C# Static cast char to string
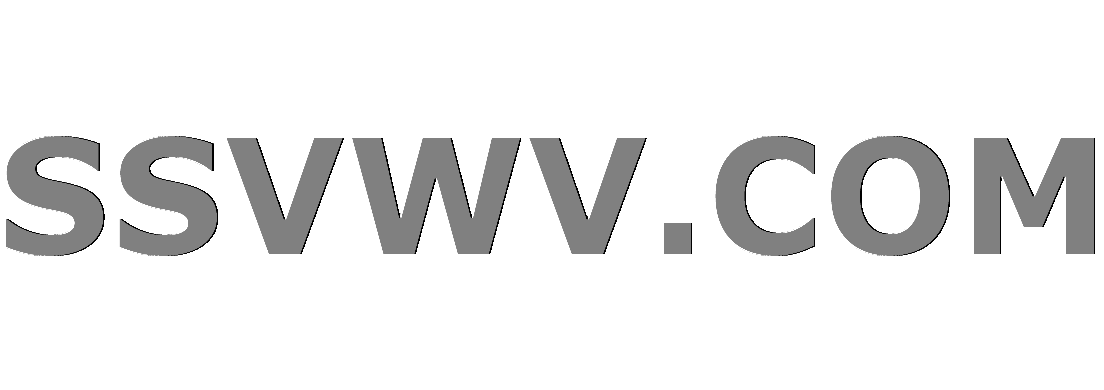
Multi tool use
I am trying to declare a constant char to hold a key and a constant string to hold a message telling the user to press the key:
...
private const KEY = 'r';
private const string MSG = "Press " + KEY + " to restart.";
...
I need to explicitly cast the key char to a string since the implicit cast is done during runtime. However, I can't figure out the way to cast a character to a string at compile time. I've seen ToString () on the internet, but it's performed at runtime and therefore doesn't work. I have the key char as a separate variable because it is used several times in the program. Does anybody know how to statically cast a char to a string?
c# string type-conversion character string-concatenation
add a comment |
I am trying to declare a constant char to hold a key and a constant string to hold a message telling the user to press the key:
...
private const KEY = 'r';
private const string MSG = "Press " + KEY + " to restart.";
...
I need to explicitly cast the key char to a string since the implicit cast is done during runtime. However, I can't figure out the way to cast a character to a string at compile time. I've seen ToString () on the internet, but it's performed at runtime and therefore doesn't work. I have the key char as a separate variable because it is used several times in the program. Does anybody know how to statically cast a char to a string?
c# string type-conversion character string-concatenation
add a comment |
I am trying to declare a constant char to hold a key and a constant string to hold a message telling the user to press the key:
...
private const KEY = 'r';
private const string MSG = "Press " + KEY + " to restart.";
...
I need to explicitly cast the key char to a string since the implicit cast is done during runtime. However, I can't figure out the way to cast a character to a string at compile time. I've seen ToString () on the internet, but it's performed at runtime and therefore doesn't work. I have the key char as a separate variable because it is used several times in the program. Does anybody know how to statically cast a char to a string?
c# string type-conversion character string-concatenation
I am trying to declare a constant char to hold a key and a constant string to hold a message telling the user to press the key:
...
private const KEY = 'r';
private const string MSG = "Press " + KEY + " to restart.";
...
I need to explicitly cast the key char to a string since the implicit cast is done during runtime. However, I can't figure out the way to cast a character to a string at compile time. I've seen ToString () on the internet, but it's performed at runtime and therefore doesn't work. I have the key char as a separate variable because it is used several times in the program. Does anybody know how to statically cast a char to a string?
c# string type-conversion character string-concatenation
c# string type-conversion character string-concatenation
asked Nov 11 at 2:30
Andrew Pratt
254
254
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The short answer is that you can't compose a string
with anything other than string
fragments.
You have a few options though. You can make both constants string
types:
private const string KEY = "r";
private const string MSG = "Press " + KEY + " to restart.";
Another option would be to compose the string
at runtime:
private const char KEY = 'r';
private static string MSG => "Press " + KEY + " to restart.";
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
add a comment |
You can't const string
with other values which is const
.
You can try to use readonly
.
readonly
can only modify values in the class constructor method, it will set that value at runtime.
private const char KEY = 'r';
private readonly string MSG = "Press " + KEY + " to restart.";
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245343%2fc-sharp-static-cast-char-to-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The short answer is that you can't compose a string
with anything other than string
fragments.
You have a few options though. You can make both constants string
types:
private const string KEY = "r";
private const string MSG = "Press " + KEY + " to restart.";
Another option would be to compose the string
at runtime:
private const char KEY = 'r';
private static string MSG => "Press " + KEY + " to restart.";
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
add a comment |
The short answer is that you can't compose a string
with anything other than string
fragments.
You have a few options though. You can make both constants string
types:
private const string KEY = "r";
private const string MSG = "Press " + KEY + " to restart.";
Another option would be to compose the string
at runtime:
private const char KEY = 'r';
private static string MSG => "Press " + KEY + " to restart.";
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
add a comment |
The short answer is that you can't compose a string
with anything other than string
fragments.
You have a few options though. You can make both constants string
types:
private const string KEY = "r";
private const string MSG = "Press " + KEY + " to restart.";
Another option would be to compose the string
at runtime:
private const char KEY = 'r';
private static string MSG => "Press " + KEY + " to restart.";
The short answer is that you can't compose a string
with anything other than string
fragments.
You have a few options though. You can make both constants string
types:
private const string KEY = "r";
private const string MSG = "Press " + KEY + " to restart.";
Another option would be to compose the string
at runtime:
private const char KEY = 'r';
private static string MSG => "Press " + KEY + " to restart.";
answered Nov 11 at 2:43


DavidG
67.8k9110125
67.8k9110125
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
add a comment |
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
Thanks, I wanted to avoid this initially, but I ended up needing it converted to a string every time I needed it, so it makes sense to store it as a string
– Andrew Pratt
Nov 11 at 3:39
add a comment |
You can't const string
with other values which is const
.
You can try to use readonly
.
readonly
can only modify values in the class constructor method, it will set that value at runtime.
private const char KEY = 'r';
private readonly string MSG = "Press " + KEY + " to restart.";
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
add a comment |
You can't const string
with other values which is const
.
You can try to use readonly
.
readonly
can only modify values in the class constructor method, it will set that value at runtime.
private const char KEY = 'r';
private readonly string MSG = "Press " + KEY + " to restart.";
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
add a comment |
You can't const string
with other values which is const
.
You can try to use readonly
.
readonly
can only modify values in the class constructor method, it will set that value at runtime.
private const char KEY = 'r';
private readonly string MSG = "Press " + KEY + " to restart.";
You can't const string
with other values which is const
.
You can try to use readonly
.
readonly
can only modify values in the class constructor method, it will set that value at runtime.
private const char KEY = 'r';
private readonly string MSG = "Press " + KEY + " to restart.";
edited Nov 11 at 3:09
answered Nov 11 at 3:00


D-Shih
25.2k61431
25.2k61431
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
add a comment |
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
Thank you, that would work, but I'm going with davidg's suggestion to change the char to be a string because I ended up only ever needing it as a string. I'll be sure to remember both ways in the future though
– Andrew Pratt
Nov 11 at 3:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245343%2fc-sharp-static-cast-char-to-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ald85MmvK9VO46ccpO0uC0SFEWQT2 F7fpZ9b,6GfY6tSk dgaNWVPnvSDfIqnG7TfJpRdVFaA 94StV