Postman - Calling a function with callback defined in Collection pre-request scripts
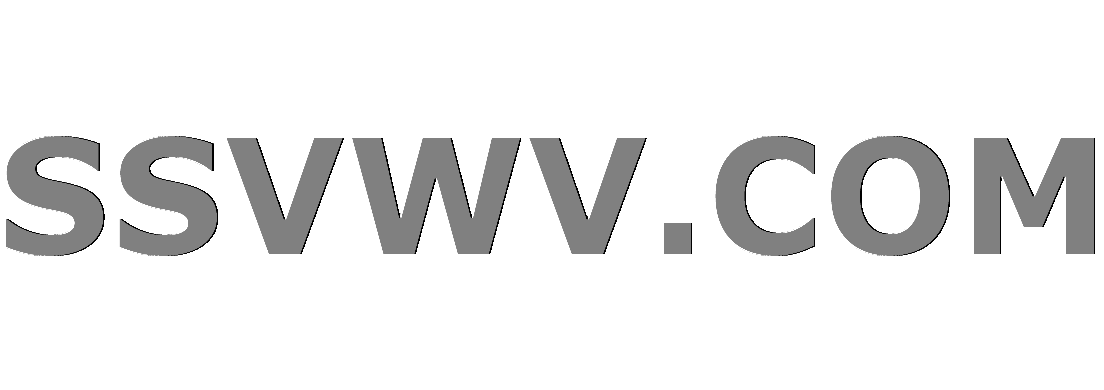
Multi tool use
I'm trying to define a function that sends a request in a collection Pre-Request scripts:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
}
glbl = {
doRequest: doRequest
}
Then, in my main test (a simple GET to google), I have this in Pre-Request script:
glbl.doRequest(() => console.log('works!'));
However, the callback is never called, and the "request sent" log is never printed.
This is the output of my postman console:
ready to send request 11:58:02.257
GET http://www.google.com 11:58:02.262
Do you know what I'm doing wrong?
I can provide the exported collection as well if it helps.
Thanks!
Edit: it I move everything in the Pre-request scripts of the request (not the collection), everything works fine
postman
add a comment |
I'm trying to define a function that sends a request in a collection Pre-Request scripts:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
}
glbl = {
doRequest: doRequest
}
Then, in my main test (a simple GET to google), I have this in Pre-Request script:
glbl.doRequest(() => console.log('works!'));
However, the callback is never called, and the "request sent" log is never printed.
This is the output of my postman console:
ready to send request 11:58:02.257
GET http://www.google.com 11:58:02.262
Do you know what I'm doing wrong?
I can provide the exported collection as well if it helps.
Thanks!
Edit: it I move everything in the Pre-request scripts of the request (not the collection), everything works fine
postman
add a comment |
I'm trying to define a function that sends a request in a collection Pre-Request scripts:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
}
glbl = {
doRequest: doRequest
}
Then, in my main test (a simple GET to google), I have this in Pre-Request script:
glbl.doRequest(() => console.log('works!'));
However, the callback is never called, and the "request sent" log is never printed.
This is the output of my postman console:
ready to send request 11:58:02.257
GET http://www.google.com 11:58:02.262
Do you know what I'm doing wrong?
I can provide the exported collection as well if it helps.
Thanks!
Edit: it I move everything in the Pre-request scripts of the request (not the collection), everything works fine
postman
I'm trying to define a function that sends a request in a collection Pre-Request scripts:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
}
glbl = {
doRequest: doRequest
}
Then, in my main test (a simple GET to google), I have this in Pre-Request script:
glbl.doRequest(() => console.log('works!'));
However, the callback is never called, and the "request sent" log is never printed.
This is the output of my postman console:
ready to send request 11:58:02.257
GET http://www.google.com 11:58:02.262
Do you know what I'm doing wrong?
I can provide the exported collection as well if it helps.
Thanks!
Edit: it I move everything in the Pre-request scripts of the request (not the collection), everything works fine
postman
postman
edited Nov 24 '18 at 10:34
Sébastien Tromp
asked Nov 23 '18 at 10:59
Sébastien TrompSébastien Tromp
1891823
1891823
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
There's a few things happening here.
Local scoping issues
Your glbl
variable is missing a var
, const
or let
keyword.
Missing this keyword does not cause the variable to bubble up and become global on it's own. The environment Pre-request Script and request Pre-request Script are of different scopes.
As you've experienced, when you move the script to solely the request level, everything is sitting in the same scope so this works fine.
Creating global functions
Postman has the facility to create your own global variables. This can be done programmatically through pm.globals.set
and pm.globals.get
.
https://www.getpostman.com/docs/v6/postman/environments_and_globals/variables#accessing-variables-through-scripts
There are some limitations to these variables: you can only store strings in them, so the object and function that you've created will not persist if we don't do something to change their type.
In this case before we set any variables we need to ensure we:
toString
any functions
JSON.stringify
any objects
Now the Pre-request script for our collection will look like:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
In order to use this at a request level, we need to update our Pre-request Script there too:
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
If you were to log outglbl
orglbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.
– Matt
Nov 28 '18 at 1:42
Adding a log just before thedoRequest
call, I have something:glbl Object
(and see I don't have an error when callingglbl.doRequest
, it means that there is something, right?)
– Sébastien Tromp
Nov 28 '18 at 8:51
add a comment |
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53445409%2fpostman-calling-a-function-with-callback-defined-in-collection-pre-request-scr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There's a few things happening here.
Local scoping issues
Your glbl
variable is missing a var
, const
or let
keyword.
Missing this keyword does not cause the variable to bubble up and become global on it's own. The environment Pre-request Script and request Pre-request Script are of different scopes.
As you've experienced, when you move the script to solely the request level, everything is sitting in the same scope so this works fine.
Creating global functions
Postman has the facility to create your own global variables. This can be done programmatically through pm.globals.set
and pm.globals.get
.
https://www.getpostman.com/docs/v6/postman/environments_and_globals/variables#accessing-variables-through-scripts
There are some limitations to these variables: you can only store strings in them, so the object and function that you've created will not persist if we don't do something to change their type.
In this case before we set any variables we need to ensure we:
toString
any functions
JSON.stringify
any objects
Now the Pre-request script for our collection will look like:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
In order to use this at a request level, we need to update our Pre-request Script there too:
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
If you were to log outglbl
orglbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.
– Matt
Nov 28 '18 at 1:42
Adding a log just before thedoRequest
call, I have something:glbl Object
(and see I don't have an error when callingglbl.doRequest
, it means that there is something, right?)
– Sébastien Tromp
Nov 28 '18 at 8:51
add a comment |
There's a few things happening here.
Local scoping issues
Your glbl
variable is missing a var
, const
or let
keyword.
Missing this keyword does not cause the variable to bubble up and become global on it's own. The environment Pre-request Script and request Pre-request Script are of different scopes.
As you've experienced, when you move the script to solely the request level, everything is sitting in the same scope so this works fine.
Creating global functions
Postman has the facility to create your own global variables. This can be done programmatically through pm.globals.set
and pm.globals.get
.
https://www.getpostman.com/docs/v6/postman/environments_and_globals/variables#accessing-variables-through-scripts
There are some limitations to these variables: you can only store strings in them, so the object and function that you've created will not persist if we don't do something to change their type.
In this case before we set any variables we need to ensure we:
toString
any functions
JSON.stringify
any objects
Now the Pre-request script for our collection will look like:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
In order to use this at a request level, we need to update our Pre-request Script there too:
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
If you were to log outglbl
orglbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.
– Matt
Nov 28 '18 at 1:42
Adding a log just before thedoRequest
call, I have something:glbl Object
(and see I don't have an error when callingglbl.doRequest
, it means that there is something, right?)
– Sébastien Tromp
Nov 28 '18 at 8:51
add a comment |
There's a few things happening here.
Local scoping issues
Your glbl
variable is missing a var
, const
or let
keyword.
Missing this keyword does not cause the variable to bubble up and become global on it's own. The environment Pre-request Script and request Pre-request Script are of different scopes.
As you've experienced, when you move the script to solely the request level, everything is sitting in the same scope so this works fine.
Creating global functions
Postman has the facility to create your own global variables. This can be done programmatically through pm.globals.set
and pm.globals.get
.
https://www.getpostman.com/docs/v6/postman/environments_and_globals/variables#accessing-variables-through-scripts
There are some limitations to these variables: you can only store strings in them, so the object and function that you've created will not persist if we don't do something to change their type.
In this case before we set any variables we need to ensure we:
toString
any functions
JSON.stringify
any objects
Now the Pre-request script for our collection will look like:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
In order to use this at a request level, we need to update our Pre-request Script there too:
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
There's a few things happening here.
Local scoping issues
Your glbl
variable is missing a var
, const
or let
keyword.
Missing this keyword does not cause the variable to bubble up and become global on it's own. The environment Pre-request Script and request Pre-request Script are of different scopes.
As you've experienced, when you move the script to solely the request level, everything is sitting in the same scope so this works fine.
Creating global functions
Postman has the facility to create your own global variables. This can be done programmatically through pm.globals.set
and pm.globals.get
.
https://www.getpostman.com/docs/v6/postman/environments_and_globals/variables#accessing-variables-through-scripts
There are some limitations to these variables: you can only store strings in them, so the object and function that you've created will not persist if we don't do something to change their type.
In this case before we set any variables we need to ensure we:
toString
any functions
JSON.stringify
any objects
Now the Pre-request script for our collection will look like:
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
In order to use this at a request level, we need to update our Pre-request Script there too:
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
const doRequest = (callback) => {
const echoPostRequest = {
url: 'https://postman-echo.com/post',
method: 'POST',
header: 'headername1:value1',
body: {
mode: 'raw',
raw: JSON.stringify({ key: 'this is json' })
}
};
console.log('ready to send request');
pm.sendRequest(echoPostRequest, function (err, res) {
console.log('request sent', err ? err : res.json());
callback();
});
};
const glbl = {
doRequest: doRequest.toString()
};
pm.globals.set('glbl', JSON.stringify(glbl));
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
const glbl = JSON.parse(pm.globals.get('glbl'));
const doRequest = eval(glbl.doRequest);
doRequest(() => console.log('works!'));
answered Nov 26 '18 at 22:44
MattMatt
135314
135314
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
If you were to log outglbl
orglbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.
– Matt
Nov 28 '18 at 1:42
Adding a log just before thedoRequest
call, I have something:glbl Object
(and see I don't have an error when callingglbl.doRequest
, it means that there is something, right?)
– Sébastien Tromp
Nov 28 '18 at 8:51
add a comment |
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
If you were to log outglbl
orglbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.
– Matt
Nov 28 '18 at 1:42
Adding a log just before thedoRequest
call, I have something:glbl Object
(and see I don't have an error when callingglbl.doRequest
, it means that there is something, right?)
– Sébastien Tromp
Nov 28 '18 at 8:51
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
Thanks, I'll try that tomorrow. But then I don't understand why I am still able to acces glbl? And the doRequest attribute is accessible and executes fine up until the postman call. Would you mind explaining to me what happens here?
– Sébastien Tromp
Nov 27 '18 at 22:22
If you were to log out
glbl
or glbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.– Matt
Nov 28 '18 at 1:42
If you were to log out
glbl
or glbl.doRequest
from within your request based Pre-request Script you'll see that nothing is there - so actually you aren't able to access it.– Matt
Nov 28 '18 at 1:42
Adding a log just before the
doRequest
call, I have something: glbl Object
(and see I don't have an error when calling glbl.doRequest
, it means that there is something, right?)– Sébastien Tromp
Nov 28 '18 at 8:51
Adding a log just before the
doRequest
call, I have something: glbl Object
(and see I don't have an error when calling glbl.doRequest
, it means that there is something, right?)– Sébastien Tromp
Nov 28 '18 at 8:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53445409%2fpostman-calling-a-function-with-callback-defined-in-collection-pre-request-scr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5 WgcGvNhJ1NAsK0A 6