creating a 3xX array in pandas Dataframe Python
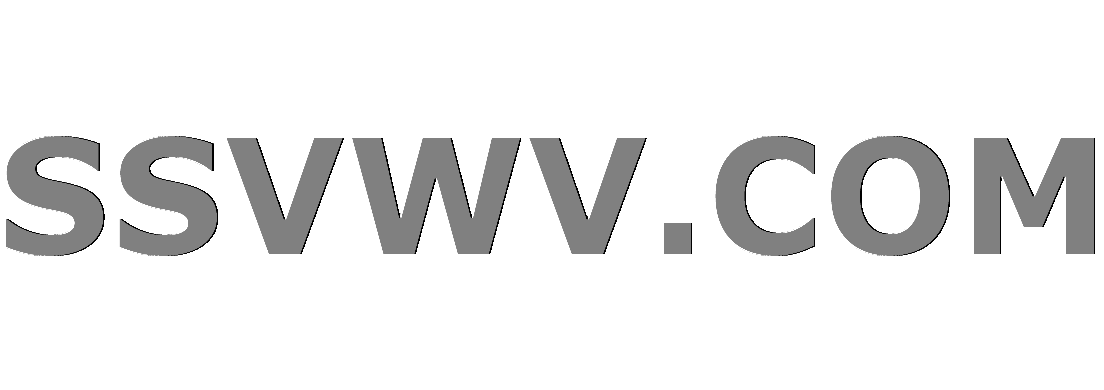
Multi tool use
#!/usr/bin/env python
from pandas import DataFrame, Series
from simple_salesforce import Salesforce
from config import *
qry_result = sf.query("SELECT Phone, Name, Account.Name FROM Contact")
logging.debug('SF QueryResult TotalSize {0}'.format(qry_result['totalSize']))
df = DataFrame(qry_result['records'])
is_done = qry_result['done'];
while not is_done:
try:
qry_result = sf.query_more(qry_result['nextRecordsUrl'], True)
df = df.append(DataFrame(qry_result['records']))
if qry_result['done']:
is_done = True;
logging.debug('SF completed')
except NameError as e:
logging.error('SOQL failed', e)
SystemExit(17)
df = df.drop('attributes', axis=1)
return df
def extract_numbers_and_canonicalize(df):
phone_numbers =
account_numbers =
name =
for row in df.itertuples():
print(row)
logging.info(row)
phone = extract(row, 'Phone' + ';')
if phone:
phone_numbers.append(phone)
account_numbers = extract(row, 'account_numbers' + ';')
if account_numbers:
account_numbers.append(account_numbers)
name = extract(row, 'name')
if name:
name.append(name)
header = [phone_numbers, account_numbers, name]
series = Series(data=header)
unique_series = series.unique()
return DataFrame(data=unique_series, columns=['numbers'';''names' ';' 'accountnames'])
def extract(tuple, column):
if not hasattr(tuple, column):
return None
value = getattr(tuple, column)
if not value:
return None
return canonicalize(value)
def query_contacts_and_write_csv():
contacts = query_sf_contacts()
numbers = extract_numbers_and_canonicalize(contacts)
DataFrame.to_csv(numbers, encoding='utf-8', path_or_buf=CSV_RESULT_FILE, index=False)
query_contacts_and_write_csv()
Hello Python People,
maybe someone can help me with my problem.
I would like to create the csv file with 3 arrays.
I assume that i have to change the unique_series
to something else.
Does this even work with the approach iam using (If I only take the phone numbers for example it works just fine).
Thanks in advance for the Tips.
python pandas dataframe
add a comment |
#!/usr/bin/env python
from pandas import DataFrame, Series
from simple_salesforce import Salesforce
from config import *
qry_result = sf.query("SELECT Phone, Name, Account.Name FROM Contact")
logging.debug('SF QueryResult TotalSize {0}'.format(qry_result['totalSize']))
df = DataFrame(qry_result['records'])
is_done = qry_result['done'];
while not is_done:
try:
qry_result = sf.query_more(qry_result['nextRecordsUrl'], True)
df = df.append(DataFrame(qry_result['records']))
if qry_result['done']:
is_done = True;
logging.debug('SF completed')
except NameError as e:
logging.error('SOQL failed', e)
SystemExit(17)
df = df.drop('attributes', axis=1)
return df
def extract_numbers_and_canonicalize(df):
phone_numbers =
account_numbers =
name =
for row in df.itertuples():
print(row)
logging.info(row)
phone = extract(row, 'Phone' + ';')
if phone:
phone_numbers.append(phone)
account_numbers = extract(row, 'account_numbers' + ';')
if account_numbers:
account_numbers.append(account_numbers)
name = extract(row, 'name')
if name:
name.append(name)
header = [phone_numbers, account_numbers, name]
series = Series(data=header)
unique_series = series.unique()
return DataFrame(data=unique_series, columns=['numbers'';''names' ';' 'accountnames'])
def extract(tuple, column):
if not hasattr(tuple, column):
return None
value = getattr(tuple, column)
if not value:
return None
return canonicalize(value)
def query_contacts_and_write_csv():
contacts = query_sf_contacts()
numbers = extract_numbers_and_canonicalize(contacts)
DataFrame.to_csv(numbers, encoding='utf-8', path_or_buf=CSV_RESULT_FILE, index=False)
query_contacts_and_write_csv()
Hello Python People,
maybe someone can help me with my problem.
I would like to create the csv file with 3 arrays.
I assume that i have to change the unique_series
to something else.
Does this even work with the approach iam using (If I only take the phone numbers for example it works just fine).
Thanks in advance for the Tips.
python pandas dataframe
1
If you could provide sample data, and a requested output it would be much easier to provide an answer for your question.
– johnnyb
Nov 20 '18 at 16:49
Hello, the Output looks like this.Phone=u'$PhoneNumber', Name=$FirstName $LastName', (u'Account_Name', $CompanyName'))
The Output should be a ; seperated csv file. With Name; Phone; CompanyName
– prax0r
Nov 21 '18 at 8:08
add a comment |
#!/usr/bin/env python
from pandas import DataFrame, Series
from simple_salesforce import Salesforce
from config import *
qry_result = sf.query("SELECT Phone, Name, Account.Name FROM Contact")
logging.debug('SF QueryResult TotalSize {0}'.format(qry_result['totalSize']))
df = DataFrame(qry_result['records'])
is_done = qry_result['done'];
while not is_done:
try:
qry_result = sf.query_more(qry_result['nextRecordsUrl'], True)
df = df.append(DataFrame(qry_result['records']))
if qry_result['done']:
is_done = True;
logging.debug('SF completed')
except NameError as e:
logging.error('SOQL failed', e)
SystemExit(17)
df = df.drop('attributes', axis=1)
return df
def extract_numbers_and_canonicalize(df):
phone_numbers =
account_numbers =
name =
for row in df.itertuples():
print(row)
logging.info(row)
phone = extract(row, 'Phone' + ';')
if phone:
phone_numbers.append(phone)
account_numbers = extract(row, 'account_numbers' + ';')
if account_numbers:
account_numbers.append(account_numbers)
name = extract(row, 'name')
if name:
name.append(name)
header = [phone_numbers, account_numbers, name]
series = Series(data=header)
unique_series = series.unique()
return DataFrame(data=unique_series, columns=['numbers'';''names' ';' 'accountnames'])
def extract(tuple, column):
if not hasattr(tuple, column):
return None
value = getattr(tuple, column)
if not value:
return None
return canonicalize(value)
def query_contacts_and_write_csv():
contacts = query_sf_contacts()
numbers = extract_numbers_and_canonicalize(contacts)
DataFrame.to_csv(numbers, encoding='utf-8', path_or_buf=CSV_RESULT_FILE, index=False)
query_contacts_and_write_csv()
Hello Python People,
maybe someone can help me with my problem.
I would like to create the csv file with 3 arrays.
I assume that i have to change the unique_series
to something else.
Does this even work with the approach iam using (If I only take the phone numbers for example it works just fine).
Thanks in advance for the Tips.
python pandas dataframe
#!/usr/bin/env python
from pandas import DataFrame, Series
from simple_salesforce import Salesforce
from config import *
qry_result = sf.query("SELECT Phone, Name, Account.Name FROM Contact")
logging.debug('SF QueryResult TotalSize {0}'.format(qry_result['totalSize']))
df = DataFrame(qry_result['records'])
is_done = qry_result['done'];
while not is_done:
try:
qry_result = sf.query_more(qry_result['nextRecordsUrl'], True)
df = df.append(DataFrame(qry_result['records']))
if qry_result['done']:
is_done = True;
logging.debug('SF completed')
except NameError as e:
logging.error('SOQL failed', e)
SystemExit(17)
df = df.drop('attributes', axis=1)
return df
def extract_numbers_and_canonicalize(df):
phone_numbers =
account_numbers =
name =
for row in df.itertuples():
print(row)
logging.info(row)
phone = extract(row, 'Phone' + ';')
if phone:
phone_numbers.append(phone)
account_numbers = extract(row, 'account_numbers' + ';')
if account_numbers:
account_numbers.append(account_numbers)
name = extract(row, 'name')
if name:
name.append(name)
header = [phone_numbers, account_numbers, name]
series = Series(data=header)
unique_series = series.unique()
return DataFrame(data=unique_series, columns=['numbers'';''names' ';' 'accountnames'])
def extract(tuple, column):
if not hasattr(tuple, column):
return None
value = getattr(tuple, column)
if not value:
return None
return canonicalize(value)
def query_contacts_and_write_csv():
contacts = query_sf_contacts()
numbers = extract_numbers_and_canonicalize(contacts)
DataFrame.to_csv(numbers, encoding='utf-8', path_or_buf=CSV_RESULT_FILE, index=False)
query_contacts_and_write_csv()
Hello Python People,
maybe someone can help me with my problem.
I would like to create the csv file with 3 arrays.
I assume that i have to change the unique_series
to something else.
Does this even work with the approach iam using (If I only take the phone numbers for example it works just fine).
Thanks in advance for the Tips.
python pandas dataframe
python pandas dataframe
asked Nov 20 '18 at 16:01
prax0rprax0r
314
314
1
If you could provide sample data, and a requested output it would be much easier to provide an answer for your question.
– johnnyb
Nov 20 '18 at 16:49
Hello, the Output looks like this.Phone=u'$PhoneNumber', Name=$FirstName $LastName', (u'Account_Name', $CompanyName'))
The Output should be a ; seperated csv file. With Name; Phone; CompanyName
– prax0r
Nov 21 '18 at 8:08
add a comment |
1
If you could provide sample data, and a requested output it would be much easier to provide an answer for your question.
– johnnyb
Nov 20 '18 at 16:49
Hello, the Output looks like this.Phone=u'$PhoneNumber', Name=$FirstName $LastName', (u'Account_Name', $CompanyName'))
The Output should be a ; seperated csv file. With Name; Phone; CompanyName
– prax0r
Nov 21 '18 at 8:08
1
1
If you could provide sample data, and a requested output it would be much easier to provide an answer for your question.
– johnnyb
Nov 20 '18 at 16:49
If you could provide sample data, and a requested output it would be much easier to provide an answer for your question.
– johnnyb
Nov 20 '18 at 16:49
Hello, the Output looks like this.
Phone=u'$PhoneNumber', Name=$FirstName $LastName', (u'Account_Name', $CompanyName'))
The Output should be a ; seperated csv file. With Name; Phone; CompanyName– prax0r
Nov 21 '18 at 8:08
Hello, the Output looks like this.
Phone=u'$PhoneNumber', Name=$FirstName $LastName', (u'Account_Name', $CompanyName'))
The Output should be a ; seperated csv file. With Name; Phone; CompanyName– prax0r
Nov 21 '18 at 8:08
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53396914%2fcreating-a-3xx-array-in-pandas-dataframe-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53396914%2fcreating-a-3xx-array-in-pandas-dataframe-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pfpiu9qXeUIgF8huueqQYcvN MlacTxGR 5tUzQAFizPA YsU,f,a1pXjWpzTwvQfd0o4nAClaYMcG7Xe,TF81fh lx
1
If you could provide sample data, and a requested output it would be much easier to provide an answer for your question.
– johnnyb
Nov 20 '18 at 16:49
Hello, the Output looks like this.
Phone=u'$PhoneNumber', Name=$FirstName $LastName', (u'Account_Name', $CompanyName'))
The Output should be a ; seperated csv file. With Name; Phone; CompanyName– prax0r
Nov 21 '18 at 8:08