CompletableFuture allOf method behavior
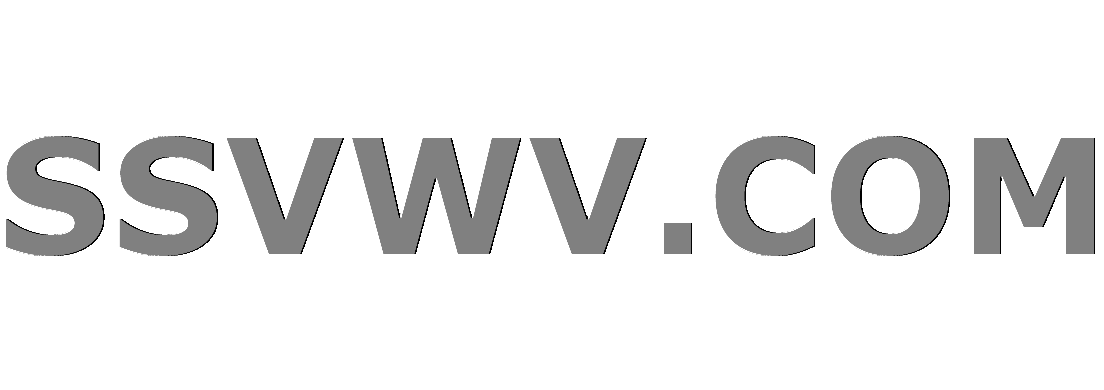
Multi tool use
I have the following piece of java code:
CompletableFuture<String> future1 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 1";
});
CompletableFuture<String> future2 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 2";
});
CompletableFuture<String> future3 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 3";
});
boolean isdone = CompletableFuture.allOf(future1, future2, future3).isDone();
if (isdone) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
When I run this code it always prints "Futures are not ready". I am using allOf method here which should wait for all the futures to get completed, but the main thread is not waiting here and priniting the else part. Can someone please help me understand what is going wrong here?
java completable-future
add a comment |
I have the following piece of java code:
CompletableFuture<String> future1 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 1";
});
CompletableFuture<String> future2 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 2";
});
CompletableFuture<String> future3 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 3";
});
boolean isdone = CompletableFuture.allOf(future1, future2, future3).isDone();
if (isdone) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
When I run this code it always prints "Futures are not ready". I am using allOf method here which should wait for all the futures to get completed, but the main thread is not waiting here and priniting the else part. Can someone please help me understand what is going wrong here?
java completable-future
add a comment |
I have the following piece of java code:
CompletableFuture<String> future1 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 1";
});
CompletableFuture<String> future2 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 2";
});
CompletableFuture<String> future3 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 3";
});
boolean isdone = CompletableFuture.allOf(future1, future2, future3).isDone();
if (isdone) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
When I run this code it always prints "Futures are not ready". I am using allOf method here which should wait for all the futures to get completed, but the main thread is not waiting here and priniting the else part. Can someone please help me understand what is going wrong here?
java completable-future
I have the following piece of java code:
CompletableFuture<String> future1 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 1";
});
CompletableFuture<String> future2 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 2";
});
CompletableFuture<String> future3 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(3);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of Future 3";
});
boolean isdone = CompletableFuture.allOf(future1, future2, future3).isDone();
if (isdone) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
When I run this code it always prints "Futures are not ready". I am using allOf method here which should wait for all the futures to get completed, but the main thread is not waiting here and priniting the else part. Can someone please help me understand what is going wrong here?
java completable-future
java completable-future
asked Nov 20 '18 at 10:34
Abhilash28AbhiAbhilash28Abhi
1108
1108
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I am using allOf method here which should wait for all the futures to get completed
That's not what allOf
does. It creates a new CompletableFuture
that is completed when all of the given CompletableFutures complete
. It does not, however, wait for that new CompletableFuture
to complete.
This means that you should call some method that waits for this CompletableFuture
to be completed, at which point all the given CompletableFuture
s are guaranteed to be complete.
For example:
CompletableFuture<Void> allof = CompletableFuture.allOf(future1, future2, future3);
allof.get();
if (allof.isDone ()) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
Output:
Future result Result of Future 1 | Result of Future 2 | Result of Future 3
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
@Abhilash28Abhi you have to wait for it to complete by calling some of theCompletableFuture
methods that does that (such asget()
). Just creating theCompletableFuture
doesn't wait for it to complete. Just like creating the original 3CompletableFuture
s doesn't wait for them to complete.
– Eran
Nov 20 '18 at 11:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391071%2fcompletablefuture-allof-method-behavior%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I am using allOf method here which should wait for all the futures to get completed
That's not what allOf
does. It creates a new CompletableFuture
that is completed when all of the given CompletableFutures complete
. It does not, however, wait for that new CompletableFuture
to complete.
This means that you should call some method that waits for this CompletableFuture
to be completed, at which point all the given CompletableFuture
s are guaranteed to be complete.
For example:
CompletableFuture<Void> allof = CompletableFuture.allOf(future1, future2, future3);
allof.get();
if (allof.isDone ()) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
Output:
Future result Result of Future 1 | Result of Future 2 | Result of Future 3
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
@Abhilash28Abhi you have to wait for it to complete by calling some of theCompletableFuture
methods that does that (such asget()
). Just creating theCompletableFuture
doesn't wait for it to complete. Just like creating the original 3CompletableFuture
s doesn't wait for them to complete.
– Eran
Nov 20 '18 at 11:18
add a comment |
I am using allOf method here which should wait for all the futures to get completed
That's not what allOf
does. It creates a new CompletableFuture
that is completed when all of the given CompletableFutures complete
. It does not, however, wait for that new CompletableFuture
to complete.
This means that you should call some method that waits for this CompletableFuture
to be completed, at which point all the given CompletableFuture
s are guaranteed to be complete.
For example:
CompletableFuture<Void> allof = CompletableFuture.allOf(future1, future2, future3);
allof.get();
if (allof.isDone ()) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
Output:
Future result Result of Future 1 | Result of Future 2 | Result of Future 3
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
@Abhilash28Abhi you have to wait for it to complete by calling some of theCompletableFuture
methods that does that (such asget()
). Just creating theCompletableFuture
doesn't wait for it to complete. Just like creating the original 3CompletableFuture
s doesn't wait for them to complete.
– Eran
Nov 20 '18 at 11:18
add a comment |
I am using allOf method here which should wait for all the futures to get completed
That's not what allOf
does. It creates a new CompletableFuture
that is completed when all of the given CompletableFutures complete
. It does not, however, wait for that new CompletableFuture
to complete.
This means that you should call some method that waits for this CompletableFuture
to be completed, at which point all the given CompletableFuture
s are guaranteed to be complete.
For example:
CompletableFuture<Void> allof = CompletableFuture.allOf(future1, future2, future3);
allof.get();
if (allof.isDone ()) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
Output:
Future result Result of Future 1 | Result of Future 2 | Result of Future 3
I am using allOf method here which should wait for all the futures to get completed
That's not what allOf
does. It creates a new CompletableFuture
that is completed when all of the given CompletableFutures complete
. It does not, however, wait for that new CompletableFuture
to complete.
This means that you should call some method that waits for this CompletableFuture
to be completed, at which point all the given CompletableFuture
s are guaranteed to be complete.
For example:
CompletableFuture<Void> allof = CompletableFuture.allOf(future1, future2, future3);
allof.get();
if (allof.isDone ()) {
System.out.println("Future result " + future1.get() + " | " + future2.get() + " | " + future3.get());
} else {
System.out.println("Futures are not ready");
}
Output:
Future result Result of Future 1 | Result of Future 2 | Result of Future 3
answered Nov 20 '18 at 10:42


EranEran
286k37467555
286k37467555
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
@Abhilash28Abhi you have to wait for it to complete by calling some of theCompletableFuture
methods that does that (such asget()
). Just creating theCompletableFuture
doesn't wait for it to complete. Just like creating the original 3CompletableFuture
s doesn't wait for them to complete.
– Eran
Nov 20 '18 at 11:18
add a comment |
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
@Abhilash28Abhi you have to wait for it to complete by calling some of theCompletableFuture
methods that does that (such asget()
). Just creating theCompletableFuture
doesn't wait for it to complete. Just like creating the original 3CompletableFuture
s doesn't wait for them to complete.
– Eran
Nov 20 '18 at 11:18
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
This works but I am still not clear on the behavior of allOf(). You said - It creates a new CompletableFuture that is completed when all of the given CompletableFutures complete. So how do we know that the new CompletableFuture is completed? Does this not mean we can make use of it rather than waiting for it to complete
– Abhilash28Abhi
Nov 20 '18 at 11:06
@Abhilash28Abhi you have to wait for it to complete by calling some of the
CompletableFuture
methods that does that (such as get()
). Just creating the CompletableFuture
doesn't wait for it to complete. Just like creating the original 3 CompletableFuture
s doesn't wait for them to complete.– Eran
Nov 20 '18 at 11:18
@Abhilash28Abhi you have to wait for it to complete by calling some of the
CompletableFuture
methods that does that (such as get()
). Just creating the CompletableFuture
doesn't wait for it to complete. Just like creating the original 3 CompletableFuture
s doesn't wait for them to complete.– Eran
Nov 20 '18 at 11:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391071%2fcompletablefuture-allof-method-behavior%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e4iYIKaFZIc,fwQ6,m vMUPS5I9mr56,uvQtrFg5 GFasZOUZzOD,PMTQEq9z,hWq