Retrieve data from Firebase into an entity
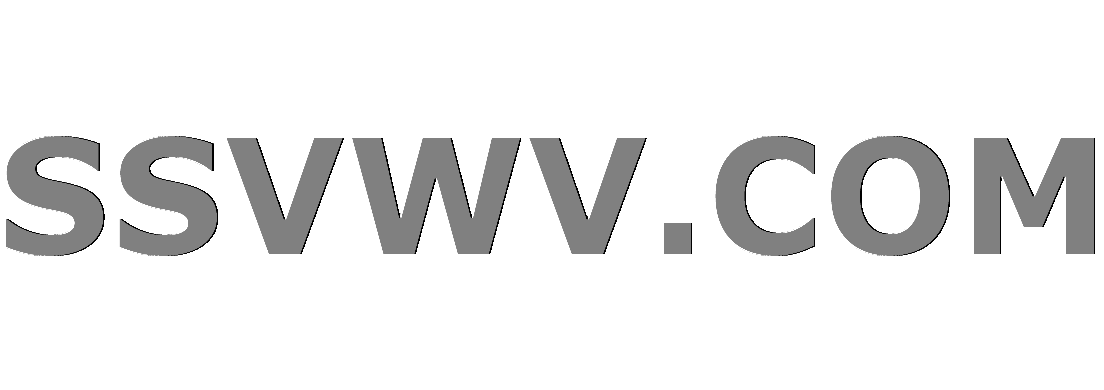
Multi tool use
up vote
0
down vote
favorite
I'm trying to retrieve data from Firebase database to add it to local database.
This code :
database = FirebaseDatabase.getInstance();
myRef = database.getReference();
Query LANGUAGES_REF = myRef.child("languages").orderByChild("id");
LANGUAGES_REF.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
if (dataSnapshot.hasChildren()) {
Iterator<DataSnapshot> iterator = dataSnapshot.getChildren().iterator();
do {
Language language = iterator.next().getValue(Language.class);
languageR.insert(language);
} while (iterator.hasNext());
}
is not working , and I know it's because Language.class is an entity.
Is there a way other than creating a new class with getters and setters and full constructor ... and repeating everything??
The error I got was:
com.google.firebase.database.DatabaseException: Can't convert object
of type java.lang.Long to type
com.android.android.Database.Entities.Language
at this line :
Language language = iterator.next().getValue(Language.class);
Thanks
edit:
firebase database structure
"languages" : {
"LHZ7cAeOdPn-LwHNjIk" : {
"id" : 1,
"langName" : "Russian"
},
"LHZ7cAtXTGBlnWsdQOd" : {
"id" : 2,
"langName" : "French"
},
"LHZ7cAvrRs0rgI-PaJd" : {
"id" : 3,
"langName" : "Turkish"
}
}
language.class
import java.io.Serializable;
import androidx.room.Entity;
import androidx.room.PrimaryKey;
@Entity
public class Language implements Serializable {
@PrimaryKey(autoGenerate = true)
private int id;
private String langName;
public int getId() {
return id;
}
public String getLangName() {
return langName;
}
public Language(String langName) {
this.langName = langName;
}
public void setId(int id) {
this.id = id;
}
}
java


add a comment |
up vote
0
down vote
favorite
I'm trying to retrieve data from Firebase database to add it to local database.
This code :
database = FirebaseDatabase.getInstance();
myRef = database.getReference();
Query LANGUAGES_REF = myRef.child("languages").orderByChild("id");
LANGUAGES_REF.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
if (dataSnapshot.hasChildren()) {
Iterator<DataSnapshot> iterator = dataSnapshot.getChildren().iterator();
do {
Language language = iterator.next().getValue(Language.class);
languageR.insert(language);
} while (iterator.hasNext());
}
is not working , and I know it's because Language.class is an entity.
Is there a way other than creating a new class with getters and setters and full constructor ... and repeating everything??
The error I got was:
com.google.firebase.database.DatabaseException: Can't convert object
of type java.lang.Long to type
com.android.android.Database.Entities.Language
at this line :
Language language = iterator.next().getValue(Language.class);
Thanks
edit:
firebase database structure
"languages" : {
"LHZ7cAeOdPn-LwHNjIk" : {
"id" : 1,
"langName" : "Russian"
},
"LHZ7cAtXTGBlnWsdQOd" : {
"id" : 2,
"langName" : "French"
},
"LHZ7cAvrRs0rgI-PaJd" : {
"id" : 3,
"langName" : "Turkish"
}
}
language.class
import java.io.Serializable;
import androidx.room.Entity;
import androidx.room.PrimaryKey;
@Entity
public class Language implements Serializable {
@PrimaryKey(autoGenerate = true)
private int id;
private String langName;
public int getId() {
return id;
}
public String getLangName() {
return langName;
}
public Language(String langName) {
this.langName = langName;
}
public void setId(int id) {
this.id = id;
}
}
java


Please add your database structure.
– Alex Mamo
Nov 8 at 17:18
1
Please also add the entire code that you are using to get the data, including the reference on which you are attaching the listener. Please responde with a comment, once you added the code.
– Alex Mamo
Nov 8 at 18:14
Sorry for posting little info earlier, actually, I did create a languageCast class and its working fine, the question now is, was there another solution using the Entity language.class?
– farid anwar
Nov 8 at 18:57
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to retrieve data from Firebase database to add it to local database.
This code :
database = FirebaseDatabase.getInstance();
myRef = database.getReference();
Query LANGUAGES_REF = myRef.child("languages").orderByChild("id");
LANGUAGES_REF.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
if (dataSnapshot.hasChildren()) {
Iterator<DataSnapshot> iterator = dataSnapshot.getChildren().iterator();
do {
Language language = iterator.next().getValue(Language.class);
languageR.insert(language);
} while (iterator.hasNext());
}
is not working , and I know it's because Language.class is an entity.
Is there a way other than creating a new class with getters and setters and full constructor ... and repeating everything??
The error I got was:
com.google.firebase.database.DatabaseException: Can't convert object
of type java.lang.Long to type
com.android.android.Database.Entities.Language
at this line :
Language language = iterator.next().getValue(Language.class);
Thanks
edit:
firebase database structure
"languages" : {
"LHZ7cAeOdPn-LwHNjIk" : {
"id" : 1,
"langName" : "Russian"
},
"LHZ7cAtXTGBlnWsdQOd" : {
"id" : 2,
"langName" : "French"
},
"LHZ7cAvrRs0rgI-PaJd" : {
"id" : 3,
"langName" : "Turkish"
}
}
language.class
import java.io.Serializable;
import androidx.room.Entity;
import androidx.room.PrimaryKey;
@Entity
public class Language implements Serializable {
@PrimaryKey(autoGenerate = true)
private int id;
private String langName;
public int getId() {
return id;
}
public String getLangName() {
return langName;
}
public Language(String langName) {
this.langName = langName;
}
public void setId(int id) {
this.id = id;
}
}
java


I'm trying to retrieve data from Firebase database to add it to local database.
This code :
database = FirebaseDatabase.getInstance();
myRef = database.getReference();
Query LANGUAGES_REF = myRef.child("languages").orderByChild("id");
LANGUAGES_REF.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
if (dataSnapshot.hasChildren()) {
Iterator<DataSnapshot> iterator = dataSnapshot.getChildren().iterator();
do {
Language language = iterator.next().getValue(Language.class);
languageR.insert(language);
} while (iterator.hasNext());
}
is not working , and I know it's because Language.class is an entity.
Is there a way other than creating a new class with getters and setters and full constructor ... and repeating everything??
The error I got was:
com.google.firebase.database.DatabaseException: Can't convert object
of type java.lang.Long to type
com.android.android.Database.Entities.Language
at this line :
Language language = iterator.next().getValue(Language.class);
Thanks
edit:
firebase database structure
"languages" : {
"LHZ7cAeOdPn-LwHNjIk" : {
"id" : 1,
"langName" : "Russian"
},
"LHZ7cAtXTGBlnWsdQOd" : {
"id" : 2,
"langName" : "French"
},
"LHZ7cAvrRs0rgI-PaJd" : {
"id" : 3,
"langName" : "Turkish"
}
}
language.class
import java.io.Serializable;
import androidx.room.Entity;
import androidx.room.PrimaryKey;
@Entity
public class Language implements Serializable {
@PrimaryKey(autoGenerate = true)
private int id;
private String langName;
public int getId() {
return id;
}
public String getLangName() {
return langName;
}
public Language(String langName) {
this.langName = langName;
}
public void setId(int id) {
this.id = id;
}
}
java


java


edited Nov 8 at 19:22


Alex Mamo
37.9k62556
37.9k62556
asked Nov 8 at 17:17


farid anwar
314
314
Please add your database structure.
– Alex Mamo
Nov 8 at 17:18
1
Please also add the entire code that you are using to get the data, including the reference on which you are attaching the listener. Please responde with a comment, once you added the code.
– Alex Mamo
Nov 8 at 18:14
Sorry for posting little info earlier, actually, I did create a languageCast class and its working fine, the question now is, was there another solution using the Entity language.class?
– farid anwar
Nov 8 at 18:57
add a comment |
Please add your database structure.
– Alex Mamo
Nov 8 at 17:18
1
Please also add the entire code that you are using to get the data, including the reference on which you are attaching the listener. Please responde with a comment, once you added the code.
– Alex Mamo
Nov 8 at 18:14
Sorry for posting little info earlier, actually, I did create a languageCast class and its working fine, the question now is, was there another solution using the Entity language.class?
– farid anwar
Nov 8 at 18:57
Please add your database structure.
– Alex Mamo
Nov 8 at 17:18
Please add your database structure.
– Alex Mamo
Nov 8 at 17:18
1
1
Please also add the entire code that you are using to get the data, including the reference on which you are attaching the listener. Please responde with a comment, once you added the code.
– Alex Mamo
Nov 8 at 18:14
Please also add the entire code that you are using to get the data, including the reference on which you are attaching the listener. Please responde with a comment, once you added the code.
– Alex Mamo
Nov 8 at 18:14
Sorry for posting little info earlier, actually, I did create a languageCast class and its working fine, the question now is, was there another solution using the Entity language.class?
– farid anwar
Nov 8 at 18:57
Sorry for posting little info earlier, actually, I did create a languageCast class and its working fine, the question now is, was there another solution using the Entity language.class?
– farid anwar
Nov 8 at 18:57
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
To solve this, you need to remove the do-while
loop because there is no need to iterate using getChildren()
method, you can get the data directly from the dataSnapshot
object like this:
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
Language language = dataSnapshot.getValue(Language.class);
languageR.insert(language);
Log.d(TAG, language.getLangName());
}
The output in your logcat will be:
Russian
French
Turkish
now i'm a getting aDatabase.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an@Ignore
annotatin and it's working fine ... Thanks a million @alexmamo
– farid anwar
Nov 8 at 19:41
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
To solve this, you need to remove the do-while
loop because there is no need to iterate using getChildren()
method, you can get the data directly from the dataSnapshot
object like this:
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
Language language = dataSnapshot.getValue(Language.class);
languageR.insert(language);
Log.d(TAG, language.getLangName());
}
The output in your logcat will be:
Russian
French
Turkish
now i'm a getting aDatabase.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an@Ignore
annotatin and it's working fine ... Thanks a million @alexmamo
– farid anwar
Nov 8 at 19:41
add a comment |
up vote
1
down vote
accepted
To solve this, you need to remove the do-while
loop because there is no need to iterate using getChildren()
method, you can get the data directly from the dataSnapshot
object like this:
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
Language language = dataSnapshot.getValue(Language.class);
languageR.insert(language);
Log.d(TAG, language.getLangName());
}
The output in your logcat will be:
Russian
French
Turkish
now i'm a getting aDatabase.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an@Ignore
annotatin and it's working fine ... Thanks a million @alexmamo
– farid anwar
Nov 8 at 19:41
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
To solve this, you need to remove the do-while
loop because there is no need to iterate using getChildren()
method, you can get the data directly from the dataSnapshot
object like this:
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
Language language = dataSnapshot.getValue(Language.class);
languageR.insert(language);
Log.d(TAG, language.getLangName());
}
The output in your logcat will be:
Russian
French
Turkish
To solve this, you need to remove the do-while
loop because there is no need to iterate using getChildren()
method, you can get the data directly from the dataSnapshot
object like this:
@Override
public void onChildAdded(@NonNull DataSnapshot dataSnapshot, @Nullable String s) {
Language language = dataSnapshot.getValue(Language.class);
languageR.insert(language);
Log.d(TAG, language.getLangName());
}
The output in your logcat will be:
Russian
French
Turkish
answered Nov 8 at 19:15


Alex Mamo
37.9k62556
37.9k62556
now i'm a getting aDatabase.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an@Ignore
annotatin and it's working fine ... Thanks a million @alexmamo
– farid anwar
Nov 8 at 19:41
add a comment |
now i'm a getting aDatabase.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an@Ignore
annotatin and it's working fine ... Thanks a million @alexmamo
– farid anwar
Nov 8 at 19:41
now i'm a getting a
Database.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an @Ignore
annotatin and it's working fine ... Thanks a million @alexmamo– farid anwar
Nov 8 at 19:41
now i'm a getting a
Database.Entities.Language does not define a no-argument constructor. If you are using ProGuard, make sure these constructors are not stripped.
I added a no argument constructor, and an @Ignore
annotatin and it's working fine ... Thanks a million @alexmamo– farid anwar
Nov 8 at 19:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53212957%2fretrieve-data-from-firebase-into-an-entity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rxzHqg g1dUe0vvl YgKrmD2PWzpo7F lMRXIQQg2 qURYEX9yeYikVSXPjXLeo JBTby,r
Please add your database structure.
– Alex Mamo
Nov 8 at 17:18
1
Please also add the entire code that you are using to get the data, including the reference on which you are attaching the listener. Please responde with a comment, once you added the code.
– Alex Mamo
Nov 8 at 18:14
Sorry for posting little info earlier, actually, I did create a languageCast class and its working fine, the question now is, was there another solution using the Entity language.class?
– farid anwar
Nov 8 at 18:57