JavaFX app that uses Executor hangs on quit
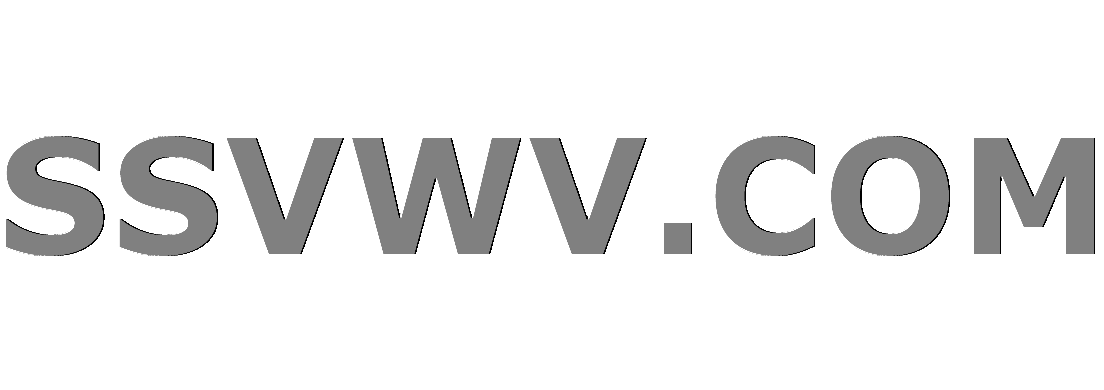
Multi tool use
up vote
1
down vote
favorite
I'm trying to make a clone of Android's AsyncTask to use in a JavaFX app. Here's the code I've come up with:
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
abstract public class AsyncTask<Param, Result>
{
private Param param;
private static Executor executor;
public AsyncTask()
{
if (executor == null)
executor = Executors.newSingleThreadExecutor();
}
protected void onPreExecute()
{
}
protected Result doInBackground(Param param)
{
return null;
}
protected void onPostExecute(Result result)
{
}
final public void execute(Param param)
{
this.param = param;
onPreExecute();
Task t = new Task();
executor.execute(t);
}
private class Task implements Runnable
{
public void run()
{
Result result = doInBackground(param);
onPostExecute(result);
}
}
}
I can use this class in my JavaFX app and it works fine except for one thing: when I close the main window, the JVM hangs rather than exiting cleanly. I have to force-quit the app.
I think the problem is related to the Executor. Because I don't issue a shutdown(), the Executor hangs waiting for more tasks to execute. Because AsyncTask is a wrapper for Java's Executor, the class that extends AsyncTask won't have direct access to the Executor and therefore can't issue a shutdown(). How can I do an orderly shutdown of the Executor?
java javafx android-asynctask executorservice java.util.concurrent
add a comment |
up vote
1
down vote
favorite
I'm trying to make a clone of Android's AsyncTask to use in a JavaFX app. Here's the code I've come up with:
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
abstract public class AsyncTask<Param, Result>
{
private Param param;
private static Executor executor;
public AsyncTask()
{
if (executor == null)
executor = Executors.newSingleThreadExecutor();
}
protected void onPreExecute()
{
}
protected Result doInBackground(Param param)
{
return null;
}
protected void onPostExecute(Result result)
{
}
final public void execute(Param param)
{
this.param = param;
onPreExecute();
Task t = new Task();
executor.execute(t);
}
private class Task implements Runnable
{
public void run()
{
Result result = doInBackground(param);
onPostExecute(result);
}
}
}
I can use this class in my JavaFX app and it works fine except for one thing: when I close the main window, the JVM hangs rather than exiting cleanly. I have to force-quit the app.
I think the problem is related to the Executor. Because I don't issue a shutdown(), the Executor hangs waiting for more tasks to execute. Because AsyncTask is a wrapper for Java's Executor, the class that extends AsyncTask won't have direct access to the Executor and therefore can't issue a shutdown(). How can I do an orderly shutdown of the Executor?
java javafx android-asynctask executorservice java.util.concurrent
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to make a clone of Android's AsyncTask to use in a JavaFX app. Here's the code I've come up with:
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
abstract public class AsyncTask<Param, Result>
{
private Param param;
private static Executor executor;
public AsyncTask()
{
if (executor == null)
executor = Executors.newSingleThreadExecutor();
}
protected void onPreExecute()
{
}
protected Result doInBackground(Param param)
{
return null;
}
protected void onPostExecute(Result result)
{
}
final public void execute(Param param)
{
this.param = param;
onPreExecute();
Task t = new Task();
executor.execute(t);
}
private class Task implements Runnable
{
public void run()
{
Result result = doInBackground(param);
onPostExecute(result);
}
}
}
I can use this class in my JavaFX app and it works fine except for one thing: when I close the main window, the JVM hangs rather than exiting cleanly. I have to force-quit the app.
I think the problem is related to the Executor. Because I don't issue a shutdown(), the Executor hangs waiting for more tasks to execute. Because AsyncTask is a wrapper for Java's Executor, the class that extends AsyncTask won't have direct access to the Executor and therefore can't issue a shutdown(). How can I do an orderly shutdown of the Executor?
java javafx android-asynctask executorservice java.util.concurrent
I'm trying to make a clone of Android's AsyncTask to use in a JavaFX app. Here's the code I've come up with:
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
abstract public class AsyncTask<Param, Result>
{
private Param param;
private static Executor executor;
public AsyncTask()
{
if (executor == null)
executor = Executors.newSingleThreadExecutor();
}
protected void onPreExecute()
{
}
protected Result doInBackground(Param param)
{
return null;
}
protected void onPostExecute(Result result)
{
}
final public void execute(Param param)
{
this.param = param;
onPreExecute();
Task t = new Task();
executor.execute(t);
}
private class Task implements Runnable
{
public void run()
{
Result result = doInBackground(param);
onPostExecute(result);
}
}
}
I can use this class in my JavaFX app and it works fine except for one thing: when I close the main window, the JVM hangs rather than exiting cleanly. I have to force-quit the app.
I think the problem is related to the Executor. Because I don't issue a shutdown(), the Executor hangs waiting for more tasks to execute. Because AsyncTask is a wrapper for Java's Executor, the class that extends AsyncTask won't have direct access to the Executor and therefore can't issue a shutdown(). How can I do an orderly shutdown of the Executor?
java javafx android-asynctask executorservice java.util.concurrent
java javafx android-asynctask executorservice java.util.concurrent
asked Nov 7 at 9:11
Barry Brown
13.2k116098
13.2k116098
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
4
down vote
accepted
You either need to shutdown the executor from the Application.stop
method or you make sure the Executor
's thread won't prevent the JVM from shutting down by using a daemon thread:
if (executor == null) {
executor = Executors.newSingleThreadExecutor(r -> {
Thread t = new Thread(r);
t.setDaemon(true);
return t;
});
}
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
You either need to shutdown the executor from the Application.stop
method or you make sure the Executor
's thread won't prevent the JVM from shutting down by using a daemon thread:
if (executor == null) {
executor = Executors.newSingleThreadExecutor(r -> {
Thread t = new Thread(r);
t.setDaemon(true);
return t;
});
}
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
add a comment |
up vote
4
down vote
accepted
You either need to shutdown the executor from the Application.stop
method or you make sure the Executor
's thread won't prevent the JVM from shutting down by using a daemon thread:
if (executor == null) {
executor = Executors.newSingleThreadExecutor(r -> {
Thread t = new Thread(r);
t.setDaemon(true);
return t;
});
}
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
You either need to shutdown the executor from the Application.stop
method or you make sure the Executor
's thread won't prevent the JVM from shutting down by using a daemon thread:
if (executor == null) {
executor = Executors.newSingleThreadExecutor(r -> {
Thread t = new Thread(r);
t.setDaemon(true);
return t;
});
}
You either need to shutdown the executor from the Application.stop
method or you make sure the Executor
's thread won't prevent the JVM from shutting down by using a daemon thread:
if (executor == null) {
executor = Executors.newSingleThreadExecutor(r -> {
Thread t = new Thread(r);
t.setDaemon(true);
return t;
});
}
answered Nov 7 at 9:35
fabian
48.7k114968
48.7k114968
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
add a comment |
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
I used the setDaemon technique. Worked like a charm! Thanks a bunch!
– Barry Brown
Nov 7 at 17:34
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53186395%2fjavafx-app-that-uses-executor-hangs-on-quit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P8qP,0v8i9FkITOtn4QdX2hYKho0O,5ojDGz,jejF,RS 0ai3Snxat3 0RI26j66xjf03,8,oNmxBee6nM 4yam3YPlEe