Prevent collision on multiple database calls in Web API
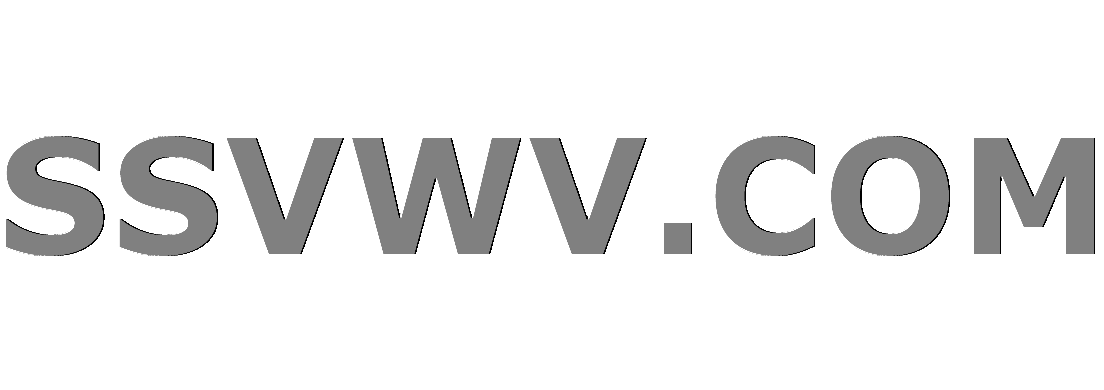
Multi tool use
I have a table of random unique number that they served as account Id for a user.
This scenario will use in Register method of Web API to get first unique Number and attached it into a user and after a successful creation, the fetched unique Number will be removed from database:
public async Task<ActionResult> Register([FromBody] RegisterDto model)
{
//get the first unique number from the database
var UniqueNumber = _context.UniqueNumbers.First();
var user = new User
{
UserName = model.Email,
Email = model.Email,
PhoneNumber = model.PhoneNumber,
FirstName = model.FirstName,
LastName = model.LastName,
UserProfile = new UserProfile()
{
AccountNumber = UniqueNumber.Number,
},
};
//creating user
var createResult = await _userManager.CreateAsync(user, model.Password);
if (!createResult.Succeeded) return BadRequest(new { isSucceeded = createResult.Succeeded, errors = createResult.Errors });
//Delete the fetched UniqueId from the database
_context.UniqueNumbers.Remove(UniqueNumber);
_context.SaveChanges();
return Ok(new
{
isSucceeded = true
});
}
My question is how do I prevent collision in multiple calls on API since it may return same unique number for multiple calls?
c# .net entity-framework rest asp.net-web-api
add a comment |
I have a table of random unique number that they served as account Id for a user.
This scenario will use in Register method of Web API to get first unique Number and attached it into a user and after a successful creation, the fetched unique Number will be removed from database:
public async Task<ActionResult> Register([FromBody] RegisterDto model)
{
//get the first unique number from the database
var UniqueNumber = _context.UniqueNumbers.First();
var user = new User
{
UserName = model.Email,
Email = model.Email,
PhoneNumber = model.PhoneNumber,
FirstName = model.FirstName,
LastName = model.LastName,
UserProfile = new UserProfile()
{
AccountNumber = UniqueNumber.Number,
},
};
//creating user
var createResult = await _userManager.CreateAsync(user, model.Password);
if (!createResult.Succeeded) return BadRequest(new { isSucceeded = createResult.Succeeded, errors = createResult.Errors });
//Delete the fetched UniqueId from the database
_context.UniqueNumbers.Remove(UniqueNumber);
_context.SaveChanges();
return Ok(new
{
isSucceeded = true
});
}
My question is how do I prevent collision in multiple calls on API since it may return same unique number for multiple calls?
c# .net entity-framework rest asp.net-web-api
What database? What version of EF? There's not a generic way to do this.
– David Browne - Microsoft
Nov 12 '18 at 22:16
@DavidBrowne-Microsoft I'm using EF Core 2.1 and MSSQL, I Was thinking about use Transaction Scope but I'm not sure how this works...
– Mehrdad Kamali
Nov 12 '18 at 22:32
Why even go to a table for this? Could you just use a guide type and generate it using a combguid from you c# code.
– Fran
Nov 13 '18 at 13:01
@Fran Well I really need this Id to be a integer value and 10 digits only. (business logic), So no I can't use a GUID.
– Mehrdad Kamali
Nov 13 '18 at 17:40
add a comment |
I have a table of random unique number that they served as account Id for a user.
This scenario will use in Register method of Web API to get first unique Number and attached it into a user and after a successful creation, the fetched unique Number will be removed from database:
public async Task<ActionResult> Register([FromBody] RegisterDto model)
{
//get the first unique number from the database
var UniqueNumber = _context.UniqueNumbers.First();
var user = new User
{
UserName = model.Email,
Email = model.Email,
PhoneNumber = model.PhoneNumber,
FirstName = model.FirstName,
LastName = model.LastName,
UserProfile = new UserProfile()
{
AccountNumber = UniqueNumber.Number,
},
};
//creating user
var createResult = await _userManager.CreateAsync(user, model.Password);
if (!createResult.Succeeded) return BadRequest(new { isSucceeded = createResult.Succeeded, errors = createResult.Errors });
//Delete the fetched UniqueId from the database
_context.UniqueNumbers.Remove(UniqueNumber);
_context.SaveChanges();
return Ok(new
{
isSucceeded = true
});
}
My question is how do I prevent collision in multiple calls on API since it may return same unique number for multiple calls?
c# .net entity-framework rest asp.net-web-api
I have a table of random unique number that they served as account Id for a user.
This scenario will use in Register method of Web API to get first unique Number and attached it into a user and after a successful creation, the fetched unique Number will be removed from database:
public async Task<ActionResult> Register([FromBody] RegisterDto model)
{
//get the first unique number from the database
var UniqueNumber = _context.UniqueNumbers.First();
var user = new User
{
UserName = model.Email,
Email = model.Email,
PhoneNumber = model.PhoneNumber,
FirstName = model.FirstName,
LastName = model.LastName,
UserProfile = new UserProfile()
{
AccountNumber = UniqueNumber.Number,
},
};
//creating user
var createResult = await _userManager.CreateAsync(user, model.Password);
if (!createResult.Succeeded) return BadRequest(new { isSucceeded = createResult.Succeeded, errors = createResult.Errors });
//Delete the fetched UniqueId from the database
_context.UniqueNumbers.Remove(UniqueNumber);
_context.SaveChanges();
return Ok(new
{
isSucceeded = true
});
}
My question is how do I prevent collision in multiple calls on API since it may return same unique number for multiple calls?
c# .net entity-framework rest asp.net-web-api
c# .net entity-framework rest asp.net-web-api
asked Nov 12 '18 at 22:01


Mehrdad KamaliMehrdad Kamali
4019
4019
What database? What version of EF? There's not a generic way to do this.
– David Browne - Microsoft
Nov 12 '18 at 22:16
@DavidBrowne-Microsoft I'm using EF Core 2.1 and MSSQL, I Was thinking about use Transaction Scope but I'm not sure how this works...
– Mehrdad Kamali
Nov 12 '18 at 22:32
Why even go to a table for this? Could you just use a guide type and generate it using a combguid from you c# code.
– Fran
Nov 13 '18 at 13:01
@Fran Well I really need this Id to be a integer value and 10 digits only. (business logic), So no I can't use a GUID.
– Mehrdad Kamali
Nov 13 '18 at 17:40
add a comment |
What database? What version of EF? There's not a generic way to do this.
– David Browne - Microsoft
Nov 12 '18 at 22:16
@DavidBrowne-Microsoft I'm using EF Core 2.1 and MSSQL, I Was thinking about use Transaction Scope but I'm not sure how this works...
– Mehrdad Kamali
Nov 12 '18 at 22:32
Why even go to a table for this? Could you just use a guide type and generate it using a combguid from you c# code.
– Fran
Nov 13 '18 at 13:01
@Fran Well I really need this Id to be a integer value and 10 digits only. (business logic), So no I can't use a GUID.
– Mehrdad Kamali
Nov 13 '18 at 17:40
What database? What version of EF? There's not a generic way to do this.
– David Browne - Microsoft
Nov 12 '18 at 22:16
What database? What version of EF? There's not a generic way to do this.
– David Browne - Microsoft
Nov 12 '18 at 22:16
@DavidBrowne-Microsoft I'm using EF Core 2.1 and MSSQL, I Was thinking about use Transaction Scope but I'm not sure how this works...
– Mehrdad Kamali
Nov 12 '18 at 22:32
@DavidBrowne-Microsoft I'm using EF Core 2.1 and MSSQL, I Was thinking about use Transaction Scope but I'm not sure how this works...
– Mehrdad Kamali
Nov 12 '18 at 22:32
Why even go to a table for this? Could you just use a guide type and generate it using a combguid from you c# code.
– Fran
Nov 13 '18 at 13:01
Why even go to a table for this? Could you just use a guide type and generate it using a combguid from you c# code.
– Fran
Nov 13 '18 at 13:01
@Fran Well I really need this Id to be a integer value and 10 digits only. (business logic), So no I can't use a GUID.
– Mehrdad Kamali
Nov 13 '18 at 17:40
@Fran Well I really need this Id to be a integer value and 10 digits only. (business logic), So no I can't use a GUID.
– Mehrdad Kamali
Nov 13 '18 at 17:40
add a comment |
1 Answer
1
active
oldest
votes
On EF Core and Microsoft SQL Server you should simply use a SEQUENCE object to generate your keys. See Sequences - EF Core
If really, really want to proceed with your original design you could use FromSql to run a DELETE … OUTPUT, something like:
var UniqueNumber = _context.UniqueNumbers.FromSql("delete top (1) from UniqueNumbers output deleted.*").Single();
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270736%2fprevent-collision-on-multiple-database-calls-in-web-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
On EF Core and Microsoft SQL Server you should simply use a SEQUENCE object to generate your keys. See Sequences - EF Core
If really, really want to proceed with your original design you could use FromSql to run a DELETE … OUTPUT, something like:
var UniqueNumber = _context.UniqueNumbers.FromSql("delete top (1) from UniqueNumbers output deleted.*").Single();
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
add a comment |
On EF Core and Microsoft SQL Server you should simply use a SEQUENCE object to generate your keys. See Sequences - EF Core
If really, really want to proceed with your original design you could use FromSql to run a DELETE … OUTPUT, something like:
var UniqueNumber = _context.UniqueNumbers.FromSql("delete top (1) from UniqueNumbers output deleted.*").Single();
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
add a comment |
On EF Core and Microsoft SQL Server you should simply use a SEQUENCE object to generate your keys. See Sequences - EF Core
If really, really want to proceed with your original design you could use FromSql to run a DELETE … OUTPUT, something like:
var UniqueNumber = _context.UniqueNumbers.FromSql("delete top (1) from UniqueNumbers output deleted.*").Single();
On EF Core and Microsoft SQL Server you should simply use a SEQUENCE object to generate your keys. See Sequences - EF Core
If really, really want to proceed with your original design you could use FromSql to run a DELETE … OUTPUT, something like:
var UniqueNumber = _context.UniqueNumbers.FromSql("delete top (1) from UniqueNumbers output deleted.*").Single();
edited Nov 13 '18 at 18:16
answered Nov 13 '18 at 0:05
David Browne - MicrosoftDavid Browne - Microsoft
14k2624
14k2624
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
add a comment |
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
Well the Sequences does not meet my requirement as I said (Not Randomly Generated.), So I think I should stick to my design. However this command will do my work perfectly I think, Many thanks!
– Mehrdad Kamali
Nov 13 '18 at 17:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270736%2fprevent-collision-on-multiple-database-calls-in-web-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FUOi6ctT4aaEWoo9H0ueY6tc,lHZr PcoBNI1k0YmFd,28BuzjzF V,ObC,9
What database? What version of EF? There's not a generic way to do this.
– David Browne - Microsoft
Nov 12 '18 at 22:16
@DavidBrowne-Microsoft I'm using EF Core 2.1 and MSSQL, I Was thinking about use Transaction Scope but I'm not sure how this works...
– Mehrdad Kamali
Nov 12 '18 at 22:32
Why even go to a table for this? Could you just use a guide type and generate it using a combguid from you c# code.
– Fran
Nov 13 '18 at 13:01
@Fran Well I really need this Id to be a integer value and 10 digits only. (business logic), So no I can't use a GUID.
– Mehrdad Kamali
Nov 13 '18 at 17:40