Most efficient way to instantiate large number of subclasses in Java?
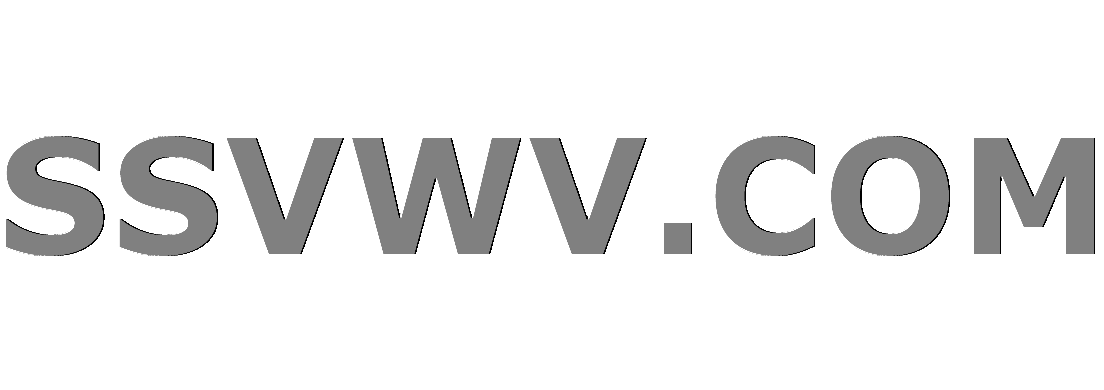
Multi tool use
I am working on a project that requires the use of a large number of objects of classes extending a particular abstract class. Instantiating manually requires a call to each object's individual constructor, which is starting to pollute my client class.
The raw code from the project is too lengthy to post on this site, so I have a simplified example below:
Suppose there is an abstract class called Letter:
public abstract class Letter{
String letter;
}
And 26 individual letter classes that extend the Letter abstract class:
public class A extends Letter{
this.letter = "A";
}
public class B extends Letter{
this.letter = "B";
}
... and so on
This is where I seek help - There is a client class called Alphabet:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = new ArrayList<>();
A a = new A();
B b = new B();
C c = new C();
list.add(a);
list.add(b);
list.add(c);
...the list goes on
return letters;
}
}
As you can see, it is not ideal to instantiate each letter manually. I am looking for a way to create a list of class objects dynamically at runtime.
Perhaps something similar to this:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = getAllClassesExtending(Letter.class);
return letters;
//list would then contain A,B,C,D,E,F,G, etc.
}
}
I ultimately want to be able to create a new class and have it automatically added to a list of objects in the client class, without needing to modify the client class (e.g. I do not want to explicitly reference each letter class in the client class).
java class dynamic reflection loading
add a comment |
I am working on a project that requires the use of a large number of objects of classes extending a particular abstract class. Instantiating manually requires a call to each object's individual constructor, which is starting to pollute my client class.
The raw code from the project is too lengthy to post on this site, so I have a simplified example below:
Suppose there is an abstract class called Letter:
public abstract class Letter{
String letter;
}
And 26 individual letter classes that extend the Letter abstract class:
public class A extends Letter{
this.letter = "A";
}
public class B extends Letter{
this.letter = "B";
}
... and so on
This is where I seek help - There is a client class called Alphabet:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = new ArrayList<>();
A a = new A();
B b = new B();
C c = new C();
list.add(a);
list.add(b);
list.add(c);
...the list goes on
return letters;
}
}
As you can see, it is not ideal to instantiate each letter manually. I am looking for a way to create a list of class objects dynamically at runtime.
Perhaps something similar to this:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = getAllClassesExtending(Letter.class);
return letters;
//list would then contain A,B,C,D,E,F,G, etc.
}
}
I ultimately want to be able to create a new class and have it automatically added to a list of objects in the client class, without needing to modify the client class (e.g. I do not want to explicitly reference each letter class in the client class).
java class dynamic reflection loading
2
Why do you think you need 26 different classes? Why not just use theLetter
class, but make a constructor where you pass in the letter?
– Dawood ibn Kareem
Nov 20 '18 at 18:48
The use/case for having a class per letter of the alphabet remains unclear or better said strange. But, if you need this, reflection is the way to go. Needs still an external project, with built-in methods it's afaik impossible - you need to scan the classpath
– AKSW
Nov 20 '18 at 19:00
Maybe usingServiceLoader
is a viable option? See also stackoverflow.com/questions/10304100/…
– Daniele
Nov 20 '18 at 19:50
The letter scenario serves only as an illustration of my problem. Yes, there are much better ways to do the letter implementation, but my real project involves many subclasses that contain unique code that can't simply be 'passed in' by a constructor.
– Thicc
Nov 20 '18 at 19:56
Is there anything I can put in the subclasses themselves so that they can add themselves to the list at runtime? Maybe an initializer?
– Thicc
Nov 20 '18 at 20:25
add a comment |
I am working on a project that requires the use of a large number of objects of classes extending a particular abstract class. Instantiating manually requires a call to each object's individual constructor, which is starting to pollute my client class.
The raw code from the project is too lengthy to post on this site, so I have a simplified example below:
Suppose there is an abstract class called Letter:
public abstract class Letter{
String letter;
}
And 26 individual letter classes that extend the Letter abstract class:
public class A extends Letter{
this.letter = "A";
}
public class B extends Letter{
this.letter = "B";
}
... and so on
This is where I seek help - There is a client class called Alphabet:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = new ArrayList<>();
A a = new A();
B b = new B();
C c = new C();
list.add(a);
list.add(b);
list.add(c);
...the list goes on
return letters;
}
}
As you can see, it is not ideal to instantiate each letter manually. I am looking for a way to create a list of class objects dynamically at runtime.
Perhaps something similar to this:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = getAllClassesExtending(Letter.class);
return letters;
//list would then contain A,B,C,D,E,F,G, etc.
}
}
I ultimately want to be able to create a new class and have it automatically added to a list of objects in the client class, without needing to modify the client class (e.g. I do not want to explicitly reference each letter class in the client class).
java class dynamic reflection loading
I am working on a project that requires the use of a large number of objects of classes extending a particular abstract class. Instantiating manually requires a call to each object's individual constructor, which is starting to pollute my client class.
The raw code from the project is too lengthy to post on this site, so I have a simplified example below:
Suppose there is an abstract class called Letter:
public abstract class Letter{
String letter;
}
And 26 individual letter classes that extend the Letter abstract class:
public class A extends Letter{
this.letter = "A";
}
public class B extends Letter{
this.letter = "B";
}
... and so on
This is where I seek help - There is a client class called Alphabet:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = new ArrayList<>();
A a = new A();
B b = new B();
C c = new C();
list.add(a);
list.add(b);
list.add(c);
...the list goes on
return letters;
}
}
As you can see, it is not ideal to instantiate each letter manually. I am looking for a way to create a list of class objects dynamically at runtime.
Perhaps something similar to this:
public class Alphabet{
public List<Letter> getLetters(){
List<Letter> letters = getAllClassesExtending(Letter.class);
return letters;
//list would then contain A,B,C,D,E,F,G, etc.
}
}
I ultimately want to be able to create a new class and have it automatically added to a list of objects in the client class, without needing to modify the client class (e.g. I do not want to explicitly reference each letter class in the client class).
java class dynamic reflection loading
java class dynamic reflection loading
edited Nov 20 '18 at 18:43
Thicc
asked Nov 20 '18 at 18:24
ThiccThicc
61
61
2
Why do you think you need 26 different classes? Why not just use theLetter
class, but make a constructor where you pass in the letter?
– Dawood ibn Kareem
Nov 20 '18 at 18:48
The use/case for having a class per letter of the alphabet remains unclear or better said strange. But, if you need this, reflection is the way to go. Needs still an external project, with built-in methods it's afaik impossible - you need to scan the classpath
– AKSW
Nov 20 '18 at 19:00
Maybe usingServiceLoader
is a viable option? See also stackoverflow.com/questions/10304100/…
– Daniele
Nov 20 '18 at 19:50
The letter scenario serves only as an illustration of my problem. Yes, there are much better ways to do the letter implementation, but my real project involves many subclasses that contain unique code that can't simply be 'passed in' by a constructor.
– Thicc
Nov 20 '18 at 19:56
Is there anything I can put in the subclasses themselves so that they can add themselves to the list at runtime? Maybe an initializer?
– Thicc
Nov 20 '18 at 20:25
add a comment |
2
Why do you think you need 26 different classes? Why not just use theLetter
class, but make a constructor where you pass in the letter?
– Dawood ibn Kareem
Nov 20 '18 at 18:48
The use/case for having a class per letter of the alphabet remains unclear or better said strange. But, if you need this, reflection is the way to go. Needs still an external project, with built-in methods it's afaik impossible - you need to scan the classpath
– AKSW
Nov 20 '18 at 19:00
Maybe usingServiceLoader
is a viable option? See also stackoverflow.com/questions/10304100/…
– Daniele
Nov 20 '18 at 19:50
The letter scenario serves only as an illustration of my problem. Yes, there are much better ways to do the letter implementation, but my real project involves many subclasses that contain unique code that can't simply be 'passed in' by a constructor.
– Thicc
Nov 20 '18 at 19:56
Is there anything I can put in the subclasses themselves so that they can add themselves to the list at runtime? Maybe an initializer?
– Thicc
Nov 20 '18 at 20:25
2
2
Why do you think you need 26 different classes? Why not just use the
Letter
class, but make a constructor where you pass in the letter?– Dawood ibn Kareem
Nov 20 '18 at 18:48
Why do you think you need 26 different classes? Why not just use the
Letter
class, but make a constructor where you pass in the letter?– Dawood ibn Kareem
Nov 20 '18 at 18:48
The use/case for having a class per letter of the alphabet remains unclear or better said strange. But, if you need this, reflection is the way to go. Needs still an external project, with built-in methods it's afaik impossible - you need to scan the classpath
– AKSW
Nov 20 '18 at 19:00
The use/case for having a class per letter of the alphabet remains unclear or better said strange. But, if you need this, reflection is the way to go. Needs still an external project, with built-in methods it's afaik impossible - you need to scan the classpath
– AKSW
Nov 20 '18 at 19:00
Maybe using
ServiceLoader
is a viable option? See also stackoverflow.com/questions/10304100/…– Daniele
Nov 20 '18 at 19:50
Maybe using
ServiceLoader
is a viable option? See also stackoverflow.com/questions/10304100/…– Daniele
Nov 20 '18 at 19:50
The letter scenario serves only as an illustration of my problem. Yes, there are much better ways to do the letter implementation, but my real project involves many subclasses that contain unique code that can't simply be 'passed in' by a constructor.
– Thicc
Nov 20 '18 at 19:56
The letter scenario serves only as an illustration of my problem. Yes, there are much better ways to do the letter implementation, but my real project involves many subclasses that contain unique code that can't simply be 'passed in' by a constructor.
– Thicc
Nov 20 '18 at 19:56
Is there anything I can put in the subclasses themselves so that they can add themselves to the list at runtime? Maybe an initializer?
– Thicc
Nov 20 '18 at 20:25
Is there anything I can put in the subclasses themselves so that they can add themselves to the list at runtime? Maybe an initializer?
– Thicc
Nov 20 '18 at 20:25
add a comment |
2 Answers
2
active
oldest
votes
This sounds like a typical use case for an enum
. The constants of an enum
can have a class body with code, overriding methods of the base type, and even declare new fields.
The task of getting an instance of all subtypes implies a closed set of subtypes, which matches the behavior of enum
types.
public enum Letter {
A {
@Override
public boolean isVowel() {
return true;
}
},
B, C, D,
E {
@Override
public boolean isVowel() {
return true;
}
},
F, G, H,
I {
@Override
public boolean isVowel() {
return true;
}
}, J, K, L, M, N,
O {
@Override
public boolean isVowel() {
return true;
}
}, P, Q, R, S, T,
U {
@Override
public boolean isVowel() {
return true;
}
}, V, W, X, Y, Z;
;
String letter = name();
public static List<Letter> getLetters() {
return Arrays.asList(values());
}
public boolean isVowel() {
return false;
}
}
This demonstrates that overriding methods is possible and also a method for getting all instances (as an array) comes for free. Further, the names are specified intrinsically without redundancy.
If you want to have an open set of subclasses instead, to be potentially extended after compiling the code, you should look at the Service Provider concept and the ServiceLoader
class. This mechanism will not automatically find subclasses but only those declared as service providers either within a file in META-INF
or through the module declararation, on the other hand, it provides you with a robust, established solution that will be maintained by the Java developers or even evolve with the platform, like the integration into the new modules concept.
add a comment |
You can use Reflections library to find the classes in runtime, e.g. if all of them are located under com.letters
package and have a no-arg constructor:
public List<? extends Letter> getLetters(){
Reflections reflections = new Reflections("com.letters");
Set<Class<? extends Letter>> classes = reflections.getSubTypesOf(Letter.class);
return classes.stream()
.map(clazz -> {
try {
return clazz.newInstance();
} catch (InstantiationException | IllegalAccessException ex) {
throw new RuntimeException(ex);
}
})
.collect(Collectors.toList());
}
It's not the most efficient way to lookup classes, you might want to cache the results if this code is on your critical path.
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399238%2fmost-efficient-way-to-instantiate-large-number-of-subclasses-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
This sounds like a typical use case for an enum
. The constants of an enum
can have a class body with code, overriding methods of the base type, and even declare new fields.
The task of getting an instance of all subtypes implies a closed set of subtypes, which matches the behavior of enum
types.
public enum Letter {
A {
@Override
public boolean isVowel() {
return true;
}
},
B, C, D,
E {
@Override
public boolean isVowel() {
return true;
}
},
F, G, H,
I {
@Override
public boolean isVowel() {
return true;
}
}, J, K, L, M, N,
O {
@Override
public boolean isVowel() {
return true;
}
}, P, Q, R, S, T,
U {
@Override
public boolean isVowel() {
return true;
}
}, V, W, X, Y, Z;
;
String letter = name();
public static List<Letter> getLetters() {
return Arrays.asList(values());
}
public boolean isVowel() {
return false;
}
}
This demonstrates that overriding methods is possible and also a method for getting all instances (as an array) comes for free. Further, the names are specified intrinsically without redundancy.
If you want to have an open set of subclasses instead, to be potentially extended after compiling the code, you should look at the Service Provider concept and the ServiceLoader
class. This mechanism will not automatically find subclasses but only those declared as service providers either within a file in META-INF
or through the module declararation, on the other hand, it provides you with a robust, established solution that will be maintained by the Java developers or even evolve with the platform, like the integration into the new modules concept.
add a comment |
This sounds like a typical use case for an enum
. The constants of an enum
can have a class body with code, overriding methods of the base type, and even declare new fields.
The task of getting an instance of all subtypes implies a closed set of subtypes, which matches the behavior of enum
types.
public enum Letter {
A {
@Override
public boolean isVowel() {
return true;
}
},
B, C, D,
E {
@Override
public boolean isVowel() {
return true;
}
},
F, G, H,
I {
@Override
public boolean isVowel() {
return true;
}
}, J, K, L, M, N,
O {
@Override
public boolean isVowel() {
return true;
}
}, P, Q, R, S, T,
U {
@Override
public boolean isVowel() {
return true;
}
}, V, W, X, Y, Z;
;
String letter = name();
public static List<Letter> getLetters() {
return Arrays.asList(values());
}
public boolean isVowel() {
return false;
}
}
This demonstrates that overriding methods is possible and also a method for getting all instances (as an array) comes for free. Further, the names are specified intrinsically without redundancy.
If you want to have an open set of subclasses instead, to be potentially extended after compiling the code, you should look at the Service Provider concept and the ServiceLoader
class. This mechanism will not automatically find subclasses but only those declared as service providers either within a file in META-INF
or through the module declararation, on the other hand, it provides you with a robust, established solution that will be maintained by the Java developers or even evolve with the platform, like the integration into the new modules concept.
add a comment |
This sounds like a typical use case for an enum
. The constants of an enum
can have a class body with code, overriding methods of the base type, and even declare new fields.
The task of getting an instance of all subtypes implies a closed set of subtypes, which matches the behavior of enum
types.
public enum Letter {
A {
@Override
public boolean isVowel() {
return true;
}
},
B, C, D,
E {
@Override
public boolean isVowel() {
return true;
}
},
F, G, H,
I {
@Override
public boolean isVowel() {
return true;
}
}, J, K, L, M, N,
O {
@Override
public boolean isVowel() {
return true;
}
}, P, Q, R, S, T,
U {
@Override
public boolean isVowel() {
return true;
}
}, V, W, X, Y, Z;
;
String letter = name();
public static List<Letter> getLetters() {
return Arrays.asList(values());
}
public boolean isVowel() {
return false;
}
}
This demonstrates that overriding methods is possible and also a method for getting all instances (as an array) comes for free. Further, the names are specified intrinsically without redundancy.
If you want to have an open set of subclasses instead, to be potentially extended after compiling the code, you should look at the Service Provider concept and the ServiceLoader
class. This mechanism will not automatically find subclasses but only those declared as service providers either within a file in META-INF
or through the module declararation, on the other hand, it provides you with a robust, established solution that will be maintained by the Java developers or even evolve with the platform, like the integration into the new modules concept.
This sounds like a typical use case for an enum
. The constants of an enum
can have a class body with code, overriding methods of the base type, and even declare new fields.
The task of getting an instance of all subtypes implies a closed set of subtypes, which matches the behavior of enum
types.
public enum Letter {
A {
@Override
public boolean isVowel() {
return true;
}
},
B, C, D,
E {
@Override
public boolean isVowel() {
return true;
}
},
F, G, H,
I {
@Override
public boolean isVowel() {
return true;
}
}, J, K, L, M, N,
O {
@Override
public boolean isVowel() {
return true;
}
}, P, Q, R, S, T,
U {
@Override
public boolean isVowel() {
return true;
}
}, V, W, X, Y, Z;
;
String letter = name();
public static List<Letter> getLetters() {
return Arrays.asList(values());
}
public boolean isVowel() {
return false;
}
}
This demonstrates that overriding methods is possible and also a method for getting all instances (as an array) comes for free. Further, the names are specified intrinsically without redundancy.
If you want to have an open set of subclasses instead, to be potentially extended after compiling the code, you should look at the Service Provider concept and the ServiceLoader
class. This mechanism will not automatically find subclasses but only those declared as service providers either within a file in META-INF
or through the module declararation, on the other hand, it provides you with a robust, established solution that will be maintained by the Java developers or even evolve with the platform, like the integration into the new modules concept.
answered Nov 22 '18 at 10:48


HolgerHolger
168k23237452
168k23237452
add a comment |
add a comment |
You can use Reflections library to find the classes in runtime, e.g. if all of them are located under com.letters
package and have a no-arg constructor:
public List<? extends Letter> getLetters(){
Reflections reflections = new Reflections("com.letters");
Set<Class<? extends Letter>> classes = reflections.getSubTypesOf(Letter.class);
return classes.stream()
.map(clazz -> {
try {
return clazz.newInstance();
} catch (InstantiationException | IllegalAccessException ex) {
throw new RuntimeException(ex);
}
})
.collect(Collectors.toList());
}
It's not the most efficient way to lookup classes, you might want to cache the results if this code is on your critical path.
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
add a comment |
You can use Reflections library to find the classes in runtime, e.g. if all of them are located under com.letters
package and have a no-arg constructor:
public List<? extends Letter> getLetters(){
Reflections reflections = new Reflections("com.letters");
Set<Class<? extends Letter>> classes = reflections.getSubTypesOf(Letter.class);
return classes.stream()
.map(clazz -> {
try {
return clazz.newInstance();
} catch (InstantiationException | IllegalAccessException ex) {
throw new RuntimeException(ex);
}
})
.collect(Collectors.toList());
}
It's not the most efficient way to lookup classes, you might want to cache the results if this code is on your critical path.
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
add a comment |
You can use Reflections library to find the classes in runtime, e.g. if all of them are located under com.letters
package and have a no-arg constructor:
public List<? extends Letter> getLetters(){
Reflections reflections = new Reflections("com.letters");
Set<Class<? extends Letter>> classes = reflections.getSubTypesOf(Letter.class);
return classes.stream()
.map(clazz -> {
try {
return clazz.newInstance();
} catch (InstantiationException | IllegalAccessException ex) {
throw new RuntimeException(ex);
}
})
.collect(Collectors.toList());
}
It's not the most efficient way to lookup classes, you might want to cache the results if this code is on your critical path.
You can use Reflections library to find the classes in runtime, e.g. if all of them are located under com.letters
package and have a no-arg constructor:
public List<? extends Letter> getLetters(){
Reflections reflections = new Reflections("com.letters");
Set<Class<? extends Letter>> classes = reflections.getSubTypesOf(Letter.class);
return classes.stream()
.map(clazz -> {
try {
return clazz.newInstance();
} catch (InstantiationException | IllegalAccessException ex) {
throw new RuntimeException(ex);
}
})
.collect(Collectors.toList());
}
It's not the most efficient way to lookup classes, you might want to cache the results if this code is on your critical path.
edited Nov 21 '18 at 10:35
answered Nov 21 '18 at 10:20


Karol DowbeckiKarol Dowbecki
22.1k93254
22.1k93254
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
add a comment |
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
I'm having trouble installing the Reflections library in my Java project. Is there a jar or zip with all of the compiled classes? (using maven is not currently an option for me).
– Thicc
Nov 21 '18 at 17:39
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
@Thicc you can take a look at reflections pom.xml to figure out transitive dependencies of the library. It is however a mundane task.
– Karol Dowbecki
Nov 21 '18 at 17:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399238%2fmost-efficient-way-to-instantiate-large-number-of-subclasses-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8gDff Hy OgYBYUS2i0
2
Why do you think you need 26 different classes? Why not just use the
Letter
class, but make a constructor where you pass in the letter?– Dawood ibn Kareem
Nov 20 '18 at 18:48
The use/case for having a class per letter of the alphabet remains unclear or better said strange. But, if you need this, reflection is the way to go. Needs still an external project, with built-in methods it's afaik impossible - you need to scan the classpath
– AKSW
Nov 20 '18 at 19:00
Maybe using
ServiceLoader
is a viable option? See also stackoverflow.com/questions/10304100/…– Daniele
Nov 20 '18 at 19:50
The letter scenario serves only as an illustration of my problem. Yes, there are much better ways to do the letter implementation, but my real project involves many subclasses that contain unique code that can't simply be 'passed in' by a constructor.
– Thicc
Nov 20 '18 at 19:56
Is there anything I can put in the subclasses themselves so that they can add themselves to the list at runtime? Maybe an initializer?
– Thicc
Nov 20 '18 at 20:25