How to parse recursive parentheses correctly?
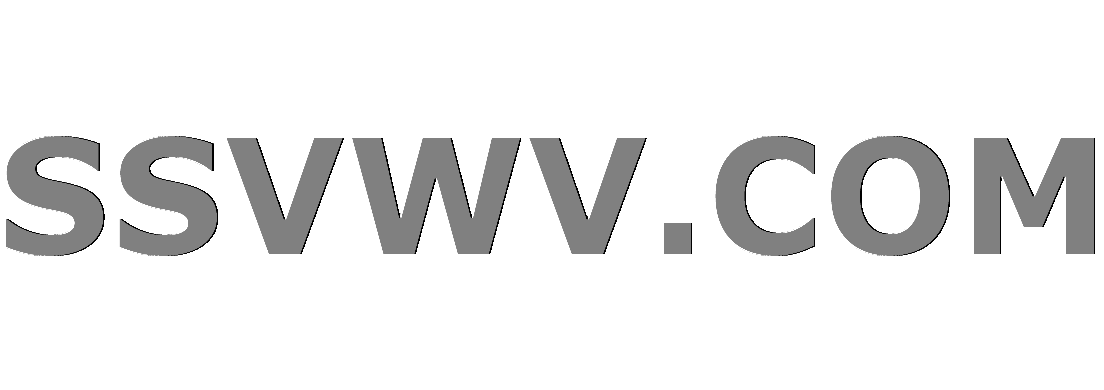
Multi tool use
I need to parse a string that contains some parentheses disposed recursively, but i'm having trouble with determining priority of parentheses.
For exemple, I have the string
$truth = "((A^¬B)->C)";
and I need to return what is between the parentheses. I've already done it with the following regex:
preg_match_all("~((.*?))~", $truth, $str);
But the problem is that it returns what is between the first "(" and the first ")", which is
(A^¬B
Instead of this, i need it to 'know' where the parentheses closes correctly, in order to return
(A^¬B)->C
How can I return this respecting the priority order? Thanks!
php regex
add a comment |
I need to parse a string that contains some parentheses disposed recursively, but i'm having trouble with determining priority of parentheses.
For exemple, I have the string
$truth = "((A^¬B)->C)";
and I need to return what is between the parentheses. I've already done it with the following regex:
preg_match_all("~((.*?))~", $truth, $str);
But the problem is that it returns what is between the first "(" and the first ")", which is
(A^¬B
Instead of this, i need it to 'know' where the parentheses closes correctly, in order to return
(A^¬B)->C
How can I return this respecting the priority order? Thanks!
php regex
You could just make an exclusion group and match anything but parenthesis with[^()]*
instead of.*
, but you might probably still run into problems depending on the complexity of the expression you're trying to parse, specially if it's malformed. Regular expressions are handy but they don't apply to every parsing problem.
– Havenard
Nov 11 at 1:43
Regular expressions are not adequate for parsing a language. Try a parser generator. stackoverflow.com/questions/3720362/…
– Ralph Ritoch
Nov 11 at 1:46
add a comment |
I need to parse a string that contains some parentheses disposed recursively, but i'm having trouble with determining priority of parentheses.
For exemple, I have the string
$truth = "((A^¬B)->C)";
and I need to return what is between the parentheses. I've already done it with the following regex:
preg_match_all("~((.*?))~", $truth, $str);
But the problem is that it returns what is between the first "(" and the first ")", which is
(A^¬B
Instead of this, i need it to 'know' where the parentheses closes correctly, in order to return
(A^¬B)->C
How can I return this respecting the priority order? Thanks!
php regex
I need to parse a string that contains some parentheses disposed recursively, but i'm having trouble with determining priority of parentheses.
For exemple, I have the string
$truth = "((A^¬B)->C)";
and I need to return what is between the parentheses. I've already done it with the following regex:
preg_match_all("~((.*?))~", $truth, $str);
But the problem is that it returns what is between the first "(" and the first ")", which is
(A^¬B
Instead of this, i need it to 'know' where the parentheses closes correctly, in order to return
(A^¬B)->C
How can I return this respecting the priority order? Thanks!
php regex
php regex
edited Nov 11 at 1:37
Nick
22.8k81535
22.8k81535
asked Nov 11 at 1:22
Nicholas Ferreira
284
284
You could just make an exclusion group and match anything but parenthesis with[^()]*
instead of.*
, but you might probably still run into problems depending on the complexity of the expression you're trying to parse, specially if it's malformed. Regular expressions are handy but they don't apply to every parsing problem.
– Havenard
Nov 11 at 1:43
Regular expressions are not adequate for parsing a language. Try a parser generator. stackoverflow.com/questions/3720362/…
– Ralph Ritoch
Nov 11 at 1:46
add a comment |
You could just make an exclusion group and match anything but parenthesis with[^()]*
instead of.*
, but you might probably still run into problems depending on the complexity of the expression you're trying to parse, specially if it's malformed. Regular expressions are handy but they don't apply to every parsing problem.
– Havenard
Nov 11 at 1:43
Regular expressions are not adequate for parsing a language. Try a parser generator. stackoverflow.com/questions/3720362/…
– Ralph Ritoch
Nov 11 at 1:46
You could just make an exclusion group and match anything but parenthesis with
[^()]*
instead of .*
, but you might probably still run into problems depending on the complexity of the expression you're trying to parse, specially if it's malformed. Regular expressions are handy but they don't apply to every parsing problem.– Havenard
Nov 11 at 1:43
You could just make an exclusion group and match anything but parenthesis with
[^()]*
instead of .*
, but you might probably still run into problems depending on the complexity of the expression you're trying to parse, specially if it's malformed. Regular expressions are handy but they don't apply to every parsing problem.– Havenard
Nov 11 at 1:43
Regular expressions are not adequate for parsing a language. Try a parser generator. stackoverflow.com/questions/3720362/…
– Ralph Ritoch
Nov 11 at 1:46
Regular expressions are not adequate for parsing a language. Try a parser generator. stackoverflow.com/questions/3720362/…
– Ralph Ritoch
Nov 11 at 1:46
add a comment |
2 Answers
2
active
oldest
votes
The main problem you have right now is the ?
non-greedy bit. If you change that to just .+
greedy it will match what you want.
$truth = "((A^¬B)->C)";
preg_match('/(.+)/', $truth, $match);
Try it
Output
(A^¬B)->C
If you want to match the inner pair you can use a recursive subpattern:
$truth = "((A^¬B)->C)";
preg_match('/(([^()]+|(?0)))/', $truth, $match);
Try It online
Output
A^¬B
If you need to go further then that you can make a lexer/parser. I have some examples here:
https://github.com/ArtisticPhoenix/MISC/tree/master/Lexers
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
add a comment |
For your sample string, something like this will recursively give you the contents of the parentheses. It works by forcing the parentheses matched to be the outermost pair by using ^[^(]*
and [^)]*$
at each end of the regex.
$truth = "((A^¬B)->C)";
while (strpos($truth, '(') !== false) {
preg_match("~^[^(]*((.*?))[^)]*$~", $truth, $str);
$truth = $str[1];
echo "$truthn";
}
Output
(A^¬B)->C
A^¬B
Note however this will not correctly parse a string such as (A+B)-(C+D)
. If that could be your scenario, this answer might help.
Demo on 3v4l.org
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245032%2fhow-to-parse-recursive-parentheses-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The main problem you have right now is the ?
non-greedy bit. If you change that to just .+
greedy it will match what you want.
$truth = "((A^¬B)->C)";
preg_match('/(.+)/', $truth, $match);
Try it
Output
(A^¬B)->C
If you want to match the inner pair you can use a recursive subpattern:
$truth = "((A^¬B)->C)";
preg_match('/(([^()]+|(?0)))/', $truth, $match);
Try It online
Output
A^¬B
If you need to go further then that you can make a lexer/parser. I have some examples here:
https://github.com/ArtisticPhoenix/MISC/tree/master/Lexers
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
add a comment |
The main problem you have right now is the ?
non-greedy bit. If you change that to just .+
greedy it will match what you want.
$truth = "((A^¬B)->C)";
preg_match('/(.+)/', $truth, $match);
Try it
Output
(A^¬B)->C
If you want to match the inner pair you can use a recursive subpattern:
$truth = "((A^¬B)->C)";
preg_match('/(([^()]+|(?0)))/', $truth, $match);
Try It online
Output
A^¬B
If you need to go further then that you can make a lexer/parser. I have some examples here:
https://github.com/ArtisticPhoenix/MISC/tree/master/Lexers
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
add a comment |
The main problem you have right now is the ?
non-greedy bit. If you change that to just .+
greedy it will match what you want.
$truth = "((A^¬B)->C)";
preg_match('/(.+)/', $truth, $match);
Try it
Output
(A^¬B)->C
If you want to match the inner pair you can use a recursive subpattern:
$truth = "((A^¬B)->C)";
preg_match('/(([^()]+|(?0)))/', $truth, $match);
Try It online
Output
A^¬B
If you need to go further then that you can make a lexer/parser. I have some examples here:
https://github.com/ArtisticPhoenix/MISC/tree/master/Lexers
The main problem you have right now is the ?
non-greedy bit. If you change that to just .+
greedy it will match what you want.
$truth = "((A^¬B)->C)";
preg_match('/(.+)/', $truth, $match);
Try it
Output
(A^¬B)->C
If you want to match the inner pair you can use a recursive subpattern:
$truth = "((A^¬B)->C)";
preg_match('/(([^()]+|(?0)))/', $truth, $match);
Try It online
Output
A^¬B
If you need to go further then that you can make a lexer/parser. I have some examples here:
https://github.com/ArtisticPhoenix/MISC/tree/master/Lexers
edited Nov 11 at 2:02
answered Nov 11 at 1:56


ArtisticPhoenix
15.1k11223
15.1k11223
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
add a comment |
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Thanks! It solved my problem. And thanks to the others too, it will be useful. =D
– Nicholas Ferreira
Nov 11 at 2:14
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
Sure I just added my output converter to my website, artisticphoenix.com/2018/11/11/output-converter it uses the same parsing idea but can convert var_export and print_r to usable arrays. Something I have to do a lot on here... lol
– ArtisticPhoenix
Nov 11 at 2:31
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
@ArtisticPhoenix I was just thinking I was going to have to write the same tool myself! Thanks for sharing...
– Nick
Nov 11 at 3:50
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
Sure, My site is still a work in progress. lol. I don't get a lot of time to work on it unfortunately
– ArtisticPhoenix
Nov 11 at 3:53
add a comment |
For your sample string, something like this will recursively give you the contents of the parentheses. It works by forcing the parentheses matched to be the outermost pair by using ^[^(]*
and [^)]*$
at each end of the regex.
$truth = "((A^¬B)->C)";
while (strpos($truth, '(') !== false) {
preg_match("~^[^(]*((.*?))[^)]*$~", $truth, $str);
$truth = $str[1];
echo "$truthn";
}
Output
(A^¬B)->C
A^¬B
Note however this will not correctly parse a string such as (A+B)-(C+D)
. If that could be your scenario, this answer might help.
Demo on 3v4l.org
add a comment |
For your sample string, something like this will recursively give you the contents of the parentheses. It works by forcing the parentheses matched to be the outermost pair by using ^[^(]*
and [^)]*$
at each end of the regex.
$truth = "((A^¬B)->C)";
while (strpos($truth, '(') !== false) {
preg_match("~^[^(]*((.*?))[^)]*$~", $truth, $str);
$truth = $str[1];
echo "$truthn";
}
Output
(A^¬B)->C
A^¬B
Note however this will not correctly parse a string such as (A+B)-(C+D)
. If that could be your scenario, this answer might help.
Demo on 3v4l.org
add a comment |
For your sample string, something like this will recursively give you the contents of the parentheses. It works by forcing the parentheses matched to be the outermost pair by using ^[^(]*
and [^)]*$
at each end of the regex.
$truth = "((A^¬B)->C)";
while (strpos($truth, '(') !== false) {
preg_match("~^[^(]*((.*?))[^)]*$~", $truth, $str);
$truth = $str[1];
echo "$truthn";
}
Output
(A^¬B)->C
A^¬B
Note however this will not correctly parse a string such as (A+B)-(C+D)
. If that could be your scenario, this answer might help.
Demo on 3v4l.org
For your sample string, something like this will recursively give you the contents of the parentheses. It works by forcing the parentheses matched to be the outermost pair by using ^[^(]*
and [^)]*$
at each end of the regex.
$truth = "((A^¬B)->C)";
while (strpos($truth, '(') !== false) {
preg_match("~^[^(]*((.*?))[^)]*$~", $truth, $str);
$truth = $str[1];
echo "$truthn";
}
Output
(A^¬B)->C
A^¬B
Note however this will not correctly parse a string such as (A+B)-(C+D)
. If that could be your scenario, this answer might help.
Demo on 3v4l.org
edited Nov 11 at 2:12
answered Nov 11 at 1:37
Nick
22.8k81535
22.8k81535
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245032%2fhow-to-parse-recursive-parentheses-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S0lD 6BscQxWA9FGTWU7pRorR32N7c9h aLZAdeaK,C0nj D
You could just make an exclusion group and match anything but parenthesis with
[^()]*
instead of.*
, but you might probably still run into problems depending on the complexity of the expression you're trying to parse, specially if it's malformed. Regular expressions are handy but they don't apply to every parsing problem.– Havenard
Nov 11 at 1:43
Regular expressions are not adequate for parsing a language. Try a parser generator. stackoverflow.com/questions/3720362/…
– Ralph Ritoch
Nov 11 at 1:46