Iterator subclass separate inclusion
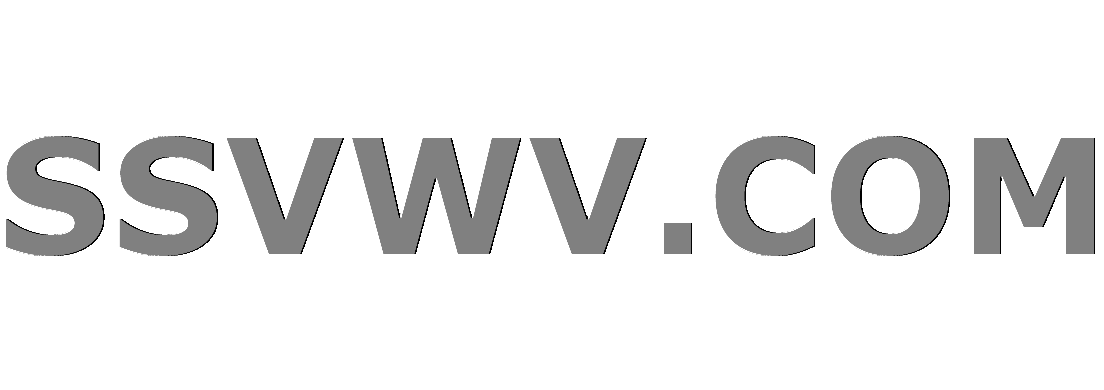
Multi tool use
I'm new to the iterator class; not in how to use iterators for containers but how to implement an iterator class. I have two files, a .h and .cpp. Right now, I'm getting a compiler error in my .cpp file that displays "fatal error: 'iterator' is not a class, namespace, or enumeration". I don't understand why its telling me this.
Note: I'm sure there are other errors in my code, but right now I'm just trying to get the existing compiler error resolved.
Base.h
#ifndef Base_h
#define Base_h
class Base {
protected:
std::vector<std::vector<std::string> > vec;
public:
class iterator {
private:
Base* p = nullptr;
size_t x, y;
public:
iterator() = default;
iterator(Base *, size_t, size_t);
~iterator();
iterator &operator++();
Base operator*() const;
}
iterator begin() const;
iterator end() const;
// bunch of other iterator::functions and Base::functions
};
#endif
Base.cpp
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
iterator::~iterator();
// Bunch of other iterator::functions including Base::functions
Compiler Error
Base.cc:254:13: fatal error: 'iterator is not a class, namespace, or enumeration
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
^
/Library/Developer/CommandLineTools/usr/include/c++/v1/iterator:522:29: note: 'iterator' declared here
struct _LIBCPP_TEMPLATE_VIS iterator
c++ iterator
add a comment |
I'm new to the iterator class; not in how to use iterators for containers but how to implement an iterator class. I have two files, a .h and .cpp. Right now, I'm getting a compiler error in my .cpp file that displays "fatal error: 'iterator' is not a class, namespace, or enumeration". I don't understand why its telling me this.
Note: I'm sure there are other errors in my code, but right now I'm just trying to get the existing compiler error resolved.
Base.h
#ifndef Base_h
#define Base_h
class Base {
protected:
std::vector<std::vector<std::string> > vec;
public:
class iterator {
private:
Base* p = nullptr;
size_t x, y;
public:
iterator() = default;
iterator(Base *, size_t, size_t);
~iterator();
iterator &operator++();
Base operator*() const;
}
iterator begin() const;
iterator end() const;
// bunch of other iterator::functions and Base::functions
};
#endif
Base.cpp
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
iterator::~iterator();
// Bunch of other iterator::functions including Base::functions
Compiler Error
Base.cc:254:13: fatal error: 'iterator is not a class, namespace, or enumeration
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
^
/Library/Developer/CommandLineTools/usr/include/c++/v1/iterator:522:29: note: 'iterator' declared here
struct _LIBCPP_TEMPLATE_VIS iterator
c++ iterator
2
iterator
is a nested class: try qualifiying the implementation in cpp file withBase::iterator::iterator
Or try avoid to declare iterator as nested class
– Gian Paolo
Nov 19 '18 at 23:31
I believe that worked. Although, it came up with another compiler error but one I can deal with. Thanks. If all else fails, I may avoid a nested class. Thanks.
– N. Colostate
Nov 19 '18 at 23:36
1
well, as a start, why do you need a nested class? (actually, I just asked this myself) see also stackoverflow.com/questions/4571355/…
– Gian Paolo
Nov 19 '18 at 23:40
I decided on using a nested class through examples provided from various .edu addresses. So, I assume it was standard practice. From the comments so far, it doesn't seem that way.
– N. Colostate
Nov 19 '18 at 23:44
the following declarationBase operator*() const;
seems suspicious. You really want to return a copy ofBase
?
– Phil1970
Nov 20 '18 at 2:01
add a comment |
I'm new to the iterator class; not in how to use iterators for containers but how to implement an iterator class. I have two files, a .h and .cpp. Right now, I'm getting a compiler error in my .cpp file that displays "fatal error: 'iterator' is not a class, namespace, or enumeration". I don't understand why its telling me this.
Note: I'm sure there are other errors in my code, but right now I'm just trying to get the existing compiler error resolved.
Base.h
#ifndef Base_h
#define Base_h
class Base {
protected:
std::vector<std::vector<std::string> > vec;
public:
class iterator {
private:
Base* p = nullptr;
size_t x, y;
public:
iterator() = default;
iterator(Base *, size_t, size_t);
~iterator();
iterator &operator++();
Base operator*() const;
}
iterator begin() const;
iterator end() const;
// bunch of other iterator::functions and Base::functions
};
#endif
Base.cpp
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
iterator::~iterator();
// Bunch of other iterator::functions including Base::functions
Compiler Error
Base.cc:254:13: fatal error: 'iterator is not a class, namespace, or enumeration
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
^
/Library/Developer/CommandLineTools/usr/include/c++/v1/iterator:522:29: note: 'iterator' declared here
struct _LIBCPP_TEMPLATE_VIS iterator
c++ iterator
I'm new to the iterator class; not in how to use iterators for containers but how to implement an iterator class. I have two files, a .h and .cpp. Right now, I'm getting a compiler error in my .cpp file that displays "fatal error: 'iterator' is not a class, namespace, or enumeration". I don't understand why its telling me this.
Note: I'm sure there are other errors in my code, but right now I'm just trying to get the existing compiler error resolved.
Base.h
#ifndef Base_h
#define Base_h
class Base {
protected:
std::vector<std::vector<std::string> > vec;
public:
class iterator {
private:
Base* p = nullptr;
size_t x, y;
public:
iterator() = default;
iterator(Base *, size_t, size_t);
~iterator();
iterator &operator++();
Base operator*() const;
}
iterator begin() const;
iterator end() const;
// bunch of other iterator::functions and Base::functions
};
#endif
Base.cpp
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
iterator::~iterator();
// Bunch of other iterator::functions including Base::functions
Compiler Error
Base.cc:254:13: fatal error: 'iterator is not a class, namespace, or enumeration
iterator::iterator(Base *b, size_t i, size_t j): p(b), x(i), y(j) {}
^
/Library/Developer/CommandLineTools/usr/include/c++/v1/iterator:522:29: note: 'iterator' declared here
struct _LIBCPP_TEMPLATE_VIS iterator
c++ iterator
c++ iterator
asked Nov 19 '18 at 23:27
N. ColostateN. Colostate
356
356
2
iterator
is a nested class: try qualifiying the implementation in cpp file withBase::iterator::iterator
Or try avoid to declare iterator as nested class
– Gian Paolo
Nov 19 '18 at 23:31
I believe that worked. Although, it came up with another compiler error but one I can deal with. Thanks. If all else fails, I may avoid a nested class. Thanks.
– N. Colostate
Nov 19 '18 at 23:36
1
well, as a start, why do you need a nested class? (actually, I just asked this myself) see also stackoverflow.com/questions/4571355/…
– Gian Paolo
Nov 19 '18 at 23:40
I decided on using a nested class through examples provided from various .edu addresses. So, I assume it was standard practice. From the comments so far, it doesn't seem that way.
– N. Colostate
Nov 19 '18 at 23:44
the following declarationBase operator*() const;
seems suspicious. You really want to return a copy ofBase
?
– Phil1970
Nov 20 '18 at 2:01
add a comment |
2
iterator
is a nested class: try qualifiying the implementation in cpp file withBase::iterator::iterator
Or try avoid to declare iterator as nested class
– Gian Paolo
Nov 19 '18 at 23:31
I believe that worked. Although, it came up with another compiler error but one I can deal with. Thanks. If all else fails, I may avoid a nested class. Thanks.
– N. Colostate
Nov 19 '18 at 23:36
1
well, as a start, why do you need a nested class? (actually, I just asked this myself) see also stackoverflow.com/questions/4571355/…
– Gian Paolo
Nov 19 '18 at 23:40
I decided on using a nested class through examples provided from various .edu addresses. So, I assume it was standard practice. From the comments so far, it doesn't seem that way.
– N. Colostate
Nov 19 '18 at 23:44
the following declarationBase operator*() const;
seems suspicious. You really want to return a copy ofBase
?
– Phil1970
Nov 20 '18 at 2:01
2
2
iterator
is a nested class: try qualifiying the implementation in cpp file with Base::iterator::iterator
Or try avoid to declare iterator as nested class– Gian Paolo
Nov 19 '18 at 23:31
iterator
is a nested class: try qualifiying the implementation in cpp file with Base::iterator::iterator
Or try avoid to declare iterator as nested class– Gian Paolo
Nov 19 '18 at 23:31
I believe that worked. Although, it came up with another compiler error but one I can deal with. Thanks. If all else fails, I may avoid a nested class. Thanks.
– N. Colostate
Nov 19 '18 at 23:36
I believe that worked. Although, it came up with another compiler error but one I can deal with. Thanks. If all else fails, I may avoid a nested class. Thanks.
– N. Colostate
Nov 19 '18 at 23:36
1
1
well, as a start, why do you need a nested class? (actually, I just asked this myself) see also stackoverflow.com/questions/4571355/…
– Gian Paolo
Nov 19 '18 at 23:40
well, as a start, why do you need a nested class? (actually, I just asked this myself) see also stackoverflow.com/questions/4571355/…
– Gian Paolo
Nov 19 '18 at 23:40
I decided on using a nested class through examples provided from various .edu addresses. So, I assume it was standard practice. From the comments so far, it doesn't seem that way.
– N. Colostate
Nov 19 '18 at 23:44
I decided on using a nested class through examples provided from various .edu addresses. So, I assume it was standard practice. From the comments so far, it doesn't seem that way.
– N. Colostate
Nov 19 '18 at 23:44
the following declaration
Base operator*() const;
seems suspicious. You really want to return a copy of Base
?– Phil1970
Nov 20 '18 at 2:01
the following declaration
Base operator*() const;
seems suspicious. You really want to return a copy of Base
?– Phil1970
Nov 20 '18 at 2:01
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384120%2fiterator-subclass-separate-inclusion%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384120%2fiterator-subclass-separate-inclusion%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xuFcA6zwhhYNsHfO9 fPAyubxUDcjb,B
2
iterator
is a nested class: try qualifiying the implementation in cpp file withBase::iterator::iterator
Or try avoid to declare iterator as nested class– Gian Paolo
Nov 19 '18 at 23:31
I believe that worked. Although, it came up with another compiler error but one I can deal with. Thanks. If all else fails, I may avoid a nested class. Thanks.
– N. Colostate
Nov 19 '18 at 23:36
1
well, as a start, why do you need a nested class? (actually, I just asked this myself) see also stackoverflow.com/questions/4571355/…
– Gian Paolo
Nov 19 '18 at 23:40
I decided on using a nested class through examples provided from various .edu addresses. So, I assume it was standard practice. From the comments so far, it doesn't seem that way.
– N. Colostate
Nov 19 '18 at 23:44
the following declaration
Base operator*() const;
seems suspicious. You really want to return a copy ofBase
?– Phil1970
Nov 20 '18 at 2:01