Custom Logging and Future Methods
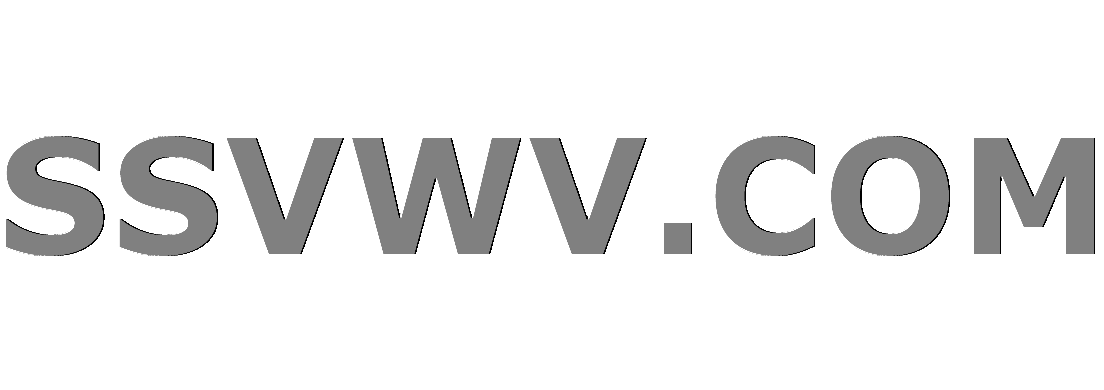
Multi tool use
I have been reading up on the custom logging, DML, and exceptions but haven't seen anything that talks about @Future methods and exceptions.
I have two scenarios:
- A Button is clicked on a Lead, class A is called (class A has a try/catch with logging), a Webservice class is called, the Webservice fails, an exception is thrown, the exception trickles back up to class A, logging class is called, and a new logging record is inserted.
- A Lead is inserted, a Trigger is fired, a Webservice is called from a future method, the Webservice fails, an exception is thrown.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
future method
. This breaks my pattern of always putting logging on the root level.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
Is there a way to push the exception up from a @future method
to the method or class that calls it? Is there a way to put logging on the root class in all scenarios even if there is a @future method
involved?
trigger AllLeadTrigger on Lead (before insert, before update, before delete, after insert, after update, after delete, after undelete) {
if( Trigger.isInsert ){
if(Trigger.isBefore) {
...
}else {
...
}
}else if (Trigger.isUpdate ) {
if(Trigger.isBefore) {
...
}else {
try{
LeadEncryptedEmailStringCreation les = new LeadEncryptedEmailStringCreation();
les.leadEncryptedEmailStringCreate(Trigger.newMap, Trigger.oldMap);
}catch(Exception ex){
NFLogger.logError('AllLeadTrigger, LeadEncryptedEmailStringCreation, trigger', 'Call to leadEncryptedEmailStringCreate failed.', ex);
}
}
}
}
public with sharing class LeadEncryptedEmailStringCreation {
public void leadEncryptedEmailStringCreate(Map<Id, Lead> newLeads, Map<Id, Lead> oldLeads) {
List<Id> leadIDList = new List<Id>();
try {
for (Lead l : [SELECT Id, pi__url__c, IsConverted, Web_Id_NatFund__c, Encrypted_Email_String__c FROM Lead
WHERE Id IN :newLeads.keySet() AND pi__url__c != NULL AND IsConverted = FALSE AND Web_Id_NatFund__c != NULL
AND Encrypted_Email_String__c = NULL]) {
if (l.pi__url__c != oldLeads.get(l.id).pi__url__c) {
leadIDList.add(l.Id);
}
}
if (leadIDList.size() > 0) {
updateEncryptedEmailStringForLeads(leadIDList);
}
} catch (Exception e) {
throw e;
}
}
@Future(callout=true)
public static void updateEncryptedEmailStringForLeads(List<Id> leadIdList) {
try {
Map<String, String> idAndTokenMap = new Map<String, String>();
List<Lead> leadListUpdate = new List<Lead>();
TokenGenerator tg = new TokenGenerator();
idAndTokenMap = tg.getTokenizedPayloadForBulkLeads(leadIdList); //webservice is called here
for (String leadId : idAndTokenMap.keySet()) {
Lead l = new Lead(Id = leadId, Encrypted_Email_String__c = idAndTokenMap.get(leadId));
leadListUpdate.add(l);
}
update leadListUpdate;
}catch (Exception e) {
//throw e //this does persist upwards so I log here
NFLogger.logError('LeadEncryptedEmailStringCreation, tokenizer', 'There has been an error in the updateEncryptedEmailStringForLeads on Lead(s): ' + leadIdList, e);
}
}
}
apex trigger future asynchronous logging
add a comment |
I have been reading up on the custom logging, DML, and exceptions but haven't seen anything that talks about @Future methods and exceptions.
I have two scenarios:
- A Button is clicked on a Lead, class A is called (class A has a try/catch with logging), a Webservice class is called, the Webservice fails, an exception is thrown, the exception trickles back up to class A, logging class is called, and a new logging record is inserted.
- A Lead is inserted, a Trigger is fired, a Webservice is called from a future method, the Webservice fails, an exception is thrown.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
future method
. This breaks my pattern of always putting logging on the root level.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
Is there a way to push the exception up from a @future method
to the method or class that calls it? Is there a way to put logging on the root class in all scenarios even if there is a @future method
involved?
trigger AllLeadTrigger on Lead (before insert, before update, before delete, after insert, after update, after delete, after undelete) {
if( Trigger.isInsert ){
if(Trigger.isBefore) {
...
}else {
...
}
}else if (Trigger.isUpdate ) {
if(Trigger.isBefore) {
...
}else {
try{
LeadEncryptedEmailStringCreation les = new LeadEncryptedEmailStringCreation();
les.leadEncryptedEmailStringCreate(Trigger.newMap, Trigger.oldMap);
}catch(Exception ex){
NFLogger.logError('AllLeadTrigger, LeadEncryptedEmailStringCreation, trigger', 'Call to leadEncryptedEmailStringCreate failed.', ex);
}
}
}
}
public with sharing class LeadEncryptedEmailStringCreation {
public void leadEncryptedEmailStringCreate(Map<Id, Lead> newLeads, Map<Id, Lead> oldLeads) {
List<Id> leadIDList = new List<Id>();
try {
for (Lead l : [SELECT Id, pi__url__c, IsConverted, Web_Id_NatFund__c, Encrypted_Email_String__c FROM Lead
WHERE Id IN :newLeads.keySet() AND pi__url__c != NULL AND IsConverted = FALSE AND Web_Id_NatFund__c != NULL
AND Encrypted_Email_String__c = NULL]) {
if (l.pi__url__c != oldLeads.get(l.id).pi__url__c) {
leadIDList.add(l.Id);
}
}
if (leadIDList.size() > 0) {
updateEncryptedEmailStringForLeads(leadIDList);
}
} catch (Exception e) {
throw e;
}
}
@Future(callout=true)
public static void updateEncryptedEmailStringForLeads(List<Id> leadIdList) {
try {
Map<String, String> idAndTokenMap = new Map<String, String>();
List<Lead> leadListUpdate = new List<Lead>();
TokenGenerator tg = new TokenGenerator();
idAndTokenMap = tg.getTokenizedPayloadForBulkLeads(leadIdList); //webservice is called here
for (String leadId : idAndTokenMap.keySet()) {
Lead l = new Lead(Id = leadId, Encrypted_Email_String__c = idAndTokenMap.get(leadId));
leadListUpdate.add(l);
}
update leadListUpdate;
}catch (Exception e) {
//throw e //this does persist upwards so I log here
NFLogger.logError('LeadEncryptedEmailStringCreation, tokenizer', 'There has been an error in the updateEncryptedEmailStringForLeads on Lead(s): ' + leadIdList, e);
}
}
}
apex trigger future asynchronous logging
add a comment |
I have been reading up on the custom logging, DML, and exceptions but haven't seen anything that talks about @Future methods and exceptions.
I have two scenarios:
- A Button is clicked on a Lead, class A is called (class A has a try/catch with logging), a Webservice class is called, the Webservice fails, an exception is thrown, the exception trickles back up to class A, logging class is called, and a new logging record is inserted.
- A Lead is inserted, a Trigger is fired, a Webservice is called from a future method, the Webservice fails, an exception is thrown.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
future method
. This breaks my pattern of always putting logging on the root level.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
Is there a way to push the exception up from a @future method
to the method or class that calls it? Is there a way to put logging on the root class in all scenarios even if there is a @future method
involved?
trigger AllLeadTrigger on Lead (before insert, before update, before delete, after insert, after update, after delete, after undelete) {
if( Trigger.isInsert ){
if(Trigger.isBefore) {
...
}else {
...
}
}else if (Trigger.isUpdate ) {
if(Trigger.isBefore) {
...
}else {
try{
LeadEncryptedEmailStringCreation les = new LeadEncryptedEmailStringCreation();
les.leadEncryptedEmailStringCreate(Trigger.newMap, Trigger.oldMap);
}catch(Exception ex){
NFLogger.logError('AllLeadTrigger, LeadEncryptedEmailStringCreation, trigger', 'Call to leadEncryptedEmailStringCreate failed.', ex);
}
}
}
}
public with sharing class LeadEncryptedEmailStringCreation {
public void leadEncryptedEmailStringCreate(Map<Id, Lead> newLeads, Map<Id, Lead> oldLeads) {
List<Id> leadIDList = new List<Id>();
try {
for (Lead l : [SELECT Id, pi__url__c, IsConverted, Web_Id_NatFund__c, Encrypted_Email_String__c FROM Lead
WHERE Id IN :newLeads.keySet() AND pi__url__c != NULL AND IsConverted = FALSE AND Web_Id_NatFund__c != NULL
AND Encrypted_Email_String__c = NULL]) {
if (l.pi__url__c != oldLeads.get(l.id).pi__url__c) {
leadIDList.add(l.Id);
}
}
if (leadIDList.size() > 0) {
updateEncryptedEmailStringForLeads(leadIDList);
}
} catch (Exception e) {
throw e;
}
}
@Future(callout=true)
public static void updateEncryptedEmailStringForLeads(List<Id> leadIdList) {
try {
Map<String, String> idAndTokenMap = new Map<String, String>();
List<Lead> leadListUpdate = new List<Lead>();
TokenGenerator tg = new TokenGenerator();
idAndTokenMap = tg.getTokenizedPayloadForBulkLeads(leadIdList); //webservice is called here
for (String leadId : idAndTokenMap.keySet()) {
Lead l = new Lead(Id = leadId, Encrypted_Email_String__c = idAndTokenMap.get(leadId));
leadListUpdate.add(l);
}
update leadListUpdate;
}catch (Exception e) {
//throw e //this does persist upwards so I log here
NFLogger.logError('LeadEncryptedEmailStringCreation, tokenizer', 'There has been an error in the updateEncryptedEmailStringForLeads on Lead(s): ' + leadIdList, e);
}
}
}
apex trigger future asynchronous logging
I have been reading up on the custom logging, DML, and exceptions but haven't seen anything that talks about @Future methods and exceptions.
I have two scenarios:
- A Button is clicked on a Lead, class A is called (class A has a try/catch with logging), a Webservice class is called, the Webservice fails, an exception is thrown, the exception trickles back up to class A, logging class is called, and a new logging record is inserted.
- A Lead is inserted, a Trigger is fired, a Webservice is called from a future method, the Webservice fails, an exception is thrown.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
future method
. This breaks my pattern of always putting logging on the root level.
- I want to put logging on the Trigger but because of the future method, the exception does not make it's way back up to the root level. I have found that if I want to log the exception, then I have to put the log on the
Is there a way to push the exception up from a @future method
to the method or class that calls it? Is there a way to put logging on the root class in all scenarios even if there is a @future method
involved?
trigger AllLeadTrigger on Lead (before insert, before update, before delete, after insert, after update, after delete, after undelete) {
if( Trigger.isInsert ){
if(Trigger.isBefore) {
...
}else {
...
}
}else if (Trigger.isUpdate ) {
if(Trigger.isBefore) {
...
}else {
try{
LeadEncryptedEmailStringCreation les = new LeadEncryptedEmailStringCreation();
les.leadEncryptedEmailStringCreate(Trigger.newMap, Trigger.oldMap);
}catch(Exception ex){
NFLogger.logError('AllLeadTrigger, LeadEncryptedEmailStringCreation, trigger', 'Call to leadEncryptedEmailStringCreate failed.', ex);
}
}
}
}
public with sharing class LeadEncryptedEmailStringCreation {
public void leadEncryptedEmailStringCreate(Map<Id, Lead> newLeads, Map<Id, Lead> oldLeads) {
List<Id> leadIDList = new List<Id>();
try {
for (Lead l : [SELECT Id, pi__url__c, IsConverted, Web_Id_NatFund__c, Encrypted_Email_String__c FROM Lead
WHERE Id IN :newLeads.keySet() AND pi__url__c != NULL AND IsConverted = FALSE AND Web_Id_NatFund__c != NULL
AND Encrypted_Email_String__c = NULL]) {
if (l.pi__url__c != oldLeads.get(l.id).pi__url__c) {
leadIDList.add(l.Id);
}
}
if (leadIDList.size() > 0) {
updateEncryptedEmailStringForLeads(leadIDList);
}
} catch (Exception e) {
throw e;
}
}
@Future(callout=true)
public static void updateEncryptedEmailStringForLeads(List<Id> leadIdList) {
try {
Map<String, String> idAndTokenMap = new Map<String, String>();
List<Lead> leadListUpdate = new List<Lead>();
TokenGenerator tg = new TokenGenerator();
idAndTokenMap = tg.getTokenizedPayloadForBulkLeads(leadIdList); //webservice is called here
for (String leadId : idAndTokenMap.keySet()) {
Lead l = new Lead(Id = leadId, Encrypted_Email_String__c = idAndTokenMap.get(leadId));
leadListUpdate.add(l);
}
update leadListUpdate;
}catch (Exception e) {
//throw e //this does persist upwards so I log here
NFLogger.logError('LeadEncryptedEmailStringCreation, tokenizer', 'There has been an error in the updateEncryptedEmailStringForLeads on Lead(s): ' + leadIdList, e);
}
}
}
apex trigger future asynchronous logging
apex trigger future asynchronous logging
asked Nov 12 '18 at 18:28
OliviaOlivia
1,286423
1,286423
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The future method simply hasn't executed yet by the time the caller completes.
Maybe what you could do is pass an identifier from the calling class to the future method. The future method passes this into the custom logger which locates the same log entry that was used for the caller and appends to it.
2
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "459"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f239090%2fcustom-logging-and-future-methods%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The future method simply hasn't executed yet by the time the caller completes.
Maybe what you could do is pass an identifier from the calling class to the future method. The future method passes this into the custom logger which locates the same log entry that was used for the caller and appends to it.
2
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
add a comment |
The future method simply hasn't executed yet by the time the caller completes.
Maybe what you could do is pass an identifier from the calling class to the future method. The future method passes this into the custom logger which locates the same log entry that was used for the caller and appends to it.
2
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
add a comment |
The future method simply hasn't executed yet by the time the caller completes.
Maybe what you could do is pass an identifier from the calling class to the future method. The future method passes this into the custom logger which locates the same log entry that was used for the caller and appends to it.
The future method simply hasn't executed yet by the time the caller completes.
Maybe what you could do is pass an identifier from the calling class to the future method. The future method passes this into the custom logger which locates the same log entry that was used for the caller and appends to it.
answered Nov 12 '18 at 18:39
Charles TCharles T
6,2361822
6,2361822
2
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
add a comment |
2
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
2
2
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
More to the point, the future method is even guaranteed not to execute before the caller finishes, so it can be rolled back if anything cancels it.
– sfdcfox
Nov 12 '18 at 18:41
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
Interesting. Good approach, I will look into implementing something along those lines. Another thought is, I could change my pattern to put loggers on the class level vs the trigger. This way I can put the logger directly on the future method if applicable.
– Olivia
Nov 12 '18 at 19:29
add a comment |
Thanks for contributing an answer to Salesforce Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f239090%2fcustom-logging-and-future-methods%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e,HlKfK5,aM,mRxg9TB YB4eL0Ft vxlZpsPhm8q4ZFdfnECI0n1qf,W vS2QJuX,tu2kktpgAFw,zk,v mOMw7uCCuaW EKi