Replacing multiple value names in a panda dataframe
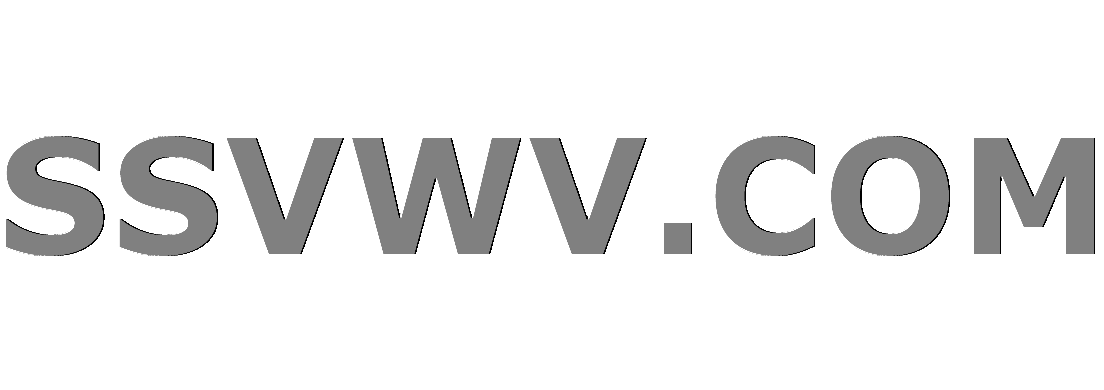
Multi tool use
I have a column colA
that has multiple values in a pandas dataframe. I want every value that starts with spare1
in this column to be replaced with the words email_petition
. e.g. spare1signed
, spare1not
signed yet' etc. will all be converted to just email_petition
.
I am using the following code:
petition = df.colA.str.startswith('spare1')
if df.colA == petition:
df.colA.replace(petition, 'email_petition', inplace=True)
but I get the following error:
The truth value of a Series is ambiguous. Use a.empty
, a.bool()
, a.item()
, a.any()
or a.all()
.
I also tried the following code which doesn't give me an error but doesn't seem to work as the values don't change:
petition = df.colA.str.startswith('spare1')
if df.colA is petition:
df.colA.replace(petition, 'email_petition', inplace=True)
would love some advice on this!
thanks
python pandas
add a comment |
I have a column colA
that has multiple values in a pandas dataframe. I want every value that starts with spare1
in this column to be replaced with the words email_petition
. e.g. spare1signed
, spare1not
signed yet' etc. will all be converted to just email_petition
.
I am using the following code:
petition = df.colA.str.startswith('spare1')
if df.colA == petition:
df.colA.replace(petition, 'email_petition', inplace=True)
but I get the following error:
The truth value of a Series is ambiguous. Use a.empty
, a.bool()
, a.item()
, a.any()
or a.all()
.
I also tried the following code which doesn't give me an error but doesn't seem to work as the values don't change:
petition = df.colA.str.startswith('spare1')
if df.colA is petition:
df.colA.replace(petition, 'email_petition', inplace=True)
would love some advice on this!
thanks
python pandas
Does my answer helped you ?
– Rahul Agarwal
Nov 22 '18 at 16:45
@.. user8322222 what i should do when someone answers my question must learn!
– pygo
Nov 23 '18 at 19:18
add a comment |
I have a column colA
that has multiple values in a pandas dataframe. I want every value that starts with spare1
in this column to be replaced with the words email_petition
. e.g. spare1signed
, spare1not
signed yet' etc. will all be converted to just email_petition
.
I am using the following code:
petition = df.colA.str.startswith('spare1')
if df.colA == petition:
df.colA.replace(petition, 'email_petition', inplace=True)
but I get the following error:
The truth value of a Series is ambiguous. Use a.empty
, a.bool()
, a.item()
, a.any()
or a.all()
.
I also tried the following code which doesn't give me an error but doesn't seem to work as the values don't change:
petition = df.colA.str.startswith('spare1')
if df.colA is petition:
df.colA.replace(petition, 'email_petition', inplace=True)
would love some advice on this!
thanks
python pandas
I have a column colA
that has multiple values in a pandas dataframe. I want every value that starts with spare1
in this column to be replaced with the words email_petition
. e.g. spare1signed
, spare1not
signed yet' etc. will all be converted to just email_petition
.
I am using the following code:
petition = df.colA.str.startswith('spare1')
if df.colA == petition:
df.colA.replace(petition, 'email_petition', inplace=True)
but I get the following error:
The truth value of a Series is ambiguous. Use a.empty
, a.bool()
, a.item()
, a.any()
or a.all()
.
I also tried the following code which doesn't give me an error but doesn't seem to work as the values don't change:
petition = df.colA.str.startswith('spare1')
if df.colA is petition:
df.colA.replace(petition, 'email_petition', inplace=True)
would love some advice on this!
thanks
python pandas
python pandas
edited Nov 22 '18 at 17:18


Ali AzG
7131717
7131717
asked Nov 22 '18 at 15:51
user8322222user8322222
1178
1178
Does my answer helped you ?
– Rahul Agarwal
Nov 22 '18 at 16:45
@.. user8322222 what i should do when someone answers my question must learn!
– pygo
Nov 23 '18 at 19:18
add a comment |
Does my answer helped you ?
– Rahul Agarwal
Nov 22 '18 at 16:45
@.. user8322222 what i should do when someone answers my question must learn!
– pygo
Nov 23 '18 at 19:18
Does my answer helped you ?
– Rahul Agarwal
Nov 22 '18 at 16:45
Does my answer helped you ?
– Rahul Agarwal
Nov 22 '18 at 16:45
@.. user8322222 what i should do when someone answers my question must learn!
– pygo
Nov 23 '18 at 19:18
@.. user8322222 what i should do when someone answers my question must learn!
– pygo
Nov 23 '18 at 19:18
add a comment |
3 Answers
3
active
oldest
votes
Try this:
df.colA.replace({'spare1':'email_petition'}, regex=True)
For complete removal:
df['colA'].replace({'spare1signed':'email_petition','spare1notsigned':'email_petition'})
add a comment |
When possible always vectorize your operations on a dataframe. In your case the for
loop is not required, you can simply apply a function to the whole column.
df = pd.DataFrame({'colA':['spare1signed','spare1not signed','no action']})
df.colA = df.colA.apply(lambda x: 'email_sent' if 'spare1' in x else x)
df
>>
colA
0 email_sent
1 email_sent
2 no action
Here we assign the column with a lambda function that replaces any value in the column if spare1
is found with email_sent
.
add a comment |
This can be simplified with replace
using regex pattern:
Borrowed the data from @BernardL
Example DataFrame with column name colA
:
>>> df
colA
0 spare1signed
1 spare1not signed
2 no action
Applying regex method which says whatever ends with signed$
Just replace them to email_sent
:
Result:
>>> df['colA'] = df.colA.replace(r'.*signed$', 'email_sent', regex=True)
>>> df
colA
0 email_sent
1 email_sent
2 no action
Regex Meaning:
.*
matches any character (except for line terminators)
*
Quantifier — Matches between zero and unlimited times, as many
times as possible, giving back as needed (greedy)
signed
matches the characters signed literally (case sensitive)
$
asserts position at the end of a line
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53434489%2freplacing-multiple-value-names-in-a-panda-dataframe%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try this:
df.colA.replace({'spare1':'email_petition'}, regex=True)
For complete removal:
df['colA'].replace({'spare1signed':'email_petition','spare1notsigned':'email_petition'})
add a comment |
Try this:
df.colA.replace({'spare1':'email_petition'}, regex=True)
For complete removal:
df['colA'].replace({'spare1signed':'email_petition','spare1notsigned':'email_petition'})
add a comment |
Try this:
df.colA.replace({'spare1':'email_petition'}, regex=True)
For complete removal:
df['colA'].replace({'spare1signed':'email_petition','spare1notsigned':'email_petition'})
Try this:
df.colA.replace({'spare1':'email_petition'}, regex=True)
For complete removal:
df['colA'].replace({'spare1signed':'email_petition','spare1notsigned':'email_petition'})
answered Nov 22 '18 at 15:55


Rahul AgarwalRahul Agarwal
2,32851129
2,32851129
add a comment |
add a comment |
When possible always vectorize your operations on a dataframe. In your case the for
loop is not required, you can simply apply a function to the whole column.
df = pd.DataFrame({'colA':['spare1signed','spare1not signed','no action']})
df.colA = df.colA.apply(lambda x: 'email_sent' if 'spare1' in x else x)
df
>>
colA
0 email_sent
1 email_sent
2 no action
Here we assign the column with a lambda function that replaces any value in the column if spare1
is found with email_sent
.
add a comment |
When possible always vectorize your operations on a dataframe. In your case the for
loop is not required, you can simply apply a function to the whole column.
df = pd.DataFrame({'colA':['spare1signed','spare1not signed','no action']})
df.colA = df.colA.apply(lambda x: 'email_sent' if 'spare1' in x else x)
df
>>
colA
0 email_sent
1 email_sent
2 no action
Here we assign the column with a lambda function that replaces any value in the column if spare1
is found with email_sent
.
add a comment |
When possible always vectorize your operations on a dataframe. In your case the for
loop is not required, you can simply apply a function to the whole column.
df = pd.DataFrame({'colA':['spare1signed','spare1not signed','no action']})
df.colA = df.colA.apply(lambda x: 'email_sent' if 'spare1' in x else x)
df
>>
colA
0 email_sent
1 email_sent
2 no action
Here we assign the column with a lambda function that replaces any value in the column if spare1
is found with email_sent
.
When possible always vectorize your operations on a dataframe. In your case the for
loop is not required, you can simply apply a function to the whole column.
df = pd.DataFrame({'colA':['spare1signed','spare1not signed','no action']})
df.colA = df.colA.apply(lambda x: 'email_sent' if 'spare1' in x else x)
df
>>
colA
0 email_sent
1 email_sent
2 no action
Here we assign the column with a lambda function that replaces any value in the column if spare1
is found with email_sent
.
answered Nov 22 '18 at 16:16


BernardLBernardL
2,40811130
2,40811130
add a comment |
add a comment |
This can be simplified with replace
using regex pattern:
Borrowed the data from @BernardL
Example DataFrame with column name colA
:
>>> df
colA
0 spare1signed
1 spare1not signed
2 no action
Applying regex method which says whatever ends with signed$
Just replace them to email_sent
:
Result:
>>> df['colA'] = df.colA.replace(r'.*signed$', 'email_sent', regex=True)
>>> df
colA
0 email_sent
1 email_sent
2 no action
Regex Meaning:
.*
matches any character (except for line terminators)
*
Quantifier — Matches between zero and unlimited times, as many
times as possible, giving back as needed (greedy)
signed
matches the characters signed literally (case sensitive)
$
asserts position at the end of a line
add a comment |
This can be simplified with replace
using regex pattern:
Borrowed the data from @BernardL
Example DataFrame with column name colA
:
>>> df
colA
0 spare1signed
1 spare1not signed
2 no action
Applying regex method which says whatever ends with signed$
Just replace them to email_sent
:
Result:
>>> df['colA'] = df.colA.replace(r'.*signed$', 'email_sent', regex=True)
>>> df
colA
0 email_sent
1 email_sent
2 no action
Regex Meaning:
.*
matches any character (except for line terminators)
*
Quantifier — Matches between zero and unlimited times, as many
times as possible, giving back as needed (greedy)
signed
matches the characters signed literally (case sensitive)
$
asserts position at the end of a line
add a comment |
This can be simplified with replace
using regex pattern:
Borrowed the data from @BernardL
Example DataFrame with column name colA
:
>>> df
colA
0 spare1signed
1 spare1not signed
2 no action
Applying regex method which says whatever ends with signed$
Just replace them to email_sent
:
Result:
>>> df['colA'] = df.colA.replace(r'.*signed$', 'email_sent', regex=True)
>>> df
colA
0 email_sent
1 email_sent
2 no action
Regex Meaning:
.*
matches any character (except for line terminators)
*
Quantifier — Matches between zero and unlimited times, as many
times as possible, giving back as needed (greedy)
signed
matches the characters signed literally (case sensitive)
$
asserts position at the end of a line
This can be simplified with replace
using regex pattern:
Borrowed the data from @BernardL
Example DataFrame with column name colA
:
>>> df
colA
0 spare1signed
1 spare1not signed
2 no action
Applying regex method which says whatever ends with signed$
Just replace them to email_sent
:
Result:
>>> df['colA'] = df.colA.replace(r'.*signed$', 'email_sent', regex=True)
>>> df
colA
0 email_sent
1 email_sent
2 no action
Regex Meaning:
.*
matches any character (except for line terminators)
*
Quantifier — Matches between zero and unlimited times, as many
times as possible, giving back as needed (greedy)
signed
matches the characters signed literally (case sensitive)
$
asserts position at the end of a line
answered Nov 22 '18 at 18:16


pygopygo
3,1961721
3,1961721
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53434489%2freplacing-multiple-value-names-in-a-panda-dataframe%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0YUpyN2qn
Does my answer helped you ?
– Rahul Agarwal
Nov 22 '18 at 16:45
@.. user8322222 what i should do when someone answers my question must learn!
– pygo
Nov 23 '18 at 19:18