Flutter - BLoC pattern - How to use streams to invoke a method of another widget, i.e. an animation?
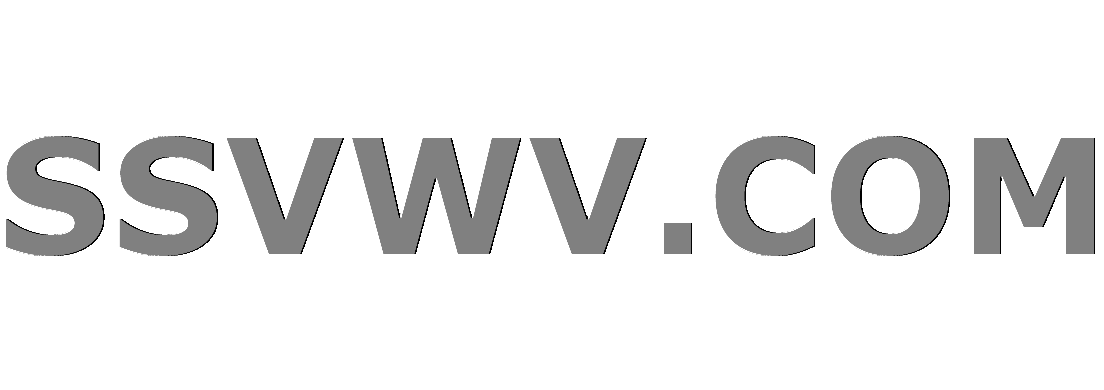
Multi tool use
Suppose there is a widget with a method controlling visibility animation, toggleVisibility()
. In a BLoC pattern, I want to use a stream to invoke that function. I find this pretty tricky.
- Since it is an animation rather than a complete redraw, a StreamBuilder doesn't fit.
Manually add a listener to the BLoC streams isn't convenient either.
- In
initeState()
function of the target widget, we don't have a context, so it's hard to get a reference to the BLoC.
Edit: This is not the case after I read Rémi Rousselet's answer. We can access the context even outside of
build()
function, becauseState<T>
class has a property named 'context' and is documented in Flutter's docs.... I wasn't aware of that.
- In
build(context)
function of the target widget, we have the context. But the widget can be frequently re-built, so you have to manually clean the outdated subscriptions. Otherwise it will create tons of garbages.
- In
A hack with StreamBuilder can do, since the StreamBuilder has implemented all the subscription and unsubscription functionalities. Insert a StreamBuilder somewhere in the layout of the target widget.
StreamBuilder(
stream: Bloc.of(context).stream,
builder: (context, snapshot){
toggleVisiblity();
return Container():
}
);
But this is really a hack. It mixed layout with logic and introduced a useless widget which could cause layout bugs.
So I wonder if there is a good way of doing this in flutter.
dart

add a comment |
Suppose there is a widget with a method controlling visibility animation, toggleVisibility()
. In a BLoC pattern, I want to use a stream to invoke that function. I find this pretty tricky.
- Since it is an animation rather than a complete redraw, a StreamBuilder doesn't fit.
Manually add a listener to the BLoC streams isn't convenient either.
- In
initeState()
function of the target widget, we don't have a context, so it's hard to get a reference to the BLoC.
Edit: This is not the case after I read Rémi Rousselet's answer. We can access the context even outside of
build()
function, becauseState<T>
class has a property named 'context' and is documented in Flutter's docs.... I wasn't aware of that.
- In
build(context)
function of the target widget, we have the context. But the widget can be frequently re-built, so you have to manually clean the outdated subscriptions. Otherwise it will create tons of garbages.
- In
A hack with StreamBuilder can do, since the StreamBuilder has implemented all the subscription and unsubscription functionalities. Insert a StreamBuilder somewhere in the layout of the target widget.
StreamBuilder(
stream: Bloc.of(context).stream,
builder: (context, snapshot){
toggleVisiblity();
return Container():
}
);
But this is really a hack. It mixed layout with logic and introduced a useless widget which could cause layout bugs.
So I wonder if there is a good way of doing this in flutter.
dart

add a comment |
Suppose there is a widget with a method controlling visibility animation, toggleVisibility()
. In a BLoC pattern, I want to use a stream to invoke that function. I find this pretty tricky.
- Since it is an animation rather than a complete redraw, a StreamBuilder doesn't fit.
Manually add a listener to the BLoC streams isn't convenient either.
- In
initeState()
function of the target widget, we don't have a context, so it's hard to get a reference to the BLoC.
Edit: This is not the case after I read Rémi Rousselet's answer. We can access the context even outside of
build()
function, becauseState<T>
class has a property named 'context' and is documented in Flutter's docs.... I wasn't aware of that.
- In
build(context)
function of the target widget, we have the context. But the widget can be frequently re-built, so you have to manually clean the outdated subscriptions. Otherwise it will create tons of garbages.
- In
A hack with StreamBuilder can do, since the StreamBuilder has implemented all the subscription and unsubscription functionalities. Insert a StreamBuilder somewhere in the layout of the target widget.
StreamBuilder(
stream: Bloc.of(context).stream,
builder: (context, snapshot){
toggleVisiblity();
return Container():
}
);
But this is really a hack. It mixed layout with logic and introduced a useless widget which could cause layout bugs.
So I wonder if there is a good way of doing this in flutter.
dart

Suppose there is a widget with a method controlling visibility animation, toggleVisibility()
. In a BLoC pattern, I want to use a stream to invoke that function. I find this pretty tricky.
- Since it is an animation rather than a complete redraw, a StreamBuilder doesn't fit.
Manually add a listener to the BLoC streams isn't convenient either.
- In
initeState()
function of the target widget, we don't have a context, so it's hard to get a reference to the BLoC.
Edit: This is not the case after I read Rémi Rousselet's answer. We can access the context even outside of
build()
function, becauseState<T>
class has a property named 'context' and is documented in Flutter's docs.... I wasn't aware of that.
- In
build(context)
function of the target widget, we have the context. But the widget can be frequently re-built, so you have to manually clean the outdated subscriptions. Otherwise it will create tons of garbages.
- In
A hack with StreamBuilder can do, since the StreamBuilder has implemented all the subscription and unsubscription functionalities. Insert a StreamBuilder somewhere in the layout of the target widget.
StreamBuilder(
stream: Bloc.of(context).stream,
builder: (context, snapshot){
toggleVisiblity();
return Container():
}
);
But this is really a hack. It mixed layout with logic and introduced a useless widget which could cause layout bugs.
So I wonder if there is a good way of doing this in flutter.
dart

dart

edited Nov 23 '18 at 13:55
First_Strike
asked Nov 23 '18 at 9:25


First_StrikeFirst_Strike
1599
1599
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You cannot use StreamBuilder
to do that. You have to manually listen to the stream
class Example extends StatefulWidget {
@override
ExampleState createState() => ExampleState();
}
class ExampleState extends State<Example> {
StreamSubscription subscription;
@override
void didChangeDependencies() {
super.didChangeDependencies();
Stream stream = Bloc.of(context).foo;
subscription?.cancel();
subscription = stream.listen((value) {
// do something
});
}
@override
void dispose() {
subscription?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53443827%2fflutter-bloc-pattern-how-to-use-streams-to-invoke-a-method-of-another-widget%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You cannot use StreamBuilder
to do that. You have to manually listen to the stream
class Example extends StatefulWidget {
@override
ExampleState createState() => ExampleState();
}
class ExampleState extends State<Example> {
StreamSubscription subscription;
@override
void didChangeDependencies() {
super.didChangeDependencies();
Stream stream = Bloc.of(context).foo;
subscription?.cancel();
subscription = stream.listen((value) {
// do something
});
}
@override
void dispose() {
subscription?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
add a comment |
You cannot use StreamBuilder
to do that. You have to manually listen to the stream
class Example extends StatefulWidget {
@override
ExampleState createState() => ExampleState();
}
class ExampleState extends State<Example> {
StreamSubscription subscription;
@override
void didChangeDependencies() {
super.didChangeDependencies();
Stream stream = Bloc.of(context).foo;
subscription?.cancel();
subscription = stream.listen((value) {
// do something
});
}
@override
void dispose() {
subscription?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
add a comment |
You cannot use StreamBuilder
to do that. You have to manually listen to the stream
class Example extends StatefulWidget {
@override
ExampleState createState() => ExampleState();
}
class ExampleState extends State<Example> {
StreamSubscription subscription;
@override
void didChangeDependencies() {
super.didChangeDependencies();
Stream stream = Bloc.of(context).foo;
subscription?.cancel();
subscription = stream.listen((value) {
// do something
});
}
@override
void dispose() {
subscription?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
You cannot use StreamBuilder
to do that. You have to manually listen to the stream
class Example extends StatefulWidget {
@override
ExampleState createState() => ExampleState();
}
class ExampleState extends State<Example> {
StreamSubscription subscription;
@override
void didChangeDependencies() {
super.didChangeDependencies();
Stream stream = Bloc.of(context).foo;
subscription?.cancel();
subscription = stream.listen((value) {
// do something
});
}
@override
void dispose() {
subscription?.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
answered Nov 23 '18 at 9:55
Rémi RousseletRémi Rousselet
36.1k484109
36.1k484109
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
add a comment |
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
Oh my. Your answer is SUPER HELPFUL. Previously I was unaware that the State<T> class has a 'context' property itself. It is only after I tried out your code and find it is actually in Flutter's source code and hidden in Flutter's docs. Thanks!
– First_Strike
Nov 23 '18 at 13:49
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@remi do you know if streams work with animation? Working with AnimatedOpacity widget the duration does not working when setting the value with a stream. Only after calling setState but that seems counter to what I have been reading. Is there a proper way to do it?
– Claude
Jan 6 at 18:23
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
@Claude They do, but be aware that listening to a stream is asynchronous. So you might see things a frame late. This is usually unnoticeable, but just keep it in mind.
– Rémi Rousselet
Jan 6 at 21:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53443827%2fflutter-bloc-pattern-how-to-use-streams-to-invoke-a-method-of-another-widget%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OiJhbPhGO8YSaWkU,QaJN7t,vEm,ZRQG L