3D reconstruction algorithm implementation is not working
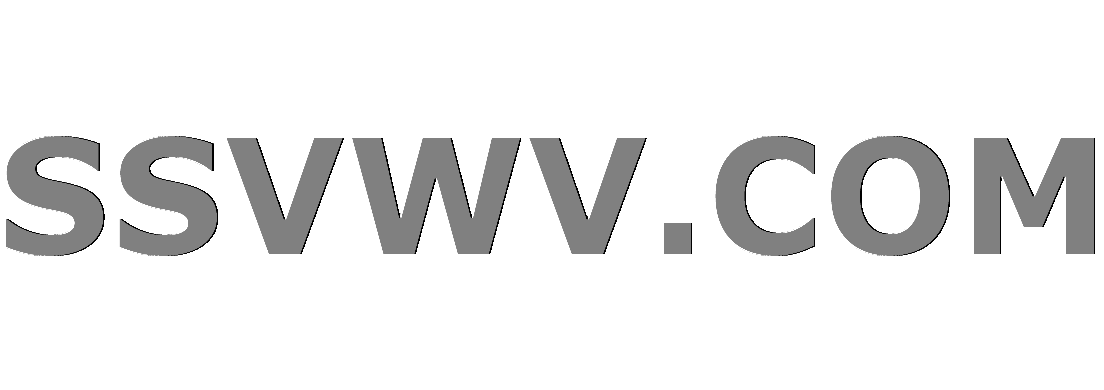
Multi tool use
I am trying to implement an algorithm to reconstruct 3D coordinates with known Z-component from 2D picture coordinates.
Using the Camera Calibration app of matlab, I calculated the intrinsic parameters. I used those and 4 points in the picture that I know the real world coordinates of to get extrinsic parameters.
I then used the method proposed In the original stack overflow post for 3d reconstruction:
After having all matrices, this equation that can help me with
transforming image point to wolrd coordinates:
where M is cameraMatrix, R - rotationMatrix, t - tvec, and s is an
unknown. Zconst represents the height where the orange ball is, in
this example it is 285 mm. So, first I need to solve previous
equation, to get "s", and after I can find out X and Y coordinate by
selecting image point:
%% Intrinsic camera parameters
cameraData = load("CameraParams.mat");
cameraParams = cameraData.cameraParams;
intrinsicMatrix = cameraParams.IntrinsicMatrix';
%% Points with known world coordinates
imagePoints = [224 92; 963 81; 200 653; 988 650];
worldPoints = [0 0; 114 0; 0 85; 114 85];
%% Points with unknown world xy-coordinates
unknownPoints = [416 280; 773 275; 414 479; 778 477];
zOffset = 0;
%% Extrinsic camera parameters
[rotationMatrix, translationVector] = extrinsics(imagePoints, worldPoints, cameraParams);
%% Transform image to world coordinates
results = zeros(length(unknownPoints), 3);
A = inv(intrinsicMatrix * rotationMatrix);
for i = 1:length(unknownPoints)
P = [unknownPoints(i,:) 1]';
AP = A * P;
At = A * translationVector';
s = (zOffset + At(3)) / AP(3);
results(i,:) = s * AP - At;
end
%% Visualization
allWorldPoints = [[worldPoints zeros(size(worldPoints, 1), 1)]; results];
allImagePoints = [imagePoints; unknownPoints];
[orientation, location] = extrinsicsToCameraPose(rotationMatrix, translationVector);
figure;
scatter(allImagePoints(:,1), allImagePoints(:,2));
figure;
plotCamera("Location", location, "Orientation", orientation, "Size", 20);
hold on;
pcshow(allWorldPoints, [0 0 0], "VerticalAxisDir", "down", "MarkerSize", 40);
The original picture looks like this (y-direction is flipped):
Whereas the reconstruction looks like this:
As you can see, the 4 fixed points used to compute the extrinsic parameters are displayed correctly but the reconstructed ones are in the wrong spots.
What is causing this error and how can I fix it?
matlab computer-vision projection 3d-reconstruction
add a comment |
I am trying to implement an algorithm to reconstruct 3D coordinates with known Z-component from 2D picture coordinates.
Using the Camera Calibration app of matlab, I calculated the intrinsic parameters. I used those and 4 points in the picture that I know the real world coordinates of to get extrinsic parameters.
I then used the method proposed In the original stack overflow post for 3d reconstruction:
After having all matrices, this equation that can help me with
transforming image point to wolrd coordinates:
where M is cameraMatrix, R - rotationMatrix, t - tvec, and s is an
unknown. Zconst represents the height where the orange ball is, in
this example it is 285 mm. So, first I need to solve previous
equation, to get "s", and after I can find out X and Y coordinate by
selecting image point:
%% Intrinsic camera parameters
cameraData = load("CameraParams.mat");
cameraParams = cameraData.cameraParams;
intrinsicMatrix = cameraParams.IntrinsicMatrix';
%% Points with known world coordinates
imagePoints = [224 92; 963 81; 200 653; 988 650];
worldPoints = [0 0; 114 0; 0 85; 114 85];
%% Points with unknown world xy-coordinates
unknownPoints = [416 280; 773 275; 414 479; 778 477];
zOffset = 0;
%% Extrinsic camera parameters
[rotationMatrix, translationVector] = extrinsics(imagePoints, worldPoints, cameraParams);
%% Transform image to world coordinates
results = zeros(length(unknownPoints), 3);
A = inv(intrinsicMatrix * rotationMatrix);
for i = 1:length(unknownPoints)
P = [unknownPoints(i,:) 1]';
AP = A * P;
At = A * translationVector';
s = (zOffset + At(3)) / AP(3);
results(i,:) = s * AP - At;
end
%% Visualization
allWorldPoints = [[worldPoints zeros(size(worldPoints, 1), 1)]; results];
allImagePoints = [imagePoints; unknownPoints];
[orientation, location] = extrinsicsToCameraPose(rotationMatrix, translationVector);
figure;
scatter(allImagePoints(:,1), allImagePoints(:,2));
figure;
plotCamera("Location", location, "Orientation", orientation, "Size", 20);
hold on;
pcshow(allWorldPoints, [0 0 0], "VerticalAxisDir", "down", "MarkerSize", 40);
The original picture looks like this (y-direction is flipped):
Whereas the reconstruction looks like this:
As you can see, the 4 fixed points used to compute the extrinsic parameters are displayed correctly but the reconstructed ones are in the wrong spots.
What is causing this error and how can I fix it?
matlab computer-vision projection 3d-reconstruction
add a comment |
I am trying to implement an algorithm to reconstruct 3D coordinates with known Z-component from 2D picture coordinates.
Using the Camera Calibration app of matlab, I calculated the intrinsic parameters. I used those and 4 points in the picture that I know the real world coordinates of to get extrinsic parameters.
I then used the method proposed In the original stack overflow post for 3d reconstruction:
After having all matrices, this equation that can help me with
transforming image point to wolrd coordinates:
where M is cameraMatrix, R - rotationMatrix, t - tvec, and s is an
unknown. Zconst represents the height where the orange ball is, in
this example it is 285 mm. So, first I need to solve previous
equation, to get "s", and after I can find out X and Y coordinate by
selecting image point:
%% Intrinsic camera parameters
cameraData = load("CameraParams.mat");
cameraParams = cameraData.cameraParams;
intrinsicMatrix = cameraParams.IntrinsicMatrix';
%% Points with known world coordinates
imagePoints = [224 92; 963 81; 200 653; 988 650];
worldPoints = [0 0; 114 0; 0 85; 114 85];
%% Points with unknown world xy-coordinates
unknownPoints = [416 280; 773 275; 414 479; 778 477];
zOffset = 0;
%% Extrinsic camera parameters
[rotationMatrix, translationVector] = extrinsics(imagePoints, worldPoints, cameraParams);
%% Transform image to world coordinates
results = zeros(length(unknownPoints), 3);
A = inv(intrinsicMatrix * rotationMatrix);
for i = 1:length(unknownPoints)
P = [unknownPoints(i,:) 1]';
AP = A * P;
At = A * translationVector';
s = (zOffset + At(3)) / AP(3);
results(i,:) = s * AP - At;
end
%% Visualization
allWorldPoints = [[worldPoints zeros(size(worldPoints, 1), 1)]; results];
allImagePoints = [imagePoints; unknownPoints];
[orientation, location] = extrinsicsToCameraPose(rotationMatrix, translationVector);
figure;
scatter(allImagePoints(:,1), allImagePoints(:,2));
figure;
plotCamera("Location", location, "Orientation", orientation, "Size", 20);
hold on;
pcshow(allWorldPoints, [0 0 0], "VerticalAxisDir", "down", "MarkerSize", 40);
The original picture looks like this (y-direction is flipped):
Whereas the reconstruction looks like this:
As you can see, the 4 fixed points used to compute the extrinsic parameters are displayed correctly but the reconstructed ones are in the wrong spots.
What is causing this error and how can I fix it?
matlab computer-vision projection 3d-reconstruction
I am trying to implement an algorithm to reconstruct 3D coordinates with known Z-component from 2D picture coordinates.
Using the Camera Calibration app of matlab, I calculated the intrinsic parameters. I used those and 4 points in the picture that I know the real world coordinates of to get extrinsic parameters.
I then used the method proposed In the original stack overflow post for 3d reconstruction:
After having all matrices, this equation that can help me with
transforming image point to wolrd coordinates:
where M is cameraMatrix, R - rotationMatrix, t - tvec, and s is an
unknown. Zconst represents the height where the orange ball is, in
this example it is 285 mm. So, first I need to solve previous
equation, to get "s", and after I can find out X and Y coordinate by
selecting image point:
%% Intrinsic camera parameters
cameraData = load("CameraParams.mat");
cameraParams = cameraData.cameraParams;
intrinsicMatrix = cameraParams.IntrinsicMatrix';
%% Points with known world coordinates
imagePoints = [224 92; 963 81; 200 653; 988 650];
worldPoints = [0 0; 114 0; 0 85; 114 85];
%% Points with unknown world xy-coordinates
unknownPoints = [416 280; 773 275; 414 479; 778 477];
zOffset = 0;
%% Extrinsic camera parameters
[rotationMatrix, translationVector] = extrinsics(imagePoints, worldPoints, cameraParams);
%% Transform image to world coordinates
results = zeros(length(unknownPoints), 3);
A = inv(intrinsicMatrix * rotationMatrix);
for i = 1:length(unknownPoints)
P = [unknownPoints(i,:) 1]';
AP = A * P;
At = A * translationVector';
s = (zOffset + At(3)) / AP(3);
results(i,:) = s * AP - At;
end
%% Visualization
allWorldPoints = [[worldPoints zeros(size(worldPoints, 1), 1)]; results];
allImagePoints = [imagePoints; unknownPoints];
[orientation, location] = extrinsicsToCameraPose(rotationMatrix, translationVector);
figure;
scatter(allImagePoints(:,1), allImagePoints(:,2));
figure;
plotCamera("Location", location, "Orientation", orientation, "Size", 20);
hold on;
pcshow(allWorldPoints, [0 0 0], "VerticalAxisDir", "down", "MarkerSize", 40);
The original picture looks like this (y-direction is flipped):
Whereas the reconstruction looks like this:
As you can see, the 4 fixed points used to compute the extrinsic parameters are displayed correctly but the reconstructed ones are in the wrong spots.
What is causing this error and how can I fix it?
matlab computer-vision projection 3d-reconstruction
matlab computer-vision projection 3d-reconstruction
edited Nov 18 '18 at 9:26
RobinW
asked Nov 17 '18 at 12:42
RobinWRobinW
7111
7111
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53351367%2f3d-reconstruction-algorithm-implementation-is-not-working%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53351367%2f3d-reconstruction-algorithm-implementation-is-not-working%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gzT,D,b4DYfAM9BIwSJRRB9efQSsby4FRD75rY7Ah8X4,V,JKrBAJaqZ