JavaFX Rubik Cube - cubie selection
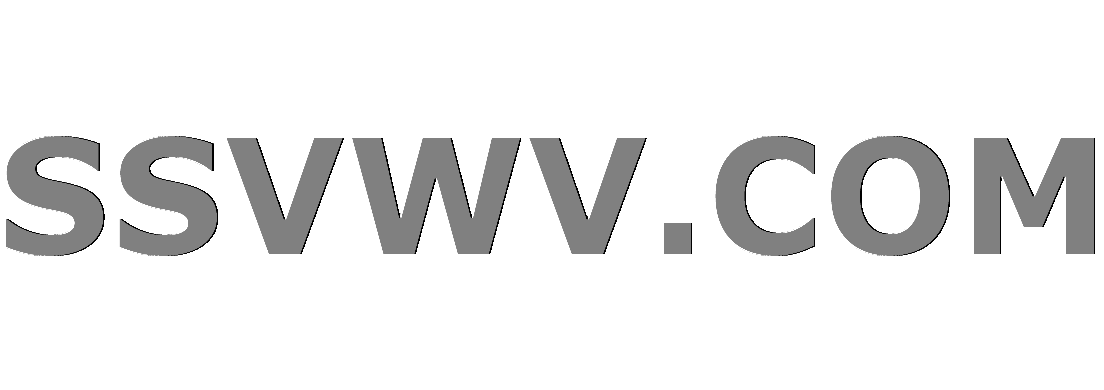
Multi tool use
I am trying to build a Rubiks Cube from scratch as my first real
app using JavaFx. In the Moves
class Im selecting Boxes from ObservableList based on position. The problem is that the Box.getTranslate dosent update so when I try to move the front and the left faces successively the Boxes selected by both are always moved resulting in.. chaos. How could I re-write this so that the Move methods correctly select the boxes to move? Here is the code, work in progress.
Main
package ro.adrianpush;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.*;
import javafx.scene.input.KeyEvent;
import javafx.scene.layout.AnchorPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Box;
import javafx.stage.Stage;
public class Main extends Application {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
final Group root = new Group();
@Override
public void start(Stage primaryStage) throws Exception{
Camera camera = new PerspectiveCamera();
camera.translateXProperty().setValue(-200);
camera.translateYProperty().setValue(0);
camera.translateZProperty().set(-500);
AnchorPane pane = new AnchorPane();
Rubik rubik = new Rubik();
ObservableList<Box> boxArrayList = rubik.getBoxArrayList();
for (Box box: boxArrayList
) {
pane.getChildren().addAll(box);
}
primaryStage.addEventHandler(KeyEvent.KEY_PRESSED, event -> {
switch (event.getCode()){
case E:
Moves.rotateFront(boxArrayList, "clockwise");
break;
case Q:
Moves.rotateFront(boxArrayList, "counterclockwise");
break;
case A:
Moves.rotateLeft(boxArrayList, "clockwise");
break;
case D:
Moves.rotateLeft(boxArrayList, "counterclockwise");
break;
}
});
root.getChildren().add(pane);
Scene scene = new Scene(root, WIDTH, HEIGHT, true);
scene.setCamera(camera);
scene.setFill(Color.ROYALBLUE);
primaryStage.setTitle("The game nobody wants to play");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String args) {
launch(args);
}
}
Rubik
package ro.adrianpush;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
public class Rubik {
private ObservableList<Box> boxArrayList = FXCollections.observableArrayList();
public Rubik(){
for(int i = 1; i < 4; i+=1){
for( int j = 1; j < 4; j++){
for(int k = 1; k < 4; k++){
Box box = new Box(100,100,100);
box.setTranslateX(i*100);
box.setTranslateY(j*100);
box.setTranslateZ(k*100);
boxArrayList.add(box);
}
}
}
}
public ObservableList<Box> getBoxArrayList() {
return boxArrayList;
}
}
Moves
package ro.adrianpush;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
import javafx.scene.transform.Rotate;
public class Moves {
public static void rotateFront(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.Z_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateBack(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 300){
Rotate rotate = new Rotate();
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateLeft(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateX() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.X_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if (box.getTranslateY() == 300){
rotate.setPivotY(-100);
} if(box.getTranslateZ() == 100){
rotate.setPivotZ(100);
} if(box.getTranslateZ() == 300){
rotate.setPivotZ(-100);
}
box.getTransforms().add(rotate);
}
}
}
}
java javafx observablelist javafx-3d rubiks-cube
add a comment |
I am trying to build a Rubiks Cube from scratch as my first real
app using JavaFx. In the Moves
class Im selecting Boxes from ObservableList based on position. The problem is that the Box.getTranslate dosent update so when I try to move the front and the left faces successively the Boxes selected by both are always moved resulting in.. chaos. How could I re-write this so that the Move methods correctly select the boxes to move? Here is the code, work in progress.
Main
package ro.adrianpush;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.*;
import javafx.scene.input.KeyEvent;
import javafx.scene.layout.AnchorPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Box;
import javafx.stage.Stage;
public class Main extends Application {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
final Group root = new Group();
@Override
public void start(Stage primaryStage) throws Exception{
Camera camera = new PerspectiveCamera();
camera.translateXProperty().setValue(-200);
camera.translateYProperty().setValue(0);
camera.translateZProperty().set(-500);
AnchorPane pane = new AnchorPane();
Rubik rubik = new Rubik();
ObservableList<Box> boxArrayList = rubik.getBoxArrayList();
for (Box box: boxArrayList
) {
pane.getChildren().addAll(box);
}
primaryStage.addEventHandler(KeyEvent.KEY_PRESSED, event -> {
switch (event.getCode()){
case E:
Moves.rotateFront(boxArrayList, "clockwise");
break;
case Q:
Moves.rotateFront(boxArrayList, "counterclockwise");
break;
case A:
Moves.rotateLeft(boxArrayList, "clockwise");
break;
case D:
Moves.rotateLeft(boxArrayList, "counterclockwise");
break;
}
});
root.getChildren().add(pane);
Scene scene = new Scene(root, WIDTH, HEIGHT, true);
scene.setCamera(camera);
scene.setFill(Color.ROYALBLUE);
primaryStage.setTitle("The game nobody wants to play");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String args) {
launch(args);
}
}
Rubik
package ro.adrianpush;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
public class Rubik {
private ObservableList<Box> boxArrayList = FXCollections.observableArrayList();
public Rubik(){
for(int i = 1; i < 4; i+=1){
for( int j = 1; j < 4; j++){
for(int k = 1; k < 4; k++){
Box box = new Box(100,100,100);
box.setTranslateX(i*100);
box.setTranslateY(j*100);
box.setTranslateZ(k*100);
boxArrayList.add(box);
}
}
}
}
public ObservableList<Box> getBoxArrayList() {
return boxArrayList;
}
}
Moves
package ro.adrianpush;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
import javafx.scene.transform.Rotate;
public class Moves {
public static void rotateFront(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.Z_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateBack(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 300){
Rotate rotate = new Rotate();
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateLeft(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateX() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.X_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if (box.getTranslateY() == 300){
rotate.setPivotY(-100);
} if(box.getTranslateZ() == 100){
rotate.setPivotZ(100);
} if(box.getTranslateZ() == 300){
rotate.setPivotZ(-100);
}
box.getTransforms().add(rotate);
}
}
}
}
java javafx observablelist javafx-3d rubiks-cube
1
You might want to have a look at this take on the task.
– user1803551
Nov 11 at 9:56
Yourfor
loops are not formatted properly, the(
should be on the same line and usually the{
is too.
– user1803551
Nov 11 at 10:04
As @user1803551 mentioned, that blog post is a good way to start. I discussed about the use ofprepend
transform instead ofadd
. See also the projects LiteRubikFX and RubikFX, or even Rubiks-cube on mobile. See this for a lighter version of the cube.
– José Pereda
Nov 11 at 13:15
add a comment |
I am trying to build a Rubiks Cube from scratch as my first real
app using JavaFx. In the Moves
class Im selecting Boxes from ObservableList based on position. The problem is that the Box.getTranslate dosent update so when I try to move the front and the left faces successively the Boxes selected by both are always moved resulting in.. chaos. How could I re-write this so that the Move methods correctly select the boxes to move? Here is the code, work in progress.
Main
package ro.adrianpush;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.*;
import javafx.scene.input.KeyEvent;
import javafx.scene.layout.AnchorPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Box;
import javafx.stage.Stage;
public class Main extends Application {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
final Group root = new Group();
@Override
public void start(Stage primaryStage) throws Exception{
Camera camera = new PerspectiveCamera();
camera.translateXProperty().setValue(-200);
camera.translateYProperty().setValue(0);
camera.translateZProperty().set(-500);
AnchorPane pane = new AnchorPane();
Rubik rubik = new Rubik();
ObservableList<Box> boxArrayList = rubik.getBoxArrayList();
for (Box box: boxArrayList
) {
pane.getChildren().addAll(box);
}
primaryStage.addEventHandler(KeyEvent.KEY_PRESSED, event -> {
switch (event.getCode()){
case E:
Moves.rotateFront(boxArrayList, "clockwise");
break;
case Q:
Moves.rotateFront(boxArrayList, "counterclockwise");
break;
case A:
Moves.rotateLeft(boxArrayList, "clockwise");
break;
case D:
Moves.rotateLeft(boxArrayList, "counterclockwise");
break;
}
});
root.getChildren().add(pane);
Scene scene = new Scene(root, WIDTH, HEIGHT, true);
scene.setCamera(camera);
scene.setFill(Color.ROYALBLUE);
primaryStage.setTitle("The game nobody wants to play");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String args) {
launch(args);
}
}
Rubik
package ro.adrianpush;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
public class Rubik {
private ObservableList<Box> boxArrayList = FXCollections.observableArrayList();
public Rubik(){
for(int i = 1; i < 4; i+=1){
for( int j = 1; j < 4; j++){
for(int k = 1; k < 4; k++){
Box box = new Box(100,100,100);
box.setTranslateX(i*100);
box.setTranslateY(j*100);
box.setTranslateZ(k*100);
boxArrayList.add(box);
}
}
}
}
public ObservableList<Box> getBoxArrayList() {
return boxArrayList;
}
}
Moves
package ro.adrianpush;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
import javafx.scene.transform.Rotate;
public class Moves {
public static void rotateFront(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.Z_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateBack(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 300){
Rotate rotate = new Rotate();
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateLeft(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateX() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.X_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if (box.getTranslateY() == 300){
rotate.setPivotY(-100);
} if(box.getTranslateZ() == 100){
rotate.setPivotZ(100);
} if(box.getTranslateZ() == 300){
rotate.setPivotZ(-100);
}
box.getTransforms().add(rotate);
}
}
}
}
java javafx observablelist javafx-3d rubiks-cube
I am trying to build a Rubiks Cube from scratch as my first real
app using JavaFx. In the Moves
class Im selecting Boxes from ObservableList based on position. The problem is that the Box.getTranslate dosent update so when I try to move the front and the left faces successively the Boxes selected by both are always moved resulting in.. chaos. How could I re-write this so that the Move methods correctly select the boxes to move? Here is the code, work in progress.
Main
package ro.adrianpush;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.*;
import javafx.scene.input.KeyEvent;
import javafx.scene.layout.AnchorPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Box;
import javafx.stage.Stage;
public class Main extends Application {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
final Group root = new Group();
@Override
public void start(Stage primaryStage) throws Exception{
Camera camera = new PerspectiveCamera();
camera.translateXProperty().setValue(-200);
camera.translateYProperty().setValue(0);
camera.translateZProperty().set(-500);
AnchorPane pane = new AnchorPane();
Rubik rubik = new Rubik();
ObservableList<Box> boxArrayList = rubik.getBoxArrayList();
for (Box box: boxArrayList
) {
pane.getChildren().addAll(box);
}
primaryStage.addEventHandler(KeyEvent.KEY_PRESSED, event -> {
switch (event.getCode()){
case E:
Moves.rotateFront(boxArrayList, "clockwise");
break;
case Q:
Moves.rotateFront(boxArrayList, "counterclockwise");
break;
case A:
Moves.rotateLeft(boxArrayList, "clockwise");
break;
case D:
Moves.rotateLeft(boxArrayList, "counterclockwise");
break;
}
});
root.getChildren().add(pane);
Scene scene = new Scene(root, WIDTH, HEIGHT, true);
scene.setCamera(camera);
scene.setFill(Color.ROYALBLUE);
primaryStage.setTitle("The game nobody wants to play");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String args) {
launch(args);
}
}
Rubik
package ro.adrianpush;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
public class Rubik {
private ObservableList<Box> boxArrayList = FXCollections.observableArrayList();
public Rubik(){
for(int i = 1; i < 4; i+=1){
for( int j = 1; j < 4; j++){
for(int k = 1; k < 4; k++){
Box box = new Box(100,100,100);
box.setTranslateX(i*100);
box.setTranslateY(j*100);
box.setTranslateZ(k*100);
boxArrayList.add(box);
}
}
}
}
public ObservableList<Box> getBoxArrayList() {
return boxArrayList;
}
}
Moves
package ro.adrianpush;
import javafx.collections.ObservableList;
import javafx.scene.shape.Box;
import javafx.scene.transform.Rotate;
public class Moves {
public static void rotateFront(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.Z_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateBack(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateZ() == 300){
Rotate rotate = new Rotate();
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateX() == 100){
rotate.setPivotX(100);
} if (box.getTranslateX() == 300){
rotate.setPivotX(-100);
} if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if(box.getTranslateY() == 300){
rotate.setPivotY(-100);
}
box.getTransforms().add(rotate);
}
}
}
public static void rotateLeft(ObservableList<Box> boxArrayList, String direction) {
for (Box box: boxArrayList
) {
if(box.getTranslateX() == 100){
Rotate rotate = new Rotate();
rotate.setAxis(Rotate.X_AXIS);
if(direction == "clockwise"){
rotate.setAngle(5);
} else if (direction == "counterclockwise"){
rotate.setAngle(-5);
}
if(box.getTranslateY() == 100){
rotate.setPivotY(100);
} if (box.getTranslateY() == 300){
rotate.setPivotY(-100);
} if(box.getTranslateZ() == 100){
rotate.setPivotZ(100);
} if(box.getTranslateZ() == 300){
rotate.setPivotZ(-100);
}
box.getTransforms().add(rotate);
}
}
}
}
java javafx observablelist javafx-3d rubiks-cube
java javafx observablelist javafx-3d rubiks-cube
edited Nov 11 at 13:16


José Pereda
24.9k33865
24.9k33865
asked Nov 11 at 2:47


Adrian Puşcuţă
63
63
1
You might want to have a look at this take on the task.
– user1803551
Nov 11 at 9:56
Yourfor
loops are not formatted properly, the(
should be on the same line and usually the{
is too.
– user1803551
Nov 11 at 10:04
As @user1803551 mentioned, that blog post is a good way to start. I discussed about the use ofprepend
transform instead ofadd
. See also the projects LiteRubikFX and RubikFX, or even Rubiks-cube on mobile. See this for a lighter version of the cube.
– José Pereda
Nov 11 at 13:15
add a comment |
1
You might want to have a look at this take on the task.
– user1803551
Nov 11 at 9:56
Yourfor
loops are not formatted properly, the(
should be on the same line and usually the{
is too.
– user1803551
Nov 11 at 10:04
As @user1803551 mentioned, that blog post is a good way to start. I discussed about the use ofprepend
transform instead ofadd
. See also the projects LiteRubikFX and RubikFX, or even Rubiks-cube on mobile. See this for a lighter version of the cube.
– José Pereda
Nov 11 at 13:15
1
1
You might want to have a look at this take on the task.
– user1803551
Nov 11 at 9:56
You might want to have a look at this take on the task.
– user1803551
Nov 11 at 9:56
Your
for
loops are not formatted properly, the (
should be on the same line and usually the {
is too.– user1803551
Nov 11 at 10:04
Your
for
loops are not formatted properly, the (
should be on the same line and usually the {
is too.– user1803551
Nov 11 at 10:04
As @user1803551 mentioned, that blog post is a good way to start. I discussed about the use of
prepend
transform instead of add
. See also the projects LiteRubikFX and RubikFX, or even Rubiks-cube on mobile. See this for a lighter version of the cube.– José Pereda
Nov 11 at 13:15
As @user1803551 mentioned, that blog post is a good way to start. I discussed about the use of
prepend
transform instead of add
. See also the projects LiteRubikFX and RubikFX, or even Rubiks-cube on mobile. See this for a lighter version of the cube.– José Pereda
Nov 11 at 13:15
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245417%2fjavafx-rubik-cube-cubie-selection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245417%2fjavafx-rubik-cube-cubie-selection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h xnKhFc 5qN,c,wYE,EnZBRfSN,nRj5rK10aJ7Q BgbRZ
1
You might want to have a look at this take on the task.
– user1803551
Nov 11 at 9:56
Your
for
loops are not formatted properly, the(
should be on the same line and usually the{
is too.– user1803551
Nov 11 at 10:04
As @user1803551 mentioned, that blog post is a good way to start. I discussed about the use of
prepend
transform instead ofadd
. See also the projects LiteRubikFX and RubikFX, or even Rubiks-cube on mobile. See this for a lighter version of the cube.– José Pereda
Nov 11 at 13:15