How to properly render array parameter with swagger and flask restplus?
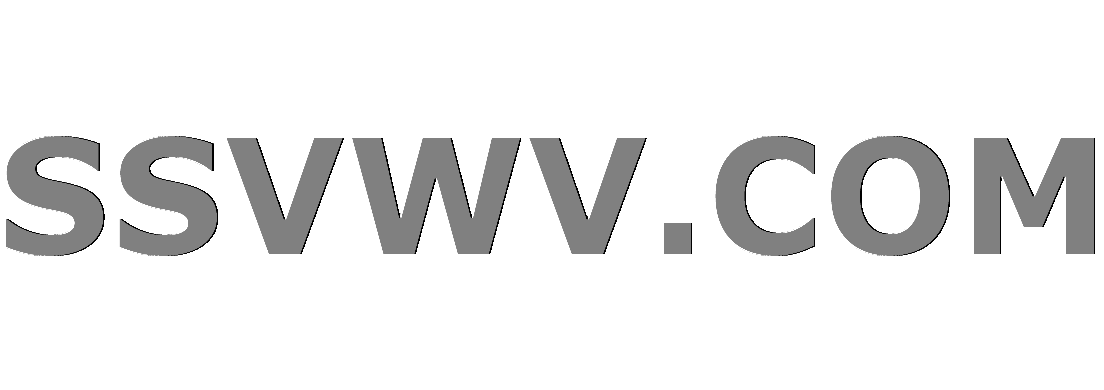
Multi tool use
I am implementing a Flask Application with Flask RestPlus that must receive a list of ids (integers) on a HTTP GET endpoint, so the application client can get a list of results matching those identifiers, for instance, with...
GET /resource/1,2,3,4
GET /resource?id=1,2,3
GET /resource?id=1&id=2&id=3
... which is not supported by default by none of werkzeug bultin converters, but following the steps in this stackoverflow post the url parsing is working properly and I can receive the list of ids with this converter...
class ListOfIntegerConverter(BaseConverter):
def __init__(self, url_map, randomify=False):
self.regex = r'd+(?:,d+)*,?'
def to_python(self, value):
return [int(x) for x in value.split(',')]
def to_url(self, value):
return ','.join(str(x) for x in value)
... which is then properly registered as stated here ...
app.url_map.converters['list_of_int'] = ListOfIntegerConverter
... and used to parse the url parameters ...
@my_namespace.route('/<list_of_int:list_of_ids>/', methods=["GET"])
class MyResourceById(Resource):
def get(self, list_of_ids):
[print(id, type(id)) for id in list_of_ids]
... but the generated swagger documentation is rendering the field as a plain string instead of an array or list of integers, which should be rendered like this...
I know that swagger supports multi-value parameters, but I can't manage to make Flask RestPlus render this field accordingly. Is there anyone who knows how to do it that can help me out with some advice?
Thanks in advance! :)
python python-3.x flask swagger flask-restplus
add a comment |
I am implementing a Flask Application with Flask RestPlus that must receive a list of ids (integers) on a HTTP GET endpoint, so the application client can get a list of results matching those identifiers, for instance, with...
GET /resource/1,2,3,4
GET /resource?id=1,2,3
GET /resource?id=1&id=2&id=3
... which is not supported by default by none of werkzeug bultin converters, but following the steps in this stackoverflow post the url parsing is working properly and I can receive the list of ids with this converter...
class ListOfIntegerConverter(BaseConverter):
def __init__(self, url_map, randomify=False):
self.regex = r'd+(?:,d+)*,?'
def to_python(self, value):
return [int(x) for x in value.split(',')]
def to_url(self, value):
return ','.join(str(x) for x in value)
... which is then properly registered as stated here ...
app.url_map.converters['list_of_int'] = ListOfIntegerConverter
... and used to parse the url parameters ...
@my_namespace.route('/<list_of_int:list_of_ids>/', methods=["GET"])
class MyResourceById(Resource):
def get(self, list_of_ids):
[print(id, type(id)) for id in list_of_ids]
... but the generated swagger documentation is rendering the field as a plain string instead of an array or list of integers, which should be rendered like this...
I know that swagger supports multi-value parameters, but I can't manage to make Flask RestPlus render this field accordingly. Is there anyone who knows how to do it that can help me out with some advice?
Thanks in advance! :)
python python-3.x flask swagger flask-restplus
add a comment |
I am implementing a Flask Application with Flask RestPlus that must receive a list of ids (integers) on a HTTP GET endpoint, so the application client can get a list of results matching those identifiers, for instance, with...
GET /resource/1,2,3,4
GET /resource?id=1,2,3
GET /resource?id=1&id=2&id=3
... which is not supported by default by none of werkzeug bultin converters, but following the steps in this stackoverflow post the url parsing is working properly and I can receive the list of ids with this converter...
class ListOfIntegerConverter(BaseConverter):
def __init__(self, url_map, randomify=False):
self.regex = r'd+(?:,d+)*,?'
def to_python(self, value):
return [int(x) for x in value.split(',')]
def to_url(self, value):
return ','.join(str(x) for x in value)
... which is then properly registered as stated here ...
app.url_map.converters['list_of_int'] = ListOfIntegerConverter
... and used to parse the url parameters ...
@my_namespace.route('/<list_of_int:list_of_ids>/', methods=["GET"])
class MyResourceById(Resource):
def get(self, list_of_ids):
[print(id, type(id)) for id in list_of_ids]
... but the generated swagger documentation is rendering the field as a plain string instead of an array or list of integers, which should be rendered like this...
I know that swagger supports multi-value parameters, but I can't manage to make Flask RestPlus render this field accordingly. Is there anyone who knows how to do it that can help me out with some advice?
Thanks in advance! :)
python python-3.x flask swagger flask-restplus
I am implementing a Flask Application with Flask RestPlus that must receive a list of ids (integers) on a HTTP GET endpoint, so the application client can get a list of results matching those identifiers, for instance, with...
GET /resource/1,2,3,4
GET /resource?id=1,2,3
GET /resource?id=1&id=2&id=3
... which is not supported by default by none of werkzeug bultin converters, but following the steps in this stackoverflow post the url parsing is working properly and I can receive the list of ids with this converter...
class ListOfIntegerConverter(BaseConverter):
def __init__(self, url_map, randomify=False):
self.regex = r'd+(?:,d+)*,?'
def to_python(self, value):
return [int(x) for x in value.split(',')]
def to_url(self, value):
return ','.join(str(x) for x in value)
... which is then properly registered as stated here ...
app.url_map.converters['list_of_int'] = ListOfIntegerConverter
... and used to parse the url parameters ...
@my_namespace.route('/<list_of_int:list_of_ids>/', methods=["GET"])
class MyResourceById(Resource):
def get(self, list_of_ids):
[print(id, type(id)) for id in list_of_ids]
... but the generated swagger documentation is rendering the field as a plain string instead of an array or list of integers, which should be rendered like this...
I know that swagger supports multi-value parameters, but I can't manage to make Flask RestPlus render this field accordingly. Is there anyone who knows how to do it that can help me out with some advice?
Thanks in advance! :)
python python-3.x flask swagger flask-restplus
python python-3.x flask swagger flask-restplus
edited Nov 14 '18 at 9:03
danieldeveloper001
asked Nov 13 '18 at 17:02


danieldeveloper001danieldeveloper001
879613
879613
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think you have to use arg parsers:
id_parser = api.parser()
id_parser.add_argument('id', type=int, action='append')
@api.route('/ids', methods=["GET"])
@api.doc(parser=id_parser)
class MyResourceById(Resource):
@staticmethod
def get():
ids = request.args.getlist('id')
print(ids)
return ids
I'd do it as above, but you can probably get it working with your converter too.
The documents say parser is being deprecated but not anytime soon, and I couldn't personally find an alternative.
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53286097%2fhow-to-properly-render-array-parameter-with-swagger-and-flask-restplus%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you have to use arg parsers:
id_parser = api.parser()
id_parser.add_argument('id', type=int, action='append')
@api.route('/ids', methods=["GET"])
@api.doc(parser=id_parser)
class MyResourceById(Resource):
@staticmethod
def get():
ids = request.args.getlist('id')
print(ids)
return ids
I'd do it as above, but you can probably get it working with your converter too.
The documents say parser is being deprecated but not anytime soon, and I couldn't personally find an alternative.
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
add a comment |
I think you have to use arg parsers:
id_parser = api.parser()
id_parser.add_argument('id', type=int, action='append')
@api.route('/ids', methods=["GET"])
@api.doc(parser=id_parser)
class MyResourceById(Resource):
@staticmethod
def get():
ids = request.args.getlist('id')
print(ids)
return ids
I'd do it as above, but you can probably get it working with your converter too.
The documents say parser is being deprecated but not anytime soon, and I couldn't personally find an alternative.
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
add a comment |
I think you have to use arg parsers:
id_parser = api.parser()
id_parser.add_argument('id', type=int, action='append')
@api.route('/ids', methods=["GET"])
@api.doc(parser=id_parser)
class MyResourceById(Resource):
@staticmethod
def get():
ids = request.args.getlist('id')
print(ids)
return ids
I'd do it as above, but you can probably get it working with your converter too.
The documents say parser is being deprecated but not anytime soon, and I couldn't personally find an alternative.
I think you have to use arg parsers:
id_parser = api.parser()
id_parser.add_argument('id', type=int, action='append')
@api.route('/ids', methods=["GET"])
@api.doc(parser=id_parser)
class MyResourceById(Resource):
@staticmethod
def get():
ids = request.args.getlist('id')
print(ids)
return ids
I'd do it as above, but you can probably get it working with your converter too.
The documents say parser is being deprecated but not anytime soon, and I couldn't personally find an alternative.
answered Nov 19 '18 at 1:25
MelSchlemmingMelSchlemming
262
262
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
add a comment |
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
You seem to be a new contributor, welcome, and thanks, your answer is correct and made me notice one mistake on my code! It is fair that I do can parse the parameters but cannot see it as a list on swagger, since I'm only using the api.marshal_with decorator, which documents the get method, but not the api.doc decorator, which documents the resource.
– danieldeveloper001
Nov 19 '18 at 10:30
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll upvote, it do solves the documentation problem, even though the flask restfull documentation says the parser is deprecated, but I'll still try to figure out how to use my custom parser to complement your solution, probably getting it from the url_map.
– danieldeveloper001
Nov 19 '18 at 10:34
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
I'll accept the answer, since I couldn't find a better solution that doesn't use the deprecated parser. Thanks again!
– danieldeveloper001
Dec 5 '18 at 10:43
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53286097%2fhow-to-properly-render-array-parameter-with-swagger-and-flask-restplus%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yvKyVRM4bdbjk3rVSJU H8m7jze