OOP Javascript, get attribute of class not the event e
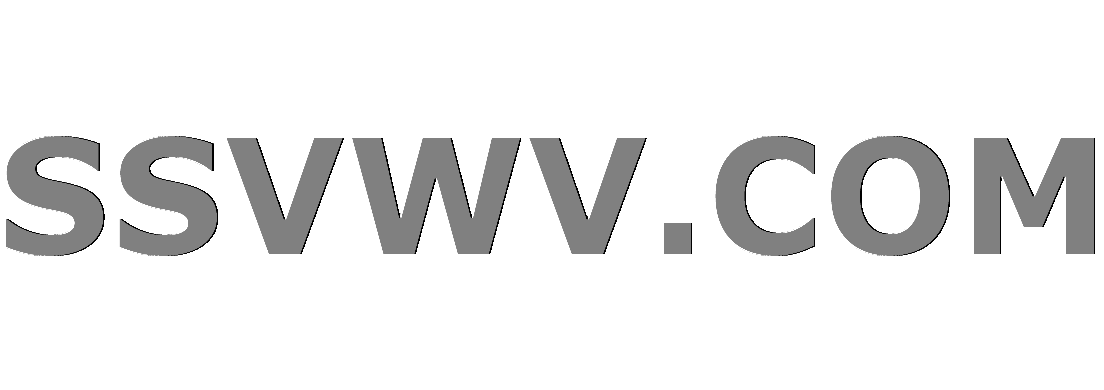
Multi tool use
In a event handler from my custom class, I want to use an attribute of my class in a private event method. Its more clearly with this simple example :
class myClass {
constructor() {
this._customAtt = "hello";
}
foo() {
this._addListeners();
}
_addListeners() {
$("input.two").click(this._anEvent);
}
_anEvent(e) {
console.log(this._customAtt); // print 'undefined'
console.log(self._customAtt); // print 'undefined'
}
}
var myIClass = new myClass();
$('input.one').on("click", function() {
myIClass.foo();
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<input type='button' value='one' class='one'>
<input type='button' value='two' class='two'>
When I click on the button one, I add an handler to the button two. If I click on the button two the method _anEvent
is called and from this method, I want use the private attribute class _customAtt
. But, in the _anEvent
method, this
is the event e, not my class.
So, how can I can the attribute _customAtt
from the method _anEvent
?
javascript oop
add a comment |
In a event handler from my custom class, I want to use an attribute of my class in a private event method. Its more clearly with this simple example :
class myClass {
constructor() {
this._customAtt = "hello";
}
foo() {
this._addListeners();
}
_addListeners() {
$("input.two").click(this._anEvent);
}
_anEvent(e) {
console.log(this._customAtt); // print 'undefined'
console.log(self._customAtt); // print 'undefined'
}
}
var myIClass = new myClass();
$('input.one').on("click", function() {
myIClass.foo();
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<input type='button' value='one' class='one'>
<input type='button' value='two' class='two'>
When I click on the button one, I add an handler to the button two. If I click on the button two the method _anEvent
is called and from this method, I want use the private attribute class _customAtt
. But, in the _anEvent
method, this
is the event e, not my class.
So, how can I can the attribute _customAtt
from the method _anEvent
?
javascript oop
add a comment |
In a event handler from my custom class, I want to use an attribute of my class in a private event method. Its more clearly with this simple example :
class myClass {
constructor() {
this._customAtt = "hello";
}
foo() {
this._addListeners();
}
_addListeners() {
$("input.two").click(this._anEvent);
}
_anEvent(e) {
console.log(this._customAtt); // print 'undefined'
console.log(self._customAtt); // print 'undefined'
}
}
var myIClass = new myClass();
$('input.one').on("click", function() {
myIClass.foo();
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<input type='button' value='one' class='one'>
<input type='button' value='two' class='two'>
When I click on the button one, I add an handler to the button two. If I click on the button two the method _anEvent
is called and from this method, I want use the private attribute class _customAtt
. But, in the _anEvent
method, this
is the event e, not my class.
So, how can I can the attribute _customAtt
from the method _anEvent
?
javascript oop
In a event handler from my custom class, I want to use an attribute of my class in a private event method. Its more clearly with this simple example :
class myClass {
constructor() {
this._customAtt = "hello";
}
foo() {
this._addListeners();
}
_addListeners() {
$("input.two").click(this._anEvent);
}
_anEvent(e) {
console.log(this._customAtt); // print 'undefined'
console.log(self._customAtt); // print 'undefined'
}
}
var myIClass = new myClass();
$('input.one').on("click", function() {
myIClass.foo();
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<input type='button' value='one' class='one'>
<input type='button' value='two' class='two'>
When I click on the button one, I add an handler to the button two. If I click on the button two the method _anEvent
is called and from this method, I want use the private attribute class _customAtt
. But, in the _anEvent
method, this
is the event e, not my class.
So, how can I can the attribute _customAtt
from the method _anEvent
?
class myClass {
constructor() {
this._customAtt = "hello";
}
foo() {
this._addListeners();
}
_addListeners() {
$("input.two").click(this._anEvent);
}
_anEvent(e) {
console.log(this._customAtt); // print 'undefined'
console.log(self._customAtt); // print 'undefined'
}
}
var myIClass = new myClass();
$('input.one').on("click", function() {
myIClass.foo();
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<input type='button' value='one' class='one'>
<input type='button' value='two' class='two'>
class myClass {
constructor() {
this._customAtt = "hello";
}
foo() {
this._addListeners();
}
_addListeners() {
$("input.two").click(this._anEvent);
}
_anEvent(e) {
console.log(this._customAtt); // print 'undefined'
console.log(self._customAtt); // print 'undefined'
}
}
var myIClass = new myClass();
$('input.one').on("click", function() {
myIClass.foo();
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<input type='button' value='one' class='one'>
<input type='button' value='two' class='two'>
javascript oop
javascript oop
edited Nov 14 '18 at 9:00


Zlytherin
1,5441526
1,5441526
asked Nov 14 '18 at 8:54
user2137454user2137454
184632
184632
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The reason for this is because the context of this
varies depending on how the function is called, not what the function was originally attached to.
One relatively easy way to do this is to bind _anEvent
to the instance of the class.
constructor() {
this._customAtt = "hello";
this._anEvent = this._anEvent.bind(this);
}
This will ensure that this
is always the instance of myClass
within the _anEvent
function.
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
@user2137454bind
will still work with anything created dynamically
– Ian
Nov 14 '18 at 9:01
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
1
You'll need to change the way you handle the event so you can passthis
(being the event) through to your handler.$("input.two").click(this._anEvent);
would becomeconst self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296243%2foop-javascript-get-attribute-of-class-not-the-event-e%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The reason for this is because the context of this
varies depending on how the function is called, not what the function was originally attached to.
One relatively easy way to do this is to bind _anEvent
to the instance of the class.
constructor() {
this._customAtt = "hello";
this._anEvent = this._anEvent.bind(this);
}
This will ensure that this
is always the instance of myClass
within the _anEvent
function.
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
@user2137454bind
will still work with anything created dynamically
– Ian
Nov 14 '18 at 9:01
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
1
You'll need to change the way you handle the event so you can passthis
(being the event) through to your handler.$("input.two").click(this._anEvent);
would becomeconst self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
add a comment |
The reason for this is because the context of this
varies depending on how the function is called, not what the function was originally attached to.
One relatively easy way to do this is to bind _anEvent
to the instance of the class.
constructor() {
this._customAtt = "hello";
this._anEvent = this._anEvent.bind(this);
}
This will ensure that this
is always the instance of myClass
within the _anEvent
function.
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
@user2137454bind
will still work with anything created dynamically
– Ian
Nov 14 '18 at 9:01
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
1
You'll need to change the way you handle the event so you can passthis
(being the event) through to your handler.$("input.two").click(this._anEvent);
would becomeconst self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
add a comment |
The reason for this is because the context of this
varies depending on how the function is called, not what the function was originally attached to.
One relatively easy way to do this is to bind _anEvent
to the instance of the class.
constructor() {
this._customAtt = "hello";
this._anEvent = this._anEvent.bind(this);
}
This will ensure that this
is always the instance of myClass
within the _anEvent
function.
The reason for this is because the context of this
varies depending on how the function is called, not what the function was originally attached to.
One relatively easy way to do this is to bind _anEvent
to the instance of the class.
constructor() {
this._customAtt = "hello";
this._anEvent = this._anEvent.bind(this);
}
This will ensure that this
is always the instance of myClass
within the _anEvent
function.
answered Nov 14 '18 at 8:56
IanIan
24.3k1781153
24.3k1781153
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
@user2137454bind
will still work with anything created dynamically
– Ian
Nov 14 '18 at 9:01
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
1
You'll need to change the way you handle the event so you can passthis
(being the event) through to your handler.$("input.two").click(this._anEvent);
would becomeconst self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
add a comment |
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
@user2137454bind
will still work with anything created dynamically
– Ian
Nov 14 '18 at 9:01
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
1
You'll need to change the way you handle the event so you can passthis
(being the event) through to your handler.$("input.two").click(this._anEvent);
would becomeconst self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
I'm sorry, I show a simple example but in my case, I create dynamically html content with some methods in my class, and finally, I bind to theses news generated elements the function '_anEvent'
– user2137454
Nov 14 '18 at 8:59
@user2137454
bind
will still work with anything created dynamically– Ian
Nov 14 '18 at 9:01
@user2137454
bind
will still work with anything created dynamically– Ian
Nov 14 '18 at 9:01
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
I'll try that...and, from the _anEvent method, if I want use 'this' as the instance of my class and 'this' as the event catched by my handler, how can I do that ?
– user2137454
Nov 14 '18 at 9:03
1
1
You'll need to change the way you handle the event so you can pass
this
(being the event) through to your handler. $("input.two").click(this._anEvent);
would become const self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
You'll need to change the way you handle the event so you can pass
this
(being the event) through to your handler. $("input.two").click(this._anEvent);
would become const self = this; $("input.two").click(() => { self._anEvent(this); });
– Ian
Nov 14 '18 at 9:23
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
thank you @ian :)
– user2137454
Nov 14 '18 at 9:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296243%2foop-javascript-get-attribute-of-class-not-the-event-e%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sCdbxybWCo CnlP1NpJLY1 4EstSkIfBzhip,XI0cR8eJVy64mhc1 DWneJ iY0majLXf,EECWPpdD 71Em,tJ7 DOx8QXuC3ShDx7aHW K,LX