ListCreateAPIView POST with an annotated field that's in serializer not model
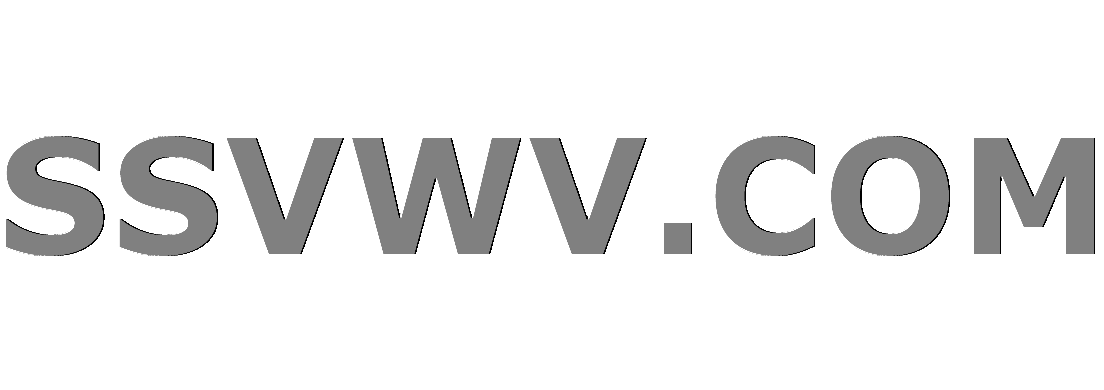
Multi tool use
up vote
0
down vote
favorite
I'm building a simple budgeting app to work on learning Django & React. I've used DRF to build an API to create and get transactions from the database. I'm currently calculating the total running balance on the fly when I do my GET. This has been working well, but when I do a POST I get an error that my dynamic balance field is required since that field is in my serializer. How can I get around this?
views.py
class CreateView(generics.ListCreateAPIView):
"""This class defines the GET & POST behavior of the rest api."""
queryset = Transaction.objects.all()
# This is the balance that's calculated on the fly
queryset_with_balance = queryset.annotate(balance=Window(Sum('amount'),
order_by=F('created_time').asc())).all().order_by('-created_time')
serializer_class = TransactionSerializer
def perform_create(self, serializer):
"""Save the post data when creating a new transaction."""
serializer.save()
def get_queryset(self):
return self.queryset_with_balance
serializers.py
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19)
class Meta:
"""Meta class to map serializer's fields with the model fields."""
model = Transaction
fields = ('id', 'date', 'payee', 'category',
'amount', 'balance', 'created_time', 'modified_time')
models.py
class Transaction(models.Model):
date = models.DateField()
payee = models.CharField(max_length=256)
category = models.CharField(max_length=256)
amount = MoneyField(max_digits=19,
decimal_places=2,
default_currency='USD')
created_time = models.DateTimeField(auto_now_add=True)
modified_time = models.DateTimeField(auto_now=True)
django django-rest-framework
add a comment |
up vote
0
down vote
favorite
I'm building a simple budgeting app to work on learning Django & React. I've used DRF to build an API to create and get transactions from the database. I'm currently calculating the total running balance on the fly when I do my GET. This has been working well, but when I do a POST I get an error that my dynamic balance field is required since that field is in my serializer. How can I get around this?
views.py
class CreateView(generics.ListCreateAPIView):
"""This class defines the GET & POST behavior of the rest api."""
queryset = Transaction.objects.all()
# This is the balance that's calculated on the fly
queryset_with_balance = queryset.annotate(balance=Window(Sum('amount'),
order_by=F('created_time').asc())).all().order_by('-created_time')
serializer_class = TransactionSerializer
def perform_create(self, serializer):
"""Save the post data when creating a new transaction."""
serializer.save()
def get_queryset(self):
return self.queryset_with_balance
serializers.py
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19)
class Meta:
"""Meta class to map serializer's fields with the model fields."""
model = Transaction
fields = ('id', 'date', 'payee', 'category',
'amount', 'balance', 'created_time', 'modified_time')
models.py
class Transaction(models.Model):
date = models.DateField()
payee = models.CharField(max_length=256)
category = models.CharField(max_length=256)
amount = MoneyField(max_digits=19,
decimal_places=2,
default_currency='USD')
created_time = models.DateTimeField(auto_now_add=True)
modified_time = models.DateTimeField(auto_now=True)
django django-rest-framework
Do you need thisbalance
field whileHTTP POST
?
– JPG
Nov 5 at 2:49
you can move queryset_with_balance or only annotate todef get_queryset(self):
– Ngoc Pham
Nov 5 at 2:50
Balance is only needed on the GET. I'm not storing that in the DB.
– brewcrazy
Nov 5 at 2:53
@brewcrazy Check my answer below :) Hope that will solve the issue :)
– JPG
Nov 5 at 2:58
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm building a simple budgeting app to work on learning Django & React. I've used DRF to build an API to create and get transactions from the database. I'm currently calculating the total running balance on the fly when I do my GET. This has been working well, but when I do a POST I get an error that my dynamic balance field is required since that field is in my serializer. How can I get around this?
views.py
class CreateView(generics.ListCreateAPIView):
"""This class defines the GET & POST behavior of the rest api."""
queryset = Transaction.objects.all()
# This is the balance that's calculated on the fly
queryset_with_balance = queryset.annotate(balance=Window(Sum('amount'),
order_by=F('created_time').asc())).all().order_by('-created_time')
serializer_class = TransactionSerializer
def perform_create(self, serializer):
"""Save the post data when creating a new transaction."""
serializer.save()
def get_queryset(self):
return self.queryset_with_balance
serializers.py
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19)
class Meta:
"""Meta class to map serializer's fields with the model fields."""
model = Transaction
fields = ('id', 'date', 'payee', 'category',
'amount', 'balance', 'created_time', 'modified_time')
models.py
class Transaction(models.Model):
date = models.DateField()
payee = models.CharField(max_length=256)
category = models.CharField(max_length=256)
amount = MoneyField(max_digits=19,
decimal_places=2,
default_currency='USD')
created_time = models.DateTimeField(auto_now_add=True)
modified_time = models.DateTimeField(auto_now=True)
django django-rest-framework
I'm building a simple budgeting app to work on learning Django & React. I've used DRF to build an API to create and get transactions from the database. I'm currently calculating the total running balance on the fly when I do my GET. This has been working well, but when I do a POST I get an error that my dynamic balance field is required since that field is in my serializer. How can I get around this?
views.py
class CreateView(generics.ListCreateAPIView):
"""This class defines the GET & POST behavior of the rest api."""
queryset = Transaction.objects.all()
# This is the balance that's calculated on the fly
queryset_with_balance = queryset.annotate(balance=Window(Sum('amount'),
order_by=F('created_time').asc())).all().order_by('-created_time')
serializer_class = TransactionSerializer
def perform_create(self, serializer):
"""Save the post data when creating a new transaction."""
serializer.save()
def get_queryset(self):
return self.queryset_with_balance
serializers.py
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19)
class Meta:
"""Meta class to map serializer's fields with the model fields."""
model = Transaction
fields = ('id', 'date', 'payee', 'category',
'amount', 'balance', 'created_time', 'modified_time')
models.py
class Transaction(models.Model):
date = models.DateField()
payee = models.CharField(max_length=256)
category = models.CharField(max_length=256)
amount = MoneyField(max_digits=19,
decimal_places=2,
default_currency='USD')
created_time = models.DateTimeField(auto_now_add=True)
modified_time = models.DateTimeField(auto_now=True)
django django-rest-framework
django django-rest-framework
edited Nov 5 at 2:50


eyllanesc
66.2k82952
66.2k82952
asked Nov 5 at 2:44
brewcrazy
8911
8911
Do you need thisbalance
field whileHTTP POST
?
– JPG
Nov 5 at 2:49
you can move queryset_with_balance or only annotate todef get_queryset(self):
– Ngoc Pham
Nov 5 at 2:50
Balance is only needed on the GET. I'm not storing that in the DB.
– brewcrazy
Nov 5 at 2:53
@brewcrazy Check my answer below :) Hope that will solve the issue :)
– JPG
Nov 5 at 2:58
add a comment |
Do you need thisbalance
field whileHTTP POST
?
– JPG
Nov 5 at 2:49
you can move queryset_with_balance or only annotate todef get_queryset(self):
– Ngoc Pham
Nov 5 at 2:50
Balance is only needed on the GET. I'm not storing that in the DB.
– brewcrazy
Nov 5 at 2:53
@brewcrazy Check my answer below :) Hope that will solve the issue :)
– JPG
Nov 5 at 2:58
Do you need this
balance
field while HTTP POST
?– JPG
Nov 5 at 2:49
Do you need this
balance
field while HTTP POST
?– JPG
Nov 5 at 2:49
you can move queryset_with_balance or only annotate to
def get_queryset(self):
– Ngoc Pham
Nov 5 at 2:50
you can move queryset_with_balance or only annotate to
def get_queryset(self):
– Ngoc Pham
Nov 5 at 2:50
Balance is only needed on the GET. I'm not storing that in the DB.
– brewcrazy
Nov 5 at 2:53
Balance is only needed on the GET. I'm not storing that in the DB.
– brewcrazy
Nov 5 at 2:53
@brewcrazy Check my answer below :) Hope that will solve the issue :)
– JPG
Nov 5 at 2:58
@brewcrazy Check my answer below :) Hope that will solve the issue :)
– JPG
Nov 5 at 2:58
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
set balance
field as read_only
as below,
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19, read_only=True)
# your code
From the DRF doc
Read-only fields are included in the API output, but should not be included in the input during create or update operations.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
set balance
field as read_only
as below,
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19, read_only=True)
# your code
From the DRF doc
Read-only fields are included in the API output, but should not be included in the input during create or update operations.
add a comment |
up vote
1
down vote
accepted
set balance
field as read_only
as below,
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19, read_only=True)
# your code
From the DRF doc
Read-only fields are included in the API output, but should not be included in the input during create or update operations.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
set balance
field as read_only
as below,
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19, read_only=True)
# your code
From the DRF doc
Read-only fields are included in the API output, but should not be included in the input during create or update operations.
set balance
field as read_only
as below,
class TransactionSerializer(serializers.ModelSerializer):
balance = serializers.DecimalField(decimal_places=2, max_digits=19, read_only=True)
# your code
From the DRF doc
Read-only fields are included in the API output, but should not be included in the input during create or update operations.
answered Nov 5 at 2:55


JPG
11.8k2829
11.8k2829
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53147657%2flistcreateapiview-post-with-an-annotated-field-thats-in-serializer-not-model%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
f58 xRA9jI,k9bNSdgyhFLsXJIZ5AvNnhX BMa47Ul1GNCGvSNaU FBJpwfA,DmSVofE4qp8Mtv,Gf,yY
Do you need this
balance
field whileHTTP POST
?– JPG
Nov 5 at 2:49
you can move queryset_with_balance or only annotate to
def get_queryset(self):
– Ngoc Pham
Nov 5 at 2:50
Balance is only needed on the GET. I'm not storing that in the DB.
– brewcrazy
Nov 5 at 2:53
@brewcrazy Check my answer below :) Hope that will solve the issue :)
– JPG
Nov 5 at 2:58