eloquent relation and multi dimensional collection reject
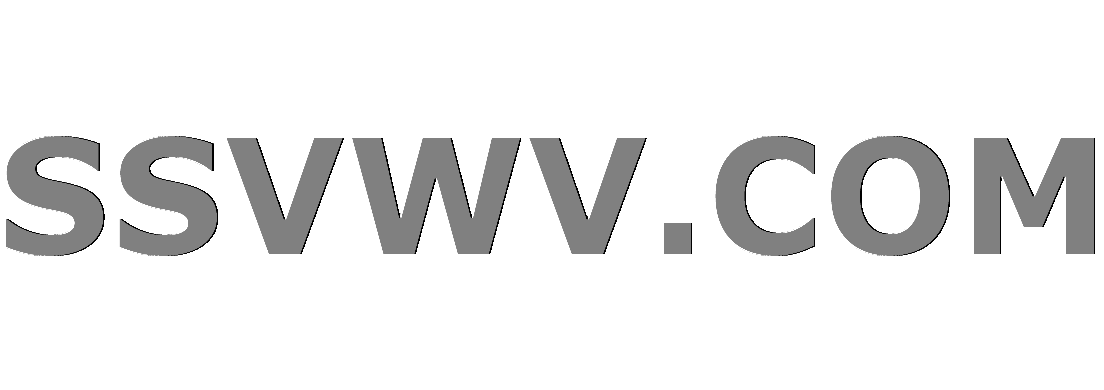
Multi tool use
up vote
0
down vote
favorite
I have a school database which hasMany teacher, and which hasMany student like this:
class School extends Model
{
public function teachers()
{
return $this->hasMany(Teacher::class,'school_id');
}
}
class Teacher extends Model
{
public function students()
{
return $this->hasMany(Student::class,'teacher_id');
}
}
class Student extends Model
{
}
The full query
$full = School::with(['teachers' => function ($query) {
$query->with('students');
}])->get();
And the result is like this:
[
{
"id": 1,
"school_name": "First Park",
"teachers": [
{
"id": 1,
"teacher_name": "Mr.Aha",
"students": [
{
"id": 1,
"student_name": "Jane",
"drop": 1
},
{
"id": 2,
"student_name": "Jon",
"drop": 0
}
]
}
]
}
]
Now I want to remove student who drop school and select teacher_name, so I try to do it with eloquent and collection, but they all fail.
The eloquent way is:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('teacher_name');
}])->get();
But the result output, teacher is an empty object.
The collection way is base on the full query
$full->map(function ($teacher) {
unset($teacher->id);//there are more column to unset in real life
$teacher->reject(function ($student) {
return $student->drop == 0;
});
});
But the result is the same as full query.
I don't know which way is better, eloquent or collection, but they won't work
php laravel eloquent
add a comment |
up vote
0
down vote
favorite
I have a school database which hasMany teacher, and which hasMany student like this:
class School extends Model
{
public function teachers()
{
return $this->hasMany(Teacher::class,'school_id');
}
}
class Teacher extends Model
{
public function students()
{
return $this->hasMany(Student::class,'teacher_id');
}
}
class Student extends Model
{
}
The full query
$full = School::with(['teachers' => function ($query) {
$query->with('students');
}])->get();
And the result is like this:
[
{
"id": 1,
"school_name": "First Park",
"teachers": [
{
"id": 1,
"teacher_name": "Mr.Aha",
"students": [
{
"id": 1,
"student_name": "Jane",
"drop": 1
},
{
"id": 2,
"student_name": "Jon",
"drop": 0
}
]
}
]
}
]
Now I want to remove student who drop school and select teacher_name, so I try to do it with eloquent and collection, but they all fail.
The eloquent way is:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('teacher_name');
}])->get();
But the result output, teacher is an empty object.
The collection way is base on the full query
$full->map(function ($teacher) {
unset($teacher->id);//there are more column to unset in real life
$teacher->reject(function ($student) {
return $student->drop == 0;
});
});
But the result is the same as full query.
I don't know which way is better, eloquent or collection, but they won't work
php laravel eloquent
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a school database which hasMany teacher, and which hasMany student like this:
class School extends Model
{
public function teachers()
{
return $this->hasMany(Teacher::class,'school_id');
}
}
class Teacher extends Model
{
public function students()
{
return $this->hasMany(Student::class,'teacher_id');
}
}
class Student extends Model
{
}
The full query
$full = School::with(['teachers' => function ($query) {
$query->with('students');
}])->get();
And the result is like this:
[
{
"id": 1,
"school_name": "First Park",
"teachers": [
{
"id": 1,
"teacher_name": "Mr.Aha",
"students": [
{
"id": 1,
"student_name": "Jane",
"drop": 1
},
{
"id": 2,
"student_name": "Jon",
"drop": 0
}
]
}
]
}
]
Now I want to remove student who drop school and select teacher_name, so I try to do it with eloquent and collection, but they all fail.
The eloquent way is:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('teacher_name');
}])->get();
But the result output, teacher is an empty object.
The collection way is base on the full query
$full->map(function ($teacher) {
unset($teacher->id);//there are more column to unset in real life
$teacher->reject(function ($student) {
return $student->drop == 0;
});
});
But the result is the same as full query.
I don't know which way is better, eloquent or collection, but they won't work
php laravel eloquent
I have a school database which hasMany teacher, and which hasMany student like this:
class School extends Model
{
public function teachers()
{
return $this->hasMany(Teacher::class,'school_id');
}
}
class Teacher extends Model
{
public function students()
{
return $this->hasMany(Student::class,'teacher_id');
}
}
class Student extends Model
{
}
The full query
$full = School::with(['teachers' => function ($query) {
$query->with('students');
}])->get();
And the result is like this:
[
{
"id": 1,
"school_name": "First Park",
"teachers": [
{
"id": 1,
"teacher_name": "Mr.Aha",
"students": [
{
"id": 1,
"student_name": "Jane",
"drop": 1
},
{
"id": 2,
"student_name": "Jon",
"drop": 0
}
]
}
]
}
]
Now I want to remove student who drop school and select teacher_name, so I try to do it with eloquent and collection, but they all fail.
The eloquent way is:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('teacher_name');
}])->get();
But the result output, teacher is an empty object.
The collection way is base on the full query
$full->map(function ($teacher) {
unset($teacher->id);//there are more column to unset in real life
$teacher->reject(function ($student) {
return $student->drop == 0;
});
});
But the result is the same as full query.
I don't know which way is better, eloquent or collection, but they won't work
php laravel eloquent
php laravel eloquent
asked Nov 7 at 9:07


Autodesk
111111
111111
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Example from original docs.
Nested Eager Loading
To eager load nested relationships, you may use "dot" syntax. For example, let's eager load all of the book's authors and all of the author's personal contacts in one Eloquent statement:
$books = AppBook::with('author.contacts')->get();
also, you can use load() method
add a comment |
up vote
0
down vote
You also have to select the id
and school_id
columns that are required for eager loading:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('id', 'school_id', 'teacher_name');
}])->get();
Then remove them afterwards or use $hidden:
$full->map(function ($teacher) {
unset($teacher->id, $teacher->school_id);
});
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Example from original docs.
Nested Eager Loading
To eager load nested relationships, you may use "dot" syntax. For example, let's eager load all of the book's authors and all of the author's personal contacts in one Eloquent statement:
$books = AppBook::with('author.contacts')->get();
also, you can use load() method
add a comment |
up vote
0
down vote
Example from original docs.
Nested Eager Loading
To eager load nested relationships, you may use "dot" syntax. For example, let's eager load all of the book's authors and all of the author's personal contacts in one Eloquent statement:
$books = AppBook::with('author.contacts')->get();
also, you can use load() method
add a comment |
up vote
0
down vote
up vote
0
down vote
Example from original docs.
Nested Eager Loading
To eager load nested relationships, you may use "dot" syntax. For example, let's eager load all of the book's authors and all of the author's personal contacts in one Eloquent statement:
$books = AppBook::with('author.contacts')->get();
also, you can use load() method
Example from original docs.
Nested Eager Loading
To eager load nested relationships, you may use "dot" syntax. For example, let's eager load all of the book's authors and all of the author's personal contacts in one Eloquent statement:
$books = AppBook::with('author.contacts')->get();
also, you can use load() method
answered Nov 7 at 10:13


Jovix
12
12
add a comment |
add a comment |
up vote
0
down vote
You also have to select the id
and school_id
columns that are required for eager loading:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('id', 'school_id', 'teacher_name');
}])->get();
Then remove them afterwards or use $hidden:
$full->map(function ($teacher) {
unset($teacher->id, $teacher->school_id);
});
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
add a comment |
up vote
0
down vote
You also have to select the id
and school_id
columns that are required for eager loading:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('id', 'school_id', 'teacher_name');
}])->get();
Then remove them afterwards or use $hidden:
$full->map(function ($teacher) {
unset($teacher->id, $teacher->school_id);
});
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
add a comment |
up vote
0
down vote
up vote
0
down vote
You also have to select the id
and school_id
columns that are required for eager loading:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('id', 'school_id', 'teacher_name');
}])->get();
Then remove them afterwards or use $hidden:
$full->map(function ($teacher) {
unset($teacher->id, $teacher->school_id);
});
You also have to select the id
and school_id
columns that are required for eager loading:
School::with(['teachers' => function ($teacher) {
$teacher->with(['students' => function ($student) {
$student->where('drop', '!=', 0)
}])->select('id', 'school_id', 'teacher_name');
}])->get();
Then remove them afterwards or use $hidden:
$full->map(function ($teacher) {
unset($teacher->id, $teacher->school_id);
});
answered Nov 7 at 21:43
Jonas Staudenmeir
11.4k2932
11.4k2932
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
add a comment |
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
Does it work for you?
– Jonas Staudenmeir
Nov 11 at 3:54
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53186337%2feloquent-relation-and-multi-dimensional-collection-reject%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BZgGaA2cNWn,us1fQqsQcmgnk9,ZbgW8cVXvGQuwRHcAYlfgiFO,yI 1He12aZ3MGWzXhHFIeDz bd3W56wiUSjV9d8YWtMpfLuzu