Cropping of ImageViews in Custom ViewGroup
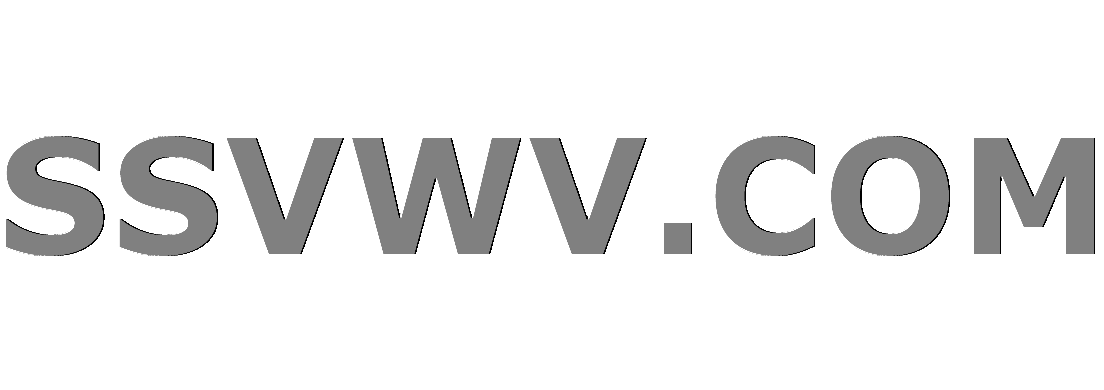
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am implementing custom ViewGroup which has overlapping ImageViews.
I load images with GlideApp. Problem is that some images are cropped a little (visible on the left side) and I cannot prevent this. The drawables which get cropped are Vector drawables and their mDrawableWidth & mDrawableHeight are larger than the height of the ViewGroup. I thought that the images would be scaled to fit the view dimensions (mScaleType = FIT_CENTER).
class OverlappingImageViewGroup : ViewGroup {
constructor(context: Context) : this(context, null)
constructor(context: Context, attrs: AttributeSet?) : this(context, attrs, 0)
constructor(context: Context, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr) {}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
val widthSize: Int = getDefaultSize(0, widthMeasureSpec)
val heightSize: Int = getDefaultSize(0, heightMeasureSpec)
val majorDimension = Math.min(widthSize, heightSize)
//Measure all child views
val childSpec = MeasureSpec.makeMeasureSpec(majorDimension, MeasureSpec.EXACTLY)
measureChildren(childSpec, childSpec)
//MUST call this to save our own dimensions
setMeasuredDimension(majorDimension, majorDimension)
}
override fun onLayout(changed: Boolean,
leftRelativeToParent: Int,
topRelativeToParent: Int,
rightRelativeToParent: Int,
bottomRelativeToParent: Int) {
val percentOverlap = 0.8f
if (childCount > 0) {
for (i in 0 until childCount) {
val child = getChildAt(i)
val childWidth = child.measuredWidth
val topBound = 0 /* there is just one row */
if (i == 0) {
child.layout(0, topBound, childWidth, childWidth)
} else {
val leftBound = i * percentOverlap * childWidth
child.layout(
leftBound.toInt(),
topBound,
leftBound.toInt() + childWidth,
topBound + childWidth)
}
}
}
}
}
ImageViews are created programmatically:
val context = holder.rootView.context
val imageView: ImageView = ImageView(context)
GlideApp.with(context)
.load(imageUrl)
.placeholder(userPlaceholder)
.fallback(userPlaceholder)
.error(userPlaceholder)
.transform(CircleWithBorderTransform())
.into(imageView)
holder.membersIcons.addView(imageView)
Glide transformation:
override fun transform(pool: BitmapPool, source: Bitmap, outWidth: Int, outHeight: Int): Bitmap {
val width = source.width
val height = source.height
val canvasBitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888)
val shader = BitmapShader(source, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP)
val paint = Paint()
paint.isAntiAlias = true
paint.shader = shader
val canvas = Canvas(canvasBitmap)
val radius = if (width > height) height.toFloat() / 2f else width.toFloat() / 2f
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
//border around the image
paint.shader = null
paint.style = Paint.Style.STROKE
paint.strokeWidth = 2f
paint.color = Color.WHITE
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
return canvasBitmap
}

add a comment |
I am implementing custom ViewGroup which has overlapping ImageViews.
I load images with GlideApp. Problem is that some images are cropped a little (visible on the left side) and I cannot prevent this. The drawables which get cropped are Vector drawables and their mDrawableWidth & mDrawableHeight are larger than the height of the ViewGroup. I thought that the images would be scaled to fit the view dimensions (mScaleType = FIT_CENTER).
class OverlappingImageViewGroup : ViewGroup {
constructor(context: Context) : this(context, null)
constructor(context: Context, attrs: AttributeSet?) : this(context, attrs, 0)
constructor(context: Context, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr) {}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
val widthSize: Int = getDefaultSize(0, widthMeasureSpec)
val heightSize: Int = getDefaultSize(0, heightMeasureSpec)
val majorDimension = Math.min(widthSize, heightSize)
//Measure all child views
val childSpec = MeasureSpec.makeMeasureSpec(majorDimension, MeasureSpec.EXACTLY)
measureChildren(childSpec, childSpec)
//MUST call this to save our own dimensions
setMeasuredDimension(majorDimension, majorDimension)
}
override fun onLayout(changed: Boolean,
leftRelativeToParent: Int,
topRelativeToParent: Int,
rightRelativeToParent: Int,
bottomRelativeToParent: Int) {
val percentOverlap = 0.8f
if (childCount > 0) {
for (i in 0 until childCount) {
val child = getChildAt(i)
val childWidth = child.measuredWidth
val topBound = 0 /* there is just one row */
if (i == 0) {
child.layout(0, topBound, childWidth, childWidth)
} else {
val leftBound = i * percentOverlap * childWidth
child.layout(
leftBound.toInt(),
topBound,
leftBound.toInt() + childWidth,
topBound + childWidth)
}
}
}
}
}
ImageViews are created programmatically:
val context = holder.rootView.context
val imageView: ImageView = ImageView(context)
GlideApp.with(context)
.load(imageUrl)
.placeholder(userPlaceholder)
.fallback(userPlaceholder)
.error(userPlaceholder)
.transform(CircleWithBorderTransform())
.into(imageView)
holder.membersIcons.addView(imageView)
Glide transformation:
override fun transform(pool: BitmapPool, source: Bitmap, outWidth: Int, outHeight: Int): Bitmap {
val width = source.width
val height = source.height
val canvasBitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888)
val shader = BitmapShader(source, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP)
val paint = Paint()
paint.isAntiAlias = true
paint.shader = shader
val canvas = Canvas(canvasBitmap)
val radius = if (width > height) height.toFloat() / 2f else width.toFloat() / 2f
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
//border around the image
paint.shader = null
paint.style = Paint.Style.STROKE
paint.strokeWidth = 2f
paint.color = Color.WHITE
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
return canvasBitmap
}

add a comment |
I am implementing custom ViewGroup which has overlapping ImageViews.
I load images with GlideApp. Problem is that some images are cropped a little (visible on the left side) and I cannot prevent this. The drawables which get cropped are Vector drawables and their mDrawableWidth & mDrawableHeight are larger than the height of the ViewGroup. I thought that the images would be scaled to fit the view dimensions (mScaleType = FIT_CENTER).
class OverlappingImageViewGroup : ViewGroup {
constructor(context: Context) : this(context, null)
constructor(context: Context, attrs: AttributeSet?) : this(context, attrs, 0)
constructor(context: Context, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr) {}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
val widthSize: Int = getDefaultSize(0, widthMeasureSpec)
val heightSize: Int = getDefaultSize(0, heightMeasureSpec)
val majorDimension = Math.min(widthSize, heightSize)
//Measure all child views
val childSpec = MeasureSpec.makeMeasureSpec(majorDimension, MeasureSpec.EXACTLY)
measureChildren(childSpec, childSpec)
//MUST call this to save our own dimensions
setMeasuredDimension(majorDimension, majorDimension)
}
override fun onLayout(changed: Boolean,
leftRelativeToParent: Int,
topRelativeToParent: Int,
rightRelativeToParent: Int,
bottomRelativeToParent: Int) {
val percentOverlap = 0.8f
if (childCount > 0) {
for (i in 0 until childCount) {
val child = getChildAt(i)
val childWidth = child.measuredWidth
val topBound = 0 /* there is just one row */
if (i == 0) {
child.layout(0, topBound, childWidth, childWidth)
} else {
val leftBound = i * percentOverlap * childWidth
child.layout(
leftBound.toInt(),
topBound,
leftBound.toInt() + childWidth,
topBound + childWidth)
}
}
}
}
}
ImageViews are created programmatically:
val context = holder.rootView.context
val imageView: ImageView = ImageView(context)
GlideApp.with(context)
.load(imageUrl)
.placeholder(userPlaceholder)
.fallback(userPlaceholder)
.error(userPlaceholder)
.transform(CircleWithBorderTransform())
.into(imageView)
holder.membersIcons.addView(imageView)
Glide transformation:
override fun transform(pool: BitmapPool, source: Bitmap, outWidth: Int, outHeight: Int): Bitmap {
val width = source.width
val height = source.height
val canvasBitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888)
val shader = BitmapShader(source, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP)
val paint = Paint()
paint.isAntiAlias = true
paint.shader = shader
val canvas = Canvas(canvasBitmap)
val radius = if (width > height) height.toFloat() / 2f else width.toFloat() / 2f
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
//border around the image
paint.shader = null
paint.style = Paint.Style.STROKE
paint.strokeWidth = 2f
paint.color = Color.WHITE
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
return canvasBitmap
}

I am implementing custom ViewGroup which has overlapping ImageViews.
I load images with GlideApp. Problem is that some images are cropped a little (visible on the left side) and I cannot prevent this. The drawables which get cropped are Vector drawables and their mDrawableWidth & mDrawableHeight are larger than the height of the ViewGroup. I thought that the images would be scaled to fit the view dimensions (mScaleType = FIT_CENTER).
class OverlappingImageViewGroup : ViewGroup {
constructor(context: Context) : this(context, null)
constructor(context: Context, attrs: AttributeSet?) : this(context, attrs, 0)
constructor(context: Context, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr) {}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
val widthSize: Int = getDefaultSize(0, widthMeasureSpec)
val heightSize: Int = getDefaultSize(0, heightMeasureSpec)
val majorDimension = Math.min(widthSize, heightSize)
//Measure all child views
val childSpec = MeasureSpec.makeMeasureSpec(majorDimension, MeasureSpec.EXACTLY)
measureChildren(childSpec, childSpec)
//MUST call this to save our own dimensions
setMeasuredDimension(majorDimension, majorDimension)
}
override fun onLayout(changed: Boolean,
leftRelativeToParent: Int,
topRelativeToParent: Int,
rightRelativeToParent: Int,
bottomRelativeToParent: Int) {
val percentOverlap = 0.8f
if (childCount > 0) {
for (i in 0 until childCount) {
val child = getChildAt(i)
val childWidth = child.measuredWidth
val topBound = 0 /* there is just one row */
if (i == 0) {
child.layout(0, topBound, childWidth, childWidth)
} else {
val leftBound = i * percentOverlap * childWidth
child.layout(
leftBound.toInt(),
topBound,
leftBound.toInt() + childWidth,
topBound + childWidth)
}
}
}
}
}
ImageViews are created programmatically:
val context = holder.rootView.context
val imageView: ImageView = ImageView(context)
GlideApp.with(context)
.load(imageUrl)
.placeholder(userPlaceholder)
.fallback(userPlaceholder)
.error(userPlaceholder)
.transform(CircleWithBorderTransform())
.into(imageView)
holder.membersIcons.addView(imageView)
Glide transformation:
override fun transform(pool: BitmapPool, source: Bitmap, outWidth: Int, outHeight: Int): Bitmap {
val width = source.width
val height = source.height
val canvasBitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888)
val shader = BitmapShader(source, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP)
val paint = Paint()
paint.isAntiAlias = true
paint.shader = shader
val canvas = Canvas(canvasBitmap)
val radius = if (width > height) height.toFloat() / 2f else width.toFloat() / 2f
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
//border around the image
paint.shader = null
paint.style = Paint.Style.STROKE
paint.strokeWidth = 2f
paint.color = Color.WHITE
canvas.drawCircle((width / 2).toFloat(), (height / 2).toFloat(), radius, paint)
return canvasBitmap
}


asked Nov 23 '18 at 15:29
AngelinaAngelina
426315
426315
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449349%2fcropping-of-imageviews-in-custom-viewgroup%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449349%2fcropping-of-imageviews-in-custom-viewgroup%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9DbGr S5Pt,B8qrz