How to call one component function from another component
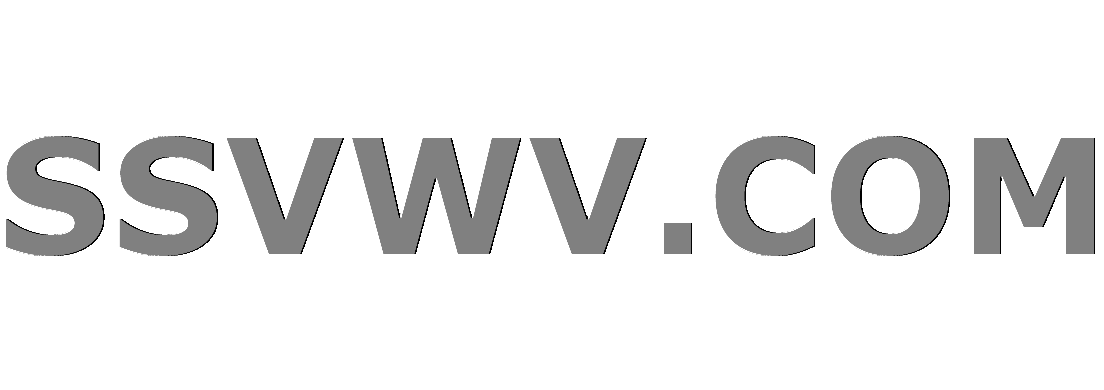
Multi tool use
Hi i am new for Angular and i am trying to call one component function from another component and I was reading other similar questions but they have a scenario where the components are child or sibling components with the declaration on the html,but my scenario is diff for this i used below code but its not working(method not calling) can some one help me please
MessageService :
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs/Observable';
import { Subject } from 'rxjs/Subject';
@Injectable()
export class MessageService {
private _listners = new Subject<any>();
listen(): Observable<any> {
return this._listners.asObservable();
}
filter(filterBy: string) {
this._listners.next(filterBy);
}
}
ClassA:
@Component({
selector: 'header',
templateUrl: `
<section class="container">
<button (click)="clickFilter()">Open filter</button>
</section>
`
})
export class HeaderComponent {
constructor(private _messageService: MessageService){}
clickFilter():void {
this._messageService.filter('Register click');
}
}
ClassB:
@Component({
selector: 'store',
template: `<article class="card">
Test
</article>`
})
export class StoreComponent {
constructor(private _messageService: MessageService){
this._messageService.listen().subscribe((m:any) => {
console.log(m);
this.onFilterClick(m);
})
}
onFilterClick(event) {
console.log('Fire onFilterClick: ', event);
}
}
angular
add a comment |
Hi i am new for Angular and i am trying to call one component function from another component and I was reading other similar questions but they have a scenario where the components are child or sibling components with the declaration on the html,but my scenario is diff for this i used below code but its not working(method not calling) can some one help me please
MessageService :
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs/Observable';
import { Subject } from 'rxjs/Subject';
@Injectable()
export class MessageService {
private _listners = new Subject<any>();
listen(): Observable<any> {
return this._listners.asObservable();
}
filter(filterBy: string) {
this._listners.next(filterBy);
}
}
ClassA:
@Component({
selector: 'header',
templateUrl: `
<section class="container">
<button (click)="clickFilter()">Open filter</button>
</section>
`
})
export class HeaderComponent {
constructor(private _messageService: MessageService){}
clickFilter():void {
this._messageService.filter('Register click');
}
}
ClassB:
@Component({
selector: 'store',
template: `<article class="card">
Test
</article>`
})
export class StoreComponent {
constructor(private _messageService: MessageService){
this._messageService.listen().subscribe((m:any) => {
console.log(m);
this.onFilterClick(m);
})
}
onFilterClick(event) {
console.log('Fire onFilterClick: ', event);
}
}
angular
1
You should change the subject into aBehaviorSubject
and be sure that your service is a singleton
– Jacopo Sciampi
Nov 12 '18 at 7:44
Are you able to create a Stackblitz that demonstrates the issue?
– user184994
Nov 12 '18 at 7:48
@Jacopo Sciampi i tried but not working
– AbhiRam
Nov 12 '18 at 9:00
are there any errors in the console?
– Mac_W
Nov 12 '18 at 12:16
add a comment |
Hi i am new for Angular and i am trying to call one component function from another component and I was reading other similar questions but they have a scenario where the components are child or sibling components with the declaration on the html,but my scenario is diff for this i used below code but its not working(method not calling) can some one help me please
MessageService :
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs/Observable';
import { Subject } from 'rxjs/Subject';
@Injectable()
export class MessageService {
private _listners = new Subject<any>();
listen(): Observable<any> {
return this._listners.asObservable();
}
filter(filterBy: string) {
this._listners.next(filterBy);
}
}
ClassA:
@Component({
selector: 'header',
templateUrl: `
<section class="container">
<button (click)="clickFilter()">Open filter</button>
</section>
`
})
export class HeaderComponent {
constructor(private _messageService: MessageService){}
clickFilter():void {
this._messageService.filter('Register click');
}
}
ClassB:
@Component({
selector: 'store',
template: `<article class="card">
Test
</article>`
})
export class StoreComponent {
constructor(private _messageService: MessageService){
this._messageService.listen().subscribe((m:any) => {
console.log(m);
this.onFilterClick(m);
})
}
onFilterClick(event) {
console.log('Fire onFilterClick: ', event);
}
}
angular
Hi i am new for Angular and i am trying to call one component function from another component and I was reading other similar questions but they have a scenario where the components are child or sibling components with the declaration on the html,but my scenario is diff for this i used below code but its not working(method not calling) can some one help me please
MessageService :
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs/Observable';
import { Subject } from 'rxjs/Subject';
@Injectable()
export class MessageService {
private _listners = new Subject<any>();
listen(): Observable<any> {
return this._listners.asObservable();
}
filter(filterBy: string) {
this._listners.next(filterBy);
}
}
ClassA:
@Component({
selector: 'header',
templateUrl: `
<section class="container">
<button (click)="clickFilter()">Open filter</button>
</section>
`
})
export class HeaderComponent {
constructor(private _messageService: MessageService){}
clickFilter():void {
this._messageService.filter('Register click');
}
}
ClassB:
@Component({
selector: 'store',
template: `<article class="card">
Test
</article>`
})
export class StoreComponent {
constructor(private _messageService: MessageService){
this._messageService.listen().subscribe((m:any) => {
console.log(m);
this.onFilterClick(m);
})
}
onFilterClick(event) {
console.log('Fire onFilterClick: ', event);
}
}
angular
angular
asked Nov 12 '18 at 7:37
AbhiRam
44711139
44711139
1
You should change the subject into aBehaviorSubject
and be sure that your service is a singleton
– Jacopo Sciampi
Nov 12 '18 at 7:44
Are you able to create a Stackblitz that demonstrates the issue?
– user184994
Nov 12 '18 at 7:48
@Jacopo Sciampi i tried but not working
– AbhiRam
Nov 12 '18 at 9:00
are there any errors in the console?
– Mac_W
Nov 12 '18 at 12:16
add a comment |
1
You should change the subject into aBehaviorSubject
and be sure that your service is a singleton
– Jacopo Sciampi
Nov 12 '18 at 7:44
Are you able to create a Stackblitz that demonstrates the issue?
– user184994
Nov 12 '18 at 7:48
@Jacopo Sciampi i tried but not working
– AbhiRam
Nov 12 '18 at 9:00
are there any errors in the console?
– Mac_W
Nov 12 '18 at 12:16
1
1
You should change the subject into a
BehaviorSubject
and be sure that your service is a singleton– Jacopo Sciampi
Nov 12 '18 at 7:44
You should change the subject into a
BehaviorSubject
and be sure that your service is a singleton– Jacopo Sciampi
Nov 12 '18 at 7:44
Are you able to create a Stackblitz that demonstrates the issue?
– user184994
Nov 12 '18 at 7:48
Are you able to create a Stackblitz that demonstrates the issue?
– user184994
Nov 12 '18 at 7:48
@Jacopo Sciampi i tried but not working
– AbhiRam
Nov 12 '18 at 9:00
@Jacopo Sciampi i tried but not working
– AbhiRam
Nov 12 '18 at 9:00
are there any errors in the console?
– Mac_W
Nov 12 '18 at 12:16
are there any errors in the console?
– Mac_W
Nov 12 '18 at 12:16
add a comment |
2 Answers
2
active
oldest
votes
Issue
Your implementation is looking fine. The only issue with is where the MessageService
is provided. You had declared MessageService
in two different Modules
of HeaderComponent
and StoreComponent
. Due to which its creating two separate instance of MessageService
as a result they are not able to communicate.
Fix
You should provide the MessageService
in Module which is common to HeaderComponent
and StoreComponent
.
If you are not sure about the common Module then you can test it by providing in AppModule
.
app.module.ts
providers: [
MessageService
]
Note : Do not forget to remove MessageService
from the providers
of other Modules
.
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
How many places you have providedMessageService
. Make sure its just inAppModule
and no where else, not even inComponent
orModule
.
– Sunil Singh
Nov 12 '18 at 8:49
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
|
show 3 more comments
MessageService must be added to parent module of ClassA and ClassB. Than your code works fine.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257661%2fhow-to-call-one-component-function-from-another-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Issue
Your implementation is looking fine. The only issue with is where the MessageService
is provided. You had declared MessageService
in two different Modules
of HeaderComponent
and StoreComponent
. Due to which its creating two separate instance of MessageService
as a result they are not able to communicate.
Fix
You should provide the MessageService
in Module which is common to HeaderComponent
and StoreComponent
.
If you are not sure about the common Module then you can test it by providing in AppModule
.
app.module.ts
providers: [
MessageService
]
Note : Do not forget to remove MessageService
from the providers
of other Modules
.
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
How many places you have providedMessageService
. Make sure its just inAppModule
and no where else, not even inComponent
orModule
.
– Sunil Singh
Nov 12 '18 at 8:49
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
|
show 3 more comments
Issue
Your implementation is looking fine. The only issue with is where the MessageService
is provided. You had declared MessageService
in two different Modules
of HeaderComponent
and StoreComponent
. Due to which its creating two separate instance of MessageService
as a result they are not able to communicate.
Fix
You should provide the MessageService
in Module which is common to HeaderComponent
and StoreComponent
.
If you are not sure about the common Module then you can test it by providing in AppModule
.
app.module.ts
providers: [
MessageService
]
Note : Do not forget to remove MessageService
from the providers
of other Modules
.
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
How many places you have providedMessageService
. Make sure its just inAppModule
and no where else, not even inComponent
orModule
.
– Sunil Singh
Nov 12 '18 at 8:49
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
|
show 3 more comments
Issue
Your implementation is looking fine. The only issue with is where the MessageService
is provided. You had declared MessageService
in two different Modules
of HeaderComponent
and StoreComponent
. Due to which its creating two separate instance of MessageService
as a result they are not able to communicate.
Fix
You should provide the MessageService
in Module which is common to HeaderComponent
and StoreComponent
.
If you are not sure about the common Module then you can test it by providing in AppModule
.
app.module.ts
providers: [
MessageService
]
Note : Do not forget to remove MessageService
from the providers
of other Modules
.
Issue
Your implementation is looking fine. The only issue with is where the MessageService
is provided. You had declared MessageService
in two different Modules
of HeaderComponent
and StoreComponent
. Due to which its creating two separate instance of MessageService
as a result they are not able to communicate.
Fix
You should provide the MessageService
in Module which is common to HeaderComponent
and StoreComponent
.
If you are not sure about the common Module then you can test it by providing in AppModule
.
app.module.ts
providers: [
MessageService
]
Note : Do not forget to remove MessageService
from the providers
of other Modules
.
edited Nov 12 '18 at 7:56
answered Nov 12 '18 at 7:49


Sunil Singh
6,1372626
6,1372626
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
How many places you have providedMessageService
. Make sure its just inAppModule
and no where else, not even inComponent
orModule
.
– Sunil Singh
Nov 12 '18 at 8:49
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
|
show 3 more comments
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
How many places you have providedMessageService
. Make sure its just inAppModule
and no where else, not even inComponent
orModule
.
– Sunil Singh
Nov 12 '18 at 8:49
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
i done everything what you said but not working
– AbhiRam
Nov 12 '18 at 8:41
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
Are you getting any error in console ?
– Sunil Singh
Nov 12 '18 at 8:43
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
no errors i am getting
– AbhiRam
Nov 12 '18 at 8:44
How many places you have provided
MessageService
. Make sure its just in AppModule
and no where else, not even in Component
or Module
.– Sunil Singh
Nov 12 '18 at 8:49
How many places you have provided
MessageService
. Make sure its just in AppModule
and no where else, not even in Component
or Module
.– Sunil Singh
Nov 12 '18 at 8:49
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
its not there more i just provided in Appmodule
– AbhiRam
Nov 12 '18 at 8:51
|
show 3 more comments
MessageService must be added to parent module of ClassA and ClassB. Than your code works fine.
add a comment |
MessageService must be added to parent module of ClassA and ClassB. Than your code works fine.
add a comment |
MessageService must be added to parent module of ClassA and ClassB. Than your code works fine.
MessageService must be added to parent module of ClassA and ClassB. Than your code works fine.
answered Nov 12 '18 at 7:59
Serkan KONAKCI
216
216
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257661%2fhow-to-call-one-component-function-from-another-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0M4,wLONjgogno,ug1lk m t 4QvIhvbw zaQt ImCol U,K
1
You should change the subject into a
BehaviorSubject
and be sure that your service is a singleton– Jacopo Sciampi
Nov 12 '18 at 7:44
Are you able to create a Stackblitz that demonstrates the issue?
– user184994
Nov 12 '18 at 7:48
@Jacopo Sciampi i tried but not working
– AbhiRam
Nov 12 '18 at 9:00
are there any errors in the console?
– Mac_W
Nov 12 '18 at 12:16