GET request to a local IP in C with sockets
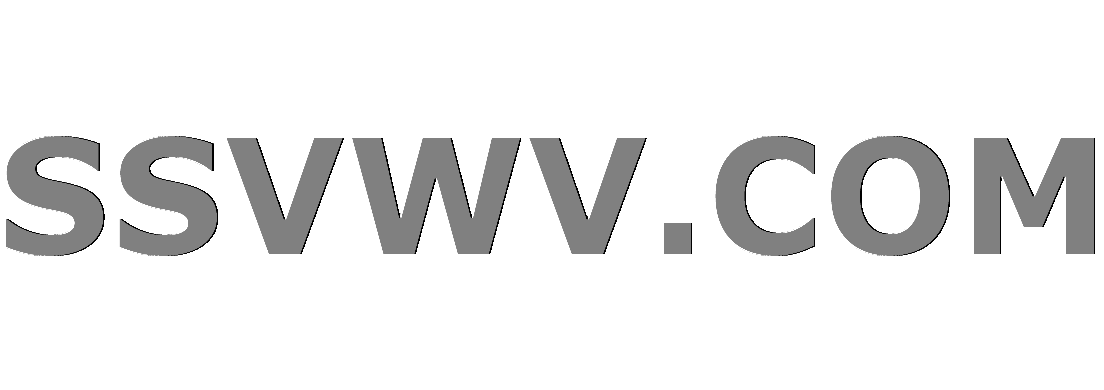
Multi tool use
I am trying to put together a C code that makes an HTTP GET
request to a local IP address (in my network) that has a webpage runing on port 80. The request should return the index.html
.
The code works for a website with a real IP that has a DNS (I have tested with www.olx.ro
and its IP), but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response..
This is the code:
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <netdb.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <arpa/inet.h>
int main(void)
{
int byte_count;
int sockfd = 0,n = 0;
char recvBuff[9000];
struct sockaddr_in serv_addr;
memset(recvBuff, '0' ,sizeof(recvBuff));
if((sockfd = socket(AF_INET, SOCK_STREAM, 0))< 0)
{
printf("n Error : Could not create socket n");
return 1;
}
else printf("Created socket successfully.n");
printf("n");
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(80);
serv_addr.sin_addr.s_addr = inet_addr("10.74.4.10");
if(connect(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr))<0)
{
printf("n Error : Connect Failed n");
return 1;
}
else printf("Connected successfully. n ");
printf("n n");
char *header = "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn";
send(sockfd,header,strlen(header),0);
byte_count = recv(sockfd,recvBuff,sizeof(recvBuff),0);
printf("Received %d bytes of data in bufn",byte_count);
printf("n");
printf("%.*s",byte_count,recvBuff);
return 0;
}
If I change inet_addr("10.74.4.10")
to inet_addr("2.16.128.73")
, and "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn"
to "GET /index.html HTTP/1.0rnHost:www.olx.ro:80rnrn"
, then the code works as intended.
Also, I would like to mention I use Repl.it as C compiler.
EDIT: I forgot to mention, the code stops at connect()
. I have tried using bind()
, still doesn't work. Maybe it's because I am trying to connect to a privileged port (80), with an online C compiler that cannot have root access?
c sockets get host repl.it
add a comment |
I am trying to put together a C code that makes an HTTP GET
request to a local IP address (in my network) that has a webpage runing on port 80. The request should return the index.html
.
The code works for a website with a real IP that has a DNS (I have tested with www.olx.ro
and its IP), but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response..
This is the code:
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <netdb.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <arpa/inet.h>
int main(void)
{
int byte_count;
int sockfd = 0,n = 0;
char recvBuff[9000];
struct sockaddr_in serv_addr;
memset(recvBuff, '0' ,sizeof(recvBuff));
if((sockfd = socket(AF_INET, SOCK_STREAM, 0))< 0)
{
printf("n Error : Could not create socket n");
return 1;
}
else printf("Created socket successfully.n");
printf("n");
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(80);
serv_addr.sin_addr.s_addr = inet_addr("10.74.4.10");
if(connect(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr))<0)
{
printf("n Error : Connect Failed n");
return 1;
}
else printf("Connected successfully. n ");
printf("n n");
char *header = "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn";
send(sockfd,header,strlen(header),0);
byte_count = recv(sockfd,recvBuff,sizeof(recvBuff),0);
printf("Received %d bytes of data in bufn",byte_count);
printf("n");
printf("%.*s",byte_count,recvBuff);
return 0;
}
If I change inet_addr("10.74.4.10")
to inet_addr("2.16.128.73")
, and "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn"
to "GET /index.html HTTP/1.0rnHost:www.olx.ro:80rnrn"
, then the code works as intended.
Also, I would like to mention I use Repl.it as C compiler.
EDIT: I forgot to mention, the code stops at connect()
. I have tried using bind()
, still doesn't work. Maybe it's because I am trying to connect to a privileged port (80), with an online C compiler that cannot have root access?
c sockets get host repl.it
add a comment |
I am trying to put together a C code that makes an HTTP GET
request to a local IP address (in my network) that has a webpage runing on port 80. The request should return the index.html
.
The code works for a website with a real IP that has a DNS (I have tested with www.olx.ro
and its IP), but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response..
This is the code:
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <netdb.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <arpa/inet.h>
int main(void)
{
int byte_count;
int sockfd = 0,n = 0;
char recvBuff[9000];
struct sockaddr_in serv_addr;
memset(recvBuff, '0' ,sizeof(recvBuff));
if((sockfd = socket(AF_INET, SOCK_STREAM, 0))< 0)
{
printf("n Error : Could not create socket n");
return 1;
}
else printf("Created socket successfully.n");
printf("n");
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(80);
serv_addr.sin_addr.s_addr = inet_addr("10.74.4.10");
if(connect(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr))<0)
{
printf("n Error : Connect Failed n");
return 1;
}
else printf("Connected successfully. n ");
printf("n n");
char *header = "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn";
send(sockfd,header,strlen(header),0);
byte_count = recv(sockfd,recvBuff,sizeof(recvBuff),0);
printf("Received %d bytes of data in bufn",byte_count);
printf("n");
printf("%.*s",byte_count,recvBuff);
return 0;
}
If I change inet_addr("10.74.4.10")
to inet_addr("2.16.128.73")
, and "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn"
to "GET /index.html HTTP/1.0rnHost:www.olx.ro:80rnrn"
, then the code works as intended.
Also, I would like to mention I use Repl.it as C compiler.
EDIT: I forgot to mention, the code stops at connect()
. I have tried using bind()
, still doesn't work. Maybe it's because I am trying to connect to a privileged port (80), with an online C compiler that cannot have root access?
c sockets get host repl.it
I am trying to put together a C code that makes an HTTP GET
request to a local IP address (in my network) that has a webpage runing on port 80. The request should return the index.html
.
The code works for a website with a real IP that has a DNS (I have tested with www.olx.ro
and its IP), but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response..
This is the code:
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <netdb.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <arpa/inet.h>
int main(void)
{
int byte_count;
int sockfd = 0,n = 0;
char recvBuff[9000];
struct sockaddr_in serv_addr;
memset(recvBuff, '0' ,sizeof(recvBuff));
if((sockfd = socket(AF_INET, SOCK_STREAM, 0))< 0)
{
printf("n Error : Could not create socket n");
return 1;
}
else printf("Created socket successfully.n");
printf("n");
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(80);
serv_addr.sin_addr.s_addr = inet_addr("10.74.4.10");
if(connect(sockfd, (struct sockaddr *)&serv_addr, sizeof(serv_addr))<0)
{
printf("n Error : Connect Failed n");
return 1;
}
else printf("Connected successfully. n ");
printf("n n");
char *header = "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn";
send(sockfd,header,strlen(header),0);
byte_count = recv(sockfd,recvBuff,sizeof(recvBuff),0);
printf("Received %d bytes of data in bufn",byte_count);
printf("n");
printf("%.*s",byte_count,recvBuff);
return 0;
}
If I change inet_addr("10.74.4.10")
to inet_addr("2.16.128.73")
, and "GET /index.html HTTP/1.0rnHost:10.74.4.10:80rnrn"
to "GET /index.html HTTP/1.0rnHost:www.olx.ro:80rnrn"
, then the code works as intended.
Also, I would like to mention I use Repl.it as C compiler.
EDIT: I forgot to mention, the code stops at connect()
. I have tried using bind()
, still doesn't work. Maybe it's because I am trying to connect to a privileged port (80), with an online C compiler that cannot have root access?
c sockets get host repl.it
c sockets get host repl.it
edited Nov 24 '18 at 10:37
Mihnea Paul
asked Nov 23 '18 at 13:28


Mihnea PaulMihnea Paul
12
12
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
... but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response.
While you send a valid HTTP request you tell the server using the Host
header that you want to access the hostname 10.74.4.10 configured at the server. If this works or not depends on the servers configuration. For example if the server is configured to only accept requests for the name example.com
and to consider everything else wrong it will only accept requests with Host: example.com
but not with Host: 10.74.4.10
even if this might be the IP address of the server.
... I forgot to mention, the code stops at connect()
This makes no sense at at all. If you get a 400 Bad Request
as you first claim the connection to the site need to be successful first, which means it could not have stopped in connect
.
FYI, theHost
header is not officially defined in HTTP 1.0, which the code is using.Host
is required only in HTTP 1.1+.
– Remy Lebeau
Nov 24 '18 at 8:44
@RemyLebeau: WhileHost
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.
– Steffen Ullrich
Nov 24 '18 at 8:56
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
|
show 13 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447613%2fget-request-to-a-local-ip-in-c-with-sockets%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
... but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response.
While you send a valid HTTP request you tell the server using the Host
header that you want to access the hostname 10.74.4.10 configured at the server. If this works or not depends on the servers configuration. For example if the server is configured to only accept requests for the name example.com
and to consider everything else wrong it will only accept requests with Host: example.com
but not with Host: 10.74.4.10
even if this might be the IP address of the server.
... I forgot to mention, the code stops at connect()
This makes no sense at at all. If you get a 400 Bad Request
as you first claim the connection to the site need to be successful first, which means it could not have stopped in connect
.
FYI, theHost
header is not officially defined in HTTP 1.0, which the code is using.Host
is required only in HTTP 1.1+.
– Remy Lebeau
Nov 24 '18 at 8:44
@RemyLebeau: WhileHost
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.
– Steffen Ullrich
Nov 24 '18 at 8:56
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
|
show 13 more comments
... but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response.
While you send a valid HTTP request you tell the server using the Host
header that you want to access the hostname 10.74.4.10 configured at the server. If this works or not depends on the servers configuration. For example if the server is configured to only accept requests for the name example.com
and to consider everything else wrong it will only accept requests with Host: example.com
but not with Host: 10.74.4.10
even if this might be the IP address of the server.
... I forgot to mention, the code stops at connect()
This makes no sense at at all. If you get a 400 Bad Request
as you first claim the connection to the site need to be successful first, which means it could not have stopped in connect
.
FYI, theHost
header is not officially defined in HTTP 1.0, which the code is using.Host
is required only in HTTP 1.1+.
– Remy Lebeau
Nov 24 '18 at 8:44
@RemyLebeau: WhileHost
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.
– Steffen Ullrich
Nov 24 '18 at 8:56
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
|
show 13 more comments
... but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response.
While you send a valid HTTP request you tell the server using the Host
header that you want to access the hostname 10.74.4.10 configured at the server. If this works or not depends on the servers configuration. For example if the server is configured to only accept requests for the name example.com
and to consider everything else wrong it will only accept requests with Host: example.com
but not with Host: 10.74.4.10
even if this might be the IP address of the server.
... I forgot to mention, the code stops at connect()
This makes no sense at at all. If you get a 400 Bad Request
as you first claim the connection to the site need to be successful first, which means it could not have stopped in connect
.
... but when I try to put the local IP (which is 10.74.4.10), I get a 400 Bad Request response.
While you send a valid HTTP request you tell the server using the Host
header that you want to access the hostname 10.74.4.10 configured at the server. If this works or not depends on the servers configuration. For example if the server is configured to only accept requests for the name example.com
and to consider everything else wrong it will only accept requests with Host: example.com
but not with Host: 10.74.4.10
even if this might be the IP address of the server.
... I forgot to mention, the code stops at connect()
This makes no sense at at all. If you get a 400 Bad Request
as you first claim the connection to the site need to be successful first, which means it could not have stopped in connect
.
answered Nov 23 '18 at 16:18


Steffen UllrichSteffen Ullrich
62.6k360102
62.6k360102
FYI, theHost
header is not officially defined in HTTP 1.0, which the code is using.Host
is required only in HTTP 1.1+.
– Remy Lebeau
Nov 24 '18 at 8:44
@RemyLebeau: WhileHost
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.
– Steffen Ullrich
Nov 24 '18 at 8:56
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
|
show 13 more comments
FYI, theHost
header is not officially defined in HTTP 1.0, which the code is using.Host
is required only in HTTP 1.1+.
– Remy Lebeau
Nov 24 '18 at 8:44
@RemyLebeau: WhileHost
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.
– Steffen Ullrich
Nov 24 '18 at 8:56
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
FYI, the
Host
header is not officially defined in HTTP 1.0, which the code is using. Host
is required only in HTTP 1.1+.– Remy Lebeau
Nov 24 '18 at 8:44
FYI, the
Host
header is not officially defined in HTTP 1.0, which the code is using. Host
is required only in HTTP 1.1+.– Remy Lebeau
Nov 24 '18 at 8:44
@RemyLebeau: While
Host
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.– Steffen Ullrich
Nov 24 '18 at 8:56
@RemyLebeau: While
Host
is in theory not required by HTTP/1.0 it is still needed when doing a HTTP request against a server which hosts multiple domains on the same IP address - since without it the server does not know which domain the client wants to access. Historically the browsers first implemented HTTP/1.0, then HTTP/1.0 with Host header and then HTTP/1.1. This is actually the same with SNI in TLS: it was not required until TLS 1.3 but still it is actually needed when accessing any CDN or other multi-domain servers.– Steffen Ullrich
Nov 24 '18 at 8:56
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
Let me clarify: FIrst, 400 bad request was happening when the i put "...Host: some real IP, not local", with both HTTP1.0 and 1.1. Second, the code stopped at connect() when inet_addr() had a local IP address
– Mihnea Paul
Nov 24 '18 at 9:45
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@MihneaPaul: I see - in the first case you likely accessed an existing server but provided a Host header not expected by this server. In the second case where the connect is hanging the server you are trying to reach either does not exist at all (they don't magically exist on all IP addresses) or is blocked by a firewall. You should get similar problems if you use a browser from the same machine to the same target.
– Steffen Ullrich
Nov 24 '18 at 9:49
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
@SteffenUllrich I had a comment about this but apparently it was deleted..thing is, the problem seemed to have been 1) the port 80 - being privileged and 2) the method connect() should have been bind(). I created a react app on port 3000, and the bind() worked, but now the program exists because of the send() method, with no explanation..
– Mihnea Paul
Nov 24 '18 at 9:52
|
show 13 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447613%2fget-request-to-a-local-ip-in-c-with-sockets%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6YwDIK,5A 3TMwkuTU29IcQKK,znBlAg,zNx,TR3bfTALcGVx 93HYnfG Yr