Create a random string each time a model object is saved to database
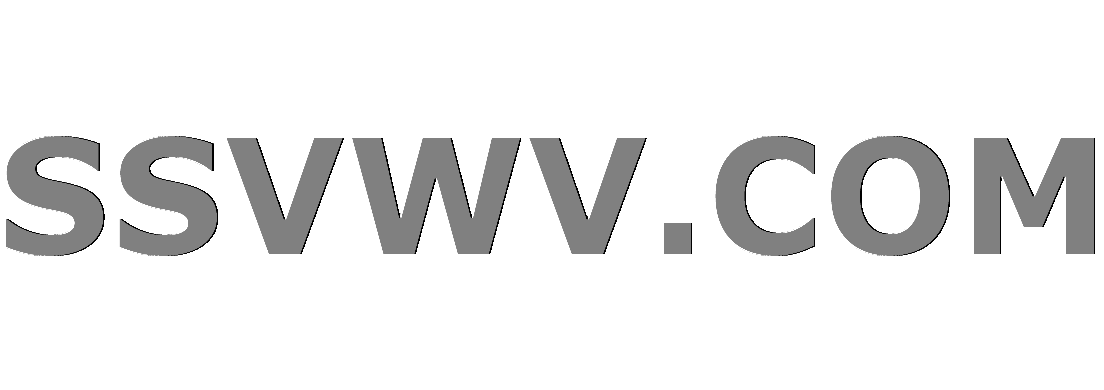
Multi tool use
up vote
0
down vote
favorite
In my models.py, I have:
def MakeOTP():
import random,string
return ''.join(random.choices(string.digits, k=4))
class Prescriptionshare(models.Model):
prid = models.AutoField(primary_key=True, unique=True)
customer = models.ForeignKey(
customer, on_delete=models.CASCADE, null=True)
time = models.DateTimeField(default=timezone.now)
checkin =models.ForeignKey(Checkins, on_delete=models.CASCADE, null=True)
otp = models.CharField(max_length=5, default=MakeOTP())
In my django shell, I've tried the following:
pq = Prescriptionshare(customer = cus, checkin = chk)
pq.save()
The problem is that each time this is executed, I get the same string in otp field. There is no random change of string.
Why is this happening?
django django-models
add a comment |
up vote
0
down vote
favorite
In my models.py, I have:
def MakeOTP():
import random,string
return ''.join(random.choices(string.digits, k=4))
class Prescriptionshare(models.Model):
prid = models.AutoField(primary_key=True, unique=True)
customer = models.ForeignKey(
customer, on_delete=models.CASCADE, null=True)
time = models.DateTimeField(default=timezone.now)
checkin =models.ForeignKey(Checkins, on_delete=models.CASCADE, null=True)
otp = models.CharField(max_length=5, default=MakeOTP())
In my django shell, I've tried the following:
pq = Prescriptionshare(customer = cus, checkin = chk)
pq.save()
The problem is that each time this is executed, I get the same string in otp field. There is no random change of string.
Why is this happening?
django django-models
Assuming the OTP is used for security, you must not use the random module. The Mersenne Twister algorithm is completely insecure for cryptographic purposes, with a relatively small sample of outputs, anyone can recover the internal state and predict future outputs. Use the secrets module ordjango.utils.crypto.get_random_string()
instead.
– knbk
Nov 7 at 13:18
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
In my models.py, I have:
def MakeOTP():
import random,string
return ''.join(random.choices(string.digits, k=4))
class Prescriptionshare(models.Model):
prid = models.AutoField(primary_key=True, unique=True)
customer = models.ForeignKey(
customer, on_delete=models.CASCADE, null=True)
time = models.DateTimeField(default=timezone.now)
checkin =models.ForeignKey(Checkins, on_delete=models.CASCADE, null=True)
otp = models.CharField(max_length=5, default=MakeOTP())
In my django shell, I've tried the following:
pq = Prescriptionshare(customer = cus, checkin = chk)
pq.save()
The problem is that each time this is executed, I get the same string in otp field. There is no random change of string.
Why is this happening?
django django-models
In my models.py, I have:
def MakeOTP():
import random,string
return ''.join(random.choices(string.digits, k=4))
class Prescriptionshare(models.Model):
prid = models.AutoField(primary_key=True, unique=True)
customer = models.ForeignKey(
customer, on_delete=models.CASCADE, null=True)
time = models.DateTimeField(default=timezone.now)
checkin =models.ForeignKey(Checkins, on_delete=models.CASCADE, null=True)
otp = models.CharField(max_length=5, default=MakeOTP())
In my django shell, I've tried the following:
pq = Prescriptionshare(customer = cus, checkin = chk)
pq.save()
The problem is that each time this is executed, I get the same string in otp field. There is no random change of string.
Why is this happening?
django django-models
django django-models
asked Nov 7 at 10:58
Joel G Mathew
1,84992643
1,84992643
Assuming the OTP is used for security, you must not use the random module. The Mersenne Twister algorithm is completely insecure for cryptographic purposes, with a relatively small sample of outputs, anyone can recover the internal state and predict future outputs. Use the secrets module ordjango.utils.crypto.get_random_string()
instead.
– knbk
Nov 7 at 13:18
add a comment |
Assuming the OTP is used for security, you must not use the random module. The Mersenne Twister algorithm is completely insecure for cryptographic purposes, with a relatively small sample of outputs, anyone can recover the internal state and predict future outputs. Use the secrets module ordjango.utils.crypto.get_random_string()
instead.
– knbk
Nov 7 at 13:18
Assuming the OTP is used for security, you must not use the random module. The Mersenne Twister algorithm is completely insecure for cryptographic purposes, with a relatively small sample of outputs, anyone can recover the internal state and predict future outputs. Use the secrets module or
django.utils.crypto.get_random_string()
instead.– knbk
Nov 7 at 13:18
Assuming the OTP is used for security, you must not use the random module. The Mersenne Twister algorithm is completely insecure for cryptographic purposes, with a relatively small sample of outputs, anyone can recover the internal state and predict future outputs. Use the secrets module or
django.utils.crypto.get_random_string()
instead.– knbk
Nov 7 at 13:18
add a comment |
1 Answer
1
active
oldest
votes
up vote
5
down vote
accepted
remove the ()
from default=MakeOTP()
class Prescriptionshare(models.Model):
# your code
otp = models.CharField(max_length=5, default=MakeOTP) # here, remove the "()"
After making changes in the models, you should migrate the DB
Why this happening?
If you use MakeOTP()
, Django takes the output of the function, where as if you use MakeOTP
(without parenthesis) Django consider it as callable function.
That is, when paranthesis used, method is called once the migrations are run and its value is used as the default value and when paranthesis are not used, function reference called everytime while object creation.
1
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
accepted
remove the ()
from default=MakeOTP()
class Prescriptionshare(models.Model):
# your code
otp = models.CharField(max_length=5, default=MakeOTP) # here, remove the "()"
After making changes in the models, you should migrate the DB
Why this happening?
If you use MakeOTP()
, Django takes the output of the function, where as if you use MakeOTP
(without parenthesis) Django consider it as callable function.
That is, when paranthesis used, method is called once the migrations are run and its value is used as the default value and when paranthesis are not used, function reference called everytime while object creation.
1
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
add a comment |
up vote
5
down vote
accepted
remove the ()
from default=MakeOTP()
class Prescriptionshare(models.Model):
# your code
otp = models.CharField(max_length=5, default=MakeOTP) # here, remove the "()"
After making changes in the models, you should migrate the DB
Why this happening?
If you use MakeOTP()
, Django takes the output of the function, where as if you use MakeOTP
(without parenthesis) Django consider it as callable function.
That is, when paranthesis used, method is called once the migrations are run and its value is used as the default value and when paranthesis are not used, function reference called everytime while object creation.
1
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
add a comment |
up vote
5
down vote
accepted
up vote
5
down vote
accepted
remove the ()
from default=MakeOTP()
class Prescriptionshare(models.Model):
# your code
otp = models.CharField(max_length=5, default=MakeOTP) # here, remove the "()"
After making changes in the models, you should migrate the DB
Why this happening?
If you use MakeOTP()
, Django takes the output of the function, where as if you use MakeOTP
(without parenthesis) Django consider it as callable function.
That is, when paranthesis used, method is called once the migrations are run and its value is used as the default value and when paranthesis are not used, function reference called everytime while object creation.
remove the ()
from default=MakeOTP()
class Prescriptionshare(models.Model):
# your code
otp = models.CharField(max_length=5, default=MakeOTP) # here, remove the "()"
After making changes in the models, you should migrate the DB
Why this happening?
If you use MakeOTP()
, Django takes the output of the function, where as if you use MakeOTP
(without parenthesis) Django consider it as callable function.
That is, when paranthesis used, method is called once the migrations are run and its value is used as the default value and when paranthesis are not used, function reference called everytime while object creation.
edited Nov 7 at 11:08
answered Nov 7 at 10:59


JPG
11.9k2829
11.9k2829
1
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
add a comment |
1
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
1
1
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
To clarify the situation here, when paranthesis used, method is called once the migrations are run and its value is used as the default value, you need to pass it as a function reference for it to be called everytime
– Ozgur Akcali
Nov 7 at 11:04
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
@OzgurAkcali Thanks for your tip :)
– JPG
Nov 7 at 11:09
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53188138%2fcreate-a-random-string-each-time-a-model-object-is-saved-to-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Dg RJgkfn jzXdMEarT
Assuming the OTP is used for security, you must not use the random module. The Mersenne Twister algorithm is completely insecure for cryptographic purposes, with a relatively small sample of outputs, anyone can recover the internal state and predict future outputs. Use the secrets module or
django.utils.crypto.get_random_string()
instead.– knbk
Nov 7 at 13:18