My Table View is not Reloading When I type in the Search Bar to Retrieve the Google Books Information
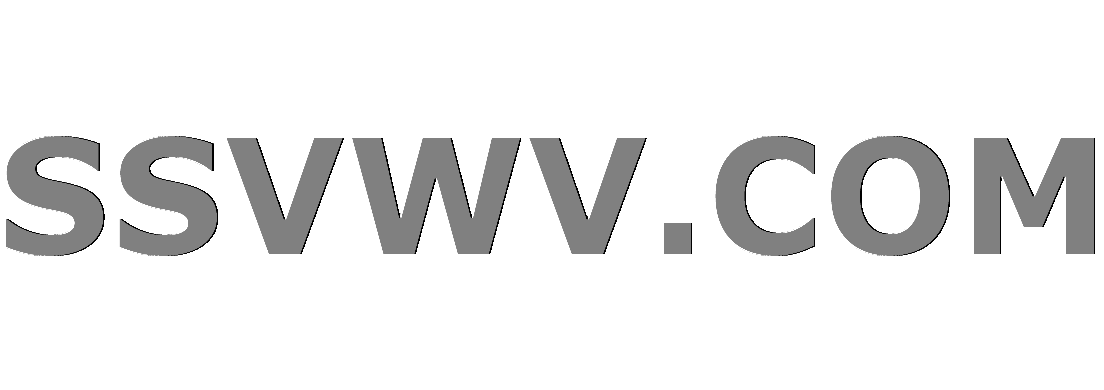
Multi tool use
This is my controller that I am using to lookup the specific books. When I type in the search bar, no book information is displayed back to me while I type or after I finish typing. I would like to understand why and find a solution that would remedy this problem.
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar!
@IBOutlet weak var tableView: UITableView!
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
let tableItems = json["Items"] as! [[String: AnyObject]]
self.booksFound = tableItems
self.tableView.reloadData()
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarButtonClicked(searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
self.queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
}
ios swift project google-books
add a comment |
This is my controller that I am using to lookup the specific books. When I type in the search bar, no book information is displayed back to me while I type or after I finish typing. I would like to understand why and find a solution that would remedy this problem.
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar!
@IBOutlet weak var tableView: UITableView!
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
let tableItems = json["Items"] as! [[String: AnyObject]]
self.booksFound = tableItems
self.tableView.reloadData()
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarButtonClicked(searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
self.queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
}
ios swift project google-books
What debugging have you done? What is happening compared to what you expect to happen?
– rmaddy
Nov 12 '18 at 20:12
@rmaddy I am able to segue to this view. When I am in this view the search bar and table view shows but once I begin to type in the search bar the query seems to not return values into the table view.
– Justin Reddick
Nov 12 '18 at 20:31
1. You make no attempt to handle text entered into the search bar. 2. TheUISearchBarDelegate
method that you show in your question isn't any method in the delegate protocol. 3. Is the search bar delegate set at all?
– rmaddy
Nov 12 '18 at 20:34
add a comment |
This is my controller that I am using to lookup the specific books. When I type in the search bar, no book information is displayed back to me while I type or after I finish typing. I would like to understand why and find a solution that would remedy this problem.
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar!
@IBOutlet weak var tableView: UITableView!
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
let tableItems = json["Items"] as! [[String: AnyObject]]
self.booksFound = tableItems
self.tableView.reloadData()
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarButtonClicked(searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
self.queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
}
ios swift project google-books
This is my controller that I am using to lookup the specific books. When I type in the search bar, no book information is displayed back to me while I type or after I finish typing. I would like to understand why and find a solution that would remedy this problem.
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar!
@IBOutlet weak var tableView: UITableView!
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
let tableItems = json["Items"] as! [[String: AnyObject]]
self.booksFound = tableItems
self.tableView.reloadData()
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarButtonClicked(searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
self.queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
}
ios swift project google-books
ios swift project google-books
edited Nov 12 '18 at 20:11


rmaddy
238k27310376
238k27310376
asked Nov 12 '18 at 19:11


Justin Reddick
264
264
What debugging have you done? What is happening compared to what you expect to happen?
– rmaddy
Nov 12 '18 at 20:12
@rmaddy I am able to segue to this view. When I am in this view the search bar and table view shows but once I begin to type in the search bar the query seems to not return values into the table view.
– Justin Reddick
Nov 12 '18 at 20:31
1. You make no attempt to handle text entered into the search bar. 2. TheUISearchBarDelegate
method that you show in your question isn't any method in the delegate protocol. 3. Is the search bar delegate set at all?
– rmaddy
Nov 12 '18 at 20:34
add a comment |
What debugging have you done? What is happening compared to what you expect to happen?
– rmaddy
Nov 12 '18 at 20:12
@rmaddy I am able to segue to this view. When I am in this view the search bar and table view shows but once I begin to type in the search bar the query seems to not return values into the table view.
– Justin Reddick
Nov 12 '18 at 20:31
1. You make no attempt to handle text entered into the search bar. 2. TheUISearchBarDelegate
method that you show in your question isn't any method in the delegate protocol. 3. Is the search bar delegate set at all?
– rmaddy
Nov 12 '18 at 20:34
What debugging have you done? What is happening compared to what you expect to happen?
– rmaddy
Nov 12 '18 at 20:12
What debugging have you done? What is happening compared to what you expect to happen?
– rmaddy
Nov 12 '18 at 20:12
@rmaddy I am able to segue to this view. When I am in this view the search bar and table view shows but once I begin to type in the search bar the query seems to not return values into the table view.
– Justin Reddick
Nov 12 '18 at 20:31
@rmaddy I am able to segue to this view. When I am in this view the search bar and table view shows but once I begin to type in the search bar the query seems to not return values into the table view.
– Justin Reddick
Nov 12 '18 at 20:31
1. You make no attempt to handle text entered into the search bar. 2. The
UISearchBarDelegate
method that you show in your question isn't any method in the delegate protocol. 3. Is the search bar delegate set at all?– rmaddy
Nov 12 '18 at 20:34
1. You make no attempt to handle text entered into the search bar. 2. The
UISearchBarDelegate
method that you show in your question isn't any method in the delegate protocol. 3. Is the search bar delegate set at all?– rmaddy
Nov 12 '18 at 20:34
add a comment |
1 Answer
1
active
oldest
votes
Probably you forget to set UISearchBar
delegate
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
Also, you need below in place of func searchBarButtonClicked(searchBar: UISearchBar)
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
// your code
}
Key in response is items
not Items
Use json["items"]
in place of json["Items"]
Complete code:
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
@IBOutlet weak var tableView: UITableView! {
didSet {
tableView.delegate = self
tableView.dataSource = self
}
}
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { [weak self] (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
guard let tableItems = json["items"] as? [[String: AnyObject]] else {
self?.booksFound = [[String: AnyObject]]()
return
}
print(tableItems)
self?.booksFound = tableItems
DispatchQueue.main.async {
self?.tableView.reloadData()
}
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@JustinReddick just check its returningjson
. check by adding a debugger orprint
statement
– Satish
Nov 12 '18 at 20:59
@JustinReddick in place ofjson["Items"]
usejson["Items"]
. Its smalli
in place of capitalI
– Satish
Nov 12 '18 at 21:06
|
show 6 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268618%2fmy-table-view-is-not-reloading-when-i-type-in-the-search-bar-to-retrieve-the-goo%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Probably you forget to set UISearchBar
delegate
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
Also, you need below in place of func searchBarButtonClicked(searchBar: UISearchBar)
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
// your code
}
Key in response is items
not Items
Use json["items"]
in place of json["Items"]
Complete code:
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
@IBOutlet weak var tableView: UITableView! {
didSet {
tableView.delegate = self
tableView.dataSource = self
}
}
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { [weak self] (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
guard let tableItems = json["items"] as? [[String: AnyObject]] else {
self?.booksFound = [[String: AnyObject]]()
return
}
print(tableItems)
self?.booksFound = tableItems
DispatchQueue.main.async {
self?.tableView.reloadData()
}
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@JustinReddick just check its returningjson
. check by adding a debugger orprint
statement
– Satish
Nov 12 '18 at 20:59
@JustinReddick in place ofjson["Items"]
usejson["Items"]
. Its smalli
in place of capitalI
– Satish
Nov 12 '18 at 21:06
|
show 6 more comments
Probably you forget to set UISearchBar
delegate
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
Also, you need below in place of func searchBarButtonClicked(searchBar: UISearchBar)
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
// your code
}
Key in response is items
not Items
Use json["items"]
in place of json["Items"]
Complete code:
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
@IBOutlet weak var tableView: UITableView! {
didSet {
tableView.delegate = self
tableView.dataSource = self
}
}
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { [weak self] (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
guard let tableItems = json["items"] as? [[String: AnyObject]] else {
self?.booksFound = [[String: AnyObject]]()
return
}
print(tableItems)
self?.booksFound = tableItems
DispatchQueue.main.async {
self?.tableView.reloadData()
}
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@JustinReddick just check its returningjson
. check by adding a debugger orprint
statement
– Satish
Nov 12 '18 at 20:59
@JustinReddick in place ofjson["Items"]
usejson["Items"]
. Its smalli
in place of capitalI
– Satish
Nov 12 '18 at 21:06
|
show 6 more comments
Probably you forget to set UISearchBar
delegate
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
Also, you need below in place of func searchBarButtonClicked(searchBar: UISearchBar)
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
// your code
}
Key in response is items
not Items
Use json["items"]
in place of json["Items"]
Complete code:
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
@IBOutlet weak var tableView: UITableView! {
didSet {
tableView.delegate = self
tableView.dataSource = self
}
}
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { [weak self] (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
guard let tableItems = json["items"] as? [[String: AnyObject]] else {
self?.booksFound = [[String: AnyObject]]()
return
}
print(tableItems)
self?.booksFound = tableItems
DispatchQueue.main.async {
self?.tableView.reloadData()
}
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
Probably you forget to set UISearchBar
delegate
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
Also, you need below in place of func searchBarButtonClicked(searchBar: UISearchBar)
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
// your code
}
Key in response is items
not Items
Use json["items"]
in place of json["Items"]
Complete code:
import UIKit
class TextbookSearchViewController: UIViewController, UITableViewDelegate {
@IBOutlet weak var searchBar: UISearchBar! {
didSet {
searchBar.delegate = self
}
}
@IBOutlet weak var tableView: UITableView! {
didSet {
tableView.delegate = self
tableView.dataSource = self
}
}
var booksFound = [[String: AnyObject]]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
}
extension TextbookSearchViewController: UITableViewDataSource {
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return booksFound.count
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCell(withIdentifier: "BookCell", for: indexPath)
if let volumeInfo = self.booksFound[indexPath.row]["volumeInfo"] as? [String: AnyObject] {
cell.textLabel?.text = volumeInfo["title"] as? String
cell.detailTextLabel?.text = volumeInfo["subtitle"] as? String
}
return cell
}
}
extension TextbookSearchViewController: UISearchBarDelegate {
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
let bookTitle = searchBar.text?.addingPercentEncoding(withAllowedCharacters: CharacterSet.urlQueryAllowed)
queryBooks(bookTitle: bookTitle!)
searchBar.resignFirstResponder()
}
func queryBooks(bookTitle: String) {
let stringURL = "https://www.googleapis.com/books/v1/volumes?q=(bookTitle)"
guard let url = URL(string: stringURL) else {
print("Problem with URL")
return
}
let urlRequest = URLRequest(url: url as URL)
let urlSession = URLSession.shared
let queryTask = urlSession.dataTask(with: urlRequest) { [weak self] (data, response, error) in
guard let jsonData = data else {
print("No Information could be Found:")
return
}
do {
let json = try JSONSerialization.jsonObject(with: jsonData, options: JSONSerialization.ReadingOptions.allowFragments) as! [String: AnyObject]
guard let tableItems = json["items"] as? [[String: AnyObject]] else {
self?.booksFound = [[String: AnyObject]]()
return
}
print(tableItems)
self?.booksFound = tableItems
DispatchQueue.main.async {
self?.tableView.reloadData()
}
} catch {
print("Error with JSON: ")
}
}
queryTask.resume()
}
}
edited Nov 12 '18 at 21:47
answered Nov 12 '18 at 20:34
Satish
1,016615
1,016615
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@JustinReddick just check its returningjson
. check by adding a debugger orprint
statement
– Satish
Nov 12 '18 at 20:59
@JustinReddick in place ofjson["Items"]
usejson["Items"]
. Its smalli
in place of capitalI
– Satish
Nov 12 '18 at 21:06
|
show 6 more comments
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@JustinReddick just check its returningjson
. check by adding a debugger orprint
statement
– Satish
Nov 12 '18 at 20:59
@JustinReddick in place ofjson["Items"]
usejson["Items"]
. Its smalli
in place of capitalI
– Satish
Nov 12 '18 at 21:06
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
It might be set in the storyboard. But there is so many other problems besides this.
– rmaddy
Nov 12 '18 at 20:34
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@rmaddy I am fairly new to Swift and wanted to see if this cool project could be done. Could you possibly comment on the other things that you see wrong with this controller?
– Justin Reddick
Nov 12 '18 at 20:53
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@Satish I implemented both of those suggestions and nothing changes. I am able to type in the search bar and the information from the query function I created is supposed to return some google books information based on the text I input but the tables are not filling with various books.
– Justin Reddick
Nov 12 '18 at 20:55
@JustinReddick just check its returning
json
. check by adding a debugger or print
statement– Satish
Nov 12 '18 at 20:59
@JustinReddick just check its returning
json
. check by adding a debugger or print
statement– Satish
Nov 12 '18 at 20:59
@JustinReddick in place of
json["Items"]
use json["Items"]
. Its small i
in place of capital I
– Satish
Nov 12 '18 at 21:06
@JustinReddick in place of
json["Items"]
use json["Items"]
. Its small i
in place of capital I
– Satish
Nov 12 '18 at 21:06
|
show 6 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268618%2fmy-table-view-is-not-reloading-when-i-type-in-the-search-bar-to-retrieve-the-goo%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sI3zMRVn HZwbkTfMEG5ZpY5YhVP,ZPZJIamY,0sDLBO
What debugging have you done? What is happening compared to what you expect to happen?
– rmaddy
Nov 12 '18 at 20:12
@rmaddy I am able to segue to this view. When I am in this view the search bar and table view shows but once I begin to type in the search bar the query seems to not return values into the table view.
– Justin Reddick
Nov 12 '18 at 20:31
1. You make no attempt to handle text entered into the search bar. 2. The
UISearchBarDelegate
method that you show in your question isn't any method in the delegate protocol. 3. Is the search bar delegate set at all?– rmaddy
Nov 12 '18 at 20:34