Override “operators” and println method in Scala
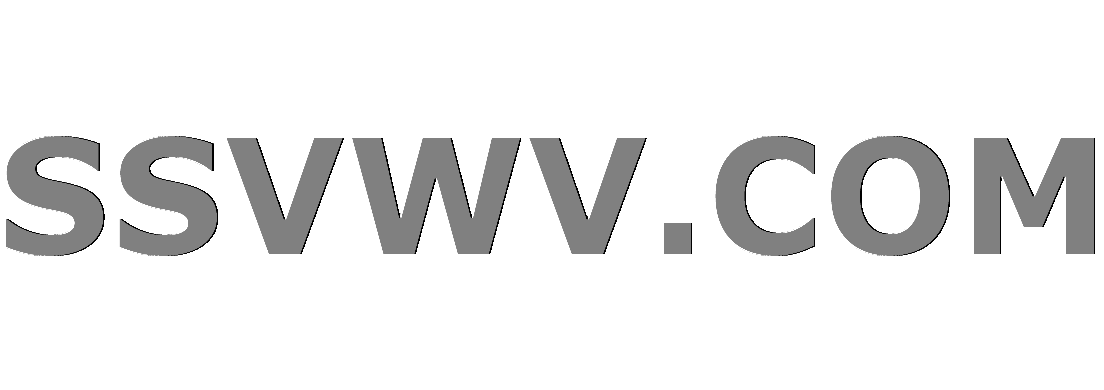
Multi tool use
up vote
0
down vote
favorite
I need to create methods for basic operations for different types so the output of the expression: println(1 + 2*I + I*3 + 2)
is 3+5i
. I am new to Scala and here is what I have so far:
class IClass() {
var value = 0
def *(number: Int): String = {
//value += number
value + "i"
}
}
object ComplexNumbers {
var TotalValue: Int = 0
var TotalString: String = ""
// ...
def Complex(num1: Int, num2: Int): String ={
num1 + "+" + num2 + "i"
}
implicit class IntMultiply(private val a: Int) extends AnyVal {
def + (b: String)= {
if(b.contains("i")){
TotalValue += a
TotalString.concat(b)
}
}
def * (b: IClass) = {
//b.value += a
a + "i"
}
}
implicit class StringAdd(private val a: String) extends AnyVal {
def + (b: String): String = {
if(b.contains("i")){
}
a + "i"
}
}
def main(args: Array[String]) {
println(Complex(1,2)) // 1+2i
val I = new IClass()
println(1 + 2*I + I*3 + 2) // 3+5i
// val c = (2+3*I + 1 + 4*I) * I
// println(-c) // 7-3i
}
}
I think I am going in a wrong direction with this because by implementing these operation methods on types I get an error in the println: Type Mismach
because of the Any
return type where I only update fields without returning anything. Any idea how to implement this?
scala operators
add a comment |
up vote
0
down vote
favorite
I need to create methods for basic operations for different types so the output of the expression: println(1 + 2*I + I*3 + 2)
is 3+5i
. I am new to Scala and here is what I have so far:
class IClass() {
var value = 0
def *(number: Int): String = {
//value += number
value + "i"
}
}
object ComplexNumbers {
var TotalValue: Int = 0
var TotalString: String = ""
// ...
def Complex(num1: Int, num2: Int): String ={
num1 + "+" + num2 + "i"
}
implicit class IntMultiply(private val a: Int) extends AnyVal {
def + (b: String)= {
if(b.contains("i")){
TotalValue += a
TotalString.concat(b)
}
}
def * (b: IClass) = {
//b.value += a
a + "i"
}
}
implicit class StringAdd(private val a: String) extends AnyVal {
def + (b: String): String = {
if(b.contains("i")){
}
a + "i"
}
}
def main(args: Array[String]) {
println(Complex(1,2)) // 1+2i
val I = new IClass()
println(1 + 2*I + I*3 + 2) // 3+5i
// val c = (2+3*I + 1 + 4*I) * I
// println(-c) // 7-3i
}
}
I think I am going in a wrong direction with this because by implementing these operation methods on types I get an error in the println: Type Mismach
because of the Any
return type where I only update fields without returning anything. Any idea how to implement this?
scala operators
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I need to create methods for basic operations for different types so the output of the expression: println(1 + 2*I + I*3 + 2)
is 3+5i
. I am new to Scala and here is what I have so far:
class IClass() {
var value = 0
def *(number: Int): String = {
//value += number
value + "i"
}
}
object ComplexNumbers {
var TotalValue: Int = 0
var TotalString: String = ""
// ...
def Complex(num1: Int, num2: Int): String ={
num1 + "+" + num2 + "i"
}
implicit class IntMultiply(private val a: Int) extends AnyVal {
def + (b: String)= {
if(b.contains("i")){
TotalValue += a
TotalString.concat(b)
}
}
def * (b: IClass) = {
//b.value += a
a + "i"
}
}
implicit class StringAdd(private val a: String) extends AnyVal {
def + (b: String): String = {
if(b.contains("i")){
}
a + "i"
}
}
def main(args: Array[String]) {
println(Complex(1,2)) // 1+2i
val I = new IClass()
println(1 + 2*I + I*3 + 2) // 3+5i
// val c = (2+3*I + 1 + 4*I) * I
// println(-c) // 7-3i
}
}
I think I am going in a wrong direction with this because by implementing these operation methods on types I get an error in the println: Type Mismach
because of the Any
return type where I only update fields without returning anything. Any idea how to implement this?
scala operators
I need to create methods for basic operations for different types so the output of the expression: println(1 + 2*I + I*3 + 2)
is 3+5i
. I am new to Scala and here is what I have so far:
class IClass() {
var value = 0
def *(number: Int): String = {
//value += number
value + "i"
}
}
object ComplexNumbers {
var TotalValue: Int = 0
var TotalString: String = ""
// ...
def Complex(num1: Int, num2: Int): String ={
num1 + "+" + num2 + "i"
}
implicit class IntMultiply(private val a: Int) extends AnyVal {
def + (b: String)= {
if(b.contains("i")){
TotalValue += a
TotalString.concat(b)
}
}
def * (b: IClass) = {
//b.value += a
a + "i"
}
}
implicit class StringAdd(private val a: String) extends AnyVal {
def + (b: String): String = {
if(b.contains("i")){
}
a + "i"
}
}
def main(args: Array[String]) {
println(Complex(1,2)) // 1+2i
val I = new IClass()
println(1 + 2*I + I*3 + 2) // 3+5i
// val c = (2+3*I + 1 + 4*I) * I
// println(-c) // 7-3i
}
}
I think I am going in a wrong direction with this because by implementing these operation methods on types I get an error in the println: Type Mismach
because of the Any
return type where I only update fields without returning anything. Any idea how to implement this?
scala operators
scala operators
asked Nov 4 at 10:02


Harun Cerim
7071619
7071619
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
You should think of the complex numbers as a class with certain behaviors, and define it first, rather than focusing on the one concrete side effect you are after at the moment. It seems counter intuitive, but implementing a more abstract/general problem often makes the job easier than trying to narrow it down to just the task at hand.
case class ComplexInt(real: Int, im: Int) {
def + (other: ComplexInt) = ComplexInt(real + other.real, im + other.im)
def * (other: ComplexInt) = ComplexInt(
real * other.real - im * other.im,
real * other.im + im * other.real
)
def unary_- = ComplexInt(-real, -im)
def -(other: ComplexInt) = this + -other
override def toString() = (if(real == 0 && im != 0) "" else real.toString) + (im match {
case 0 => ""
case 1 if real == 0 => "i"
case 1 => " + i"
case n if n < 0 || real == 0 => s"${n}i"
case n => s"+${n}i"
})
}
object ComplexInt {
val I = ComplexInt(0, 1)
implicit def fromInt(n: Int) = ComplexInt(n, 0)
}
Now, you just need to import ComplexInt.I
,
and then things like println(1 + 2*I + I*3 + 2)
will print 3+5i
etc.
You can even do (1 + 2*I)*(2 + 3*I)
(evaluates to -4+7i
).
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
1
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
You should think of the complex numbers as a class with certain behaviors, and define it first, rather than focusing on the one concrete side effect you are after at the moment. It seems counter intuitive, but implementing a more abstract/general problem often makes the job easier than trying to narrow it down to just the task at hand.
case class ComplexInt(real: Int, im: Int) {
def + (other: ComplexInt) = ComplexInt(real + other.real, im + other.im)
def * (other: ComplexInt) = ComplexInt(
real * other.real - im * other.im,
real * other.im + im * other.real
)
def unary_- = ComplexInt(-real, -im)
def -(other: ComplexInt) = this + -other
override def toString() = (if(real == 0 && im != 0) "" else real.toString) + (im match {
case 0 => ""
case 1 if real == 0 => "i"
case 1 => " + i"
case n if n < 0 || real == 0 => s"${n}i"
case n => s"+${n}i"
})
}
object ComplexInt {
val I = ComplexInt(0, 1)
implicit def fromInt(n: Int) = ComplexInt(n, 0)
}
Now, you just need to import ComplexInt.I
,
and then things like println(1 + 2*I + I*3 + 2)
will print 3+5i
etc.
You can even do (1 + 2*I)*(2 + 3*I)
(evaluates to -4+7i
).
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
1
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
add a comment |
up vote
3
down vote
accepted
You should think of the complex numbers as a class with certain behaviors, and define it first, rather than focusing on the one concrete side effect you are after at the moment. It seems counter intuitive, but implementing a more abstract/general problem often makes the job easier than trying to narrow it down to just the task at hand.
case class ComplexInt(real: Int, im: Int) {
def + (other: ComplexInt) = ComplexInt(real + other.real, im + other.im)
def * (other: ComplexInt) = ComplexInt(
real * other.real - im * other.im,
real * other.im + im * other.real
)
def unary_- = ComplexInt(-real, -im)
def -(other: ComplexInt) = this + -other
override def toString() = (if(real == 0 && im != 0) "" else real.toString) + (im match {
case 0 => ""
case 1 if real == 0 => "i"
case 1 => " + i"
case n if n < 0 || real == 0 => s"${n}i"
case n => s"+${n}i"
})
}
object ComplexInt {
val I = ComplexInt(0, 1)
implicit def fromInt(n: Int) = ComplexInt(n, 0)
}
Now, you just need to import ComplexInt.I
,
and then things like println(1 + 2*I + I*3 + 2)
will print 3+5i
etc.
You can even do (1 + 2*I)*(2 + 3*I)
(evaluates to -4+7i
).
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
1
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
You should think of the complex numbers as a class with certain behaviors, and define it first, rather than focusing on the one concrete side effect you are after at the moment. It seems counter intuitive, but implementing a more abstract/general problem often makes the job easier than trying to narrow it down to just the task at hand.
case class ComplexInt(real: Int, im: Int) {
def + (other: ComplexInt) = ComplexInt(real + other.real, im + other.im)
def * (other: ComplexInt) = ComplexInt(
real * other.real - im * other.im,
real * other.im + im * other.real
)
def unary_- = ComplexInt(-real, -im)
def -(other: ComplexInt) = this + -other
override def toString() = (if(real == 0 && im != 0) "" else real.toString) + (im match {
case 0 => ""
case 1 if real == 0 => "i"
case 1 => " + i"
case n if n < 0 || real == 0 => s"${n}i"
case n => s"+${n}i"
})
}
object ComplexInt {
val I = ComplexInt(0, 1)
implicit def fromInt(n: Int) = ComplexInt(n, 0)
}
Now, you just need to import ComplexInt.I
,
and then things like println(1 + 2*I + I*3 + 2)
will print 3+5i
etc.
You can even do (1 + 2*I)*(2 + 3*I)
(evaluates to -4+7i
).
You should think of the complex numbers as a class with certain behaviors, and define it first, rather than focusing on the one concrete side effect you are after at the moment. It seems counter intuitive, but implementing a more abstract/general problem often makes the job easier than trying to narrow it down to just the task at hand.
case class ComplexInt(real: Int, im: Int) {
def + (other: ComplexInt) = ComplexInt(real + other.real, im + other.im)
def * (other: ComplexInt) = ComplexInt(
real * other.real - im * other.im,
real * other.im + im * other.real
)
def unary_- = ComplexInt(-real, -im)
def -(other: ComplexInt) = this + -other
override def toString() = (if(real == 0 && im != 0) "" else real.toString) + (im match {
case 0 => ""
case 1 if real == 0 => "i"
case 1 => " + i"
case n if n < 0 || real == 0 => s"${n}i"
case n => s"+${n}i"
})
}
object ComplexInt {
val I = ComplexInt(0, 1)
implicit def fromInt(n: Int) = ComplexInt(n, 0)
}
Now, you just need to import ComplexInt.I
,
and then things like println(1 + 2*I + I*3 + 2)
will print 3+5i
etc.
You can even do (1 + 2*I)*(2 + 3*I)
(evaluates to -4+7i
).
edited 2 days ago
answered 2 days ago
Dima
22.9k32134
22.9k32134
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
1
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
add a comment |
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
1
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
As I said, I just started learning Scala and this was my first assignment. I knew I shouldn't narrow the solution to only one scenario but I thought it would be somehow easier to get the answer. This is actually pretty amazing what you did. Thank you very much.
– Harun Cerim
2 days ago
1
1
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
pretty gnarly stuff for a first assignment
– Seth Tisue
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53139608%2foverride-operators-and-println-method-in-scala%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
qgLt0hKIWPbli4SHsXMe JTNJSLxt6YMtvU c3KXeZJbHiqJQu9Xxqxnf