JFrame labels appear overlapped
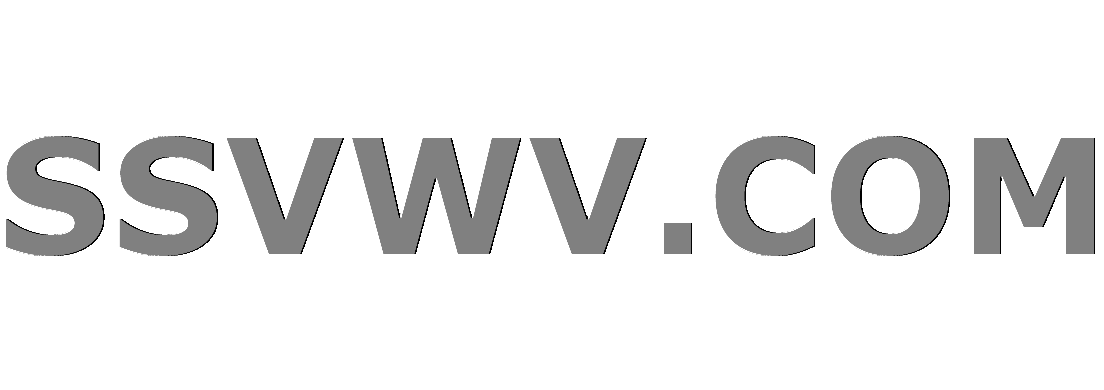
Multi tool use
i just programmed a program which has a JFrame containing an array of JLabels. The array gets the postition of the single JLabels assigned by a for-loop:
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
Do not get confused, my JFrame's name is "dialog".
There is one simple problem: The for-loop doesnt work like a for-loop should, and i dont know why, here is the result in my JFrame:
Imgur.
Dont care about the single JLabel Entries, the interesting thing is the position of "Telefon".
If i set the beginning of the loop to
for(int i=0; i<label_entries.length-1; i++){...}
it is the same problem, just with another JLabel.
I hope you can help me,
Greetings from Germany
Edit: Here is the full code:
JFrame dialog = new JFrame();
dialog.setBounds(25, 50, 500, 500);
dialog.setTitle("Eintrag hinzufügen");
dialog.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
JLabel label_entries = new JLabel[11];
JTextField textfields = new JTextField[11];
label_entries[0] = new JLabel("Vorname :");
label_entries[1] = new JLabel("Nachname :");
label_entries[2] = new JLabel("Nummer :");
label_entries[3] = new JLabel("Geburtstag :");
label_entries[4] = new JLabel("Land :");
label_entries[5] = new JLabel("PLZ :");
label_entries[6] = new JLabel("Stadt :");
label_entries[7] = new JLabel("Strasse :");
label_entries[8] = new JLabel("Hausnummer :");
label_entries[9] = new JLabel("E-Mail :");
label_entries[10] = new JLabel("Telefon :");
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
This should be easier to understand...
java jframe jlabel
add a comment |
i just programmed a program which has a JFrame containing an array of JLabels. The array gets the postition of the single JLabels assigned by a for-loop:
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
Do not get confused, my JFrame's name is "dialog".
There is one simple problem: The for-loop doesnt work like a for-loop should, and i dont know why, here is the result in my JFrame:
Imgur.
Dont care about the single JLabel Entries, the interesting thing is the position of "Telefon".
If i set the beginning of the loop to
for(int i=0; i<label_entries.length-1; i++){...}
it is the same problem, just with another JLabel.
I hope you can help me,
Greetings from Germany
Edit: Here is the full code:
JFrame dialog = new JFrame();
dialog.setBounds(25, 50, 500, 500);
dialog.setTitle("Eintrag hinzufügen");
dialog.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
JLabel label_entries = new JLabel[11];
JTextField textfields = new JTextField[11];
label_entries[0] = new JLabel("Vorname :");
label_entries[1] = new JLabel("Nachname :");
label_entries[2] = new JLabel("Nummer :");
label_entries[3] = new JLabel("Geburtstag :");
label_entries[4] = new JLabel("Land :");
label_entries[5] = new JLabel("PLZ :");
label_entries[6] = new JLabel("Stadt :");
label_entries[7] = new JLabel("Strasse :");
label_entries[8] = new JLabel("Hausnummer :");
label_entries[9] = new JLabel("E-Mail :");
label_entries[10] = new JLabel("Telefon :");
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
This should be easier to understand...
java jframe jlabel
add a comment |
i just programmed a program which has a JFrame containing an array of JLabels. The array gets the postition of the single JLabels assigned by a for-loop:
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
Do not get confused, my JFrame's name is "dialog".
There is one simple problem: The for-loop doesnt work like a for-loop should, and i dont know why, here is the result in my JFrame:
Imgur.
Dont care about the single JLabel Entries, the interesting thing is the position of "Telefon".
If i set the beginning of the loop to
for(int i=0; i<label_entries.length-1; i++){...}
it is the same problem, just with another JLabel.
I hope you can help me,
Greetings from Germany
Edit: Here is the full code:
JFrame dialog = new JFrame();
dialog.setBounds(25, 50, 500, 500);
dialog.setTitle("Eintrag hinzufügen");
dialog.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
JLabel label_entries = new JLabel[11];
JTextField textfields = new JTextField[11];
label_entries[0] = new JLabel("Vorname :");
label_entries[1] = new JLabel("Nachname :");
label_entries[2] = new JLabel("Nummer :");
label_entries[3] = new JLabel("Geburtstag :");
label_entries[4] = new JLabel("Land :");
label_entries[5] = new JLabel("PLZ :");
label_entries[6] = new JLabel("Stadt :");
label_entries[7] = new JLabel("Strasse :");
label_entries[8] = new JLabel("Hausnummer :");
label_entries[9] = new JLabel("E-Mail :");
label_entries[10] = new JLabel("Telefon :");
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
This should be easier to understand...
java jframe jlabel
i just programmed a program which has a JFrame containing an array of JLabels. The array gets the postition of the single JLabels assigned by a for-loop:
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
Do not get confused, my JFrame's name is "dialog".
There is one simple problem: The for-loop doesnt work like a for-loop should, and i dont know why, here is the result in my JFrame:
Imgur.
Dont care about the single JLabel Entries, the interesting thing is the position of "Telefon".
If i set the beginning of the loop to
for(int i=0; i<label_entries.length-1; i++){...}
it is the same problem, just with another JLabel.
I hope you can help me,
Greetings from Germany
Edit: Here is the full code:
JFrame dialog = new JFrame();
dialog.setBounds(25, 50, 500, 500);
dialog.setTitle("Eintrag hinzufügen");
dialog.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
JLabel label_entries = new JLabel[11];
JTextField textfields = new JTextField[11];
label_entries[0] = new JLabel("Vorname :");
label_entries[1] = new JLabel("Nachname :");
label_entries[2] = new JLabel("Nummer :");
label_entries[3] = new JLabel("Geburtstag :");
label_entries[4] = new JLabel("Land :");
label_entries[5] = new JLabel("PLZ :");
label_entries[6] = new JLabel("Stadt :");
label_entries[7] = new JLabel("Strasse :");
label_entries[8] = new JLabel("Hausnummer :");
label_entries[9] = new JLabel("E-Mail :");
label_entries[10] = new JLabel("Telefon :");
for(int i=0; i<label_entries.length; i++){
label_entries[i].setLocation(10, i*30);
label_entries[i].setSize(120,30);
dialog.add(label_entries[i]);
}
This should be easier to understand...
java jframe jlabel
java jframe jlabel
edited Nov 21 '18 at 2:13


Sotirios Delimanolis
211k40493583
211k40493583
asked Nov 21 '18 at 0:37


AnimalzAnimalz
104
104
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
One obvious problem is that you are setting absolute positions of your components. Typically a LayoutManager
is used for this purpose.
To clear whatever the default layout manager of a JFrame
's content pane is, set it to null
just after creating the frame.
JFrame dialog = new JFrame();
dialog.setLayout(null);
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
add a comment |
As the javadoc of JFrame
says:
The default content pane will have a
BorderLayout
manager set on it.
Javadoc of BorderLayout
says:
A border layout lays out a container, arranging and resizing its components to fit in five regions: north, south, east, west, and center. Each region may contain no more than one component, and is identified by a corresponding constant:
NORTH
,SOUTH
,EAST
,WEST
, andCENTER
. When adding a component to a container with a border layout, use one of these five constants, for example:
Panel p = new Panel();
p.setLayout(new BorderLayout());
p.add(new Button("Okay"), BorderLayout.SOUTH);
As a convenience,
BorderLayout
interprets the absence of a string specification the same as the constantCENTER
:
Panel p2 = new Panel();
p2.setLayout(new BorderLayout());
p2.add(new TextArea()); // Same as p.add(new TextArea(), BorderLayout.CENTER);
Since you call the 1-arg version of add()
, all your JLabels are added with BorderLayout.CENTER
, and so the last one wins, and the BorderLayout
manager then auto-positions it at left-center.
To prevent that from happening, just remove the layout manager:
dialog.setLayout(null);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403711%2fjframe-labels-appear-overlapped%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
One obvious problem is that you are setting absolute positions of your components. Typically a LayoutManager
is used for this purpose.
To clear whatever the default layout manager of a JFrame
's content pane is, set it to null
just after creating the frame.
JFrame dialog = new JFrame();
dialog.setLayout(null);
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
add a comment |
One obvious problem is that you are setting absolute positions of your components. Typically a LayoutManager
is used for this purpose.
To clear whatever the default layout manager of a JFrame
's content pane is, set it to null
just after creating the frame.
JFrame dialog = new JFrame();
dialog.setLayout(null);
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
add a comment |
One obvious problem is that you are setting absolute positions of your components. Typically a LayoutManager
is used for this purpose.
To clear whatever the default layout manager of a JFrame
's content pane is, set it to null
just after creating the frame.
JFrame dialog = new JFrame();
dialog.setLayout(null);
One obvious problem is that you are setting absolute positions of your components. Typically a LayoutManager
is used for this purpose.
To clear whatever the default layout manager of a JFrame
's content pane is, set it to null
just after creating the frame.
JFrame dialog = new JFrame();
dialog.setLayout(null);
answered Nov 21 '18 at 1:02


Tom Hawtin - tacklineTom Hawtin - tackline
127k28183272
127k28183272
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
add a comment |
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
Thanks for your answers, i just used this method at another JFrame and i cant explain why i did not use it this time...
– Animalz
Nov 21 '18 at 1:08
add a comment |
As the javadoc of JFrame
says:
The default content pane will have a
BorderLayout
manager set on it.
Javadoc of BorderLayout
says:
A border layout lays out a container, arranging and resizing its components to fit in five regions: north, south, east, west, and center. Each region may contain no more than one component, and is identified by a corresponding constant:
NORTH
,SOUTH
,EAST
,WEST
, andCENTER
. When adding a component to a container with a border layout, use one of these five constants, for example:
Panel p = new Panel();
p.setLayout(new BorderLayout());
p.add(new Button("Okay"), BorderLayout.SOUTH);
As a convenience,
BorderLayout
interprets the absence of a string specification the same as the constantCENTER
:
Panel p2 = new Panel();
p2.setLayout(new BorderLayout());
p2.add(new TextArea()); // Same as p.add(new TextArea(), BorderLayout.CENTER);
Since you call the 1-arg version of add()
, all your JLabels are added with BorderLayout.CENTER
, and so the last one wins, and the BorderLayout
manager then auto-positions it at left-center.
To prevent that from happening, just remove the layout manager:
dialog.setLayout(null);
add a comment |
As the javadoc of JFrame
says:
The default content pane will have a
BorderLayout
manager set on it.
Javadoc of BorderLayout
says:
A border layout lays out a container, arranging and resizing its components to fit in five regions: north, south, east, west, and center. Each region may contain no more than one component, and is identified by a corresponding constant:
NORTH
,SOUTH
,EAST
,WEST
, andCENTER
. When adding a component to a container with a border layout, use one of these five constants, for example:
Panel p = new Panel();
p.setLayout(new BorderLayout());
p.add(new Button("Okay"), BorderLayout.SOUTH);
As a convenience,
BorderLayout
interprets the absence of a string specification the same as the constantCENTER
:
Panel p2 = new Panel();
p2.setLayout(new BorderLayout());
p2.add(new TextArea()); // Same as p.add(new TextArea(), BorderLayout.CENTER);
Since you call the 1-arg version of add()
, all your JLabels are added with BorderLayout.CENTER
, and so the last one wins, and the BorderLayout
manager then auto-positions it at left-center.
To prevent that from happening, just remove the layout manager:
dialog.setLayout(null);
add a comment |
As the javadoc of JFrame
says:
The default content pane will have a
BorderLayout
manager set on it.
Javadoc of BorderLayout
says:
A border layout lays out a container, arranging and resizing its components to fit in five regions: north, south, east, west, and center. Each region may contain no more than one component, and is identified by a corresponding constant:
NORTH
,SOUTH
,EAST
,WEST
, andCENTER
. When adding a component to a container with a border layout, use one of these five constants, for example:
Panel p = new Panel();
p.setLayout(new BorderLayout());
p.add(new Button("Okay"), BorderLayout.SOUTH);
As a convenience,
BorderLayout
interprets the absence of a string specification the same as the constantCENTER
:
Panel p2 = new Panel();
p2.setLayout(new BorderLayout());
p2.add(new TextArea()); // Same as p.add(new TextArea(), BorderLayout.CENTER);
Since you call the 1-arg version of add()
, all your JLabels are added with BorderLayout.CENTER
, and so the last one wins, and the BorderLayout
manager then auto-positions it at left-center.
To prevent that from happening, just remove the layout manager:
dialog.setLayout(null);
As the javadoc of JFrame
says:
The default content pane will have a
BorderLayout
manager set on it.
Javadoc of BorderLayout
says:
A border layout lays out a container, arranging and resizing its components to fit in five regions: north, south, east, west, and center. Each region may contain no more than one component, and is identified by a corresponding constant:
NORTH
,SOUTH
,EAST
,WEST
, andCENTER
. When adding a component to a container with a border layout, use one of these five constants, for example:
Panel p = new Panel();
p.setLayout(new BorderLayout());
p.add(new Button("Okay"), BorderLayout.SOUTH);
As a convenience,
BorderLayout
interprets the absence of a string specification the same as the constantCENTER
:
Panel p2 = new Panel();
p2.setLayout(new BorderLayout());
p2.add(new TextArea()); // Same as p.add(new TextArea(), BorderLayout.CENTER);
Since you call the 1-arg version of add()
, all your JLabels are added with BorderLayout.CENTER
, and so the last one wins, and the BorderLayout
manager then auto-positions it at left-center.
To prevent that from happening, just remove the layout manager:
dialog.setLayout(null);
answered Nov 21 '18 at 1:05


AndreasAndreas
77.9k464126
77.9k464126
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403711%2fjframe-labels-appear-overlapped%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kL,5yh0PGwCQ0OuZmO8dsuN,1A9yJYwWq,c7M,jqNig mLHAL,iWkVoDwLruQZ0tMJ8pupVHUCRGixyR3RPD0g2jAB