ActiveStorage and direct uploads won't work in development
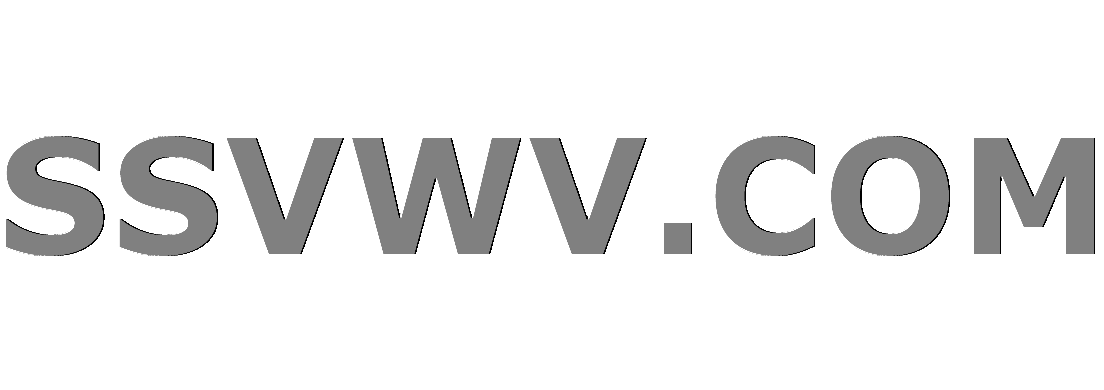
Multi tool use
up vote
0
down vote
favorite
I'm experimenting with ActiveStorage, because I want to create a Rails app where the user can upload directly to DigitalOcean spaces.
However, I have already run into a CSRF problem.
I have declared digital ocean in my storage.yml:
do:
service: S3
access_key_id: this_is_a_secret
secret_access_key: this_is_also_a_secret
region: ams3
bucket: this_is_also_a_secret
endpoint: 'https://this_is_also_a_secret.ams3.digitaloceanspaces.com'
I have chosen that storage service in my development.rb
config.active_storage.service = :do
and I have added the aws gem to Gemfile
gem "aws-sdk-s3", require: false
I have required activestorage
in my javascript bundle.
and I have added the following to my view for testing:
= form_for(VideoUpload.first, url: "/") do |form|
div = form.file_field :file, direct_upload: true
div = form.submit("Send that file")
When I submit the form, the activestorage.js library kicks in. It calls http://127.0.0.1:3000/rails/active_storage/direct_uploads
which in turns throws a 422 due to a missing CSRF token. I have inspected the request and the controller is not wrong. There is no CSRF token set in the HTTP headers, X-CSRF-Token
is oddly set to undefined
.
The request body contains: {"blob":{"filename":"9T6qqsA-png-wallpapers.jpg","content_type":"image/jpeg","byte_size":122990,"checksum":"gqU9VBgS9mmH7s45qHIbug=="}}
, so no CSRF token here either.
But the form in which the input file element is specified contains a token, so I would assume that activestorage.js
would pick it up.
What am I missing here? The documentation at https://edgeguides.rubyonrails.org/active_storage_overview.html#direct-uploads
doesn't imply that any special configuration in the JS side is necessary.
I am using Rails 5.2.1 and Ruby 2.5.1
ruby-on-rails rails-activestorage
add a comment |
up vote
0
down vote
favorite
I'm experimenting with ActiveStorage, because I want to create a Rails app where the user can upload directly to DigitalOcean spaces.
However, I have already run into a CSRF problem.
I have declared digital ocean in my storage.yml:
do:
service: S3
access_key_id: this_is_a_secret
secret_access_key: this_is_also_a_secret
region: ams3
bucket: this_is_also_a_secret
endpoint: 'https://this_is_also_a_secret.ams3.digitaloceanspaces.com'
I have chosen that storage service in my development.rb
config.active_storage.service = :do
and I have added the aws gem to Gemfile
gem "aws-sdk-s3", require: false
I have required activestorage
in my javascript bundle.
and I have added the following to my view for testing:
= form_for(VideoUpload.first, url: "/") do |form|
div = form.file_field :file, direct_upload: true
div = form.submit("Send that file")
When I submit the form, the activestorage.js library kicks in. It calls http://127.0.0.1:3000/rails/active_storage/direct_uploads
which in turns throws a 422 due to a missing CSRF token. I have inspected the request and the controller is not wrong. There is no CSRF token set in the HTTP headers, X-CSRF-Token
is oddly set to undefined
.
The request body contains: {"blob":{"filename":"9T6qqsA-png-wallpapers.jpg","content_type":"image/jpeg","byte_size":122990,"checksum":"gqU9VBgS9mmH7s45qHIbug=="}}
, so no CSRF token here either.
But the form in which the input file element is specified contains a token, so I would assume that activestorage.js
would pick it up.
What am I missing here? The documentation at https://edgeguides.rubyonrails.org/active_storage_overview.html#direct-uploads
doesn't imply that any special configuration in the JS side is necessary.
I am using Rails 5.2.1 and Ruby 2.5.1
ruby-on-rails rails-activestorage
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm experimenting with ActiveStorage, because I want to create a Rails app where the user can upload directly to DigitalOcean spaces.
However, I have already run into a CSRF problem.
I have declared digital ocean in my storage.yml:
do:
service: S3
access_key_id: this_is_a_secret
secret_access_key: this_is_also_a_secret
region: ams3
bucket: this_is_also_a_secret
endpoint: 'https://this_is_also_a_secret.ams3.digitaloceanspaces.com'
I have chosen that storage service in my development.rb
config.active_storage.service = :do
and I have added the aws gem to Gemfile
gem "aws-sdk-s3", require: false
I have required activestorage
in my javascript bundle.
and I have added the following to my view for testing:
= form_for(VideoUpload.first, url: "/") do |form|
div = form.file_field :file, direct_upload: true
div = form.submit("Send that file")
When I submit the form, the activestorage.js library kicks in. It calls http://127.0.0.1:3000/rails/active_storage/direct_uploads
which in turns throws a 422 due to a missing CSRF token. I have inspected the request and the controller is not wrong. There is no CSRF token set in the HTTP headers, X-CSRF-Token
is oddly set to undefined
.
The request body contains: {"blob":{"filename":"9T6qqsA-png-wallpapers.jpg","content_type":"image/jpeg","byte_size":122990,"checksum":"gqU9VBgS9mmH7s45qHIbug=="}}
, so no CSRF token here either.
But the form in which the input file element is specified contains a token, so I would assume that activestorage.js
would pick it up.
What am I missing here? The documentation at https://edgeguides.rubyonrails.org/active_storage_overview.html#direct-uploads
doesn't imply that any special configuration in the JS side is necessary.
I am using Rails 5.2.1 and Ruby 2.5.1
ruby-on-rails rails-activestorage
I'm experimenting with ActiveStorage, because I want to create a Rails app where the user can upload directly to DigitalOcean spaces.
However, I have already run into a CSRF problem.
I have declared digital ocean in my storage.yml:
do:
service: S3
access_key_id: this_is_a_secret
secret_access_key: this_is_also_a_secret
region: ams3
bucket: this_is_also_a_secret
endpoint: 'https://this_is_also_a_secret.ams3.digitaloceanspaces.com'
I have chosen that storage service in my development.rb
config.active_storage.service = :do
and I have added the aws gem to Gemfile
gem "aws-sdk-s3", require: false
I have required activestorage
in my javascript bundle.
and I have added the following to my view for testing:
= form_for(VideoUpload.first, url: "/") do |form|
div = form.file_field :file, direct_upload: true
div = form.submit("Send that file")
When I submit the form, the activestorage.js library kicks in. It calls http://127.0.0.1:3000/rails/active_storage/direct_uploads
which in turns throws a 422 due to a missing CSRF token. I have inspected the request and the controller is not wrong. There is no CSRF token set in the HTTP headers, X-CSRF-Token
is oddly set to undefined
.
The request body contains: {"blob":{"filename":"9T6qqsA-png-wallpapers.jpg","content_type":"image/jpeg","byte_size":122990,"checksum":"gqU9VBgS9mmH7s45qHIbug=="}}
, so no CSRF token here either.
But the form in which the input file element is specified contains a token, so I would assume that activestorage.js
would pick it up.
What am I missing here? The documentation at https://edgeguides.rubyonrails.org/active_storage_overview.html#direct-uploads
doesn't imply that any special configuration in the JS side is necessary.
I am using Rails 5.2.1 and Ruby 2.5.1
ruby-on-rails rails-activestorage
ruby-on-rails rails-activestorage
asked Nov 7 at 9:16
Niels B.
3,38111230
3,38111230
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53186471%2factivestorage-and-direct-uploads-wont-work-in-development%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bAR0BX vjf,B VIXZwHtLA7aN,9ouv43IV8AEOA64lwFs9FRVsoJ52Yy p