Android wear device vibrator is not vibrating programmatically all the time
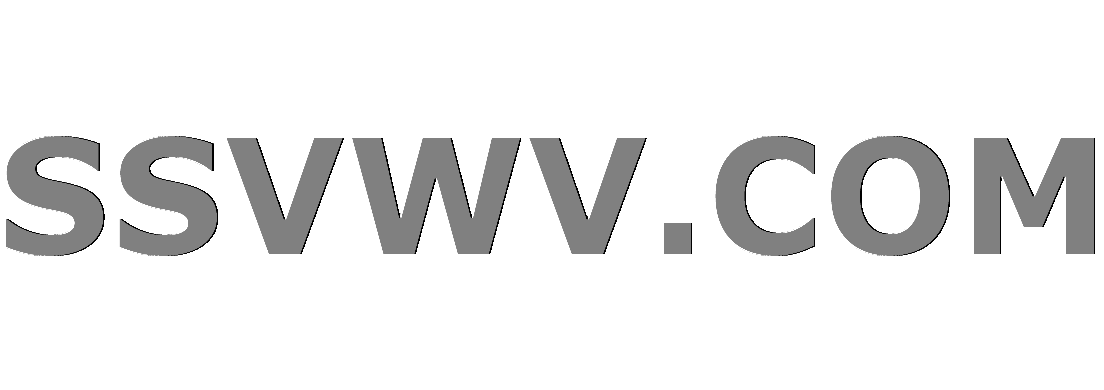
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm developing for a Huawei watch 2. When an alarm sms arrives, the watch should switch to the AlertScreen, and start vibrating. It works like 99% of the time, but sometimes the vibrating is not happening. I've already refactored the code a lot of times, but can't figure out where the bug is.
MainActivity
I'm registering a listener for receiving the SMS, and then calling the startAlert
function to start the vibrating:
public class MainActivity extends FragmentActivity {
private final FragmentManager supportFragmentManager = getSupportFragmentManager();
private final SmsBroadcastReceiver smsBroadcastReceiver = new SmsBroadcastReceiver();
private final AlertFragment alertFragment = new AlertFragment();
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
registerReceiver(smsBroadcastReceiver, new IntentFilter(Telephony.Sms.Intents.SMS_RECEIVED_ACTION));
smsBroadcastReceiver.setListener(new SmsListener() {
@Override
public void messageReceived(final String phoneNumber) {
startAlert(phoneNumber);
}
});
}
private void startAlert(final String phoneNumber) {
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
if (alertFragment.isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, alertFragment).commitAllowingStateLoss();
alertFragment.startAlert(phoneNumber);
}
}
}, 4000);
}
}
AlertFragment:
In this fragment in the startAlert
Im adding a helper class to the executorService
, which will start the actual vibrating. The isRunning
variable is here, because while running if the watch receives a new message from another number, it usually stopped the vibration.
public class AlertFragment extends Fragment {
private Button cancelButton;
private Future longRunningTaskFuture;
private ConnectionService mService;
private PowerManager powerManager;
private FragmentManager supportFragmentManager;
private PowerManager.WakeLock wakeLock;
AlertHelper longRunningTask = new AlertHelper();
public boolean isRunning = false;
private boolean mBound = false;
private final String TYPE = "feedback";
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
private View.OnClickListener onAlertButtonPressedListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
mService.sendMessage(new Message(TYPE, "1").toJson());
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
stopAlert();
}
};
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onStart() {
super.onStart();
supportFragmentManager = getActivity().getSupportFragmentManager();
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
wakeUpWatch();
Intent intent = new Intent(context, ConnectionService.class);
context.bindService(intent, mConnection, Context.BIND_AUTO_CREATE);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.alert_fragment, container, false);
if (view != null) {
cancelButton = (Button) view.findViewById(R.id.stop_button);
cancelButton.setOnClickListener(onAlertButtonPressedListener);
}
((DrawerLock) getActivity()).setDrawerLocked(true);
return view;
}
@Override
public void onSaveInstanceState(Bundle outState) {
//No call for super(). Bug on API Level > 11.
}
@Override
public void onDestroyView() {
super.onDestroyView();
((DrawerLock) getActivity()).setDrawerLocked(false);
}
public void startAlert(String phoneNumber) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
// submit task to threadpool:
if (isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
longRunningTaskFuture = executorService.submit(longRunningTask);
isRunning = true;
}
}
public void stopAlert() {
longRunningTaskFuture.cancel(true);
longRunningTaskFuture = null;
isRunning = false;
longRunningTask.vibrator.cancel();
releaseWakeLock();
}
private void wakeUpWatch() {
Intent intent = new Intent(getActivity().getApplicationContext(), MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // You need this if starting
// the activity from a service
intent.setAction(Intent.ACTION_MAIN);
intent.addCategory(Intent.CATEGORY_LAUNCHER);
startActivity(intent);
powerManager = (PowerManager) MyApplication.getAppContext().getSystemService(POWER_SERVICE);
wakeLock = powerManager.newWakeLock(PowerManager.ACQUIRE_CAUSES_WAKEUP | PowerManager.SCREEN_BRIGHT_WAKE_LOCK,"MyWakelockTag");
wakeLock.acquire();
}
private void releaseWakeLock() {
if (wakeLock != null && wakeLock.isHeld()) {
wakeLock.release();
}
}
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName className,
IBinder service) {
ConnectionService.LocalBinder binder = (ConnectionService.LocalBinder) service;
mService = binder.getServiceInstance();
mBound = true;
}
@Override
public void onServiceDisconnected(ComponentName arg0) {
mBound = false;
}
};
}
And finally, the AlertHelper
class will actually start the vibration:
public class AlertHelper implements Runnable {
Vibrator vibrator = (Vibrator) MyApplication.getAppContext().getSystemService(Context.VIBRATOR_SERVICE);
public volatile boolean isRunning;
private final long mVibratePattern = new long{0, 400, 800, 600, 800, 800, 800, 1000};
private final int mAmplitudes = new int{0, 255, 0, 255, 0, 255, 0, 255};
@Override
public void run() {
this.isRunning = true;
if (this.vibrator != null) {
this.vibrator.vibrate(VibrationEffect.createWaveform(mVibratePattern, mAmplitudes, 0));
}
}
}
So i have no idea, the vibration usually works as it should, but sometimes it just don't. I always had this bug with every implementation, so I'm starting to think that it could be a bug I'm not aware of. What could be the solution here?
EDIT:
The bug is not in the MainActivity, since the screen is always changing as it should, so the problem must be with the alert classes
java

add a comment |
I'm developing for a Huawei watch 2. When an alarm sms arrives, the watch should switch to the AlertScreen, and start vibrating. It works like 99% of the time, but sometimes the vibrating is not happening. I've already refactored the code a lot of times, but can't figure out where the bug is.
MainActivity
I'm registering a listener for receiving the SMS, and then calling the startAlert
function to start the vibrating:
public class MainActivity extends FragmentActivity {
private final FragmentManager supportFragmentManager = getSupportFragmentManager();
private final SmsBroadcastReceiver smsBroadcastReceiver = new SmsBroadcastReceiver();
private final AlertFragment alertFragment = new AlertFragment();
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
registerReceiver(smsBroadcastReceiver, new IntentFilter(Telephony.Sms.Intents.SMS_RECEIVED_ACTION));
smsBroadcastReceiver.setListener(new SmsListener() {
@Override
public void messageReceived(final String phoneNumber) {
startAlert(phoneNumber);
}
});
}
private void startAlert(final String phoneNumber) {
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
if (alertFragment.isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, alertFragment).commitAllowingStateLoss();
alertFragment.startAlert(phoneNumber);
}
}
}, 4000);
}
}
AlertFragment:
In this fragment in the startAlert
Im adding a helper class to the executorService
, which will start the actual vibrating. The isRunning
variable is here, because while running if the watch receives a new message from another number, it usually stopped the vibration.
public class AlertFragment extends Fragment {
private Button cancelButton;
private Future longRunningTaskFuture;
private ConnectionService mService;
private PowerManager powerManager;
private FragmentManager supportFragmentManager;
private PowerManager.WakeLock wakeLock;
AlertHelper longRunningTask = new AlertHelper();
public boolean isRunning = false;
private boolean mBound = false;
private final String TYPE = "feedback";
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
private View.OnClickListener onAlertButtonPressedListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
mService.sendMessage(new Message(TYPE, "1").toJson());
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
stopAlert();
}
};
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onStart() {
super.onStart();
supportFragmentManager = getActivity().getSupportFragmentManager();
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
wakeUpWatch();
Intent intent = new Intent(context, ConnectionService.class);
context.bindService(intent, mConnection, Context.BIND_AUTO_CREATE);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.alert_fragment, container, false);
if (view != null) {
cancelButton = (Button) view.findViewById(R.id.stop_button);
cancelButton.setOnClickListener(onAlertButtonPressedListener);
}
((DrawerLock) getActivity()).setDrawerLocked(true);
return view;
}
@Override
public void onSaveInstanceState(Bundle outState) {
//No call for super(). Bug on API Level > 11.
}
@Override
public void onDestroyView() {
super.onDestroyView();
((DrawerLock) getActivity()).setDrawerLocked(false);
}
public void startAlert(String phoneNumber) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
// submit task to threadpool:
if (isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
longRunningTaskFuture = executorService.submit(longRunningTask);
isRunning = true;
}
}
public void stopAlert() {
longRunningTaskFuture.cancel(true);
longRunningTaskFuture = null;
isRunning = false;
longRunningTask.vibrator.cancel();
releaseWakeLock();
}
private void wakeUpWatch() {
Intent intent = new Intent(getActivity().getApplicationContext(), MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // You need this if starting
// the activity from a service
intent.setAction(Intent.ACTION_MAIN);
intent.addCategory(Intent.CATEGORY_LAUNCHER);
startActivity(intent);
powerManager = (PowerManager) MyApplication.getAppContext().getSystemService(POWER_SERVICE);
wakeLock = powerManager.newWakeLock(PowerManager.ACQUIRE_CAUSES_WAKEUP | PowerManager.SCREEN_BRIGHT_WAKE_LOCK,"MyWakelockTag");
wakeLock.acquire();
}
private void releaseWakeLock() {
if (wakeLock != null && wakeLock.isHeld()) {
wakeLock.release();
}
}
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName className,
IBinder service) {
ConnectionService.LocalBinder binder = (ConnectionService.LocalBinder) service;
mService = binder.getServiceInstance();
mBound = true;
}
@Override
public void onServiceDisconnected(ComponentName arg0) {
mBound = false;
}
};
}
And finally, the AlertHelper
class will actually start the vibration:
public class AlertHelper implements Runnable {
Vibrator vibrator = (Vibrator) MyApplication.getAppContext().getSystemService(Context.VIBRATOR_SERVICE);
public volatile boolean isRunning;
private final long mVibratePattern = new long{0, 400, 800, 600, 800, 800, 800, 1000};
private final int mAmplitudes = new int{0, 255, 0, 255, 0, 255, 0, 255};
@Override
public void run() {
this.isRunning = true;
if (this.vibrator != null) {
this.vibrator.vibrate(VibrationEffect.createWaveform(mVibratePattern, mAmplitudes, 0));
}
}
}
So i have no idea, the vibration usually works as it should, but sometimes it just don't. I always had this bug with every implementation, so I'm starting to think that it could be a bug I'm not aware of. What could be the solution here?
EDIT:
The bug is not in the MainActivity, since the screen is always changing as it should, so the problem must be with the alert classes
java

add a comment |
I'm developing for a Huawei watch 2. When an alarm sms arrives, the watch should switch to the AlertScreen, and start vibrating. It works like 99% of the time, but sometimes the vibrating is not happening. I've already refactored the code a lot of times, but can't figure out where the bug is.
MainActivity
I'm registering a listener for receiving the SMS, and then calling the startAlert
function to start the vibrating:
public class MainActivity extends FragmentActivity {
private final FragmentManager supportFragmentManager = getSupportFragmentManager();
private final SmsBroadcastReceiver smsBroadcastReceiver = new SmsBroadcastReceiver();
private final AlertFragment alertFragment = new AlertFragment();
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
registerReceiver(smsBroadcastReceiver, new IntentFilter(Telephony.Sms.Intents.SMS_RECEIVED_ACTION));
smsBroadcastReceiver.setListener(new SmsListener() {
@Override
public void messageReceived(final String phoneNumber) {
startAlert(phoneNumber);
}
});
}
private void startAlert(final String phoneNumber) {
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
if (alertFragment.isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, alertFragment).commitAllowingStateLoss();
alertFragment.startAlert(phoneNumber);
}
}
}, 4000);
}
}
AlertFragment:
In this fragment in the startAlert
Im adding a helper class to the executorService
, which will start the actual vibrating. The isRunning
variable is here, because while running if the watch receives a new message from another number, it usually stopped the vibration.
public class AlertFragment extends Fragment {
private Button cancelButton;
private Future longRunningTaskFuture;
private ConnectionService mService;
private PowerManager powerManager;
private FragmentManager supportFragmentManager;
private PowerManager.WakeLock wakeLock;
AlertHelper longRunningTask = new AlertHelper();
public boolean isRunning = false;
private boolean mBound = false;
private final String TYPE = "feedback";
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
private View.OnClickListener onAlertButtonPressedListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
mService.sendMessage(new Message(TYPE, "1").toJson());
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
stopAlert();
}
};
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onStart() {
super.onStart();
supportFragmentManager = getActivity().getSupportFragmentManager();
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
wakeUpWatch();
Intent intent = new Intent(context, ConnectionService.class);
context.bindService(intent, mConnection, Context.BIND_AUTO_CREATE);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.alert_fragment, container, false);
if (view != null) {
cancelButton = (Button) view.findViewById(R.id.stop_button);
cancelButton.setOnClickListener(onAlertButtonPressedListener);
}
((DrawerLock) getActivity()).setDrawerLocked(true);
return view;
}
@Override
public void onSaveInstanceState(Bundle outState) {
//No call for super(). Bug on API Level > 11.
}
@Override
public void onDestroyView() {
super.onDestroyView();
((DrawerLock) getActivity()).setDrawerLocked(false);
}
public void startAlert(String phoneNumber) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
// submit task to threadpool:
if (isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
longRunningTaskFuture = executorService.submit(longRunningTask);
isRunning = true;
}
}
public void stopAlert() {
longRunningTaskFuture.cancel(true);
longRunningTaskFuture = null;
isRunning = false;
longRunningTask.vibrator.cancel();
releaseWakeLock();
}
private void wakeUpWatch() {
Intent intent = new Intent(getActivity().getApplicationContext(), MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // You need this if starting
// the activity from a service
intent.setAction(Intent.ACTION_MAIN);
intent.addCategory(Intent.CATEGORY_LAUNCHER);
startActivity(intent);
powerManager = (PowerManager) MyApplication.getAppContext().getSystemService(POWER_SERVICE);
wakeLock = powerManager.newWakeLock(PowerManager.ACQUIRE_CAUSES_WAKEUP | PowerManager.SCREEN_BRIGHT_WAKE_LOCK,"MyWakelockTag");
wakeLock.acquire();
}
private void releaseWakeLock() {
if (wakeLock != null && wakeLock.isHeld()) {
wakeLock.release();
}
}
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName className,
IBinder service) {
ConnectionService.LocalBinder binder = (ConnectionService.LocalBinder) service;
mService = binder.getServiceInstance();
mBound = true;
}
@Override
public void onServiceDisconnected(ComponentName arg0) {
mBound = false;
}
};
}
And finally, the AlertHelper
class will actually start the vibration:
public class AlertHelper implements Runnable {
Vibrator vibrator = (Vibrator) MyApplication.getAppContext().getSystemService(Context.VIBRATOR_SERVICE);
public volatile boolean isRunning;
private final long mVibratePattern = new long{0, 400, 800, 600, 800, 800, 800, 1000};
private final int mAmplitudes = new int{0, 255, 0, 255, 0, 255, 0, 255};
@Override
public void run() {
this.isRunning = true;
if (this.vibrator != null) {
this.vibrator.vibrate(VibrationEffect.createWaveform(mVibratePattern, mAmplitudes, 0));
}
}
}
So i have no idea, the vibration usually works as it should, but sometimes it just don't. I always had this bug with every implementation, so I'm starting to think that it could be a bug I'm not aware of. What could be the solution here?
EDIT:
The bug is not in the MainActivity, since the screen is always changing as it should, so the problem must be with the alert classes
java

I'm developing for a Huawei watch 2. When an alarm sms arrives, the watch should switch to the AlertScreen, and start vibrating. It works like 99% of the time, but sometimes the vibrating is not happening. I've already refactored the code a lot of times, but can't figure out where the bug is.
MainActivity
I'm registering a listener for receiving the SMS, and then calling the startAlert
function to start the vibrating:
public class MainActivity extends FragmentActivity {
private final FragmentManager supportFragmentManager = getSupportFragmentManager();
private final SmsBroadcastReceiver smsBroadcastReceiver = new SmsBroadcastReceiver();
private final AlertFragment alertFragment = new AlertFragment();
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
registerReceiver(smsBroadcastReceiver, new IntentFilter(Telephony.Sms.Intents.SMS_RECEIVED_ACTION));
smsBroadcastReceiver.setListener(new SmsListener() {
@Override
public void messageReceived(final String phoneNumber) {
startAlert(phoneNumber);
}
});
}
private void startAlert(final String phoneNumber) {
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
if (alertFragment.isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, alertFragment).commitAllowingStateLoss();
alertFragment.startAlert(phoneNumber);
}
}
}, 4000);
}
}
AlertFragment:
In this fragment in the startAlert
Im adding a helper class to the executorService
, which will start the actual vibrating. The isRunning
variable is here, because while running if the watch receives a new message from another number, it usually stopped the vibration.
public class AlertFragment extends Fragment {
private Button cancelButton;
private Future longRunningTaskFuture;
private ConnectionService mService;
private PowerManager powerManager;
private FragmentManager supportFragmentManager;
private PowerManager.WakeLock wakeLock;
AlertHelper longRunningTask = new AlertHelper();
public boolean isRunning = false;
private boolean mBound = false;
private final String TYPE = "feedback";
private static final String SENDER_PHONE_NUMBER = MyApplication.getAppContext().getResources().getString(R.string.sender_phone);
private View.OnClickListener onAlertButtonPressedListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
mService.sendMessage(new Message(TYPE, "1").toJson());
supportFragmentManager.beginTransaction().replace(R.id.fragment_container, new MainFragment()).commit();
stopAlert();
}
};
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public void onStart() {
super.onStart();
supportFragmentManager = getActivity().getSupportFragmentManager();
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
wakeUpWatch();
Intent intent = new Intent(context, ConnectionService.class);
context.bindService(intent, mConnection, Context.BIND_AUTO_CREATE);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.alert_fragment, container, false);
if (view != null) {
cancelButton = (Button) view.findViewById(R.id.stop_button);
cancelButton.setOnClickListener(onAlertButtonPressedListener);
}
((DrawerLock) getActivity()).setDrawerLocked(true);
return view;
}
@Override
public void onSaveInstanceState(Bundle outState) {
//No call for super(). Bug on API Level > 11.
}
@Override
public void onDestroyView() {
super.onDestroyView();
((DrawerLock) getActivity()).setDrawerLocked(false);
}
public void startAlert(String phoneNumber) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
// submit task to threadpool:
if (isRunning || phoneNumber.equals(SENDER_PHONE_NUMBER)) {
longRunningTaskFuture = executorService.submit(longRunningTask);
isRunning = true;
}
}
public void stopAlert() {
longRunningTaskFuture.cancel(true);
longRunningTaskFuture = null;
isRunning = false;
longRunningTask.vibrator.cancel();
releaseWakeLock();
}
private void wakeUpWatch() {
Intent intent = new Intent(getActivity().getApplicationContext(), MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // You need this if starting
// the activity from a service
intent.setAction(Intent.ACTION_MAIN);
intent.addCategory(Intent.CATEGORY_LAUNCHER);
startActivity(intent);
powerManager = (PowerManager) MyApplication.getAppContext().getSystemService(POWER_SERVICE);
wakeLock = powerManager.newWakeLock(PowerManager.ACQUIRE_CAUSES_WAKEUP | PowerManager.SCREEN_BRIGHT_WAKE_LOCK,"MyWakelockTag");
wakeLock.acquire();
}
private void releaseWakeLock() {
if (wakeLock != null && wakeLock.isHeld()) {
wakeLock.release();
}
}
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName className,
IBinder service) {
ConnectionService.LocalBinder binder = (ConnectionService.LocalBinder) service;
mService = binder.getServiceInstance();
mBound = true;
}
@Override
public void onServiceDisconnected(ComponentName arg0) {
mBound = false;
}
};
}
And finally, the AlertHelper
class will actually start the vibration:
public class AlertHelper implements Runnable {
Vibrator vibrator = (Vibrator) MyApplication.getAppContext().getSystemService(Context.VIBRATOR_SERVICE);
public volatile boolean isRunning;
private final long mVibratePattern = new long{0, 400, 800, 600, 800, 800, 800, 1000};
private final int mAmplitudes = new int{0, 255, 0, 255, 0, 255, 0, 255};
@Override
public void run() {
this.isRunning = true;
if (this.vibrator != null) {
this.vibrator.vibrate(VibrationEffect.createWaveform(mVibratePattern, mAmplitudes, 0));
}
}
}
So i have no idea, the vibration usually works as it should, but sometimes it just don't. I always had this bug with every implementation, so I'm starting to think that it could be a bug I'm not aware of. What could be the solution here?
EDIT:
The bug is not in the MainActivity, since the screen is always changing as it should, so the problem must be with the alert classes
java

java

edited Nov 23 '18 at 15:48
adamb
asked Nov 23 '18 at 15:42
adambadamb
136110
136110
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449509%2fandroid-wear-device-vibrator-is-not-vibrating-programmatically-all-the-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449509%2fandroid-wear-device-vibrator-is-not-vibrating-programmatically-all-the-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xwbNQj8lGejFFxJ,Z JeycR1YL0LFygFQ3V2lODA,hR,ZYao7qRFnZDwmIL3 a15OK0TOUoC,hqav,Of 2rVvx7 be7R 1 T5HyAiDHK,sfWP 8