Disable Shiny Plots that Need to be Recalculated
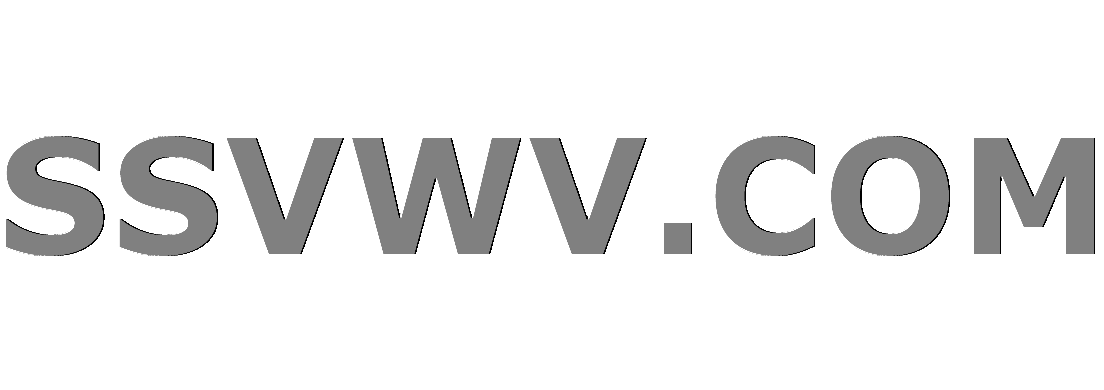
Multi tool use
up vote
2
down vote
favorite
So here's my problem:
- My shiny app has multiple graphs and maps and using the simple reactive model means it recalcs the plots ever time a checkbox is changed - too slow when the user may want to change more than one option.
- I've setup isolation and a "go button" as described in Stop reactions with isolate()
- I have more than one "row" of filters and graphs - basically I have two groups of filters that update their corresponding graphs.
The problem:
- I want to show the user that the graphs are "out of date" when they change an input value so they are prompted to recalculate with the "go" button.
I've tried:
- Using javascript handler on input/select change to add
.recalculating
to the plots. However when one of the go buttons is pressed, it recalculates only the plots related to that button BUT it removes the.recalculating
class from ALL plots (even though some have not been recalculated).
Workaround is to have either go button update all plots but that's not ideal from a resource standpoint.
To reproduce use the code below and:
- Click both buttons to generate the graphs.
- Change both filters (checkbox and radio) which will show both graphs as needing recalculating.
- Press just one button to regenerate graphs.
- See that both graphs appear to be recalculated when only one has in fact changed.
Here's a working example of how my shiny app is organized:
library('shiny')
library('ggplot2')
ui <- fluidPage(
checkboxGroupInput(inputId = 'cyl', label = 'Cylinders:', choices = unique(mtcars$cyl), selected = unique(mtcars$cyl)),
actionButton('goButton', 'Update graph!'),
plotOutput('plot'),
radioButtons(inputId = 'vs', label = 'V-shaped 09:', choices = unique(mtcars$vs), selected = 1),
actionButton('goButton2', 'Update 2nd graph!'),
plotOutput('plot2'),
#change handlers
tags$script(HTML("$(document).on('change', 'input, select', function(event) {
$('.shiny-bound-output').addClass('recalculating')
})"))
)
server <- function(input, output) {
#first data.frame - cyl
df <- reactive({
mtcars[mtcars$cyl %in% input$cyl,]
})
#second data frame - vs9
df2 <- reactive({
mtcars[mtcars$vs == input$vs,]
})
output$plot <- renderPlot({
#only run if goButton pressed
if (input$goButton == 0)
return()
isolate({
ggplot(df(), aes(x=hp, y=disp)) +
geom_point()
})
})
output$plot2 <- renderPlot({
#only update if goButton2 pressed
if(input$goButton2 == 0)
return()
isolate({
ggplot(df2(), aes(x=hp, y=disp)) +
geom_point()
})
})
}
shinyApp(ui, server)
r shiny
add a comment |
up vote
2
down vote
favorite
So here's my problem:
- My shiny app has multiple graphs and maps and using the simple reactive model means it recalcs the plots ever time a checkbox is changed - too slow when the user may want to change more than one option.
- I've setup isolation and a "go button" as described in Stop reactions with isolate()
- I have more than one "row" of filters and graphs - basically I have two groups of filters that update their corresponding graphs.
The problem:
- I want to show the user that the graphs are "out of date" when they change an input value so they are prompted to recalculate with the "go" button.
I've tried:
- Using javascript handler on input/select change to add
.recalculating
to the plots. However when one of the go buttons is pressed, it recalculates only the plots related to that button BUT it removes the.recalculating
class from ALL plots (even though some have not been recalculated).
Workaround is to have either go button update all plots but that's not ideal from a resource standpoint.
To reproduce use the code below and:
- Click both buttons to generate the graphs.
- Change both filters (checkbox and radio) which will show both graphs as needing recalculating.
- Press just one button to regenerate graphs.
- See that both graphs appear to be recalculated when only one has in fact changed.
Here's a working example of how my shiny app is organized:
library('shiny')
library('ggplot2')
ui <- fluidPage(
checkboxGroupInput(inputId = 'cyl', label = 'Cylinders:', choices = unique(mtcars$cyl), selected = unique(mtcars$cyl)),
actionButton('goButton', 'Update graph!'),
plotOutput('plot'),
radioButtons(inputId = 'vs', label = 'V-shaped 09:', choices = unique(mtcars$vs), selected = 1),
actionButton('goButton2', 'Update 2nd graph!'),
plotOutput('plot2'),
#change handlers
tags$script(HTML("$(document).on('change', 'input, select', function(event) {
$('.shiny-bound-output').addClass('recalculating')
})"))
)
server <- function(input, output) {
#first data.frame - cyl
df <- reactive({
mtcars[mtcars$cyl %in% input$cyl,]
})
#second data frame - vs9
df2 <- reactive({
mtcars[mtcars$vs == input$vs,]
})
output$plot <- renderPlot({
#only run if goButton pressed
if (input$goButton == 0)
return()
isolate({
ggplot(df(), aes(x=hp, y=disp)) +
geom_point()
})
})
output$plot2 <- renderPlot({
#only update if goButton2 pressed
if(input$goButton2 == 0)
return()
isolate({
ggplot(df2(), aes(x=hp, y=disp)) +
geom_point()
})
})
}
shinyApp(ui, server)
r shiny
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
So here's my problem:
- My shiny app has multiple graphs and maps and using the simple reactive model means it recalcs the plots ever time a checkbox is changed - too slow when the user may want to change more than one option.
- I've setup isolation and a "go button" as described in Stop reactions with isolate()
- I have more than one "row" of filters and graphs - basically I have two groups of filters that update their corresponding graphs.
The problem:
- I want to show the user that the graphs are "out of date" when they change an input value so they are prompted to recalculate with the "go" button.
I've tried:
- Using javascript handler on input/select change to add
.recalculating
to the plots. However when one of the go buttons is pressed, it recalculates only the plots related to that button BUT it removes the.recalculating
class from ALL plots (even though some have not been recalculated).
Workaround is to have either go button update all plots but that's not ideal from a resource standpoint.
To reproduce use the code below and:
- Click both buttons to generate the graphs.
- Change both filters (checkbox and radio) which will show both graphs as needing recalculating.
- Press just one button to regenerate graphs.
- See that both graphs appear to be recalculated when only one has in fact changed.
Here's a working example of how my shiny app is organized:
library('shiny')
library('ggplot2')
ui <- fluidPage(
checkboxGroupInput(inputId = 'cyl', label = 'Cylinders:', choices = unique(mtcars$cyl), selected = unique(mtcars$cyl)),
actionButton('goButton', 'Update graph!'),
plotOutput('plot'),
radioButtons(inputId = 'vs', label = 'V-shaped 09:', choices = unique(mtcars$vs), selected = 1),
actionButton('goButton2', 'Update 2nd graph!'),
plotOutput('plot2'),
#change handlers
tags$script(HTML("$(document).on('change', 'input, select', function(event) {
$('.shiny-bound-output').addClass('recalculating')
})"))
)
server <- function(input, output) {
#first data.frame - cyl
df <- reactive({
mtcars[mtcars$cyl %in% input$cyl,]
})
#second data frame - vs9
df2 <- reactive({
mtcars[mtcars$vs == input$vs,]
})
output$plot <- renderPlot({
#only run if goButton pressed
if (input$goButton == 0)
return()
isolate({
ggplot(df(), aes(x=hp, y=disp)) +
geom_point()
})
})
output$plot2 <- renderPlot({
#only update if goButton2 pressed
if(input$goButton2 == 0)
return()
isolate({
ggplot(df2(), aes(x=hp, y=disp)) +
geom_point()
})
})
}
shinyApp(ui, server)
r shiny
So here's my problem:
- My shiny app has multiple graphs and maps and using the simple reactive model means it recalcs the plots ever time a checkbox is changed - too slow when the user may want to change more than one option.
- I've setup isolation and a "go button" as described in Stop reactions with isolate()
- I have more than one "row" of filters and graphs - basically I have two groups of filters that update their corresponding graphs.
The problem:
- I want to show the user that the graphs are "out of date" when they change an input value so they are prompted to recalculate with the "go" button.
I've tried:
- Using javascript handler on input/select change to add
.recalculating
to the plots. However when one of the go buttons is pressed, it recalculates only the plots related to that button BUT it removes the.recalculating
class from ALL plots (even though some have not been recalculated).
Workaround is to have either go button update all plots but that's not ideal from a resource standpoint.
To reproduce use the code below and:
- Click both buttons to generate the graphs.
- Change both filters (checkbox and radio) which will show both graphs as needing recalculating.
- Press just one button to regenerate graphs.
- See that both graphs appear to be recalculated when only one has in fact changed.
Here's a working example of how my shiny app is organized:
library('shiny')
library('ggplot2')
ui <- fluidPage(
checkboxGroupInput(inputId = 'cyl', label = 'Cylinders:', choices = unique(mtcars$cyl), selected = unique(mtcars$cyl)),
actionButton('goButton', 'Update graph!'),
plotOutput('plot'),
radioButtons(inputId = 'vs', label = 'V-shaped 09:', choices = unique(mtcars$vs), selected = 1),
actionButton('goButton2', 'Update 2nd graph!'),
plotOutput('plot2'),
#change handlers
tags$script(HTML("$(document).on('change', 'input, select', function(event) {
$('.shiny-bound-output').addClass('recalculating')
})"))
)
server <- function(input, output) {
#first data.frame - cyl
df <- reactive({
mtcars[mtcars$cyl %in% input$cyl,]
})
#second data frame - vs9
df2 <- reactive({
mtcars[mtcars$vs == input$vs,]
})
output$plot <- renderPlot({
#only run if goButton pressed
if (input$goButton == 0)
return()
isolate({
ggplot(df(), aes(x=hp, y=disp)) +
geom_point()
})
})
output$plot2 <- renderPlot({
#only update if goButton2 pressed
if(input$goButton2 == 0)
return()
isolate({
ggplot(df2(), aes(x=hp, y=disp)) +
geom_point()
})
})
}
shinyApp(ui, server)
r shiny
r shiny
asked Nov 8 at 18:44
Michael Tallino
690314
690314
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53214225%2fdisable-shiny-plots-that-need-to-be-recalculated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5IJBxxOf zzOe9yBjngpC3G9