ASP.NET Core 2.1 - Authentication cookie deleted but the user could still login without being redirected to...
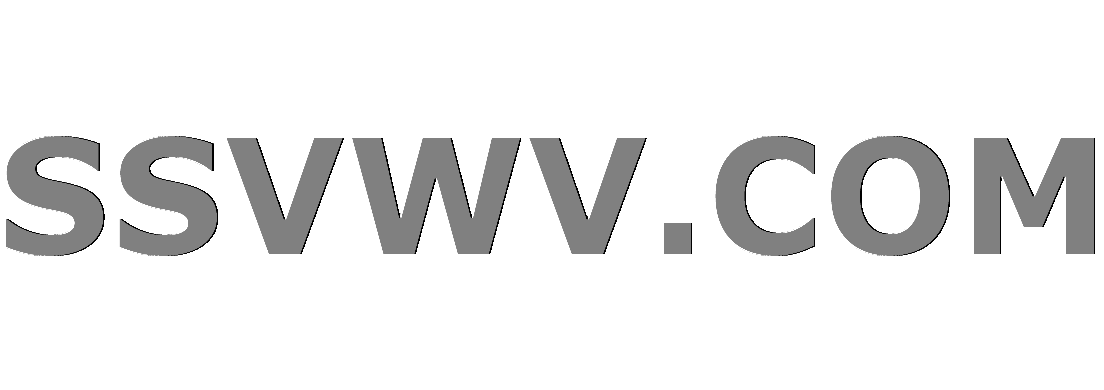
Multi tool use
up vote
0
down vote
favorite
Good day! I am currently creating a website which utilises the Google authentication to enable content personalisation. I have no problem with sign-in and retrieving the logged in user's info, but .NET is not signing the user out completely when I call the SignOutAsync() function, as the user could immediately log in when clicking on the Login button again. Once I clear the browser cache, the user will be redirected to the Google sign-in page when clicking on the Login button.
The services configuration at Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
// Configure authentication service
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "Google";
})
.AddCookie("Cookies")
.AddGoogle("Google", options =>
{
options.ClientId = Configuration["Authentication:Google:ClientId"];
options.ClientSecret = Configuration["Authentication:Google:ClientSecret"];
});
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddSingleton<IRecommender, OntologyRecommender>();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
}
The middleware configuration at Startup.cs:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
Login action at the UserController.cs:
public IActionResult Login()
{
return Challenge(new AuthenticationProperties() { RedirectUri = "/" });
}
Logout action at the UserController.cs:
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return RedirectToAction("Index", "Home");
}
I am new to the ASP.NET Core authentication area, so I would appreciate if anyone could just assist me on this matter, thank you!
c# authentication asp.net-core asp.net-core-mvc google-authentication
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
Good day! I am currently creating a website which utilises the Google authentication to enable content personalisation. I have no problem with sign-in and retrieving the logged in user's info, but .NET is not signing the user out completely when I call the SignOutAsync() function, as the user could immediately log in when clicking on the Login button again. Once I clear the browser cache, the user will be redirected to the Google sign-in page when clicking on the Login button.
The services configuration at Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
// Configure authentication service
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "Google";
})
.AddCookie("Cookies")
.AddGoogle("Google", options =>
{
options.ClientId = Configuration["Authentication:Google:ClientId"];
options.ClientSecret = Configuration["Authentication:Google:ClientSecret"];
});
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddSingleton<IRecommender, OntologyRecommender>();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
}
The middleware configuration at Startup.cs:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
Login action at the UserController.cs:
public IActionResult Login()
{
return Challenge(new AuthenticationProperties() { RedirectUri = "/" });
}
Logout action at the UserController.cs:
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return RedirectToAction("Index", "Home");
}
I am new to the ASP.NET Core authentication area, so I would appreciate if anyone could just assist me on this matter, thank you!
c# authentication asp.net-core asp.net-core-mvc google-authentication
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
2
possible duplicate: stackoverflow.com/questions/33083824/…
– JohnB
Nov 5 at 3:45
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Good day! I am currently creating a website which utilises the Google authentication to enable content personalisation. I have no problem with sign-in and retrieving the logged in user's info, but .NET is not signing the user out completely when I call the SignOutAsync() function, as the user could immediately log in when clicking on the Login button again. Once I clear the browser cache, the user will be redirected to the Google sign-in page when clicking on the Login button.
The services configuration at Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
// Configure authentication service
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "Google";
})
.AddCookie("Cookies")
.AddGoogle("Google", options =>
{
options.ClientId = Configuration["Authentication:Google:ClientId"];
options.ClientSecret = Configuration["Authentication:Google:ClientSecret"];
});
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddSingleton<IRecommender, OntologyRecommender>();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
}
The middleware configuration at Startup.cs:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
Login action at the UserController.cs:
public IActionResult Login()
{
return Challenge(new AuthenticationProperties() { RedirectUri = "/" });
}
Logout action at the UserController.cs:
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return RedirectToAction("Index", "Home");
}
I am new to the ASP.NET Core authentication area, so I would appreciate if anyone could just assist me on this matter, thank you!
c# authentication asp.net-core asp.net-core-mvc google-authentication
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Good day! I am currently creating a website which utilises the Google authentication to enable content personalisation. I have no problem with sign-in and retrieving the logged in user's info, but .NET is not signing the user out completely when I call the SignOutAsync() function, as the user could immediately log in when clicking on the Login button again. Once I clear the browser cache, the user will be redirected to the Google sign-in page when clicking on the Login button.
The services configuration at Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
// Configure authentication service
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = "Google";
})
.AddCookie("Cookies")
.AddGoogle("Google", options =>
{
options.ClientId = Configuration["Authentication:Google:ClientId"];
options.ClientSecret = Configuration["Authentication:Google:ClientSecret"];
});
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddSingleton<IRecommender, OntologyRecommender>();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
}
The middleware configuration at Startup.cs:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
Login action at the UserController.cs:
public IActionResult Login()
{
return Challenge(new AuthenticationProperties() { RedirectUri = "/" });
}
Logout action at the UserController.cs:
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return RedirectToAction("Index", "Home");
}
I am new to the ASP.NET Core authentication area, so I would appreciate if anyone could just assist me on this matter, thank you!
c# authentication asp.net-core asp.net-core-mvc google-authentication
c# authentication asp.net-core asp.net-core-mvc google-authentication
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Nov 5 at 13:51


JohnB
880715
880715
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 5 at 3:38


Samuel Cheah
11
11
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Samuel Cheah is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
2
possible duplicate: stackoverflow.com/questions/33083824/…
– JohnB
Nov 5 at 3:45
add a comment |
2
possible duplicate: stackoverflow.com/questions/33083824/…
– JohnB
Nov 5 at 3:45
2
2
possible duplicate: stackoverflow.com/questions/33083824/…
– JohnB
Nov 5 at 3:45
possible duplicate: stackoverflow.com/questions/33083824/…
– JohnB
Nov 5 at 3:45
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
you need to loop thru the application cookies - here is a sample code snippet:
if (HttpContext.Request.Cookies[".MyCookie"] != null)
{
var siteCookies = HttpContext.Request.Cookies.Where(c => c.Key.StartsWith(".MyCookie"));
foreach (var cookie in siteCookies)
{
Response.Cookies.Delete(cookie.Key);
}
}
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
add a comment |
up vote
1
down vote
You can redirect user to Google's logout endpoint to logout :
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return Redirect("https://www.google.com/accounts/Logout?continue=https://appengine.google.com/_ah/logout?continue=https://localhost:44310");
Replace "https://localhost:44310" with your own website url . After that , when user click login again , user will be redirected to the Google sign-in page .
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
you need to loop thru the application cookies - here is a sample code snippet:
if (HttpContext.Request.Cookies[".MyCookie"] != null)
{
var siteCookies = HttpContext.Request.Cookies.Where(c => c.Key.StartsWith(".MyCookie"));
foreach (var cookie in siteCookies)
{
Response.Cookies.Delete(cookie.Key);
}
}
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
add a comment |
up vote
1
down vote
you need to loop thru the application cookies - here is a sample code snippet:
if (HttpContext.Request.Cookies[".MyCookie"] != null)
{
var siteCookies = HttpContext.Request.Cookies.Where(c => c.Key.StartsWith(".MyCookie"));
foreach (var cookie in siteCookies)
{
Response.Cookies.Delete(cookie.Key);
}
}
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
add a comment |
up vote
1
down vote
up vote
1
down vote
you need to loop thru the application cookies - here is a sample code snippet:
if (HttpContext.Request.Cookies[".MyCookie"] != null)
{
var siteCookies = HttpContext.Request.Cookies.Where(c => c.Key.StartsWith(".MyCookie"));
foreach (var cookie in siteCookies)
{
Response.Cookies.Delete(cookie.Key);
}
}
you need to loop thru the application cookies - here is a sample code snippet:
if (HttpContext.Request.Cookies[".MyCookie"] != null)
{
var siteCookies = HttpContext.Request.Cookies.Where(c => c.Key.StartsWith(".MyCookie"));
foreach (var cookie in siteCookies)
{
Response.Cookies.Delete(cookie.Key);
}
}
answered Nov 5 at 3:57


JohnB
880715
880715
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
add a comment |
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
Thanks for your prompt response. I looped through the application cookies and deleted them as suggested; however, it seems like the browser is still keeping the cookies that allow the user to immediately sign in without being redirected to the Google sign-in page.
– Samuel Cheah
Nov 5 at 4:24
add a comment |
up vote
1
down vote
You can redirect user to Google's logout endpoint to logout :
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return Redirect("https://www.google.com/accounts/Logout?continue=https://appengine.google.com/_ah/logout?continue=https://localhost:44310");
Replace "https://localhost:44310" with your own website url . After that , when user click login again , user will be redirected to the Google sign-in page .
add a comment |
up vote
1
down vote
You can redirect user to Google's logout endpoint to logout :
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return Redirect("https://www.google.com/accounts/Logout?continue=https://appengine.google.com/_ah/logout?continue=https://localhost:44310");
Replace "https://localhost:44310" with your own website url . After that , when user click login again , user will be redirected to the Google sign-in page .
add a comment |
up vote
1
down vote
up vote
1
down vote
You can redirect user to Google's logout endpoint to logout :
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return Redirect("https://www.google.com/accounts/Logout?continue=https://appengine.google.com/_ah/logout?continue=https://localhost:44310");
Replace "https://localhost:44310" with your own website url . After that , when user click login again , user will be redirected to the Google sign-in page .
You can redirect user to Google's logout endpoint to logout :
await HttpContext.SignOutAsync();
HttpContext.Response.Cookies.Delete(".AspNetCore.Cookies");
return Redirect("https://www.google.com/accounts/Logout?continue=https://appengine.google.com/_ah/logout?continue=https://localhost:44310");
Replace "https://localhost:44310" with your own website url . After that , when user click login again , user will be redirected to the Google sign-in page .
answered Nov 6 at 6:04


Nan Yu
5,6252646
5,6252646
add a comment |
add a comment |
Samuel Cheah is a new contributor. Be nice, and check out our Code of Conduct.
Samuel Cheah is a new contributor. Be nice, and check out our Code of Conduct.
Samuel Cheah is a new contributor. Be nice, and check out our Code of Conduct.
Samuel Cheah is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53147993%2fasp-net-core-2-1-authentication-cookie-deleted-but-the-user-could-still-login%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
0JBc76Fnt EkW7 sESgDIRX4 AFHzIgDE u5B 2 luqgX MuAq9
2
possible duplicate: stackoverflow.com/questions/33083824/…
– JohnB
Nov 5 at 3:45