Permission Denial: showing images in recyclerview after phone restart android
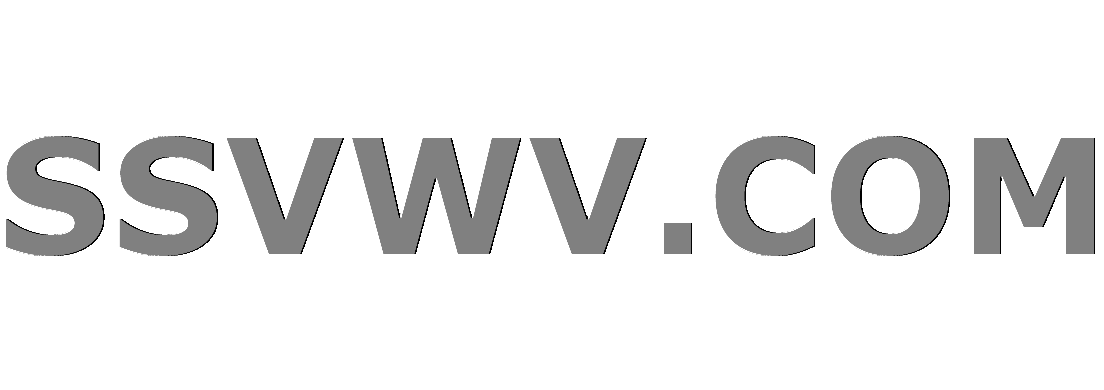
Multi tool use
up vote
0
down vote
favorite
storing images uri in sqlite database and showing them in recyclerview it works fine until the phone is restarted.
after restart the app crashes when ShowProductsAdapter is called with error.
"java.lang.SecurityException: Permission Denial: opening provider com.android.providers.media.MediaDocumentsProvider from ProcessRecord{91fdba7 5931:com.example.waqar.aatachaki/u0a240} (pid=5931, uid=10240) requires android.permission.MANAGE_DOCUMENTS or android.permission.MANAGE_DOCUMENTS"
here is my addproduct activity class
public class AddProduct extends AppCompatActivity {
EditText product_title,sale_price,price_shortcut,weight_shortcut;
Button openGallery ;
DBManager dbManager;
String productTitle ="";
String salePrice ="";
ImageView product_image;
String priceshortcut="";
String weightshortcut="";
private Bitmap currentImage;
Uri selectedImage;
Intent photoPickerIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_product);
product_title=(EditText)findViewById(R.id.p_title);
sale_price=(EditText)findViewById(R.id.p_salePrice);
price_shortcut =(EditText)findViewById(R.id.p_shortcut);
weight_shortcut=(EditText)findViewById(R.id.w_shortcut);
product_image=(ImageView) findViewById(R.id.product_image);
dbManager=new DBManager(this,null,null,5);
openGallery = (Button) findViewById(R.id.gallery);
openGallery.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
photoPickerIntent = new Intent(Intent.ACTION_OPEN_DOCUMENT );
photoPickerIntent.addCategory(Intent.CATEGORY_OPENABLE);
photoPickerIntent.setType("image/*");
startActivityForResult(photoPickerIntent, 1);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
selectedImage = data.getData();
try {
Bitmap bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), selectedImage);
product_image.setImageBitmap(bitmap);
// to access the file permission
int takeFlags = photoPickerIntent.getFlags();
takeFlags &=( Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
this.getContentResolver().takePersistableUriPermission(selectedImage, takeFlags);
this.grantUriPermission("com.example.waqar.aatachaki", selectedImage, Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void addproduct(View view) {
productTitle = product_title.getText().toString();
salePrice= sale_price.getText().toString();
priceshortcut= price_shortcut.getText().toString();
weightshortcut= weight_shortcut.getText().toString();
if (productTitle.isEmpty()) {
Toast.makeText(this, "You did not enter a Product Title", Toast.LENGTH_LONG).show();
return;
}
if (salePrice.isEmpty()) {
Toast.makeText(this, "You did not enter a Sale Price", Toast.LENGTH_LONG).show();
return;
}
if (product_image==null) {
Toast.makeText(this, "Please Select a Picture", Toast.LENGTH_LONG).show();
return;
}
ContentValues values=new ContentValues();
values.put("title",productTitle);
values.put("sale_price",salePrice);
values.put("price_shortcut", priceshortcut);
values.put("weight_shortcut", weightshortcut);
values.put("IMAGE", String.valueOf(selectedImage));
long check = dbManager.Insert(values);
if (check == 0) {
Toast.makeText(this,"record not inserted is = "+check+"",Toast.LENGTH_LONG).show();
finish();
} else {
Toast.makeText(this, "record inserted ID is = " + check + "", Toast.LENGTH_LONG).show();
finish();
}
}
public void goback(View view) {
Intent intent = new Intent(this,MainActivity.class);
startActivity(intent);
finish();
}
public void onStop() {
super.onStop();
if (currentImage != null) {
currentImage.recycle();
currentImage = null;
System.gc();
}
}
}
and the showproductadapter is..
public class showProductsAdapter extends RecyclerView.Adapter {
Context context;
java.util.List<showProducts> List;
public showProductsAdapter(Context context, List<showProducts> list) {
this.context = context;
this.List = list;
}
@NonNull
@Override
public showProductsAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view= LayoutInflater.from(parent.getContext()).inflate(R.layout.list_row,parent,false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull showProductsAdapter.ViewHolder holder, int position) {
showProducts item=List.get(position);
Uri myUri = Uri.parse(item.getImageData());
Bitmap bitmap = null;
try {
bitmap = getBitmapFromUri(myUri);
//bitmap = MediaStore.Images.Media.getBitmap(context.getContentResolver(), myUri);
} catch (IOException e) {
e.printStackTrace();
}
holder.image.setImageBitmap(bitmap);
holder.productName.setText(item.getProductName());
holder.price.setText(String.format("%s/- KG", item.getSalePrice()));
}
@Override
public int getItemCount()
{
return List.size();
}
private Bitmap getBitmapFromUri(Uri uri) throws IOException {
ParcelFileDescriptor parcelFileDescriptor =
context.getContentResolver().openFileDescriptor(uri, "r");
FileDescriptor fileDescriptor = parcelFileDescriptor.getFileDescriptor();
Bitmap image = BitmapFactory.decodeFileDescriptor(fileDescriptor);
parcelFileDescriptor.close();
return image;
}
public class ViewHolder extends RecyclerView.ViewHolder implements View.OnClickListener {
public TextView productName;
public TextView price;
public ImageView image;
public ViewHolder(View view) {
super(view);
view.setOnClickListener(this);
productName = view.findViewById(R.id.productName);
price = view.findViewById(R.id.productPrice);
image = view.findViewById(R.id.profile_image);
}
@Override
public void onClick(View v) {
int position = getAdapterPosition();
showProducts item=List.get(position);
Toast.makeText(context,"Name is "+item.getProductName(),Toast.LENGTH_LONG).show();
Intent intent = new Intent(context, UpdateProduct.class);
intent.putExtra("name", item.getProductName());
intent.putExtra("_id", item.get_id());
intent.putExtra("saleprice", item.getSalePrice());
intent.putExtra("weightshortcut", item.getWeightShortcut());
intent.putExtra("priceshortcut", item.getPriceShortcut());
intent.putExtra("image", item.getImageData());
context.startActivity(intent);
}
}
}
recyclerview activity where the inserted products are shown..
public class Products extends AppCompatActivity implements View.OnClickListener {
private RecyclerView recyclerView;
private RecyclerView.Adapter adapter;
private List<showProducts> List;
DBManager dbManager;
Button add;
Button refresh;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_products);
add=findViewById(R.id.add);
refresh=findViewById(R.id.refresh);
add.setOnClickListener(this);
refresh.setOnClickListener(this);
recyclerView=findViewById(R.id.productslist);
recyclerView.setHasFixedSize(true);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
dbManager=new DBManager(this,null,null,1);
SQLiteDatabase sqLiteDatabase=dbManager.getReadableDatabase();
Cursor cursor=dbManager.getAllProducts();
List =new ArrayList<>();
cursor.moveToFirst();
if (cursor != null && cursor.moveToFirst()) {
do {
showProducts showProducts=new showProducts(cursor.getString(0),cursor.getString(1),cursor.getString(2),cursor.getString(3),cursor.getString(4),cursor.getString(5));
List.add(showProducts);
}
while (cursor.moveToNext());
adapter= new showProductsAdapter(this,List);
recyclerView.setAdapter(adapter);
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.add:{
Intent intent = new Intent(this,AddProduct.class);
startActivity(intent);
finish();
}
break;
case R.id.refresh:{
Intent intent = new Intent(this,Products.class);
startActivity(intent);
finish();
//adapter.notifyDataSetChanged();
}
break;
}
}
}

add a comment |
up vote
0
down vote
favorite
storing images uri in sqlite database and showing them in recyclerview it works fine until the phone is restarted.
after restart the app crashes when ShowProductsAdapter is called with error.
"java.lang.SecurityException: Permission Denial: opening provider com.android.providers.media.MediaDocumentsProvider from ProcessRecord{91fdba7 5931:com.example.waqar.aatachaki/u0a240} (pid=5931, uid=10240) requires android.permission.MANAGE_DOCUMENTS or android.permission.MANAGE_DOCUMENTS"
here is my addproduct activity class
public class AddProduct extends AppCompatActivity {
EditText product_title,sale_price,price_shortcut,weight_shortcut;
Button openGallery ;
DBManager dbManager;
String productTitle ="";
String salePrice ="";
ImageView product_image;
String priceshortcut="";
String weightshortcut="";
private Bitmap currentImage;
Uri selectedImage;
Intent photoPickerIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_product);
product_title=(EditText)findViewById(R.id.p_title);
sale_price=(EditText)findViewById(R.id.p_salePrice);
price_shortcut =(EditText)findViewById(R.id.p_shortcut);
weight_shortcut=(EditText)findViewById(R.id.w_shortcut);
product_image=(ImageView) findViewById(R.id.product_image);
dbManager=new DBManager(this,null,null,5);
openGallery = (Button) findViewById(R.id.gallery);
openGallery.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
photoPickerIntent = new Intent(Intent.ACTION_OPEN_DOCUMENT );
photoPickerIntent.addCategory(Intent.CATEGORY_OPENABLE);
photoPickerIntent.setType("image/*");
startActivityForResult(photoPickerIntent, 1);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
selectedImage = data.getData();
try {
Bitmap bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), selectedImage);
product_image.setImageBitmap(bitmap);
// to access the file permission
int takeFlags = photoPickerIntent.getFlags();
takeFlags &=( Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
this.getContentResolver().takePersistableUriPermission(selectedImage, takeFlags);
this.grantUriPermission("com.example.waqar.aatachaki", selectedImage, Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void addproduct(View view) {
productTitle = product_title.getText().toString();
salePrice= sale_price.getText().toString();
priceshortcut= price_shortcut.getText().toString();
weightshortcut= weight_shortcut.getText().toString();
if (productTitle.isEmpty()) {
Toast.makeText(this, "You did not enter a Product Title", Toast.LENGTH_LONG).show();
return;
}
if (salePrice.isEmpty()) {
Toast.makeText(this, "You did not enter a Sale Price", Toast.LENGTH_LONG).show();
return;
}
if (product_image==null) {
Toast.makeText(this, "Please Select a Picture", Toast.LENGTH_LONG).show();
return;
}
ContentValues values=new ContentValues();
values.put("title",productTitle);
values.put("sale_price",salePrice);
values.put("price_shortcut", priceshortcut);
values.put("weight_shortcut", weightshortcut);
values.put("IMAGE", String.valueOf(selectedImage));
long check = dbManager.Insert(values);
if (check == 0) {
Toast.makeText(this,"record not inserted is = "+check+"",Toast.LENGTH_LONG).show();
finish();
} else {
Toast.makeText(this, "record inserted ID is = " + check + "", Toast.LENGTH_LONG).show();
finish();
}
}
public void goback(View view) {
Intent intent = new Intent(this,MainActivity.class);
startActivity(intent);
finish();
}
public void onStop() {
super.onStop();
if (currentImage != null) {
currentImage.recycle();
currentImage = null;
System.gc();
}
}
}
and the showproductadapter is..
public class showProductsAdapter extends RecyclerView.Adapter {
Context context;
java.util.List<showProducts> List;
public showProductsAdapter(Context context, List<showProducts> list) {
this.context = context;
this.List = list;
}
@NonNull
@Override
public showProductsAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view= LayoutInflater.from(parent.getContext()).inflate(R.layout.list_row,parent,false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull showProductsAdapter.ViewHolder holder, int position) {
showProducts item=List.get(position);
Uri myUri = Uri.parse(item.getImageData());
Bitmap bitmap = null;
try {
bitmap = getBitmapFromUri(myUri);
//bitmap = MediaStore.Images.Media.getBitmap(context.getContentResolver(), myUri);
} catch (IOException e) {
e.printStackTrace();
}
holder.image.setImageBitmap(bitmap);
holder.productName.setText(item.getProductName());
holder.price.setText(String.format("%s/- KG", item.getSalePrice()));
}
@Override
public int getItemCount()
{
return List.size();
}
private Bitmap getBitmapFromUri(Uri uri) throws IOException {
ParcelFileDescriptor parcelFileDescriptor =
context.getContentResolver().openFileDescriptor(uri, "r");
FileDescriptor fileDescriptor = parcelFileDescriptor.getFileDescriptor();
Bitmap image = BitmapFactory.decodeFileDescriptor(fileDescriptor);
parcelFileDescriptor.close();
return image;
}
public class ViewHolder extends RecyclerView.ViewHolder implements View.OnClickListener {
public TextView productName;
public TextView price;
public ImageView image;
public ViewHolder(View view) {
super(view);
view.setOnClickListener(this);
productName = view.findViewById(R.id.productName);
price = view.findViewById(R.id.productPrice);
image = view.findViewById(R.id.profile_image);
}
@Override
public void onClick(View v) {
int position = getAdapterPosition();
showProducts item=List.get(position);
Toast.makeText(context,"Name is "+item.getProductName(),Toast.LENGTH_LONG).show();
Intent intent = new Intent(context, UpdateProduct.class);
intent.putExtra("name", item.getProductName());
intent.putExtra("_id", item.get_id());
intent.putExtra("saleprice", item.getSalePrice());
intent.putExtra("weightshortcut", item.getWeightShortcut());
intent.putExtra("priceshortcut", item.getPriceShortcut());
intent.putExtra("image", item.getImageData());
context.startActivity(intent);
}
}
}
recyclerview activity where the inserted products are shown..
public class Products extends AppCompatActivity implements View.OnClickListener {
private RecyclerView recyclerView;
private RecyclerView.Adapter adapter;
private List<showProducts> List;
DBManager dbManager;
Button add;
Button refresh;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_products);
add=findViewById(R.id.add);
refresh=findViewById(R.id.refresh);
add.setOnClickListener(this);
refresh.setOnClickListener(this);
recyclerView=findViewById(R.id.productslist);
recyclerView.setHasFixedSize(true);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
dbManager=new DBManager(this,null,null,1);
SQLiteDatabase sqLiteDatabase=dbManager.getReadableDatabase();
Cursor cursor=dbManager.getAllProducts();
List =new ArrayList<>();
cursor.moveToFirst();
if (cursor != null && cursor.moveToFirst()) {
do {
showProducts showProducts=new showProducts(cursor.getString(0),cursor.getString(1),cursor.getString(2),cursor.getString(3),cursor.getString(4),cursor.getString(5));
List.add(showProducts);
}
while (cursor.moveToNext());
adapter= new showProductsAdapter(this,List);
recyclerView.setAdapter(adapter);
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.add:{
Intent intent = new Intent(this,AddProduct.class);
startActivity(intent);
finish();
}
break;
case R.id.refresh:{
Intent intent = new Intent(this,Products.class);
startActivity(intent);
finish();
//adapter.notifyDataSetChanged();
}
break;
}
}
}

add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
storing images uri in sqlite database and showing them in recyclerview it works fine until the phone is restarted.
after restart the app crashes when ShowProductsAdapter is called with error.
"java.lang.SecurityException: Permission Denial: opening provider com.android.providers.media.MediaDocumentsProvider from ProcessRecord{91fdba7 5931:com.example.waqar.aatachaki/u0a240} (pid=5931, uid=10240) requires android.permission.MANAGE_DOCUMENTS or android.permission.MANAGE_DOCUMENTS"
here is my addproduct activity class
public class AddProduct extends AppCompatActivity {
EditText product_title,sale_price,price_shortcut,weight_shortcut;
Button openGallery ;
DBManager dbManager;
String productTitle ="";
String salePrice ="";
ImageView product_image;
String priceshortcut="";
String weightshortcut="";
private Bitmap currentImage;
Uri selectedImage;
Intent photoPickerIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_product);
product_title=(EditText)findViewById(R.id.p_title);
sale_price=(EditText)findViewById(R.id.p_salePrice);
price_shortcut =(EditText)findViewById(R.id.p_shortcut);
weight_shortcut=(EditText)findViewById(R.id.w_shortcut);
product_image=(ImageView) findViewById(R.id.product_image);
dbManager=new DBManager(this,null,null,5);
openGallery = (Button) findViewById(R.id.gallery);
openGallery.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
photoPickerIntent = new Intent(Intent.ACTION_OPEN_DOCUMENT );
photoPickerIntent.addCategory(Intent.CATEGORY_OPENABLE);
photoPickerIntent.setType("image/*");
startActivityForResult(photoPickerIntent, 1);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
selectedImage = data.getData();
try {
Bitmap bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), selectedImage);
product_image.setImageBitmap(bitmap);
// to access the file permission
int takeFlags = photoPickerIntent.getFlags();
takeFlags &=( Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
this.getContentResolver().takePersistableUriPermission(selectedImage, takeFlags);
this.grantUriPermission("com.example.waqar.aatachaki", selectedImage, Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void addproduct(View view) {
productTitle = product_title.getText().toString();
salePrice= sale_price.getText().toString();
priceshortcut= price_shortcut.getText().toString();
weightshortcut= weight_shortcut.getText().toString();
if (productTitle.isEmpty()) {
Toast.makeText(this, "You did not enter a Product Title", Toast.LENGTH_LONG).show();
return;
}
if (salePrice.isEmpty()) {
Toast.makeText(this, "You did not enter a Sale Price", Toast.LENGTH_LONG).show();
return;
}
if (product_image==null) {
Toast.makeText(this, "Please Select a Picture", Toast.LENGTH_LONG).show();
return;
}
ContentValues values=new ContentValues();
values.put("title",productTitle);
values.put("sale_price",salePrice);
values.put("price_shortcut", priceshortcut);
values.put("weight_shortcut", weightshortcut);
values.put("IMAGE", String.valueOf(selectedImage));
long check = dbManager.Insert(values);
if (check == 0) {
Toast.makeText(this,"record not inserted is = "+check+"",Toast.LENGTH_LONG).show();
finish();
} else {
Toast.makeText(this, "record inserted ID is = " + check + "", Toast.LENGTH_LONG).show();
finish();
}
}
public void goback(View view) {
Intent intent = new Intent(this,MainActivity.class);
startActivity(intent);
finish();
}
public void onStop() {
super.onStop();
if (currentImage != null) {
currentImage.recycle();
currentImage = null;
System.gc();
}
}
}
and the showproductadapter is..
public class showProductsAdapter extends RecyclerView.Adapter {
Context context;
java.util.List<showProducts> List;
public showProductsAdapter(Context context, List<showProducts> list) {
this.context = context;
this.List = list;
}
@NonNull
@Override
public showProductsAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view= LayoutInflater.from(parent.getContext()).inflate(R.layout.list_row,parent,false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull showProductsAdapter.ViewHolder holder, int position) {
showProducts item=List.get(position);
Uri myUri = Uri.parse(item.getImageData());
Bitmap bitmap = null;
try {
bitmap = getBitmapFromUri(myUri);
//bitmap = MediaStore.Images.Media.getBitmap(context.getContentResolver(), myUri);
} catch (IOException e) {
e.printStackTrace();
}
holder.image.setImageBitmap(bitmap);
holder.productName.setText(item.getProductName());
holder.price.setText(String.format("%s/- KG", item.getSalePrice()));
}
@Override
public int getItemCount()
{
return List.size();
}
private Bitmap getBitmapFromUri(Uri uri) throws IOException {
ParcelFileDescriptor parcelFileDescriptor =
context.getContentResolver().openFileDescriptor(uri, "r");
FileDescriptor fileDescriptor = parcelFileDescriptor.getFileDescriptor();
Bitmap image = BitmapFactory.decodeFileDescriptor(fileDescriptor);
parcelFileDescriptor.close();
return image;
}
public class ViewHolder extends RecyclerView.ViewHolder implements View.OnClickListener {
public TextView productName;
public TextView price;
public ImageView image;
public ViewHolder(View view) {
super(view);
view.setOnClickListener(this);
productName = view.findViewById(R.id.productName);
price = view.findViewById(R.id.productPrice);
image = view.findViewById(R.id.profile_image);
}
@Override
public void onClick(View v) {
int position = getAdapterPosition();
showProducts item=List.get(position);
Toast.makeText(context,"Name is "+item.getProductName(),Toast.LENGTH_LONG).show();
Intent intent = new Intent(context, UpdateProduct.class);
intent.putExtra("name", item.getProductName());
intent.putExtra("_id", item.get_id());
intent.putExtra("saleprice", item.getSalePrice());
intent.putExtra("weightshortcut", item.getWeightShortcut());
intent.putExtra("priceshortcut", item.getPriceShortcut());
intent.putExtra("image", item.getImageData());
context.startActivity(intent);
}
}
}
recyclerview activity where the inserted products are shown..
public class Products extends AppCompatActivity implements View.OnClickListener {
private RecyclerView recyclerView;
private RecyclerView.Adapter adapter;
private List<showProducts> List;
DBManager dbManager;
Button add;
Button refresh;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_products);
add=findViewById(R.id.add);
refresh=findViewById(R.id.refresh);
add.setOnClickListener(this);
refresh.setOnClickListener(this);
recyclerView=findViewById(R.id.productslist);
recyclerView.setHasFixedSize(true);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
dbManager=new DBManager(this,null,null,1);
SQLiteDatabase sqLiteDatabase=dbManager.getReadableDatabase();
Cursor cursor=dbManager.getAllProducts();
List =new ArrayList<>();
cursor.moveToFirst();
if (cursor != null && cursor.moveToFirst()) {
do {
showProducts showProducts=new showProducts(cursor.getString(0),cursor.getString(1),cursor.getString(2),cursor.getString(3),cursor.getString(4),cursor.getString(5));
List.add(showProducts);
}
while (cursor.moveToNext());
adapter= new showProductsAdapter(this,List);
recyclerView.setAdapter(adapter);
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.add:{
Intent intent = new Intent(this,AddProduct.class);
startActivity(intent);
finish();
}
break;
case R.id.refresh:{
Intent intent = new Intent(this,Products.class);
startActivity(intent);
finish();
//adapter.notifyDataSetChanged();
}
break;
}
}
}

storing images uri in sqlite database and showing them in recyclerview it works fine until the phone is restarted.
after restart the app crashes when ShowProductsAdapter is called with error.
"java.lang.SecurityException: Permission Denial: opening provider com.android.providers.media.MediaDocumentsProvider from ProcessRecord{91fdba7 5931:com.example.waqar.aatachaki/u0a240} (pid=5931, uid=10240) requires android.permission.MANAGE_DOCUMENTS or android.permission.MANAGE_DOCUMENTS"
here is my addproduct activity class
public class AddProduct extends AppCompatActivity {
EditText product_title,sale_price,price_shortcut,weight_shortcut;
Button openGallery ;
DBManager dbManager;
String productTitle ="";
String salePrice ="";
ImageView product_image;
String priceshortcut="";
String weightshortcut="";
private Bitmap currentImage;
Uri selectedImage;
Intent photoPickerIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_product);
product_title=(EditText)findViewById(R.id.p_title);
sale_price=(EditText)findViewById(R.id.p_salePrice);
price_shortcut =(EditText)findViewById(R.id.p_shortcut);
weight_shortcut=(EditText)findViewById(R.id.w_shortcut);
product_image=(ImageView) findViewById(R.id.product_image);
dbManager=new DBManager(this,null,null,5);
openGallery = (Button) findViewById(R.id.gallery);
openGallery.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
photoPickerIntent = new Intent(Intent.ACTION_OPEN_DOCUMENT );
photoPickerIntent.addCategory(Intent.CATEGORY_OPENABLE);
photoPickerIntent.setType("image/*");
startActivityForResult(photoPickerIntent, 1);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
selectedImage = data.getData();
try {
Bitmap bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), selectedImage);
product_image.setImageBitmap(bitmap);
// to access the file permission
int takeFlags = photoPickerIntent.getFlags();
takeFlags &=( Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
this.getContentResolver().takePersistableUriPermission(selectedImage, takeFlags);
this.grantUriPermission("com.example.waqar.aatachaki", selectedImage, Intent.FLAG_GRANT_WRITE_URI_PERMISSION | Intent.FLAG_GRANT_READ_URI_PERMISSION);
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void addproduct(View view) {
productTitle = product_title.getText().toString();
salePrice= sale_price.getText().toString();
priceshortcut= price_shortcut.getText().toString();
weightshortcut= weight_shortcut.getText().toString();
if (productTitle.isEmpty()) {
Toast.makeText(this, "You did not enter a Product Title", Toast.LENGTH_LONG).show();
return;
}
if (salePrice.isEmpty()) {
Toast.makeText(this, "You did not enter a Sale Price", Toast.LENGTH_LONG).show();
return;
}
if (product_image==null) {
Toast.makeText(this, "Please Select a Picture", Toast.LENGTH_LONG).show();
return;
}
ContentValues values=new ContentValues();
values.put("title",productTitle);
values.put("sale_price",salePrice);
values.put("price_shortcut", priceshortcut);
values.put("weight_shortcut", weightshortcut);
values.put("IMAGE", String.valueOf(selectedImage));
long check = dbManager.Insert(values);
if (check == 0) {
Toast.makeText(this,"record not inserted is = "+check+"",Toast.LENGTH_LONG).show();
finish();
} else {
Toast.makeText(this, "record inserted ID is = " + check + "", Toast.LENGTH_LONG).show();
finish();
}
}
public void goback(View view) {
Intent intent = new Intent(this,MainActivity.class);
startActivity(intent);
finish();
}
public void onStop() {
super.onStop();
if (currentImage != null) {
currentImage.recycle();
currentImage = null;
System.gc();
}
}
}
and the showproductadapter is..
public class showProductsAdapter extends RecyclerView.Adapter {
Context context;
java.util.List<showProducts> List;
public showProductsAdapter(Context context, List<showProducts> list) {
this.context = context;
this.List = list;
}
@NonNull
@Override
public showProductsAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view= LayoutInflater.from(parent.getContext()).inflate(R.layout.list_row,parent,false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull showProductsAdapter.ViewHolder holder, int position) {
showProducts item=List.get(position);
Uri myUri = Uri.parse(item.getImageData());
Bitmap bitmap = null;
try {
bitmap = getBitmapFromUri(myUri);
//bitmap = MediaStore.Images.Media.getBitmap(context.getContentResolver(), myUri);
} catch (IOException e) {
e.printStackTrace();
}
holder.image.setImageBitmap(bitmap);
holder.productName.setText(item.getProductName());
holder.price.setText(String.format("%s/- KG", item.getSalePrice()));
}
@Override
public int getItemCount()
{
return List.size();
}
private Bitmap getBitmapFromUri(Uri uri) throws IOException {
ParcelFileDescriptor parcelFileDescriptor =
context.getContentResolver().openFileDescriptor(uri, "r");
FileDescriptor fileDescriptor = parcelFileDescriptor.getFileDescriptor();
Bitmap image = BitmapFactory.decodeFileDescriptor(fileDescriptor);
parcelFileDescriptor.close();
return image;
}
public class ViewHolder extends RecyclerView.ViewHolder implements View.OnClickListener {
public TextView productName;
public TextView price;
public ImageView image;
public ViewHolder(View view) {
super(view);
view.setOnClickListener(this);
productName = view.findViewById(R.id.productName);
price = view.findViewById(R.id.productPrice);
image = view.findViewById(R.id.profile_image);
}
@Override
public void onClick(View v) {
int position = getAdapterPosition();
showProducts item=List.get(position);
Toast.makeText(context,"Name is "+item.getProductName(),Toast.LENGTH_LONG).show();
Intent intent = new Intent(context, UpdateProduct.class);
intent.putExtra("name", item.getProductName());
intent.putExtra("_id", item.get_id());
intent.putExtra("saleprice", item.getSalePrice());
intent.putExtra("weightshortcut", item.getWeightShortcut());
intent.putExtra("priceshortcut", item.getPriceShortcut());
intent.putExtra("image", item.getImageData());
context.startActivity(intent);
}
}
}
recyclerview activity where the inserted products are shown..
public class Products extends AppCompatActivity implements View.OnClickListener {
private RecyclerView recyclerView;
private RecyclerView.Adapter adapter;
private List<showProducts> List;
DBManager dbManager;
Button add;
Button refresh;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_products);
add=findViewById(R.id.add);
refresh=findViewById(R.id.refresh);
add.setOnClickListener(this);
refresh.setOnClickListener(this);
recyclerView=findViewById(R.id.productslist);
recyclerView.setHasFixedSize(true);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
dbManager=new DBManager(this,null,null,1);
SQLiteDatabase sqLiteDatabase=dbManager.getReadableDatabase();
Cursor cursor=dbManager.getAllProducts();
List =new ArrayList<>();
cursor.moveToFirst();
if (cursor != null && cursor.moveToFirst()) {
do {
showProducts showProducts=new showProducts(cursor.getString(0),cursor.getString(1),cursor.getString(2),cursor.getString(3),cursor.getString(4),cursor.getString(5));
List.add(showProducts);
}
while (cursor.moveToNext());
adapter= new showProductsAdapter(this,List);
recyclerView.setAdapter(adapter);
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.add:{
Intent intent = new Intent(this,AddProduct.class);
startActivity(intent);
finish();
}
break;
case R.id.refresh:{
Intent intent = new Intent(this,Products.class);
startActivity(intent);
finish();
//adapter.notifyDataSetChanged();
}
break;
}
}
}


edited 2 days ago


p.alexey
520111
520111
asked Nov 4 at 9:49
Waqar Saeed
82
82
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53139527%2fpermission-denial-showing-images-in-recyclerview-after-phone-restart-android%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
qndk4TYqZzw60,NSflhAWyC yZX