How to add data to an array attribute in MongoDB using NodeJS?
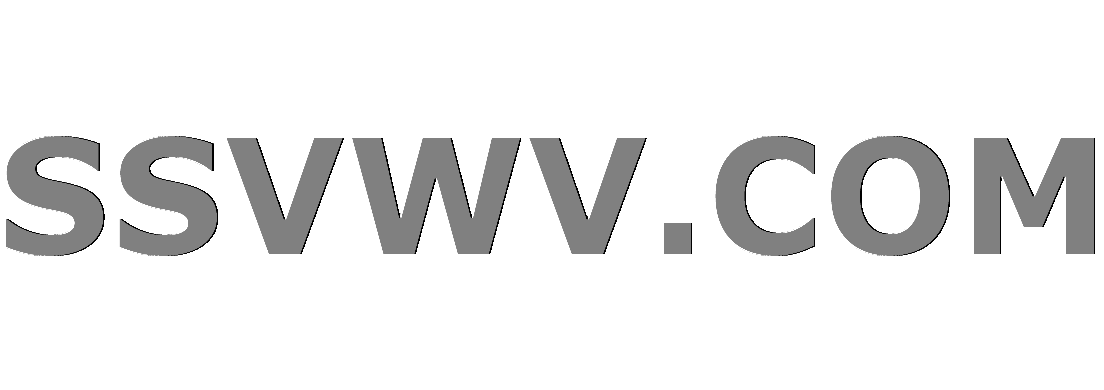
Multi tool use
I just want to add to the articles attribute the title of different articles.
My user.controller.js
file has this function
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
I have a separate router page, index.router.js
, with all my routers, which includes the route for this function
router.post("/savearticle", ctrlUser.savearticle);
Im using Postman to test the function.
In the form-data im setting a key to articles
and the value to article1
.
My response is this
{
"articles": [
null
]
}
Why is it coming out null?
I had done console.log(req.body.articles)
and it returns undefined
.
Why is it undefined
?
Shouldn't it say article1
?
This is the schema I'm making in my user.model.js
page
var userSchema = new mongoose.Schema({
fullName: {
type: String,
required: "Full name can't be empty"
},
email: {
type: String,
required: "E-mail can't be empty",
unique: true
},
password: {
type: String,
required: "Password can't be empty",
minlength: [4, "Password must be atleast 6 characters"]
},
saltSecret: String,
articles: Array
});
And this is the user registration function in the user.controller.js
file
module.exports.register = (req, res, next) => {
var user = new User();
user.fullName = req.body.fullName;
user.email = req.body.email;
user.password = req.body.password;
user.articles = ;
user.save((err, doc) => {
if (!err) {
res.send(doc);
} else {
if (err.code == 11000) {
res.status(422).send(["Duplicate email address found."]);
} else return next(err);
}
});
};
node.js mongodb express mean-stack mean
add a comment |
I just want to add to the articles attribute the title of different articles.
My user.controller.js
file has this function
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
I have a separate router page, index.router.js
, with all my routers, which includes the route for this function
router.post("/savearticle", ctrlUser.savearticle);
Im using Postman to test the function.
In the form-data im setting a key to articles
and the value to article1
.
My response is this
{
"articles": [
null
]
}
Why is it coming out null?
I had done console.log(req.body.articles)
and it returns undefined
.
Why is it undefined
?
Shouldn't it say article1
?
This is the schema I'm making in my user.model.js
page
var userSchema = new mongoose.Schema({
fullName: {
type: String,
required: "Full name can't be empty"
},
email: {
type: String,
required: "E-mail can't be empty",
unique: true
},
password: {
type: String,
required: "Password can't be empty",
minlength: [4, "Password must be atleast 6 characters"]
},
saltSecret: String,
articles: Array
});
And this is the user registration function in the user.controller.js
file
module.exports.register = (req, res, next) => {
var user = new User();
user.fullName = req.body.fullName;
user.email = req.body.email;
user.password = req.body.password;
user.articles = ;
user.save((err, doc) => {
if (!err) {
res.send(doc);
} else {
if (err.code == 11000) {
res.status(422).send(["Duplicate email address found."]);
} else return next(err);
}
});
};
node.js mongodb express mean-stack mean
How could we know? You're not showing us the JSON body you're passing to your API. This doesn't really have anything to do with Mongo as far as I can tell.
– John
Nov 11 at 2:20
Okay I added the schema that I'm sending and the user registration. Is this what you are looking for?
– Bryan Bastida
Nov 11 at 2:25
Not exactly. What isreq.body.articles
? What is the value ofreq.body.articles
andreq.body
at the point where you use it in your mongo query? I'd bet that it's not what you expect.
– John
Nov 11 at 2:29
oh. yeah i'm extremely new to this and following along with a tutorial so i'm not 100% sure whatreq.body.articles
is. i thought that in postman, the key value pair would setreq.body.articles
toarticle1
which would in turn addarticle1
to the database
– Bryan Bastida
Nov 11 at 2:37
I think you need to post a JSON body something like{"articles": {"a":1,"b":2}}
(which makes "articles" as opposed to "article" an odd name.
– John
Nov 11 at 2:38
add a comment |
I just want to add to the articles attribute the title of different articles.
My user.controller.js
file has this function
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
I have a separate router page, index.router.js
, with all my routers, which includes the route for this function
router.post("/savearticle", ctrlUser.savearticle);
Im using Postman to test the function.
In the form-data im setting a key to articles
and the value to article1
.
My response is this
{
"articles": [
null
]
}
Why is it coming out null?
I had done console.log(req.body.articles)
and it returns undefined
.
Why is it undefined
?
Shouldn't it say article1
?
This is the schema I'm making in my user.model.js
page
var userSchema = new mongoose.Schema({
fullName: {
type: String,
required: "Full name can't be empty"
},
email: {
type: String,
required: "E-mail can't be empty",
unique: true
},
password: {
type: String,
required: "Password can't be empty",
minlength: [4, "Password must be atleast 6 characters"]
},
saltSecret: String,
articles: Array
});
And this is the user registration function in the user.controller.js
file
module.exports.register = (req, res, next) => {
var user = new User();
user.fullName = req.body.fullName;
user.email = req.body.email;
user.password = req.body.password;
user.articles = ;
user.save((err, doc) => {
if (!err) {
res.send(doc);
} else {
if (err.code == 11000) {
res.status(422).send(["Duplicate email address found."]);
} else return next(err);
}
});
};
node.js mongodb express mean-stack mean
I just want to add to the articles attribute the title of different articles.
My user.controller.js
file has this function
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
I have a separate router page, index.router.js
, with all my routers, which includes the route for this function
router.post("/savearticle", ctrlUser.savearticle);
Im using Postman to test the function.
In the form-data im setting a key to articles
and the value to article1
.
My response is this
{
"articles": [
null
]
}
Why is it coming out null?
I had done console.log(req.body.articles)
and it returns undefined
.
Why is it undefined
?
Shouldn't it say article1
?
This is the schema I'm making in my user.model.js
page
var userSchema = new mongoose.Schema({
fullName: {
type: String,
required: "Full name can't be empty"
},
email: {
type: String,
required: "E-mail can't be empty",
unique: true
},
password: {
type: String,
required: "Password can't be empty",
minlength: [4, "Password must be atleast 6 characters"]
},
saltSecret: String,
articles: Array
});
And this is the user registration function in the user.controller.js
file
module.exports.register = (req, res, next) => {
var user = new User();
user.fullName = req.body.fullName;
user.email = req.body.email;
user.password = req.body.password;
user.articles = ;
user.save((err, doc) => {
if (!err) {
res.send(doc);
} else {
if (err.code == 11000) {
res.status(422).send(["Duplicate email address found."]);
} else return next(err);
}
});
};
node.js mongodb express mean-stack mean
node.js mongodb express mean-stack mean
edited Nov 11 at 2:24
asked Nov 11 at 2:15
Bryan Bastida
105
105
How could we know? You're not showing us the JSON body you're passing to your API. This doesn't really have anything to do with Mongo as far as I can tell.
– John
Nov 11 at 2:20
Okay I added the schema that I'm sending and the user registration. Is this what you are looking for?
– Bryan Bastida
Nov 11 at 2:25
Not exactly. What isreq.body.articles
? What is the value ofreq.body.articles
andreq.body
at the point where you use it in your mongo query? I'd bet that it's not what you expect.
– John
Nov 11 at 2:29
oh. yeah i'm extremely new to this and following along with a tutorial so i'm not 100% sure whatreq.body.articles
is. i thought that in postman, the key value pair would setreq.body.articles
toarticle1
which would in turn addarticle1
to the database
– Bryan Bastida
Nov 11 at 2:37
I think you need to post a JSON body something like{"articles": {"a":1,"b":2}}
(which makes "articles" as opposed to "article" an odd name.
– John
Nov 11 at 2:38
add a comment |
How could we know? You're not showing us the JSON body you're passing to your API. This doesn't really have anything to do with Mongo as far as I can tell.
– John
Nov 11 at 2:20
Okay I added the schema that I'm sending and the user registration. Is this what you are looking for?
– Bryan Bastida
Nov 11 at 2:25
Not exactly. What isreq.body.articles
? What is the value ofreq.body.articles
andreq.body
at the point where you use it in your mongo query? I'd bet that it's not what you expect.
– John
Nov 11 at 2:29
oh. yeah i'm extremely new to this and following along with a tutorial so i'm not 100% sure whatreq.body.articles
is. i thought that in postman, the key value pair would setreq.body.articles
toarticle1
which would in turn addarticle1
to the database
– Bryan Bastida
Nov 11 at 2:37
I think you need to post a JSON body something like{"articles": {"a":1,"b":2}}
(which makes "articles" as opposed to "article" an odd name.
– John
Nov 11 at 2:38
How could we know? You're not showing us the JSON body you're passing to your API. This doesn't really have anything to do with Mongo as far as I can tell.
– John
Nov 11 at 2:20
How could we know? You're not showing us the JSON body you're passing to your API. This doesn't really have anything to do with Mongo as far as I can tell.
– John
Nov 11 at 2:20
Okay I added the schema that I'm sending and the user registration. Is this what you are looking for?
– Bryan Bastida
Nov 11 at 2:25
Okay I added the schema that I'm sending and the user registration. Is this what you are looking for?
– Bryan Bastida
Nov 11 at 2:25
Not exactly. What is
req.body.articles
? What is the value of req.body.articles
and req.body
at the point where you use it in your mongo query? I'd bet that it's not what you expect.– John
Nov 11 at 2:29
Not exactly. What is
req.body.articles
? What is the value of req.body.articles
and req.body
at the point where you use it in your mongo query? I'd bet that it's not what you expect.– John
Nov 11 at 2:29
oh. yeah i'm extremely new to this and following along with a tutorial so i'm not 100% sure what
req.body.articles
is. i thought that in postman, the key value pair would set req.body.articles
to article1
which would in turn add article1
to the database– Bryan Bastida
Nov 11 at 2:37
oh. yeah i'm extremely new to this and following along with a tutorial so i'm not 100% sure what
req.body.articles
is. i thought that in postman, the key value pair would set req.body.articles
to article1
which would in turn add article1
to the database– Bryan Bastida
Nov 11 at 2:37
I think you need to post a JSON body something like
{"articles": {"a":1,"b":2}}
(which makes "articles" as opposed to "article" an odd name.– John
Nov 11 at 2:38
I think you need to post a JSON body something like
{"articles": {"a":1,"b":2}}
(which makes "articles" as opposed to "article" an odd name.– John
Nov 11 at 2:38
add a comment |
1 Answer
1
active
oldest
votes
You are using findOneAndUpdate
with invalid parameters. First check req._id type (by breakpoint on debug or console.log(req._id)), if it's string
, you should convert it to mongoose.mongo.ObjectId
.
module.exports.savearticle = (req, res, next) => {
let userId = req._id;
if((typeof userId) === 'string'){
userId = mongoose.mongo.ObjectId(userId);
}
User.findOneAndUpdate(
{_id : userId},
{
$push: {articles: {$each : req.body.articles } } //$each need if req.body.articles is an array, else use $push: {articles: req.body.articles } instead of
},
{new: true},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245275%2fhow-to-add-data-to-an-array-attribute-in-mongodb-using-nodejs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are using findOneAndUpdate
with invalid parameters. First check req._id type (by breakpoint on debug or console.log(req._id)), if it's string
, you should convert it to mongoose.mongo.ObjectId
.
module.exports.savearticle = (req, res, next) => {
let userId = req._id;
if((typeof userId) === 'string'){
userId = mongoose.mongo.ObjectId(userId);
}
User.findOneAndUpdate(
{_id : userId},
{
$push: {articles: {$each : req.body.articles } } //$each need if req.body.articles is an array, else use $push: {articles: req.body.articles } instead of
},
{new: true},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
add a comment |
You are using findOneAndUpdate
with invalid parameters. First check req._id type (by breakpoint on debug or console.log(req._id)), if it's string
, you should convert it to mongoose.mongo.ObjectId
.
module.exports.savearticle = (req, res, next) => {
let userId = req._id;
if((typeof userId) === 'string'){
userId = mongoose.mongo.ObjectId(userId);
}
User.findOneAndUpdate(
{_id : userId},
{
$push: {articles: {$each : req.body.articles } } //$each need if req.body.articles is an array, else use $push: {articles: req.body.articles } instead of
},
{new: true},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
add a comment |
You are using findOneAndUpdate
with invalid parameters. First check req._id type (by breakpoint on debug or console.log(req._id)), if it's string
, you should convert it to mongoose.mongo.ObjectId
.
module.exports.savearticle = (req, res, next) => {
let userId = req._id;
if((typeof userId) === 'string'){
userId = mongoose.mongo.ObjectId(userId);
}
User.findOneAndUpdate(
{_id : userId},
{
$push: {articles: {$each : req.body.articles } } //$each need if req.body.articles is an array, else use $push: {articles: req.body.articles } instead of
},
{new: true},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
You are using findOneAndUpdate
with invalid parameters. First check req._id type (by breakpoint on debug or console.log(req._id)), if it's string
, you should convert it to mongoose.mongo.ObjectId
.
module.exports.savearticle = (req, res, next) => {
let userId = req._id;
if((typeof userId) === 'string'){
userId = mongoose.mongo.ObjectId(userId);
}
User.findOneAndUpdate(
{_id : userId},
{
$push: {articles: {$each : req.body.articles } } //$each need if req.body.articles is an array, else use $push: {articles: req.body.articles } instead of
},
{new: true},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
answered Nov 11 at 6:19
Milad Aghamohammadi
791515
791515
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245275%2fhow-to-add-data-to-an-array-attribute-in-mongodb-using-nodejs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XqUafHWM HGfBM,dMsJVewiGxbge M,bfftYwW,fO94ityGwADjTWx4ore0t4W TpW24plPsT1jQdt,E0q21
How could we know? You're not showing us the JSON body you're passing to your API. This doesn't really have anything to do with Mongo as far as I can tell.
– John
Nov 11 at 2:20
Okay I added the schema that I'm sending and the user registration. Is this what you are looking for?
– Bryan Bastida
Nov 11 at 2:25
Not exactly. What is
req.body.articles
? What is the value ofreq.body.articles
andreq.body
at the point where you use it in your mongo query? I'd bet that it's not what you expect.– John
Nov 11 at 2:29
oh. yeah i'm extremely new to this and following along with a tutorial so i'm not 100% sure what
req.body.articles
is. i thought that in postman, the key value pair would setreq.body.articles
toarticle1
which would in turn addarticle1
to the database– Bryan Bastida
Nov 11 at 2:37
I think you need to post a JSON body something like
{"articles": {"a":1,"b":2}}
(which makes "articles" as opposed to "article" an odd name.– John
Nov 11 at 2:38