C# doesn't connect to DataBase (SQL)
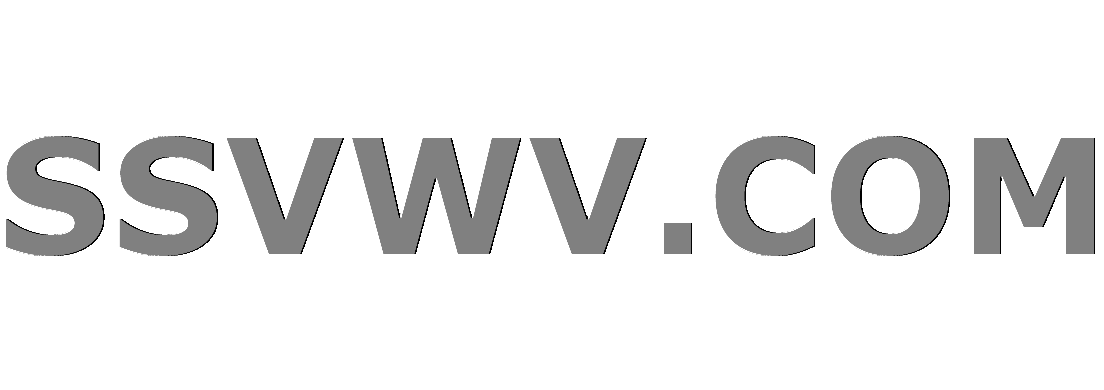
Multi tool use
up vote
0
down vote
favorite
I have a database to manage a school (students and classes).
I have a class with the code to connect to the DataBase and then I call the functions in the main program.
When I try to interact with the DataBase, it warns me that it could not connect to the DataBase or it exceeded the connection time.
I tried to add an ssslmode but it didn't work. I also tried to add a port but it didn't work.
Code for the class:
public class ligacao
{
public MySqlConnection connection;
string server;
public string data_base;
string user_id;
string password;
public void inicializa()
{
server = "localhost";
data_base = "escola";
user_id = "root";
password = "usbw";
string connection_string;
string sslmode = "none";
connection_string = "SERVER=" + server + ";" + "DATABASE=" + data_base + ";" + "UID=" + user_id + "PASSWORD=" + password + ";" + "SslMode=" + sslmode + ";";
connection = new MySqlConnection(connection_string);
}
public bool open_connection()
{
try
{
connection.Open();
return true;
}
catch (MySqlException ex)
{
switch (ex.Number)
{
case 0: MessageBox.Show("Couldn't connect t DataBase."); break; // couldn't connect to database
case 1042: MessageBox.Show("Exceded the connection time"); break; // exceeded the connection time
case 1045: MessageBox.Show("Username/password are incorrect"); break;
}
return false;
}
}
public bool close_connection()
{
try
{
connection.Close();
return true;
}
catch (MySqlException ex)
{
MessageBox.Show(ex.Message);
return false;
}
}
}
Code for the Main Program:
public partial class consultas : Form
{
ligacao x = new ligacao();
public consultas()
{
InitializeComponent();
x.inicializa();
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
}
private void consultas_Load(object sender, EventArgs e)
{
//define query
string query = "SELECT designacao FROM disciplinas";
//open connection
if (x.open_connection())
{
//create the comand and associates the query with the connection through the connector
MySqlCommand cmd = new MySqlCommand(query, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["designacao"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
//define query
string queryBI = "SELECT bi FROM alunos";
//open connection
if (x.open_connection())
{
//create the commando and associate the query with the connection through the constructor
MySqlCommand cmd = new MySqlCommand(queryBI, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["bi"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
}
}
c# mysql
add a comment |
up vote
0
down vote
favorite
I have a database to manage a school (students and classes).
I have a class with the code to connect to the DataBase and then I call the functions in the main program.
When I try to interact with the DataBase, it warns me that it could not connect to the DataBase or it exceeded the connection time.
I tried to add an ssslmode but it didn't work. I also tried to add a port but it didn't work.
Code for the class:
public class ligacao
{
public MySqlConnection connection;
string server;
public string data_base;
string user_id;
string password;
public void inicializa()
{
server = "localhost";
data_base = "escola";
user_id = "root";
password = "usbw";
string connection_string;
string sslmode = "none";
connection_string = "SERVER=" + server + ";" + "DATABASE=" + data_base + ";" + "UID=" + user_id + "PASSWORD=" + password + ";" + "SslMode=" + sslmode + ";";
connection = new MySqlConnection(connection_string);
}
public bool open_connection()
{
try
{
connection.Open();
return true;
}
catch (MySqlException ex)
{
switch (ex.Number)
{
case 0: MessageBox.Show("Couldn't connect t DataBase."); break; // couldn't connect to database
case 1042: MessageBox.Show("Exceded the connection time"); break; // exceeded the connection time
case 1045: MessageBox.Show("Username/password are incorrect"); break;
}
return false;
}
}
public bool close_connection()
{
try
{
connection.Close();
return true;
}
catch (MySqlException ex)
{
MessageBox.Show(ex.Message);
return false;
}
}
}
Code for the Main Program:
public partial class consultas : Form
{
ligacao x = new ligacao();
public consultas()
{
InitializeComponent();
x.inicializa();
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
}
private void consultas_Load(object sender, EventArgs e)
{
//define query
string query = "SELECT designacao FROM disciplinas";
//open connection
if (x.open_connection())
{
//create the comand and associates the query with the connection through the connector
MySqlCommand cmd = new MySqlCommand(query, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["designacao"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
//define query
string queryBI = "SELECT bi FROM alunos";
//open connection
if (x.open_connection())
{
//create the commando and associate the query with the connection through the constructor
MySqlCommand cmd = new MySqlCommand(queryBI, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["bi"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
}
}
c# mysql
Possible duplicate of How to form a correct MySQL connection string?
– duDE
Nov 7 at 12:44
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a database to manage a school (students and classes).
I have a class with the code to connect to the DataBase and then I call the functions in the main program.
When I try to interact with the DataBase, it warns me that it could not connect to the DataBase or it exceeded the connection time.
I tried to add an ssslmode but it didn't work. I also tried to add a port but it didn't work.
Code for the class:
public class ligacao
{
public MySqlConnection connection;
string server;
public string data_base;
string user_id;
string password;
public void inicializa()
{
server = "localhost";
data_base = "escola";
user_id = "root";
password = "usbw";
string connection_string;
string sslmode = "none";
connection_string = "SERVER=" + server + ";" + "DATABASE=" + data_base + ";" + "UID=" + user_id + "PASSWORD=" + password + ";" + "SslMode=" + sslmode + ";";
connection = new MySqlConnection(connection_string);
}
public bool open_connection()
{
try
{
connection.Open();
return true;
}
catch (MySqlException ex)
{
switch (ex.Number)
{
case 0: MessageBox.Show("Couldn't connect t DataBase."); break; // couldn't connect to database
case 1042: MessageBox.Show("Exceded the connection time"); break; // exceeded the connection time
case 1045: MessageBox.Show("Username/password are incorrect"); break;
}
return false;
}
}
public bool close_connection()
{
try
{
connection.Close();
return true;
}
catch (MySqlException ex)
{
MessageBox.Show(ex.Message);
return false;
}
}
}
Code for the Main Program:
public partial class consultas : Form
{
ligacao x = new ligacao();
public consultas()
{
InitializeComponent();
x.inicializa();
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
}
private void consultas_Load(object sender, EventArgs e)
{
//define query
string query = "SELECT designacao FROM disciplinas";
//open connection
if (x.open_connection())
{
//create the comand and associates the query with the connection through the connector
MySqlCommand cmd = new MySqlCommand(query, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["designacao"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
//define query
string queryBI = "SELECT bi FROM alunos";
//open connection
if (x.open_connection())
{
//create the commando and associate the query with the connection through the constructor
MySqlCommand cmd = new MySqlCommand(queryBI, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["bi"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
}
}
c# mysql
I have a database to manage a school (students and classes).
I have a class with the code to connect to the DataBase and then I call the functions in the main program.
When I try to interact with the DataBase, it warns me that it could not connect to the DataBase or it exceeded the connection time.
I tried to add an ssslmode but it didn't work. I also tried to add a port but it didn't work.
Code for the class:
public class ligacao
{
public MySqlConnection connection;
string server;
public string data_base;
string user_id;
string password;
public void inicializa()
{
server = "localhost";
data_base = "escola";
user_id = "root";
password = "usbw";
string connection_string;
string sslmode = "none";
connection_string = "SERVER=" + server + ";" + "DATABASE=" + data_base + ";" + "UID=" + user_id + "PASSWORD=" + password + ";" + "SslMode=" + sslmode + ";";
connection = new MySqlConnection(connection_string);
}
public bool open_connection()
{
try
{
connection.Open();
return true;
}
catch (MySqlException ex)
{
switch (ex.Number)
{
case 0: MessageBox.Show("Couldn't connect t DataBase."); break; // couldn't connect to database
case 1042: MessageBox.Show("Exceded the connection time"); break; // exceeded the connection time
case 1045: MessageBox.Show("Username/password are incorrect"); break;
}
return false;
}
}
public bool close_connection()
{
try
{
connection.Close();
return true;
}
catch (MySqlException ex)
{
MessageBox.Show(ex.Message);
return false;
}
}
}
Code for the Main Program:
public partial class consultas : Form
{
ligacao x = new ligacao();
public consultas()
{
InitializeComponent();
x.inicializa();
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
}
private void consultas_Load(object sender, EventArgs e)
{
//define query
string query = "SELECT designacao FROM disciplinas";
//open connection
if (x.open_connection())
{
//create the comand and associates the query with the connection through the connector
MySqlCommand cmd = new MySqlCommand(query, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["designacao"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
//define query
string queryBI = "SELECT bi FROM alunos";
//open connection
if (x.open_connection())
{
//create the commando and associate the query with the connection through the constructor
MySqlCommand cmd = new MySqlCommand(queryBI, x.connection);
//create datareader and execute the command
MySqlDataReader dataReader = cmd.ExecuteReader();
//show data in combobox1
if (dataReader.Read())
{
comboBox1.Items.Add(dataReader["bi"]);
}
//close dataReader
dataReader.Close();
//close connection
x.close_connection();
}
}
}
c# mysql
c# mysql
edited Nov 7 at 12:39
mjwills
14.2k42439
14.2k42439
asked Nov 7 at 12:36


Joana Ferrão
105
105
Possible duplicate of How to form a correct MySQL connection string?
– duDE
Nov 7 at 12:44
add a comment |
Possible duplicate of How to form a correct MySQL connection string?
– duDE
Nov 7 at 12:44
Possible duplicate of How to form a correct MySQL connection string?
– duDE
Nov 7 at 12:44
Possible duplicate of How to form a correct MySQL connection string?
– duDE
Nov 7 at 12:44
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
I think there is something wrong with your connection string. Try using the MySqlConnectionStringBuilder:
MySqlConnectionStringBuilder builder = new MySqlConnectionStringBuilder();
builder.Host = "localhost";
builder.UserId = "root";
builder.Database = "escola";
builder.Password = "usbw";
connection = new MySqlConnection(builder.ConnectionString);
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
add a comment |
up vote
-1
down vote
Try This :
connection_string = @"Data Source = " + server + "; Initial Catalog = " + data_base + "; Integrated Security=True;uid=myUser;password=myPass;";
1
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I think there is something wrong with your connection string. Try using the MySqlConnectionStringBuilder:
MySqlConnectionStringBuilder builder = new MySqlConnectionStringBuilder();
builder.Host = "localhost";
builder.UserId = "root";
builder.Database = "escola";
builder.Password = "usbw";
connection = new MySqlConnection(builder.ConnectionString);
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
add a comment |
up vote
0
down vote
I think there is something wrong with your connection string. Try using the MySqlConnectionStringBuilder:
MySqlConnectionStringBuilder builder = new MySqlConnectionStringBuilder();
builder.Host = "localhost";
builder.UserId = "root";
builder.Database = "escola";
builder.Password = "usbw";
connection = new MySqlConnection(builder.ConnectionString);
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
add a comment |
up vote
0
down vote
up vote
0
down vote
I think there is something wrong with your connection string. Try using the MySqlConnectionStringBuilder:
MySqlConnectionStringBuilder builder = new MySqlConnectionStringBuilder();
builder.Host = "localhost";
builder.UserId = "root";
builder.Database = "escola";
builder.Password = "usbw";
connection = new MySqlConnection(builder.ConnectionString);
I think there is something wrong with your connection string. Try using the MySqlConnectionStringBuilder:
MySqlConnectionStringBuilder builder = new MySqlConnectionStringBuilder();
builder.Host = "localhost";
builder.UserId = "root";
builder.Database = "escola";
builder.Password = "usbw";
connection = new MySqlConnection(builder.ConnectionString);
answered Nov 7 at 12:56


Palle Due
1,8551717
1,8551717
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
add a comment |
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
Thank you for the help but now an error shows up saying "CS1061 C# does not contain a definition for and no extension method accepting a first argument of type could be found (are you missing a using directive or an assembly reference?)". Do you know how to fix it?
– Joana Ferrão
Nov 10 at 16:56
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
@JoanaFerrão: You missed the culprit. Where do you get the error?
– Palle Due
Nov 12 at 9:40
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
it warns me that it could not connect to the DataBase or it exceeded the connection time
– Joana Ferrão
Nov 12 at 9:56
add a comment |
up vote
-1
down vote
Try This :
connection_string = @"Data Source = " + server + "; Initial Catalog = " + data_base + "; Integrated Security=True;uid=myUser;password=myPass;";
1
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
add a comment |
up vote
-1
down vote
Try This :
connection_string = @"Data Source = " + server + "; Initial Catalog = " + data_base + "; Integrated Security=True;uid=myUser;password=myPass;";
1
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
add a comment |
up vote
-1
down vote
up vote
-1
down vote
Try This :
connection_string = @"Data Source = " + server + "; Initial Catalog = " + data_base + "; Integrated Security=True;uid=myUser;password=myPass;";
Try This :
connection_string = @"Data Source = " + server + "; Initial Catalog = " + data_base + "; Integrated Security=True;uid=myUser;password=myPass;";
edited Nov 7 at 12:52
answered Nov 7 at 12:45


Rajendran S
1317
1317
1
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
add a comment |
1
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
1
1
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
That won't help much if you want to connect with user name and password.
– Palle Due
Nov 7 at 12:48
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53189623%2fc-sharp-doesnt-connect-to-database-sql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TO,Iga8Ah9NEVxSOULVhGL2zzaCO8,70WNGUKaQyiKy3qXRf
Possible duplicate of How to form a correct MySQL connection string?
– duDE
Nov 7 at 12:44