WPF MessageBox is not displayed at all if long message is passed
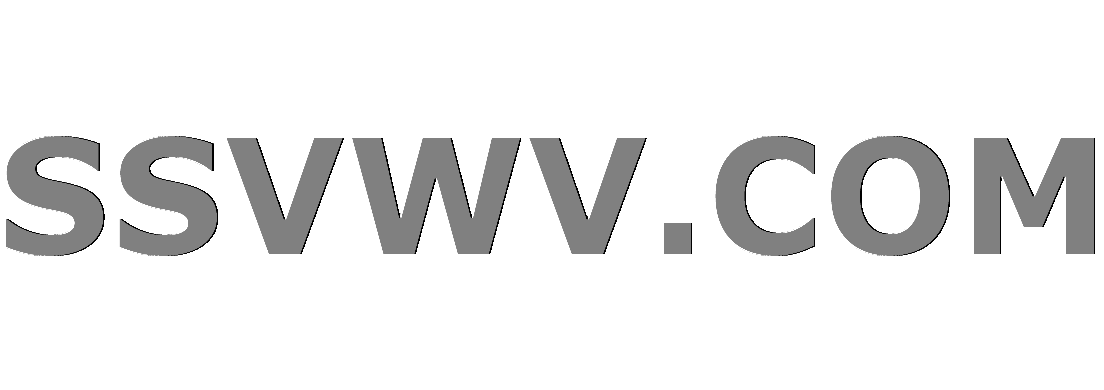
Multi tool use
up vote
0
down vote
favorite
I ran into situation when some MessageBoxes with error messages were not displayed at all. After closer investigation, I was able to narrow down the problem to cases when a very long string is passed as messageBoxText
.
In such cases, call MessageBox.Show
does not display anything, returns MessageBoxResult.No
and, in most cases, there's a message in the Output
window saying The thread 0xHEXNUMBER has exited with code 0 (0x0)
. For me, this method silently fails.
This is very strange - WPF is a very mature technology and I expect this code to work according to spec or throw some exception (e.g. OutOfMemory, StackOverflowException). I've debugged the program and no manged or unmanged exceptions are thrown and caught.
What is the root cause of MessageBox not being displayed? Is there some easy way to debug such things (since no exceptions are thrown or logged). What is the messageBoxText length limit and what does it depend on (I could check that empirically on my computer, but would be conclusive for one OS/platform/.NET Framework version at best)?
Repro:
Here's a code which demonstrates the problem (it can be used with standard WPF Application template in Visual Studio).
MainWindow.xaml:
<Window x:Class="LongMessageInMessageBox.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Button Grid.Row="0" Grid.Column="0" Click="ShortMessage_Clicked">Short Message</Button>
<Button Grid.Row="1" Grid.Column="0" Click="LongMessage_Clicked">Long Message</Button>
</Grid>
MainWindow.xaml.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace LongMessageInMessageBox
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
/// Method which shows that displaying short message works correctly
private void ShortMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("ShortMessage_Clicked");
var result = MessageBox.Show("Short Message");
Console.WriteLine(result);
}
/// Method which shows that displaying a long message does not work
private void LongMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("LongMessage_Clicked");
var longTextBuilder = new System.Text.StringBuilder(10000);
longTextBuilder.Append("Long Message n");
for (int i = 1; i <= 100000; i++)
{
longTextBuilder.Append(" ").Append(i);
}
var result = MessageBox.Show(longTextBuilder.ToString());
Console.WriteLine(result);
}
}
}
Sample from Output
window:
ShortMessage_Clicked
OK
ShortMessage_Clicked
OK
LongMessage_Clicked
No
The thread 0x55bc has exited with code 0 (0x0).
wpf messagebox
add a comment |
up vote
0
down vote
favorite
I ran into situation when some MessageBoxes with error messages were not displayed at all. After closer investigation, I was able to narrow down the problem to cases when a very long string is passed as messageBoxText
.
In such cases, call MessageBox.Show
does not display anything, returns MessageBoxResult.No
and, in most cases, there's a message in the Output
window saying The thread 0xHEXNUMBER has exited with code 0 (0x0)
. For me, this method silently fails.
This is very strange - WPF is a very mature technology and I expect this code to work according to spec or throw some exception (e.g. OutOfMemory, StackOverflowException). I've debugged the program and no manged or unmanged exceptions are thrown and caught.
What is the root cause of MessageBox not being displayed? Is there some easy way to debug such things (since no exceptions are thrown or logged). What is the messageBoxText length limit and what does it depend on (I could check that empirically on my computer, but would be conclusive for one OS/platform/.NET Framework version at best)?
Repro:
Here's a code which demonstrates the problem (it can be used with standard WPF Application template in Visual Studio).
MainWindow.xaml:
<Window x:Class="LongMessageInMessageBox.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Button Grid.Row="0" Grid.Column="0" Click="ShortMessage_Clicked">Short Message</Button>
<Button Grid.Row="1" Grid.Column="0" Click="LongMessage_Clicked">Long Message</Button>
</Grid>
MainWindow.xaml.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace LongMessageInMessageBox
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
/// Method which shows that displaying short message works correctly
private void ShortMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("ShortMessage_Clicked");
var result = MessageBox.Show("Short Message");
Console.WriteLine(result);
}
/// Method which shows that displaying a long message does not work
private void LongMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("LongMessage_Clicked");
var longTextBuilder = new System.Text.StringBuilder(10000);
longTextBuilder.Append("Long Message n");
for (int i = 1; i <= 100000; i++)
{
longTextBuilder.Append(" ").Append(i);
}
var result = MessageBox.Show(longTextBuilder.ToString());
Console.WriteLine(result);
}
}
}
Sample from Output
window:
ShortMessage_Clicked
OK
ShortMessage_Clicked
OK
LongMessage_Clicked
No
The thread 0x55bc has exited with code 0 (0x0).
wpf messagebox
My guess is that it fails silently as WPF does often. Did you check?
– Jan
Nov 9 at 18:15
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I ran into situation when some MessageBoxes with error messages were not displayed at all. After closer investigation, I was able to narrow down the problem to cases when a very long string is passed as messageBoxText
.
In such cases, call MessageBox.Show
does not display anything, returns MessageBoxResult.No
and, in most cases, there's a message in the Output
window saying The thread 0xHEXNUMBER has exited with code 0 (0x0)
. For me, this method silently fails.
This is very strange - WPF is a very mature technology and I expect this code to work according to spec or throw some exception (e.g. OutOfMemory, StackOverflowException). I've debugged the program and no manged or unmanged exceptions are thrown and caught.
What is the root cause of MessageBox not being displayed? Is there some easy way to debug such things (since no exceptions are thrown or logged). What is the messageBoxText length limit and what does it depend on (I could check that empirically on my computer, but would be conclusive for one OS/platform/.NET Framework version at best)?
Repro:
Here's a code which demonstrates the problem (it can be used with standard WPF Application template in Visual Studio).
MainWindow.xaml:
<Window x:Class="LongMessageInMessageBox.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Button Grid.Row="0" Grid.Column="0" Click="ShortMessage_Clicked">Short Message</Button>
<Button Grid.Row="1" Grid.Column="0" Click="LongMessage_Clicked">Long Message</Button>
</Grid>
MainWindow.xaml.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace LongMessageInMessageBox
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
/// Method which shows that displaying short message works correctly
private void ShortMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("ShortMessage_Clicked");
var result = MessageBox.Show("Short Message");
Console.WriteLine(result);
}
/// Method which shows that displaying a long message does not work
private void LongMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("LongMessage_Clicked");
var longTextBuilder = new System.Text.StringBuilder(10000);
longTextBuilder.Append("Long Message n");
for (int i = 1; i <= 100000; i++)
{
longTextBuilder.Append(" ").Append(i);
}
var result = MessageBox.Show(longTextBuilder.ToString());
Console.WriteLine(result);
}
}
}
Sample from Output
window:
ShortMessage_Clicked
OK
ShortMessage_Clicked
OK
LongMessage_Clicked
No
The thread 0x55bc has exited with code 0 (0x0).
wpf messagebox
I ran into situation when some MessageBoxes with error messages were not displayed at all. After closer investigation, I was able to narrow down the problem to cases when a very long string is passed as messageBoxText
.
In such cases, call MessageBox.Show
does not display anything, returns MessageBoxResult.No
and, in most cases, there's a message in the Output
window saying The thread 0xHEXNUMBER has exited with code 0 (0x0)
. For me, this method silently fails.
This is very strange - WPF is a very mature technology and I expect this code to work according to spec or throw some exception (e.g. OutOfMemory, StackOverflowException). I've debugged the program and no manged or unmanged exceptions are thrown and caught.
What is the root cause of MessageBox not being displayed? Is there some easy way to debug such things (since no exceptions are thrown or logged). What is the messageBoxText length limit and what does it depend on (I could check that empirically on my computer, but would be conclusive for one OS/platform/.NET Framework version at best)?
Repro:
Here's a code which demonstrates the problem (it can be used with standard WPF Application template in Visual Studio).
MainWindow.xaml:
<Window x:Class="LongMessageInMessageBox.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Button Grid.Row="0" Grid.Column="0" Click="ShortMessage_Clicked">Short Message</Button>
<Button Grid.Row="1" Grid.Column="0" Click="LongMessage_Clicked">Long Message</Button>
</Grid>
MainWindow.xaml.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace LongMessageInMessageBox
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
/// Method which shows that displaying short message works correctly
private void ShortMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("ShortMessage_Clicked");
var result = MessageBox.Show("Short Message");
Console.WriteLine(result);
}
/// Method which shows that displaying a long message does not work
private void LongMessage_Clicked(object sender, RoutedEventArgs e)
{
Console.WriteLine("LongMessage_Clicked");
var longTextBuilder = new System.Text.StringBuilder(10000);
longTextBuilder.Append("Long Message n");
for (int i = 1; i <= 100000; i++)
{
longTextBuilder.Append(" ").Append(i);
}
var result = MessageBox.Show(longTextBuilder.ToString());
Console.WriteLine(result);
}
}
}
Sample from Output
window:
ShortMessage_Clicked
OK
ShortMessage_Clicked
OK
LongMessage_Clicked
No
The thread 0x55bc has exited with code 0 (0x0).
wpf messagebox
wpf messagebox
asked Nov 9 at 18:09


Tomasz Maczyński
743720
743720
My guess is that it fails silently as WPF does often. Did you check?
– Jan
Nov 9 at 18:15
add a comment |
My guess is that it fails silently as WPF does often. Did you check?
– Jan
Nov 9 at 18:15
My guess is that it fails silently as WPF does often. Did you check?
– Jan
Nov 9 at 18:15
My guess is that it fails silently as WPF does often. Did you check?
– Jan
Nov 9 at 18:15
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
MessageBox
class in WPF
(and WinForms) wraps the Win32 MessageBox
function, and doesn't throw an exception. In the old Win32 world error is reported by setting the last-error code, and if you don’t call GetLastError
to check, you can just keep going without knowing something is broken.
From Reference Source
//so it just translates the return code to a MessageBoxResult
MessageBoxResult result = Win32ToMessageBoxResult (UnsafeNativeMethods.MessageBox (new HandleRef (null, owner), messageBoxText, caption, style));
I have not tested the capacity of the lpText
string in the Win32 MessageBox function. But it is not surprising if the capacity limit is something like 1024
, 4096
or 65536
, after all, MessageBox
function is designed to display short message.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53231198%2fwpf-messagebox-is-not-displayed-at-all-if-long-message-is-passed%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
MessageBox
class in WPF
(and WinForms) wraps the Win32 MessageBox
function, and doesn't throw an exception. In the old Win32 world error is reported by setting the last-error code, and if you don’t call GetLastError
to check, you can just keep going without knowing something is broken.
From Reference Source
//so it just translates the return code to a MessageBoxResult
MessageBoxResult result = Win32ToMessageBoxResult (UnsafeNativeMethods.MessageBox (new HandleRef (null, owner), messageBoxText, caption, style));
I have not tested the capacity of the lpText
string in the Win32 MessageBox function. But it is not surprising if the capacity limit is something like 1024
, 4096
or 65536
, after all, MessageBox
function is designed to display short message.
add a comment |
up vote
1
down vote
MessageBox
class in WPF
(and WinForms) wraps the Win32 MessageBox
function, and doesn't throw an exception. In the old Win32 world error is reported by setting the last-error code, and if you don’t call GetLastError
to check, you can just keep going without knowing something is broken.
From Reference Source
//so it just translates the return code to a MessageBoxResult
MessageBoxResult result = Win32ToMessageBoxResult (UnsafeNativeMethods.MessageBox (new HandleRef (null, owner), messageBoxText, caption, style));
I have not tested the capacity of the lpText
string in the Win32 MessageBox function. But it is not surprising if the capacity limit is something like 1024
, 4096
or 65536
, after all, MessageBox
function is designed to display short message.
add a comment |
up vote
1
down vote
up vote
1
down vote
MessageBox
class in WPF
(and WinForms) wraps the Win32 MessageBox
function, and doesn't throw an exception. In the old Win32 world error is reported by setting the last-error code, and if you don’t call GetLastError
to check, you can just keep going without knowing something is broken.
From Reference Source
//so it just translates the return code to a MessageBoxResult
MessageBoxResult result = Win32ToMessageBoxResult (UnsafeNativeMethods.MessageBox (new HandleRef (null, owner), messageBoxText, caption, style));
I have not tested the capacity of the lpText
string in the Win32 MessageBox function. But it is not surprising if the capacity limit is something like 1024
, 4096
or 65536
, after all, MessageBox
function is designed to display short message.
MessageBox
class in WPF
(and WinForms) wraps the Win32 MessageBox
function, and doesn't throw an exception. In the old Win32 world error is reported by setting the last-error code, and if you don’t call GetLastError
to check, you can just keep going without knowing something is broken.
From Reference Source
//so it just translates the return code to a MessageBoxResult
MessageBoxResult result = Win32ToMessageBoxResult (UnsafeNativeMethods.MessageBox (new HandleRef (null, owner), messageBoxText, caption, style));
I have not tested the capacity of the lpText
string in the Win32 MessageBox function. But it is not surprising if the capacity limit is something like 1024
, 4096
or 65536
, after all, MessageBox
function is designed to display short message.
edited Nov 11 at 0:56
answered Nov 10 at 9:56


kennyzx
9,75542263
9,75542263
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53231198%2fwpf-messagebox-is-not-displayed-at-all-if-long-message-is-passed%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qEus2TdKKptu7HI Ks mqFr0jfaXO L tyfobfvllf4vF z o I2j6k1w1p9j2h
My guess is that it fails silently as WPF does often. Did you check?
– Jan
Nov 9 at 18:15