objects are not initialized in another .js file?
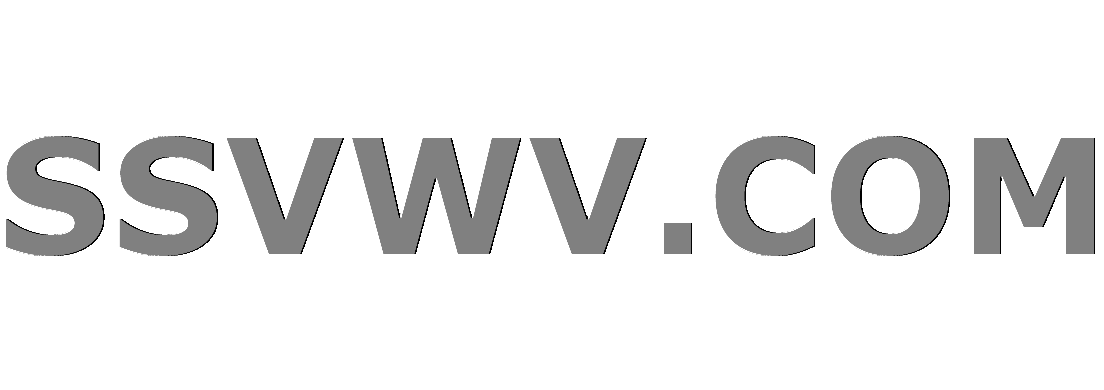
Multi tool use
[SOLVED - see at the end please]
[EDITED]
I have the following source:
index.js:
functions = require('firebase-functions');
admin = require('firebase-admin');
globals = require('./globals');
.....
admin.initializeApp();
exports.gateway = functions.https.onCall((data, context) => {
.....
return globals.dumpItemName(1);
});
exports.POST_gateway = functions.https.onRequest((req, res) => {
.....
return globals.dumpItemName(1);
});
globals.dumpItemName(1);
globals.js:
....
ITEM_NAMES = new Map()
.set(1, 'item1')
.set(2, 'item2');
....
getMapValue = function(map, key) {
let value = map.get(key);
if (value === undefined) {
throw new Error('Missing value for key ' + key);
}
return value;
}
....
dumpItemName = function(key) {
console.log(getMapValue(ITEM_NAMES, key));
}
exports.dumpItemName = dumpItemName;
I'm facing the following problem:
If I run/debug it locally by F5 it executes fine and dumps the expected value.
If I try to deploy it by firebase deploy --only functions
and then call via postman an https.onRequest(...) or https.onCall(....) function which tries to do the same:
globals.dumpItemName(1);
it fails with 'Missing value for key 1'
So I guess when I run it locally with F5 it executes index.js like a program and loads required modules and also run the code in them - so the Map in globals.js is initialized.
But when I deploy it via firebase deploy --functions only it does not execute the code.
So what should I do in order to have Map initialized after deploy ?
[SOLVED - THE REASON FOR THE "PROBLEM"]
Extremely dummy of my side.
Obviously for some of the local tests
(I have by now 10 end-to-end test scenario functions) I've put in index.js the following piece of code:
//!!! JUST FOR TEST - REMOVE IT !!!
ITEM_NAMES = new Map()
.set(globals.ITEM_TEST, 'test');
//!!! JUST FOR TEST - REMOVE IT !!!
so this map was redeclared with just one value in it. and the value of globals.ITEM_TEST is 200.
and it was used in one temporary test scenario function.
And just bad coincidences:
obviously I was too tired and forgot about it.
my other local tests were commented and that's why the local run was successful - in this temporary scenario, only this value was used.
I've decided to deploy in this exact moment. And none of the values I used in my onCall() or onRequest() functions used globals.ITEM_TEST (in real and in dummy both)
So it is...
Sorry for bothering. There is no problem in fact.
javascript firebase google-cloud-functions
|
show 2 more comments
[SOLVED - see at the end please]
[EDITED]
I have the following source:
index.js:
functions = require('firebase-functions');
admin = require('firebase-admin');
globals = require('./globals');
.....
admin.initializeApp();
exports.gateway = functions.https.onCall((data, context) => {
.....
return globals.dumpItemName(1);
});
exports.POST_gateway = functions.https.onRequest((req, res) => {
.....
return globals.dumpItemName(1);
});
globals.dumpItemName(1);
globals.js:
....
ITEM_NAMES = new Map()
.set(1, 'item1')
.set(2, 'item2');
....
getMapValue = function(map, key) {
let value = map.get(key);
if (value === undefined) {
throw new Error('Missing value for key ' + key);
}
return value;
}
....
dumpItemName = function(key) {
console.log(getMapValue(ITEM_NAMES, key));
}
exports.dumpItemName = dumpItemName;
I'm facing the following problem:
If I run/debug it locally by F5 it executes fine and dumps the expected value.
If I try to deploy it by firebase deploy --only functions
and then call via postman an https.onRequest(...) or https.onCall(....) function which tries to do the same:
globals.dumpItemName(1);
it fails with 'Missing value for key 1'
So I guess when I run it locally with F5 it executes index.js like a program and loads required modules and also run the code in them - so the Map in globals.js is initialized.
But when I deploy it via firebase deploy --functions only it does not execute the code.
So what should I do in order to have Map initialized after deploy ?
[SOLVED - THE REASON FOR THE "PROBLEM"]
Extremely dummy of my side.
Obviously for some of the local tests
(I have by now 10 end-to-end test scenario functions) I've put in index.js the following piece of code:
//!!! JUST FOR TEST - REMOVE IT !!!
ITEM_NAMES = new Map()
.set(globals.ITEM_TEST, 'test');
//!!! JUST FOR TEST - REMOVE IT !!!
so this map was redeclared with just one value in it. and the value of globals.ITEM_TEST is 200.
and it was used in one temporary test scenario function.
And just bad coincidences:
obviously I was too tired and forgot about it.
my other local tests were commented and that's why the local run was successful - in this temporary scenario, only this value was used.
I've decided to deploy in this exact moment. And none of the values I used in my onCall() or onRequest() functions used globals.ITEM_TEST (in real and in dummy both)
So it is...
Sorry for bothering. There is no problem in fact.
javascript firebase google-cloud-functions
1
You haven't shown any code that actually creates an HTTP function or a callable function. You need to use the functions SDK declare your function. Where is that code? firebase.google.com/docs/functions/http-events
– Doug Stevenson
Nov 18 '18 at 21:32
I've edited the code. I have the same problem with this simple dummy code as with my real code. Initially I thought the problem was in my real code and I've put that very simple dummy code just dumping the itemname but the problem is the same: the Map() is not initialized as it is in local run with F5 with VSCode.
– Ivan Peshev
Nov 19 '18 at 5:34
1
You should check your functions withfirebase serve --only functions
– kftse
Nov 19 '18 at 6:12
Yes, the same result with firebase serve --only functions and test via Postman. Obviously the map is not initialized in globals.js as it is if I just use F5 to simply test as a normal program.
– Ivan Peshev
Nov 19 '18 at 6:27
Maybe I should put the initialization in some function and I to this function from index.js. (Map is local for globals.js because it's used only by getMapValue() function)
– Ivan Peshev
Nov 19 '18 at 6:36
|
show 2 more comments
[SOLVED - see at the end please]
[EDITED]
I have the following source:
index.js:
functions = require('firebase-functions');
admin = require('firebase-admin');
globals = require('./globals');
.....
admin.initializeApp();
exports.gateway = functions.https.onCall((data, context) => {
.....
return globals.dumpItemName(1);
});
exports.POST_gateway = functions.https.onRequest((req, res) => {
.....
return globals.dumpItemName(1);
});
globals.dumpItemName(1);
globals.js:
....
ITEM_NAMES = new Map()
.set(1, 'item1')
.set(2, 'item2');
....
getMapValue = function(map, key) {
let value = map.get(key);
if (value === undefined) {
throw new Error('Missing value for key ' + key);
}
return value;
}
....
dumpItemName = function(key) {
console.log(getMapValue(ITEM_NAMES, key));
}
exports.dumpItemName = dumpItemName;
I'm facing the following problem:
If I run/debug it locally by F5 it executes fine and dumps the expected value.
If I try to deploy it by firebase deploy --only functions
and then call via postman an https.onRequest(...) or https.onCall(....) function which tries to do the same:
globals.dumpItemName(1);
it fails with 'Missing value for key 1'
So I guess when I run it locally with F5 it executes index.js like a program and loads required modules and also run the code in them - so the Map in globals.js is initialized.
But when I deploy it via firebase deploy --functions only it does not execute the code.
So what should I do in order to have Map initialized after deploy ?
[SOLVED - THE REASON FOR THE "PROBLEM"]
Extremely dummy of my side.
Obviously for some of the local tests
(I have by now 10 end-to-end test scenario functions) I've put in index.js the following piece of code:
//!!! JUST FOR TEST - REMOVE IT !!!
ITEM_NAMES = new Map()
.set(globals.ITEM_TEST, 'test');
//!!! JUST FOR TEST - REMOVE IT !!!
so this map was redeclared with just one value in it. and the value of globals.ITEM_TEST is 200.
and it was used in one temporary test scenario function.
And just bad coincidences:
obviously I was too tired and forgot about it.
my other local tests were commented and that's why the local run was successful - in this temporary scenario, only this value was used.
I've decided to deploy in this exact moment. And none of the values I used in my onCall() or onRequest() functions used globals.ITEM_TEST (in real and in dummy both)
So it is...
Sorry for bothering. There is no problem in fact.
javascript firebase google-cloud-functions
[SOLVED - see at the end please]
[EDITED]
I have the following source:
index.js:
functions = require('firebase-functions');
admin = require('firebase-admin');
globals = require('./globals');
.....
admin.initializeApp();
exports.gateway = functions.https.onCall((data, context) => {
.....
return globals.dumpItemName(1);
});
exports.POST_gateway = functions.https.onRequest((req, res) => {
.....
return globals.dumpItemName(1);
});
globals.dumpItemName(1);
globals.js:
....
ITEM_NAMES = new Map()
.set(1, 'item1')
.set(2, 'item2');
....
getMapValue = function(map, key) {
let value = map.get(key);
if (value === undefined) {
throw new Error('Missing value for key ' + key);
}
return value;
}
....
dumpItemName = function(key) {
console.log(getMapValue(ITEM_NAMES, key));
}
exports.dumpItemName = dumpItemName;
I'm facing the following problem:
If I run/debug it locally by F5 it executes fine and dumps the expected value.
If I try to deploy it by firebase deploy --only functions
and then call via postman an https.onRequest(...) or https.onCall(....) function which tries to do the same:
globals.dumpItemName(1);
it fails with 'Missing value for key 1'
So I guess when I run it locally with F5 it executes index.js like a program and loads required modules and also run the code in them - so the Map in globals.js is initialized.
But when I deploy it via firebase deploy --functions only it does not execute the code.
So what should I do in order to have Map initialized after deploy ?
[SOLVED - THE REASON FOR THE "PROBLEM"]
Extremely dummy of my side.
Obviously for some of the local tests
(I have by now 10 end-to-end test scenario functions) I've put in index.js the following piece of code:
//!!! JUST FOR TEST - REMOVE IT !!!
ITEM_NAMES = new Map()
.set(globals.ITEM_TEST, 'test');
//!!! JUST FOR TEST - REMOVE IT !!!
so this map was redeclared with just one value in it. and the value of globals.ITEM_TEST is 200.
and it was used in one temporary test scenario function.
And just bad coincidences:
obviously I was too tired and forgot about it.
my other local tests were commented and that's why the local run was successful - in this temporary scenario, only this value was used.
I've decided to deploy in this exact moment. And none of the values I used in my onCall() or onRequest() functions used globals.ITEM_TEST (in real and in dummy both)
So it is...
Sorry for bothering. There is no problem in fact.
javascript firebase google-cloud-functions
javascript firebase google-cloud-functions
edited Nov 20 '18 at 6:21


priyanshi srivastava
938520
938520
asked Nov 18 '18 at 21:08


Ivan PeshevIvan Peshev
369
369
1
You haven't shown any code that actually creates an HTTP function or a callable function. You need to use the functions SDK declare your function. Where is that code? firebase.google.com/docs/functions/http-events
– Doug Stevenson
Nov 18 '18 at 21:32
I've edited the code. I have the same problem with this simple dummy code as with my real code. Initially I thought the problem was in my real code and I've put that very simple dummy code just dumping the itemname but the problem is the same: the Map() is not initialized as it is in local run with F5 with VSCode.
– Ivan Peshev
Nov 19 '18 at 5:34
1
You should check your functions withfirebase serve --only functions
– kftse
Nov 19 '18 at 6:12
Yes, the same result with firebase serve --only functions and test via Postman. Obviously the map is not initialized in globals.js as it is if I just use F5 to simply test as a normal program.
– Ivan Peshev
Nov 19 '18 at 6:27
Maybe I should put the initialization in some function and I to this function from index.js. (Map is local for globals.js because it's used only by getMapValue() function)
– Ivan Peshev
Nov 19 '18 at 6:36
|
show 2 more comments
1
You haven't shown any code that actually creates an HTTP function or a callable function. You need to use the functions SDK declare your function. Where is that code? firebase.google.com/docs/functions/http-events
– Doug Stevenson
Nov 18 '18 at 21:32
I've edited the code. I have the same problem with this simple dummy code as with my real code. Initially I thought the problem was in my real code and I've put that very simple dummy code just dumping the itemname but the problem is the same: the Map() is not initialized as it is in local run with F5 with VSCode.
– Ivan Peshev
Nov 19 '18 at 5:34
1
You should check your functions withfirebase serve --only functions
– kftse
Nov 19 '18 at 6:12
Yes, the same result with firebase serve --only functions and test via Postman. Obviously the map is not initialized in globals.js as it is if I just use F5 to simply test as a normal program.
– Ivan Peshev
Nov 19 '18 at 6:27
Maybe I should put the initialization in some function and I to this function from index.js. (Map is local for globals.js because it's used only by getMapValue() function)
– Ivan Peshev
Nov 19 '18 at 6:36
1
1
You haven't shown any code that actually creates an HTTP function or a callable function. You need to use the functions SDK declare your function. Where is that code? firebase.google.com/docs/functions/http-events
– Doug Stevenson
Nov 18 '18 at 21:32
You haven't shown any code that actually creates an HTTP function or a callable function. You need to use the functions SDK declare your function. Where is that code? firebase.google.com/docs/functions/http-events
– Doug Stevenson
Nov 18 '18 at 21:32
I've edited the code. I have the same problem with this simple dummy code as with my real code. Initially I thought the problem was in my real code and I've put that very simple dummy code just dumping the itemname but the problem is the same: the Map() is not initialized as it is in local run with F5 with VSCode.
– Ivan Peshev
Nov 19 '18 at 5:34
I've edited the code. I have the same problem with this simple dummy code as with my real code. Initially I thought the problem was in my real code and I've put that very simple dummy code just dumping the itemname but the problem is the same: the Map() is not initialized as it is in local run with F5 with VSCode.
– Ivan Peshev
Nov 19 '18 at 5:34
1
1
You should check your functions with
firebase serve --only functions
– kftse
Nov 19 '18 at 6:12
You should check your functions with
firebase serve --only functions
– kftse
Nov 19 '18 at 6:12
Yes, the same result with firebase serve --only functions and test via Postman. Obviously the map is not initialized in globals.js as it is if I just use F5 to simply test as a normal program.
– Ivan Peshev
Nov 19 '18 at 6:27
Yes, the same result with firebase serve --only functions and test via Postman. Obviously the map is not initialized in globals.js as it is if I just use F5 to simply test as a normal program.
– Ivan Peshev
Nov 19 '18 at 6:27
Maybe I should put the initialization in some function and I to this function from index.js. (Map is local for globals.js because it's used only by getMapValue() function)
– Ivan Peshev
Nov 19 '18 at 6:36
Maybe I should put the initialization in some function and I to this function from index.js. (Map is local for globals.js because it's used only by getMapValue() function)
– Ivan Peshev
Nov 19 '18 at 6:36
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53365478%2fobjects-are-not-initialized-in-another-js-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53365478%2fobjects-are-not-initialized-in-another-js-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZCF1yY,bXW8RUAxrKBxrxy6GKbO4 3KQHCVzCf2G8egHDTG3V6v HN6Vmg1c,inVochTL3PodSG4y2
1
You haven't shown any code that actually creates an HTTP function or a callable function. You need to use the functions SDK declare your function. Where is that code? firebase.google.com/docs/functions/http-events
– Doug Stevenson
Nov 18 '18 at 21:32
I've edited the code. I have the same problem with this simple dummy code as with my real code. Initially I thought the problem was in my real code and I've put that very simple dummy code just dumping the itemname but the problem is the same: the Map() is not initialized as it is in local run with F5 with VSCode.
– Ivan Peshev
Nov 19 '18 at 5:34
1
You should check your functions with
firebase serve --only functions
– kftse
Nov 19 '18 at 6:12
Yes, the same result with firebase serve --only functions and test via Postman. Obviously the map is not initialized in globals.js as it is if I just use F5 to simply test as a normal program.
– Ivan Peshev
Nov 19 '18 at 6:27
Maybe I should put the initialization in some function and I to this function from index.js. (Map is local for globals.js because it's used only by getMapValue() function)
– Ivan Peshev
Nov 19 '18 at 6:36