorflow memory-leaks keras
Could you add the requred imports? I believe you are mixing tf and keras commands.
– Daniel GL
Nov 8 at 10:11
Yes, a full example that we can run would be nice.
– Matias Valdenegro
Nov 8 at 10:13
I've updated the code.
– mladenk
Nov 8 at 10:43
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am new to Keras, and tensorflow in general, and have a problem. I am using some of the loss functions (binary_crossentropy and mean_squared_error mainly) to calculate the loss after prediction. Since Keras only accepts it's own variable type, I am creating one and supply it as an argument. This scenario is executed in a loop (with sleep) as such:
Get appropriate data -> predict -> calculate the lost -> return it.
Since I have multiple models that follow this pattern I created tensorflow graphs and sessions to prevent collision (also when exporting the models' weights I had problem with single graph and session so I had to create distinct ones for every single model).
However, now the memory is rising uncontrollably, from couple of MiB to 700MiB in couple of iterations. I am aware of Keras's clear_session() and gc.collect(), and I use them at the end of every iteration, but the problem is still present. Here I provide a code snippet, which is not the actual code, from the project. I've created separate script in order to isolate the problem:
import tensorflow as tf
from keras import backend as K
from keras.losses import binary_crossentropy, mean_squared_error
from time import time, sleep
import gc
from numpy.random import rand
from os import getpid
from psutil import Process
from csv import DictWriter
from keras import backend as K
this_process = Process(getpid())
graph = tf.Graph()
sess = tf.Session(graph=graph)
cnt = 0
max_c = 500
with open('/home/quark/Desktop/python-test/leak-7.csv', 'a') as file:
writer = DictWriter(file, fieldnames=['time', 'mem'])
writer.writeheader()
while cnt < max_c:
with graph.as_default(), sess.as_default():
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
rec_loss = K.eval(binary_crossentropy(y_true, y_pred))
val_loss = K.eval(mean_squared_error(y_true, y_pred))
writer.writerow({
'time': int(time()),
'mem': this_process.memory_info().rss
})
K.clear_session()
gc.collect()
cnt += 1
print(max_c - cnt)
sleep(0.1)
Additionally, I've added the plot of the memory usage:
Keras memory leak
Any help is appreciated.
python tensorflow memory-leaks keras
I am new to Keras, and tensorflow in general, and have a problem. I am using some of the loss functions (binary_crossentropy and mean_squared_error mainly) to calculate the loss after prediction. Since Keras only accepts it's own variable type, I am creating one and supply it as an argument. This scenario is executed in a loop (with sleep) as such:
Get appropriate data -> predict -> calculate the lost -> return it.
Since I have multiple models that follow this pattern I created tensorflow graphs and sessions to prevent collision (also when exporting the models' weights I had problem with single graph and session so I had to create distinct ones for every single model).
However, now the memory is rising uncontrollably, from couple of MiB to 700MiB in couple of iterations. I am aware of Keras's clear_session() and gc.collect(), and I use them at the end of every iteration, but the problem is still present. Here I provide a code snippet, which is not the actual code, from the project. I've created separate script in order to isolate the problem:
import tensorflow as tf
from keras import backend as K
from keras.losses import binary_crossentropy, mean_squared_error
from time import time, sleep
import gc
from numpy.random import rand
from os import getpid
from psutil import Process
from csv import DictWriter
from keras import backend as K
this_process = Process(getpid())
graph = tf.Graph()
sess = tf.Session(graph=graph)
cnt = 0
max_c = 500
with open('/home/quark/Desktop/python-test/leak-7.csv', 'a') as file:
writer = DictWriter(file, fieldnames=['time', 'mem'])
writer.writeheader()
while cnt < max_c:
with graph.as_default(), sess.as_default():
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
rec_loss = K.eval(binary_crossentropy(y_true, y_pred))
val_loss = K.eval(mean_squared_error(y_true, y_pred))
writer.writerow({
'time': int(time()),
'mem': this_process.memory_info().rss
})
K.clear_session()
gc.collect()
cnt += 1
print(max_c - cnt)
sleep(0.1)
Additionally, I've added the plot of the memory usage:
Keras memory leak
Any help is appreciated.
python tensorflow memory-leaks keras
python tensorflow memory-leaks keras
edited Nov 8 at 10:43
asked Nov 8 at 9:23
mladenk
12
12
Could you add the requred imports? I believe you are mixing tf and keras commands.
– Daniel GL
Nov 8 at 10:11
Yes, a full example that we can run would be nice.
– Matias Valdenegro
Nov 8 at 10:13
I've updated the code.
– mladenk
Nov 8 at 10:43
add a comment |
Could you add the requred imports? I believe you are mixing tf and keras commands.
– Daniel GL
Nov 8 at 10:11
Yes, a full example that we can run would be nice.
– Matias Valdenegro
Nov 8 at 10:13
I've updated the code.
– mladenk
Nov 8 at 10:43
Could you add the requred imports? I believe you are mixing tf and keras commands.
– Daniel GL
Nov 8 at 10:11
Could you add the requred imports? I believe you are mixing tf and keras commands.
– Daniel GL
Nov 8 at 10:11
Yes, a full example that we can run would be nice.
– Matias Valdenegro
Nov 8 at 10:13
Yes, a full example that we can run would be nice.
– Matias Valdenegro
Nov 8 at 10:13
I've updated the code.
– mladenk
Nov 8 at 10:43
I've updated the code.
– mladenk
Nov 8 at 10:43
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
I just removed the with
statement (probably some tf code), and I don't see any leak. I believe there is a difference between the keras session and the tf default session. So you were not clearing the correct session with K.clear_session()
. Probably using tf.reset_default_graph()
could work too.
while True:
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
val_loss = K.eval(binary_crossentropy(y_true, y_pred))
rec_loss = K.eval(mean_squared_error(y_true, y_pred))
K.clear_session()
gc.collect()
sleep(0.1)
I know that removing thewith
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try withtf.reset_default_graph()
.
– mladenk
Nov 8 at 10:41
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
add a comment |
up vote
0
down vote
At the end, what I did was remove the K.variable()
code out of the where
statement. In that way, the variables are part of the default graph which is later cleared by K.clear_session()
.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
I just removed the with
statement (probably some tf code), and I don't see any leak. I believe there is a difference between the keras session and the tf default session. So you were not clearing the correct session with K.clear_session()
. Probably using tf.reset_default_graph()
could work too.
while True:
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
val_loss = K.eval(binary_crossentropy(y_true, y_pred))
rec_loss = K.eval(mean_squared_error(y_true, y_pred))
K.clear_session()
gc.collect()
sleep(0.1)
I know that removing thewith
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try withtf.reset_default_graph()
.
– mladenk
Nov 8 at 10:41
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
add a comment |
up vote
1
down vote
I just removed the with
statement (probably some tf code), and I don't see any leak. I believe there is a difference between the keras session and the tf default session. So you were not clearing the correct session with K.clear_session()
. Probably using tf.reset_default_graph()
could work too.
while True:
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
val_loss = K.eval(binary_crossentropy(y_true, y_pred))
rec_loss = K.eval(mean_squared_error(y_true, y_pred))
K.clear_session()
gc.collect()
sleep(0.1)
I know that removing thewith
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try withtf.reset_default_graph()
.
– mladenk
Nov 8 at 10:41
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
add a comment |
up vote
1
down vote
up vote
1
down vote
I just removed the with
statement (probably some tf code), and I don't see any leak. I believe there is a difference between the keras session and the tf default session. So you were not clearing the correct session with K.clear_session()
. Probably using tf.reset_default_graph()
could work too.
while True:
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
val_loss = K.eval(binary_crossentropy(y_true, y_pred))
rec_loss = K.eval(mean_squared_error(y_true, y_pred))
K.clear_session()
gc.collect()
sleep(0.1)
I just removed the with
statement (probably some tf code), and I don't see any leak. I believe there is a difference between the keras session and the tf default session. So you were not clearing the correct session with K.clear_session()
. Probably using tf.reset_default_graph()
could work too.
while True:
y_true = K.variable(rand(36, 6))
y_pred = K.variable(rand(36, 6))
val_loss = K.eval(binary_crossentropy(y_true, y_pred))
rec_loss = K.eval(mean_squared_error(y_true, y_pred))
K.clear_session()
gc.collect()
sleep(0.1)
answered Nov 8 at 10:25
Daniel GL
722316
722316
I know that removing thewith
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try withtf.reset_default_graph()
.
– mladenk
Nov 8 at 10:41
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
add a comment |
I know that removing thewith
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try withtf.reset_default_graph()
.
– mladenk
Nov 8 at 10:41
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
I know that removing the
with
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try with tf.reset_default_graph()
.– mladenk
Nov 8 at 10:41
I know that removing the
with
statement solves the problem but I am afraid I cannot omit it because of the different graphs I have. Regardless, thanks and I'll try with tf.reset_default_graph()
.– mladenk
Nov 8 at 10:41
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
Then you just have to reset the appropriate graph, not just the default one
– Daniel GL
Nov 8 at 10:48
add a comment |
up vote
0
down vote
At the end, what I did was remove the K.variable()
code out of the where
statement. In that way, the variables are part of the default graph which is later cleared by K.clear_session()
.
add a comment |
up vote
0
down vote
At the end, what I did was remove the K.variable()
code out of the where
statement. In that way, the variables are part of the default graph which is later cleared by K.clear_session()
.
add a comment |
up vote
0
down vote
up vote
0
down vote
At the end, what I did was remove the K.variable()
code out of the where
statement. In that way, the variables are part of the default graph which is later cleared by K.clear_session()
.
At the end, what I did was remove the K.variable()
code out of the where
statement. In that way, the variables are part of the default graph which is later cleared by K.clear_session()
.
answered Nov 9 at 11:18
mladenk
12
12
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53204747%2fkeras-variable-memory-leak%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eo,inlt J7myD,MB4RQNrrpck03X4YLowpS,8i0BY,k IeeKuJlIVoe,PnUTNsrmhkM3
Could you add the requred imports? I believe you are mixing tf and keras commands.
– Daniel GL
Nov 8 at 10:11
Yes, a full example that we can run would be nice.
– Matias Valdenegro
Nov 8 at 10:13
I've updated the code.
– mladenk
Nov 8 at 10:43