How to get a C# .net custom control to update appearance during design time?
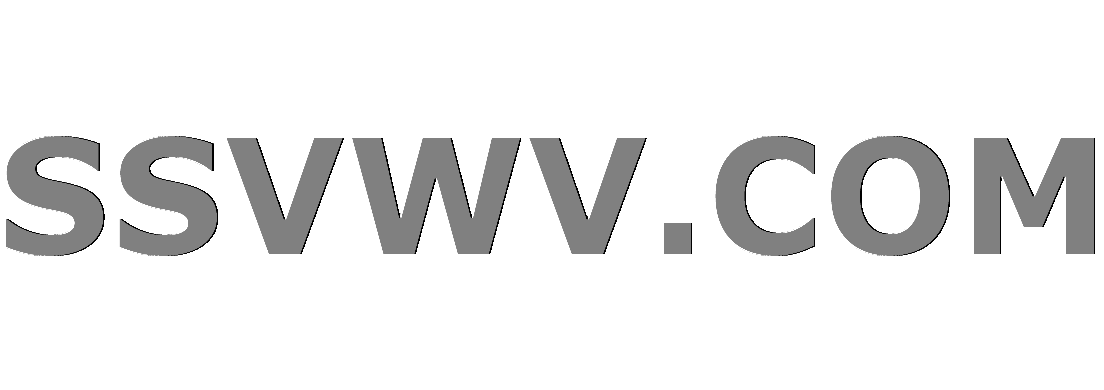
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
OK I have a custom Circular Button that I extended from Button class. See Below:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing;
namespace CircleButton
{
public class CircleButton : Button
{
private Color _fillColor = Color.Red;
private Color _hoverColor = Color.Blue;
[Category("Custom")]
[Browsable(true)]
[Description("Sets the fill color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color FillColor
{
set
{
this._fillColor = value;
}
get
{
return this._fillColor;
}
}
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
set
{
this._hoverColor = value;
}
get
{
return this._hoverColor;
}
}
protected override void OnPaint(PaintEventArgs pevent)
{
GraphicsPath gp = new GraphicsPath();
gp.AddEllipse(0, 0, ClientSize.Width, ClientSize.Height);
this.Region = new System.Drawing.Region(gp);
base.OnPaint(pevent);
}
protected override void OnCreateControl()
{
this.FlatAppearance.MouseOverBackColor = this._hoverColor;
this.FlatAppearance.BorderSize = 0;
this.BackColor = this._fillColor;
this.FlatStyle = FlatStyle.Flat;
base.OnCreateControl();
}
}
Everthing works great in the Visual Studio Designer, but when I select the FillColor and HoverColor properties during design time, the colors on the design time control do not update.
Keep in mind that the colors DO show the appropriate change during runtime.
Maybe I am missing another directive or something? I have searched but unable to come up with an answer. I have spent 2 days on this.
This control is going to be distributed to another designer and needs to work properly during design time.
Any help would be most appreciated.
c# visual-studio winforms
add a comment |
OK I have a custom Circular Button that I extended from Button class. See Below:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing;
namespace CircleButton
{
public class CircleButton : Button
{
private Color _fillColor = Color.Red;
private Color _hoverColor = Color.Blue;
[Category("Custom")]
[Browsable(true)]
[Description("Sets the fill color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color FillColor
{
set
{
this._fillColor = value;
}
get
{
return this._fillColor;
}
}
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
set
{
this._hoverColor = value;
}
get
{
return this._hoverColor;
}
}
protected override void OnPaint(PaintEventArgs pevent)
{
GraphicsPath gp = new GraphicsPath();
gp.AddEllipse(0, 0, ClientSize.Width, ClientSize.Height);
this.Region = new System.Drawing.Region(gp);
base.OnPaint(pevent);
}
protected override void OnCreateControl()
{
this.FlatAppearance.MouseOverBackColor = this._hoverColor;
this.FlatAppearance.BorderSize = 0;
this.BackColor = this._fillColor;
this.FlatStyle = FlatStyle.Flat;
base.OnCreateControl();
}
}
Everthing works great in the Visual Studio Designer, but when I select the FillColor and HoverColor properties during design time, the colors on the design time control do not update.
Keep in mind that the colors DO show the appropriate change during runtime.
Maybe I am missing another directive or something? I have searched but unable to come up with an answer. I have spent 2 days on this.
This control is going to be distributed to another designer and needs to work properly during design time.
Any help would be most appreciated.
c# visual-studio winforms
1
You need to callInvalidate()
which trigger the paint method
– Nat Pongjardenlarp
Nov 24 '18 at 23:47
See this (in a way simiilar) custom control: Translucent circle with text. See theNotifyPropertyChanged
handler (thePropertyChanged?.Invoke()
is not relevant) and when/what properties call it. This a simple/functional method to refresh a control look when some properties are change at design-time.
– Jimi
Nov 24 '18 at 23:49
add a comment |
OK I have a custom Circular Button that I extended from Button class. See Below:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing;
namespace CircleButton
{
public class CircleButton : Button
{
private Color _fillColor = Color.Red;
private Color _hoverColor = Color.Blue;
[Category("Custom")]
[Browsable(true)]
[Description("Sets the fill color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color FillColor
{
set
{
this._fillColor = value;
}
get
{
return this._fillColor;
}
}
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
set
{
this._hoverColor = value;
}
get
{
return this._hoverColor;
}
}
protected override void OnPaint(PaintEventArgs pevent)
{
GraphicsPath gp = new GraphicsPath();
gp.AddEllipse(0, 0, ClientSize.Width, ClientSize.Height);
this.Region = new System.Drawing.Region(gp);
base.OnPaint(pevent);
}
protected override void OnCreateControl()
{
this.FlatAppearance.MouseOverBackColor = this._hoverColor;
this.FlatAppearance.BorderSize = 0;
this.BackColor = this._fillColor;
this.FlatStyle = FlatStyle.Flat;
base.OnCreateControl();
}
}
Everthing works great in the Visual Studio Designer, but when I select the FillColor and HoverColor properties during design time, the colors on the design time control do not update.
Keep in mind that the colors DO show the appropriate change during runtime.
Maybe I am missing another directive or something? I have searched but unable to come up with an answer. I have spent 2 days on this.
This control is going to be distributed to another designer and needs to work properly during design time.
Any help would be most appreciated.
c# visual-studio winforms
OK I have a custom Circular Button that I extended from Button class. See Below:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing;
namespace CircleButton
{
public class CircleButton : Button
{
private Color _fillColor = Color.Red;
private Color _hoverColor = Color.Blue;
[Category("Custom")]
[Browsable(true)]
[Description("Sets the fill color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color FillColor
{
set
{
this._fillColor = value;
}
get
{
return this._fillColor;
}
}
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
set
{
this._hoverColor = value;
}
get
{
return this._hoverColor;
}
}
protected override void OnPaint(PaintEventArgs pevent)
{
GraphicsPath gp = new GraphicsPath();
gp.AddEllipse(0, 0, ClientSize.Width, ClientSize.Height);
this.Region = new System.Drawing.Region(gp);
base.OnPaint(pevent);
}
protected override void OnCreateControl()
{
this.FlatAppearance.MouseOverBackColor = this._hoverColor;
this.FlatAppearance.BorderSize = 0;
this.BackColor = this._fillColor;
this.FlatStyle = FlatStyle.Flat;
base.OnCreateControl();
}
}
Everthing works great in the Visual Studio Designer, but when I select the FillColor and HoverColor properties during design time, the colors on the design time control do not update.
Keep in mind that the colors DO show the appropriate change during runtime.
Maybe I am missing another directive or something? I have searched but unable to come up with an answer. I have spent 2 days on this.
This control is going to be distributed to another designer and needs to work properly during design time.
Any help would be most appreciated.
c# visual-studio winforms
c# visual-studio winforms
edited Nov 24 '18 at 23:54


Dai
74.6k15120209
74.6k15120209
asked Nov 24 '18 at 23:32


Jim StewartJim Stewart
11
11
1
You need to callInvalidate()
which trigger the paint method
– Nat Pongjardenlarp
Nov 24 '18 at 23:47
See this (in a way simiilar) custom control: Translucent circle with text. See theNotifyPropertyChanged
handler (thePropertyChanged?.Invoke()
is not relevant) and when/what properties call it. This a simple/functional method to refresh a control look when some properties are change at design-time.
– Jimi
Nov 24 '18 at 23:49
add a comment |
1
You need to callInvalidate()
which trigger the paint method
– Nat Pongjardenlarp
Nov 24 '18 at 23:47
See this (in a way simiilar) custom control: Translucent circle with text. See theNotifyPropertyChanged
handler (thePropertyChanged?.Invoke()
is not relevant) and when/what properties call it. This a simple/functional method to refresh a control look when some properties are change at design-time.
– Jimi
Nov 24 '18 at 23:49
1
1
You need to call
Invalidate()
which trigger the paint method– Nat Pongjardenlarp
Nov 24 '18 at 23:47
You need to call
Invalidate()
which trigger the paint method– Nat Pongjardenlarp
Nov 24 '18 at 23:47
See this (in a way simiilar) custom control: Translucent circle with text. See the
NotifyPropertyChanged
handler (the PropertyChanged?.Invoke()
is not relevant) and when/what properties call it. This a simple/functional method to refresh a control look when some properties are change at design-time.– Jimi
Nov 24 '18 at 23:49
See this (in a way simiilar) custom control: Translucent circle with text. See the
NotifyPropertyChanged
handler (the PropertyChanged?.Invoke()
is not relevant) and when/what properties call it. This a simple/functional method to refresh a control look when some properties are change at design-time.– Jimi
Nov 24 '18 at 23:49
add a comment |
2 Answers
2
active
oldest
votes
I recommend reading these articles:
- Creating a Windows Forms Control That Takes Advantage of Visual Studio Design-Time Features
- Whats the difference between Control.Invalidate, Control.Update and Control.Refresh?
In short, you need to call Invalidate()
in your property setters, as this triggers an eventual repaint (repaints are not immediate: a repaint request actually queues-up a repaint, so if two or more properties are set then the control will still only be repainted once (this is why UI properties must be set in the UI thread).
You should also perform equality checks in property setters to prevent unnecessary repainting, like so:
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
get
{
return this._hoverColor;
}
set
{
if( this._hoverColor != value )
{
this._hoverColor = value;
this.Invalidate();
}
}
}
(Style-tip: In recent years the C# developer ecosystem (and Microsoft too) have started to use _underscore
-prefixed identifiers only for static
fields and normal camelCase
for instance fields, but always accessed with this.
, so I would change this._hoverColor
to this.hoverColor
).
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using justInvalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.
– Jimi
Nov 25 '18 at 0:09
@Jimi In the "Translucent circle with text" example, theBackColor
property belongs toLabel
and not the custom control subclass, soLabel
could still be doing stuff behind-the-scenes to trigger a live update in the designer.
– Dai
Nov 25 '18 at 0:34
The BackColor property is overridden,base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also settingbase.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).
– Jimi
Nov 25 '18 at 0:41
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
|
show 1 more comment
Ok so I finally solved it by changing the property in the setter, while adding the Invalidate() Method:
if (this._fillColor != value)
{
this._fillColor = value;
this.BackColor = this._fillColor; ///<--Added this line worked
this.Invalidate();
}
Thank you for your great suggestions and answers, I truly appreciate your help!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463326%2fhow-to-get-a-c-sharp-net-custom-control-to-update-appearance-during-design-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I recommend reading these articles:
- Creating a Windows Forms Control That Takes Advantage of Visual Studio Design-Time Features
- Whats the difference between Control.Invalidate, Control.Update and Control.Refresh?
In short, you need to call Invalidate()
in your property setters, as this triggers an eventual repaint (repaints are not immediate: a repaint request actually queues-up a repaint, so if two or more properties are set then the control will still only be repainted once (this is why UI properties must be set in the UI thread).
You should also perform equality checks in property setters to prevent unnecessary repainting, like so:
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
get
{
return this._hoverColor;
}
set
{
if( this._hoverColor != value )
{
this._hoverColor = value;
this.Invalidate();
}
}
}
(Style-tip: In recent years the C# developer ecosystem (and Microsoft too) have started to use _underscore
-prefixed identifiers only for static
fields and normal camelCase
for instance fields, but always accessed with this.
, so I would change this._hoverColor
to this.hoverColor
).
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using justInvalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.
– Jimi
Nov 25 '18 at 0:09
@Jimi In the "Translucent circle with text" example, theBackColor
property belongs toLabel
and not the custom control subclass, soLabel
could still be doing stuff behind-the-scenes to trigger a live update in the designer.
– Dai
Nov 25 '18 at 0:34
The BackColor property is overridden,base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also settingbase.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).
– Jimi
Nov 25 '18 at 0:41
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
|
show 1 more comment
I recommend reading these articles:
- Creating a Windows Forms Control That Takes Advantage of Visual Studio Design-Time Features
- Whats the difference between Control.Invalidate, Control.Update and Control.Refresh?
In short, you need to call Invalidate()
in your property setters, as this triggers an eventual repaint (repaints are not immediate: a repaint request actually queues-up a repaint, so if two or more properties are set then the control will still only be repainted once (this is why UI properties must be set in the UI thread).
You should also perform equality checks in property setters to prevent unnecessary repainting, like so:
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
get
{
return this._hoverColor;
}
set
{
if( this._hoverColor != value )
{
this._hoverColor = value;
this.Invalidate();
}
}
}
(Style-tip: In recent years the C# developer ecosystem (and Microsoft too) have started to use _underscore
-prefixed identifiers only for static
fields and normal camelCase
for instance fields, but always accessed with this.
, so I would change this._hoverColor
to this.hoverColor
).
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using justInvalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.
– Jimi
Nov 25 '18 at 0:09
@Jimi In the "Translucent circle with text" example, theBackColor
property belongs toLabel
and not the custom control subclass, soLabel
could still be doing stuff behind-the-scenes to trigger a live update in the designer.
– Dai
Nov 25 '18 at 0:34
The BackColor property is overridden,base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also settingbase.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).
– Jimi
Nov 25 '18 at 0:41
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
|
show 1 more comment
I recommend reading these articles:
- Creating a Windows Forms Control That Takes Advantage of Visual Studio Design-Time Features
- Whats the difference between Control.Invalidate, Control.Update and Control.Refresh?
In short, you need to call Invalidate()
in your property setters, as this triggers an eventual repaint (repaints are not immediate: a repaint request actually queues-up a repaint, so if two or more properties are set then the control will still only be repainted once (this is why UI properties must be set in the UI thread).
You should also perform equality checks in property setters to prevent unnecessary repainting, like so:
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
get
{
return this._hoverColor;
}
set
{
if( this._hoverColor != value )
{
this._hoverColor = value;
this.Invalidate();
}
}
}
(Style-tip: In recent years the C# developer ecosystem (and Microsoft too) have started to use _underscore
-prefixed identifiers only for static
fields and normal camelCase
for instance fields, but always accessed with this.
, so I would change this._hoverColor
to this.hoverColor
).
I recommend reading these articles:
- Creating a Windows Forms Control That Takes Advantage of Visual Studio Design-Time Features
- Whats the difference between Control.Invalidate, Control.Update and Control.Refresh?
In short, you need to call Invalidate()
in your property setters, as this triggers an eventual repaint (repaints are not immediate: a repaint request actually queues-up a repaint, so if two or more properties are set then the control will still only be repainted once (this is why UI properties must be set in the UI thread).
You should also perform equality checks in property setters to prevent unnecessary repainting, like so:
[Category("Custom")]
[Browsable(true)]
[Description("Sets the Hover color of the round button")]
[Editor(typeof(System.Windows.Forms.Design.WindowsFormsComponentEditor), typeof(System.Drawing.Color))]
public Color HoverColor
{
get
{
return this._hoverColor;
}
set
{
if( this._hoverColor != value )
{
this._hoverColor = value;
this.Invalidate();
}
}
}
(Style-tip: In recent years the C# developer ecosystem (and Microsoft too) have started to use _underscore
-prefixed identifiers only for static
fields and normal camelCase
for instance fields, but always accessed with this.
, so I would change this._hoverColor
to this.hoverColor
).
edited Nov 25 '18 at 0:42
answered Nov 24 '18 at 23:58


DaiDai
74.6k15120209
74.6k15120209
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using justInvalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.
– Jimi
Nov 25 '18 at 0:09
@Jimi In the "Translucent circle with text" example, theBackColor
property belongs toLabel
and not the custom control subclass, soLabel
could still be doing stuff behind-the-scenes to trigger a live update in the designer.
– Dai
Nov 25 '18 at 0:34
The BackColor property is overridden,base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also settingbase.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).
– Jimi
Nov 25 '18 at 0:41
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
|
show 1 more comment
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using justInvalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.
– Jimi
Nov 25 '18 at 0:09
@Jimi In the "Translucent circle with text" example, theBackColor
property belongs toLabel
and not the custom control subclass, soLabel
could still be doing stuff behind-the-scenes to trigger a live update in the designer.
– Dai
Nov 25 '18 at 0:34
The BackColor property is overridden,base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also settingbase.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).
– Jimi
Nov 25 '18 at 0:41
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using just
Invalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.– Jimi
Nov 25 '18 at 0:09
It should be noted that this works for solid colors only and not in any occasion. For example, take the class that I posted in the OP's comments. Using just
Invalidate()
on a property change, the colors won't be updated correctly. You'ld have to click on the Form for the update to complete. It would require a different custom designer attached to the class to work as intended.– Jimi
Nov 25 '18 at 0:09
@Jimi In the "Translucent circle with text" example, the
BackColor
property belongs to Label
and not the custom control subclass, so Label
could still be doing stuff behind-the-scenes to trigger a live update in the designer.– Dai
Nov 25 '18 at 0:34
@Jimi In the "Translucent circle with text" example, the
BackColor
property belongs to Label
and not the custom control subclass, so Label
could still be doing stuff behind-the-scenes to trigger a live update in the designer.– Dai
Nov 25 '18 at 0:34
The BackColor property is overridden,
base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+ WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also setting base.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).– Jimi
Nov 25 '18 at 0:41
The BackColor property is overridden,
base
is not called and the Custom Label doesn't have a background color, it's not even painted (ControlStyles.Opaque
+ WS_EX_TRANSPARENT
). But you'ld have the same result with a system-painted background and also setting base.BackColor
with this Color type (ControlStyles.SupportsTransparentBackColor
). The Text property wouldn't be updated correctly, too (at design-time).– Jimi
Nov 25 '18 at 0:41
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
Unfortunately, Invalidate() does nothing. I tried it again. (I forgot to mention that I had already tried this) but still nothing. I DID add the equality check though, it was great suggestion.
– Jim Stewart
Nov 25 '18 at 2:12
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
@JimStewart You may need to rebuild your project and/or restart Visual Studio (if your control is in a loaded library instead of your active project).
– Dai
Nov 25 '18 at 2:14
|
show 1 more comment
Ok so I finally solved it by changing the property in the setter, while adding the Invalidate() Method:
if (this._fillColor != value)
{
this._fillColor = value;
this.BackColor = this._fillColor; ///<--Added this line worked
this.Invalidate();
}
Thank you for your great suggestions and answers, I truly appreciate your help!
add a comment |
Ok so I finally solved it by changing the property in the setter, while adding the Invalidate() Method:
if (this._fillColor != value)
{
this._fillColor = value;
this.BackColor = this._fillColor; ///<--Added this line worked
this.Invalidate();
}
Thank you for your great suggestions and answers, I truly appreciate your help!
add a comment |
Ok so I finally solved it by changing the property in the setter, while adding the Invalidate() Method:
if (this._fillColor != value)
{
this._fillColor = value;
this.BackColor = this._fillColor; ///<--Added this line worked
this.Invalidate();
}
Thank you for your great suggestions and answers, I truly appreciate your help!
Ok so I finally solved it by changing the property in the setter, while adding the Invalidate() Method:
if (this._fillColor != value)
{
this._fillColor = value;
this.BackColor = this._fillColor; ///<--Added this line worked
this.Invalidate();
}
Thank you for your great suggestions and answers, I truly appreciate your help!
answered Nov 25 '18 at 2:22


Jim StewartJim Stewart
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463326%2fhow-to-get-a-c-sharp-net-custom-control-to-update-appearance-during-design-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iiD,nt,cejBSyiWuFZAVF BgO4bp1j,5ouwxFGmz1ZBFx5 7EsCIzTj87swjec,RYrwrw,AaRb,U,W8Hbs
1
You need to call
Invalidate()
which trigger the paint method– Nat Pongjardenlarp
Nov 24 '18 at 23:47
See this (in a way simiilar) custom control: Translucent circle with text. See the
NotifyPropertyChanged
handler (thePropertyChanged?.Invoke()
is not relevant) and when/what properties call it. This a simple/functional method to refresh a control look when some properties are change at design-time.– Jimi
Nov 24 '18 at 23:49