Difference of plane/ray intersection with point/plane projection
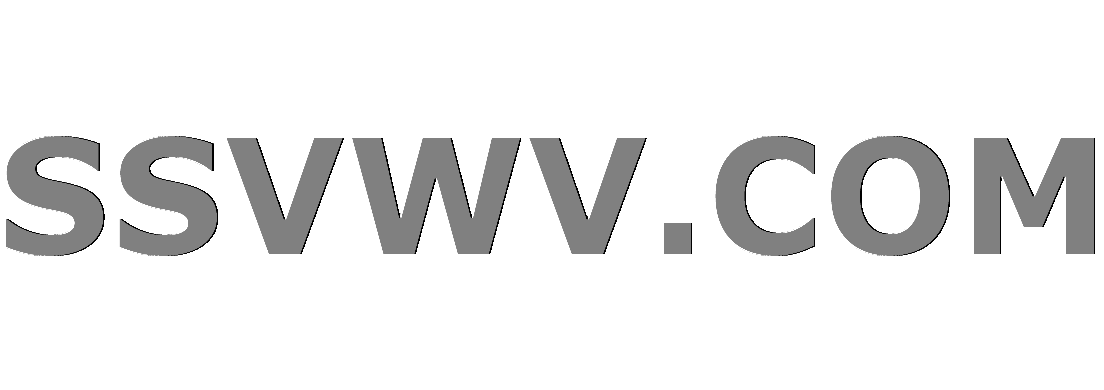
Multi tool use
up vote
0
down vote
favorite
I found a solution for the ray plane intersection code in Wikipedia, which works and where I simply solve a linear equation system.
Later I found some code for a point onto plane projection, which is obviously implemented differently and also yields different solutions under certain conditions.
However, I do not really get what is the difference between a projection of a point along a vector and the intersection of a ray (build by the point and vector). In both cases I would expect just to find the point where the ray intersects the plane?!
Is there anywhere a figure to illustrate the difference?
struct Plane {
glm::vec3 _normal;
glm::vec3 _point;
};
glm::vec3 intersection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// See: https://en.wikipedia.org/wiki/Line%E2%80%93plane_intersection
const float t = glm::dot(p._normal, point - p._point) / glm::dot(p._normal, -direction);
return point + t * direction;
}
glm::vec3 orthogonalProjection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// from https://stackoverflow.com/questions/9605556/how-to-project-a-point-onto-a-plane-in-3d
const float t = -(glm::dot(point, direction) - glm::dot(p.getOrigin(), p.getNormal()));
return point+ t * direction;
}
c++ graphics 3d
add a comment |
up vote
0
down vote
favorite
I found a solution for the ray plane intersection code in Wikipedia, which works and where I simply solve a linear equation system.
Later I found some code for a point onto plane projection, which is obviously implemented differently and also yields different solutions under certain conditions.
However, I do not really get what is the difference between a projection of a point along a vector and the intersection of a ray (build by the point and vector). In both cases I would expect just to find the point where the ray intersects the plane?!
Is there anywhere a figure to illustrate the difference?
struct Plane {
glm::vec3 _normal;
glm::vec3 _point;
};
glm::vec3 intersection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// See: https://en.wikipedia.org/wiki/Line%E2%80%93plane_intersection
const float t = glm::dot(p._normal, point - p._point) / glm::dot(p._normal, -direction);
return point + t * direction;
}
glm::vec3 orthogonalProjection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// from https://stackoverflow.com/questions/9605556/how-to-project-a-point-onto-a-plane-in-3d
const float t = -(glm::dot(point, direction) - glm::dot(p.getOrigin(), p.getNormal()));
return point+ t * direction;
}
c++ graphics 3d
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I found a solution for the ray plane intersection code in Wikipedia, which works and where I simply solve a linear equation system.
Later I found some code for a point onto plane projection, which is obviously implemented differently and also yields different solutions under certain conditions.
However, I do not really get what is the difference between a projection of a point along a vector and the intersection of a ray (build by the point and vector). In both cases I would expect just to find the point where the ray intersects the plane?!
Is there anywhere a figure to illustrate the difference?
struct Plane {
glm::vec3 _normal;
glm::vec3 _point;
};
glm::vec3 intersection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// See: https://en.wikipedia.org/wiki/Line%E2%80%93plane_intersection
const float t = glm::dot(p._normal, point - p._point) / glm::dot(p._normal, -direction);
return point + t * direction;
}
glm::vec3 orthogonalProjection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// from https://stackoverflow.com/questions/9605556/how-to-project-a-point-onto-a-plane-in-3d
const float t = -(glm::dot(point, direction) - glm::dot(p.getOrigin(), p.getNormal()));
return point+ t * direction;
}
c++ graphics 3d
I found a solution for the ray plane intersection code in Wikipedia, which works and where I simply solve a linear equation system.
Later I found some code for a point onto plane projection, which is obviously implemented differently and also yields different solutions under certain conditions.
However, I do not really get what is the difference between a projection of a point along a vector and the intersection of a ray (build by the point and vector). In both cases I would expect just to find the point where the ray intersects the plane?!
Is there anywhere a figure to illustrate the difference?
struct Plane {
glm::vec3 _normal;
glm::vec3 _point;
};
glm::vec3 intersection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// See: https://en.wikipedia.org/wiki/Line%E2%80%93plane_intersection
const float t = glm::dot(p._normal, point - p._point) / glm::dot(p._normal, -direction);
return point + t * direction;
}
glm::vec3 orthogonalProjection(const Plane &p, const glm::vec3 &point, const glm::vec3 &direction) {
// from https://stackoverflow.com/questions/9605556/how-to-project-a-point-onto-a-plane-in-3d
const float t = -(glm::dot(point, direction) - glm::dot(p.getOrigin(), p.getNormal()));
return point+ t * direction;
}
c++ graphics 3d
c++ graphics 3d
edited Nov 7 at 15:47


François Andrieux
14.8k32345
14.8k32345
asked Nov 7 at 15:33
dgrat
1,04121231
1,04121231
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
A ray is an infinite line, so it has a direction. Intersecting a ray with a plane means finding where the line passes through the plane.
A point is a dimensionless dot suspended somewhere in space. Projecting a point onto a plane means shooting a ray that passes through the point and is perpendicular to the plane (called the "normal"), and seeing where it lands.
The ray already has a direction, the point doesn't. The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined.
So you can have a special case where the ray's direction and the plane's normal coincide, in which case intersecting the ray with the plane and projecting a point that happens to lie somewhere on the ray lead to the same result, but that's just a special case.
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
A ray is an infinite line, so it has a direction. Intersecting a ray with a plane means finding where the line passes through the plane.
A point is a dimensionless dot suspended somewhere in space. Projecting a point onto a plane means shooting a ray that passes through the point and is perpendicular to the plane (called the "normal"), and seeing where it lands.
The ray already has a direction, the point doesn't. The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined.
So you can have a special case where the ray's direction and the plane's normal coincide, in which case intersecting the ray with the plane and projecting a point that happens to lie somewhere on the ray lead to the same result, but that's just a special case.
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
add a comment |
up vote
1
down vote
accepted
A ray is an infinite line, so it has a direction. Intersecting a ray with a plane means finding where the line passes through the plane.
A point is a dimensionless dot suspended somewhere in space. Projecting a point onto a plane means shooting a ray that passes through the point and is perpendicular to the plane (called the "normal"), and seeing where it lands.
The ray already has a direction, the point doesn't. The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined.
So you can have a special case where the ray's direction and the plane's normal coincide, in which case intersecting the ray with the plane and projecting a point that happens to lie somewhere on the ray lead to the same result, but that's just a special case.
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
A ray is an infinite line, so it has a direction. Intersecting a ray with a plane means finding where the line passes through the plane.
A point is a dimensionless dot suspended somewhere in space. Projecting a point onto a plane means shooting a ray that passes through the point and is perpendicular to the plane (called the "normal"), and seeing where it lands.
The ray already has a direction, the point doesn't. The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined.
So you can have a special case where the ray's direction and the plane's normal coincide, in which case intersecting the ray with the plane and projecting a point that happens to lie somewhere on the ray lead to the same result, but that's just a special case.
A ray is an infinite line, so it has a direction. Intersecting a ray with a plane means finding where the line passes through the plane.
A point is a dimensionless dot suspended somewhere in space. Projecting a point onto a plane means shooting a ray that passes through the point and is perpendicular to the plane (called the "normal"), and seeing where it lands.
The ray already has a direction, the point doesn't. The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined.
So you can have a special case where the ray's direction and the plane's normal coincide, in which case intersecting the ray with the plane and projecting a point that happens to lie somewhere on the ray lead to the same result, but that's just a special case.
answered Nov 7 at 15:44


Cyber Niki
792110
792110
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
add a comment |
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
"The direction chosen to project the point is the one perpendicular the plane simply because that's how the projection is defined." Isn't this direction of the plane's normal? Then this would equal an intersection if inserting point and plane normal.
– dgrat
Nov 7 at 16:03
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
@dgrat yes that is equivalent to intersecting the plane with a ray that is perpendicular to the plane and passes through the point. That's the special case I described at the end.
– Cyber Niki
Nov 7 at 16:05
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53192658%2fdifference-of-plane-ray-intersection-with-point-plane-projection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MVLe4 J85zXOT O2SiJ6 K,6b 1VDkwgY2SQiXMQsPRwc,DRF,s3 gSSyp,gniR