Accessing class properties from within a class
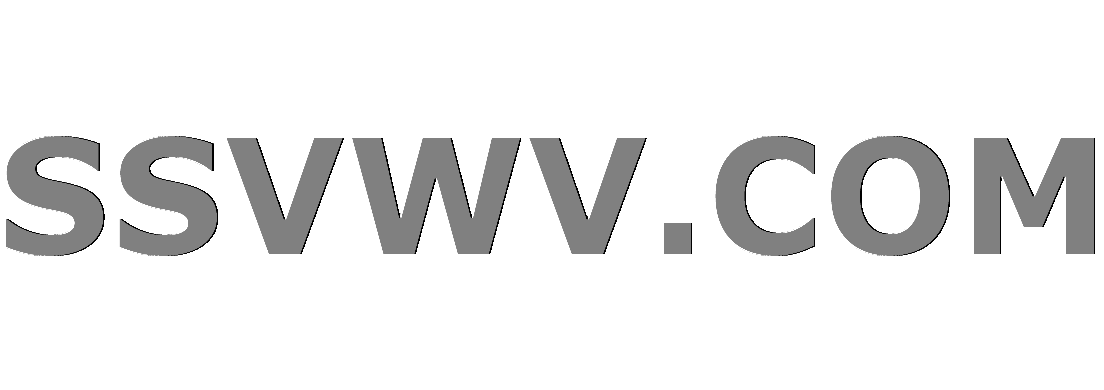
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am making an fantasy programming language for a game, and so I am writing the language and interpreter in python. The language works as a stream of a commands, each one is interpreted each "step." A string of code is inputted, and converted to a list of command objects. The interpreter handles the commands, but some of the commands need to be able to access attributes of the interpreter.
For example, the interpreter keeps track of the active command (numbered from the first command) so a goto command would need to change the active command. The current way I do this is pass the data into a command, it is modified, and the interpreter sets it's attribute equal to it.
self.current = active.evaluate(self.current)
This sets the current command number equal to the active command evaluated with the current command number inputted. This is how the whole block looks:
if active.complete(): # on command completion
type = active.return_type # get return type
# passes in requested data to modify
if type == "var": # changing var stream
self.var = active.evaluate(self.var)
elif type == "stream": # changing packet stream
self.stream = active.evaluate(self.stream)
elif type == "current": # changing current packet
self.current = active.evaluate(self.current)
elif type == "value": # returns argument for next command
self.holder = active.evaluate(self.var)
self.resolve = True
elif type == "none":
active.evaluate()
This seems like a inconvient way of doing things, is there a better way of modifying class attributes from inside of class?
python oop
add a comment |
I am making an fantasy programming language for a game, and so I am writing the language and interpreter in python. The language works as a stream of a commands, each one is interpreted each "step." A string of code is inputted, and converted to a list of command objects. The interpreter handles the commands, but some of the commands need to be able to access attributes of the interpreter.
For example, the interpreter keeps track of the active command (numbered from the first command) so a goto command would need to change the active command. The current way I do this is pass the data into a command, it is modified, and the interpreter sets it's attribute equal to it.
self.current = active.evaluate(self.current)
This sets the current command number equal to the active command evaluated with the current command number inputted. This is how the whole block looks:
if active.complete(): # on command completion
type = active.return_type # get return type
# passes in requested data to modify
if type == "var": # changing var stream
self.var = active.evaluate(self.var)
elif type == "stream": # changing packet stream
self.stream = active.evaluate(self.stream)
elif type == "current": # changing current packet
self.current = active.evaluate(self.current)
elif type == "value": # returns argument for next command
self.holder = active.evaluate(self.var)
self.resolve = True
elif type == "none":
active.evaluate()
This seems like a inconvient way of doing things, is there a better way of modifying class attributes from inside of class?
python oop
add a comment |
I am making an fantasy programming language for a game, and so I am writing the language and interpreter in python. The language works as a stream of a commands, each one is interpreted each "step." A string of code is inputted, and converted to a list of command objects. The interpreter handles the commands, but some of the commands need to be able to access attributes of the interpreter.
For example, the interpreter keeps track of the active command (numbered from the first command) so a goto command would need to change the active command. The current way I do this is pass the data into a command, it is modified, and the interpreter sets it's attribute equal to it.
self.current = active.evaluate(self.current)
This sets the current command number equal to the active command evaluated with the current command number inputted. This is how the whole block looks:
if active.complete(): # on command completion
type = active.return_type # get return type
# passes in requested data to modify
if type == "var": # changing var stream
self.var = active.evaluate(self.var)
elif type == "stream": # changing packet stream
self.stream = active.evaluate(self.stream)
elif type == "current": # changing current packet
self.current = active.evaluate(self.current)
elif type == "value": # returns argument for next command
self.holder = active.evaluate(self.var)
self.resolve = True
elif type == "none":
active.evaluate()
This seems like a inconvient way of doing things, is there a better way of modifying class attributes from inside of class?
python oop
I am making an fantasy programming language for a game, and so I am writing the language and interpreter in python. The language works as a stream of a commands, each one is interpreted each "step." A string of code is inputted, and converted to a list of command objects. The interpreter handles the commands, but some of the commands need to be able to access attributes of the interpreter.
For example, the interpreter keeps track of the active command (numbered from the first command) so a goto command would need to change the active command. The current way I do this is pass the data into a command, it is modified, and the interpreter sets it's attribute equal to it.
self.current = active.evaluate(self.current)
This sets the current command number equal to the active command evaluated with the current command number inputted. This is how the whole block looks:
if active.complete(): # on command completion
type = active.return_type # get return type
# passes in requested data to modify
if type == "var": # changing var stream
self.var = active.evaluate(self.var)
elif type == "stream": # changing packet stream
self.stream = active.evaluate(self.stream)
elif type == "current": # changing current packet
self.current = active.evaluate(self.current)
elif type == "value": # returns argument for next command
self.holder = active.evaluate(self.var)
self.resolve = True
elif type == "none":
active.evaluate()
This seems like a inconvient way of doing things, is there a better way of modifying class attributes from inside of class?
python oop
python oop
asked Nov 25 '18 at 3:35
AeolusAeolus
411315
411315
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
My simple answer would be to simply pass in the interpreter itself and let the object change the relevant property on the interpreter. If you didn't want to break too much from the format you already have, you could give the evaluate()
function two arguments:
def evaluate(interpreter, field_name):
interpreter.setattr(field_name, some_value)
which you would then call like
active.evaluate(self, "var")
However, this seems like a good place to implement polymorphism, which would solve both the problem you're asking about and eliminate the need for a growing stack of if
statements.
First, let's say you have a superclass for commands:
class Command:
def complete():
# do something, return a boolean
def evaluate(interpreter):
raise NotImplementedException()
Currently, it seems like you're using the command's return_type
to change what you're doing with the command. But why not simply detect what type of command is going to be run, and instantiate a different subclass with different behavior accordingly?
class GotoCommand(Command):
def evaluate(interpreter):
interpreter.current = some_value
class PacketCommand(Command):
def evaluate(interpreter):
interpreter.stream = some_other_value
...
after which you could just call active.evaluate(self)
in the interpreter, without even having to care what kind of command it is.
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464432%2faccessing-class-properties-from-within-a-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
My simple answer would be to simply pass in the interpreter itself and let the object change the relevant property on the interpreter. If you didn't want to break too much from the format you already have, you could give the evaluate()
function two arguments:
def evaluate(interpreter, field_name):
interpreter.setattr(field_name, some_value)
which you would then call like
active.evaluate(self, "var")
However, this seems like a good place to implement polymorphism, which would solve both the problem you're asking about and eliminate the need for a growing stack of if
statements.
First, let's say you have a superclass for commands:
class Command:
def complete():
# do something, return a boolean
def evaluate(interpreter):
raise NotImplementedException()
Currently, it seems like you're using the command's return_type
to change what you're doing with the command. But why not simply detect what type of command is going to be run, and instantiate a different subclass with different behavior accordingly?
class GotoCommand(Command):
def evaluate(interpreter):
interpreter.current = some_value
class PacketCommand(Command):
def evaluate(interpreter):
interpreter.stream = some_other_value
...
after which you could just call active.evaluate(self)
in the interpreter, without even having to care what kind of command it is.
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
add a comment |
My simple answer would be to simply pass in the interpreter itself and let the object change the relevant property on the interpreter. If you didn't want to break too much from the format you already have, you could give the evaluate()
function two arguments:
def evaluate(interpreter, field_name):
interpreter.setattr(field_name, some_value)
which you would then call like
active.evaluate(self, "var")
However, this seems like a good place to implement polymorphism, which would solve both the problem you're asking about and eliminate the need for a growing stack of if
statements.
First, let's say you have a superclass for commands:
class Command:
def complete():
# do something, return a boolean
def evaluate(interpreter):
raise NotImplementedException()
Currently, it seems like you're using the command's return_type
to change what you're doing with the command. But why not simply detect what type of command is going to be run, and instantiate a different subclass with different behavior accordingly?
class GotoCommand(Command):
def evaluate(interpreter):
interpreter.current = some_value
class PacketCommand(Command):
def evaluate(interpreter):
interpreter.stream = some_other_value
...
after which you could just call active.evaluate(self)
in the interpreter, without even having to care what kind of command it is.
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
add a comment |
My simple answer would be to simply pass in the interpreter itself and let the object change the relevant property on the interpreter. If you didn't want to break too much from the format you already have, you could give the evaluate()
function two arguments:
def evaluate(interpreter, field_name):
interpreter.setattr(field_name, some_value)
which you would then call like
active.evaluate(self, "var")
However, this seems like a good place to implement polymorphism, which would solve both the problem you're asking about and eliminate the need for a growing stack of if
statements.
First, let's say you have a superclass for commands:
class Command:
def complete():
# do something, return a boolean
def evaluate(interpreter):
raise NotImplementedException()
Currently, it seems like you're using the command's return_type
to change what you're doing with the command. But why not simply detect what type of command is going to be run, and instantiate a different subclass with different behavior accordingly?
class GotoCommand(Command):
def evaluate(interpreter):
interpreter.current = some_value
class PacketCommand(Command):
def evaluate(interpreter):
interpreter.stream = some_other_value
...
after which you could just call active.evaluate(self)
in the interpreter, without even having to care what kind of command it is.
My simple answer would be to simply pass in the interpreter itself and let the object change the relevant property on the interpreter. If you didn't want to break too much from the format you already have, you could give the evaluate()
function two arguments:
def evaluate(interpreter, field_name):
interpreter.setattr(field_name, some_value)
which you would then call like
active.evaluate(self, "var")
However, this seems like a good place to implement polymorphism, which would solve both the problem you're asking about and eliminate the need for a growing stack of if
statements.
First, let's say you have a superclass for commands:
class Command:
def complete():
# do something, return a boolean
def evaluate(interpreter):
raise NotImplementedException()
Currently, it seems like you're using the command's return_type
to change what you're doing with the command. But why not simply detect what type of command is going to be run, and instantiate a different subclass with different behavior accordingly?
class GotoCommand(Command):
def evaluate(interpreter):
interpreter.current = some_value
class PacketCommand(Command):
def evaluate(interpreter):
interpreter.stream = some_other_value
...
after which you could just call active.evaluate(self)
in the interpreter, without even having to care what kind of command it is.
answered Nov 25 '18 at 4:33
Green Cloak GuyGreen Cloak Guy
3,4201721
3,4201721
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
add a comment |
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
Thank you, this is exactly what I was looking for! All of my commands are subclasses of a Command class already, so it should be easy to implement.
– Aeolus
Nov 25 '18 at 5:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464432%2faccessing-class-properties-from-within-a-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YRnQQyrNn5Yjug3sGMtGb,yo,qOk7 QqZX6YH Ul6sCFm0dW5oHR5ouyPFu46GaEF 8OVu2cbsfYk7,r9 1 FrWc20RqTxtB