React - Passing Props
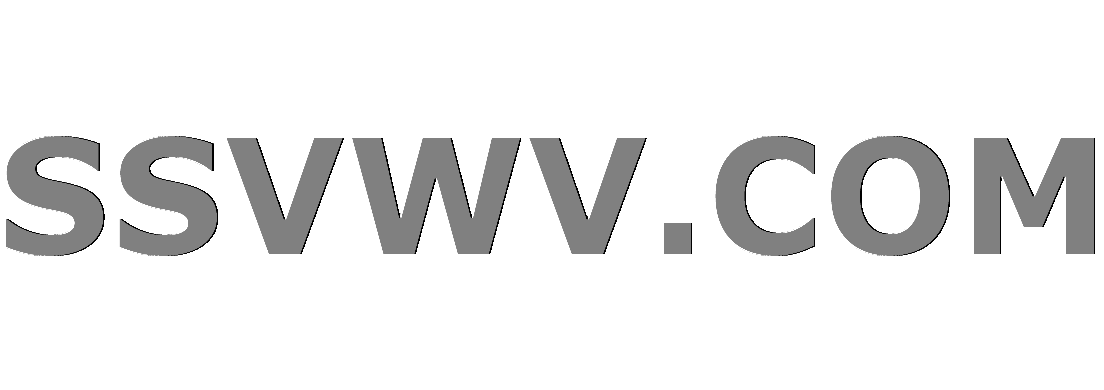
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Currently, it's giving me an error saying that isCompleted is undefined.
todo.isCompleted = todo.isCompleted ? false : true;
The above code is what's throwing the error.
The advice Im getting is to pass a todoIndex prop in App.js when you're rendering the Todo component.
Not sure how to go about doing that. Any pointers?
import React, { Component } from 'react';
import './App.css';
import ToDo from './components/ToDo.js';
class App extends Component {
constructor(props) {
super(props);
this.state = {
todos: [
{ description: 'Walk the cat', isCompleted: true },
{ description: 'Throw the dishes away', isCompleted: false },
{ description: 'Buy new dishes', isCompleted: false }],
newTodoDescription: ''
};
}
deleteToDo(deleteToDo) {
console.log(this);
let newToDos = this.state.todos.filter((todo) => {
return todo !== deleteToDo
} )
this.setState({ todos: newToDos });
}
handleChange(e) {
this.setState({ newTodoDescription: e.target.value })
}
handleSubmit(e) {
e.preventDefault();
if (!this.state.newTodoDescription) { return }
const newTodo = { description: this.state.newTodoDescription, isCompleted: false };
this.setState({ todos: [...this.state.todos, newTodo], newTodoDescription: '' });
}
toggleComplete(index) {
const todos = this.state.todos.slice();
const todo = todos[index];
todo.isCompleted = todo.isCompleted ? false : true;
this.setState({ todos: todos });
}
render() {
return (
<div className="App">
<ul>
{ this.state.todos.map( (todo, index) =>
<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted } toggleComplete={ this.toggleComplete.bind(this) } deleteToDo={() => this.deleteToDo(todo)} />
)}
</ul>
<form onSubmit={ (e) => this.handleSubmit(e) }>
<input type="text" value={ this.state.newTodoDescription } onChange={ (e) => this.handleChange(e) } />
<input type="submit" />
</form>
</div>
);
}
}
export default App;
ToDo.js
import React, { Component } from 'react';
class ToDo extends Component {
toggleComplete = () => {
this.props.toggleComplete(this.props.todoIndex)
}
render() {
return (
<ul>
<input type= "checkbox" checked= { this.props.isCompleted }
onChange= { this.handleToggleClick.bind(this)} />
<span>{ this.props.description }</span>
<button onClick={ this.props.deleteToDo }> X </button>
</ul>
);
}
}
export default ToDo;
reactjs react-props
add a comment |
Currently, it's giving me an error saying that isCompleted is undefined.
todo.isCompleted = todo.isCompleted ? false : true;
The above code is what's throwing the error.
The advice Im getting is to pass a todoIndex prop in App.js when you're rendering the Todo component.
Not sure how to go about doing that. Any pointers?
import React, { Component } from 'react';
import './App.css';
import ToDo from './components/ToDo.js';
class App extends Component {
constructor(props) {
super(props);
this.state = {
todos: [
{ description: 'Walk the cat', isCompleted: true },
{ description: 'Throw the dishes away', isCompleted: false },
{ description: 'Buy new dishes', isCompleted: false }],
newTodoDescription: ''
};
}
deleteToDo(deleteToDo) {
console.log(this);
let newToDos = this.state.todos.filter((todo) => {
return todo !== deleteToDo
} )
this.setState({ todos: newToDos });
}
handleChange(e) {
this.setState({ newTodoDescription: e.target.value })
}
handleSubmit(e) {
e.preventDefault();
if (!this.state.newTodoDescription) { return }
const newTodo = { description: this.state.newTodoDescription, isCompleted: false };
this.setState({ todos: [...this.state.todos, newTodo], newTodoDescription: '' });
}
toggleComplete(index) {
const todos = this.state.todos.slice();
const todo = todos[index];
todo.isCompleted = todo.isCompleted ? false : true;
this.setState({ todos: todos });
}
render() {
return (
<div className="App">
<ul>
{ this.state.todos.map( (todo, index) =>
<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted } toggleComplete={ this.toggleComplete.bind(this) } deleteToDo={() => this.deleteToDo(todo)} />
)}
</ul>
<form onSubmit={ (e) => this.handleSubmit(e) }>
<input type="text" value={ this.state.newTodoDescription } onChange={ (e) => this.handleChange(e) } />
<input type="submit" />
</form>
</div>
);
}
}
export default App;
ToDo.js
import React, { Component } from 'react';
class ToDo extends Component {
toggleComplete = () => {
this.props.toggleComplete(this.props.todoIndex)
}
render() {
return (
<ul>
<input type= "checkbox" checked= { this.props.isCompleted }
onChange= { this.handleToggleClick.bind(this)} />
<span>{ this.props.description }</span>
<button onClick={ this.props.deleteToDo }> X </button>
</ul>
);
}
}
export default ToDo;
reactjs react-props
add a comment |
Currently, it's giving me an error saying that isCompleted is undefined.
todo.isCompleted = todo.isCompleted ? false : true;
The above code is what's throwing the error.
The advice Im getting is to pass a todoIndex prop in App.js when you're rendering the Todo component.
Not sure how to go about doing that. Any pointers?
import React, { Component } from 'react';
import './App.css';
import ToDo from './components/ToDo.js';
class App extends Component {
constructor(props) {
super(props);
this.state = {
todos: [
{ description: 'Walk the cat', isCompleted: true },
{ description: 'Throw the dishes away', isCompleted: false },
{ description: 'Buy new dishes', isCompleted: false }],
newTodoDescription: ''
};
}
deleteToDo(deleteToDo) {
console.log(this);
let newToDos = this.state.todos.filter((todo) => {
return todo !== deleteToDo
} )
this.setState({ todos: newToDos });
}
handleChange(e) {
this.setState({ newTodoDescription: e.target.value })
}
handleSubmit(e) {
e.preventDefault();
if (!this.state.newTodoDescription) { return }
const newTodo = { description: this.state.newTodoDescription, isCompleted: false };
this.setState({ todos: [...this.state.todos, newTodo], newTodoDescription: '' });
}
toggleComplete(index) {
const todos = this.state.todos.slice();
const todo = todos[index];
todo.isCompleted = todo.isCompleted ? false : true;
this.setState({ todos: todos });
}
render() {
return (
<div className="App">
<ul>
{ this.state.todos.map( (todo, index) =>
<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted } toggleComplete={ this.toggleComplete.bind(this) } deleteToDo={() => this.deleteToDo(todo)} />
)}
</ul>
<form onSubmit={ (e) => this.handleSubmit(e) }>
<input type="text" value={ this.state.newTodoDescription } onChange={ (e) => this.handleChange(e) } />
<input type="submit" />
</form>
</div>
);
}
}
export default App;
ToDo.js
import React, { Component } from 'react';
class ToDo extends Component {
toggleComplete = () => {
this.props.toggleComplete(this.props.todoIndex)
}
render() {
return (
<ul>
<input type= "checkbox" checked= { this.props.isCompleted }
onChange= { this.handleToggleClick.bind(this)} />
<span>{ this.props.description }</span>
<button onClick={ this.props.deleteToDo }> X </button>
</ul>
);
}
}
export default ToDo;
reactjs react-props
Currently, it's giving me an error saying that isCompleted is undefined.
todo.isCompleted = todo.isCompleted ? false : true;
The above code is what's throwing the error.
The advice Im getting is to pass a todoIndex prop in App.js when you're rendering the Todo component.
Not sure how to go about doing that. Any pointers?
import React, { Component } from 'react';
import './App.css';
import ToDo from './components/ToDo.js';
class App extends Component {
constructor(props) {
super(props);
this.state = {
todos: [
{ description: 'Walk the cat', isCompleted: true },
{ description: 'Throw the dishes away', isCompleted: false },
{ description: 'Buy new dishes', isCompleted: false }],
newTodoDescription: ''
};
}
deleteToDo(deleteToDo) {
console.log(this);
let newToDos = this.state.todos.filter((todo) => {
return todo !== deleteToDo
} )
this.setState({ todos: newToDos });
}
handleChange(e) {
this.setState({ newTodoDescription: e.target.value })
}
handleSubmit(e) {
e.preventDefault();
if (!this.state.newTodoDescription) { return }
const newTodo = { description: this.state.newTodoDescription, isCompleted: false };
this.setState({ todos: [...this.state.todos, newTodo], newTodoDescription: '' });
}
toggleComplete(index) {
const todos = this.state.todos.slice();
const todo = todos[index];
todo.isCompleted = todo.isCompleted ? false : true;
this.setState({ todos: todos });
}
render() {
return (
<div className="App">
<ul>
{ this.state.todos.map( (todo, index) =>
<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted } toggleComplete={ this.toggleComplete.bind(this) } deleteToDo={() => this.deleteToDo(todo)} />
)}
</ul>
<form onSubmit={ (e) => this.handleSubmit(e) }>
<input type="text" value={ this.state.newTodoDescription } onChange={ (e) => this.handleChange(e) } />
<input type="submit" />
</form>
</div>
);
}
}
export default App;
ToDo.js
import React, { Component } from 'react';
class ToDo extends Component {
toggleComplete = () => {
this.props.toggleComplete(this.props.todoIndex)
}
render() {
return (
<ul>
<input type= "checkbox" checked= { this.props.isCompleted }
onChange= { this.handleToggleClick.bind(this)} />
<span>{ this.props.description }</span>
<button onClick={ this.props.deleteToDo }> X </button>
</ul>
);
}
}
export default ToDo;
reactjs react-props
reactjs react-props
edited Nov 25 '18 at 14:41
Nguyen You
4,9833831
4,9833831
asked Nov 24 '18 at 23:44


cjl85cjl85
7510
7510
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
To pass the index to the ToDo
component add another prop:
<ToDo key={index} todoIndex={index} ... />
and make sure that the component calls toggleComplete
with that index prop, i.e.
class ToDo extends React.Component {
toggleComplete() {
this.props.toggleComplete(this.props.todoIndex)
}
}
Also, you're mutating the todo object in your toggleComplete
function, instead of:
todo.isCompleted = todo.isCompleted ? false : true;
Better do this:
const todos[index] = {...todo, isCompleted: !todo.isCompleted }
Or with Object.assign
:
const todos[index] = Object.assign({}, todo, {isCompleted: !isCompleted})
I think Max Kurtz answer is also correct, the this
binding seems to be problematic. Bind the toggleComplete
function in the constructor or use arrow functions to make sure this
doesn't bite you.
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
has been added.
– cjl85
Nov 25 '18 at 2:10
Thanks! So it seems you're already passingthis.props.todoIndex
in the ToDo component when you callthis.props.toggleComplete
. But you're not specifying thetodoIndex
prop when you're rendering those ToDo components in the App component. On this line<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463391%2freact-passing-props%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To pass the index to the ToDo
component add another prop:
<ToDo key={index} todoIndex={index} ... />
and make sure that the component calls toggleComplete
with that index prop, i.e.
class ToDo extends React.Component {
toggleComplete() {
this.props.toggleComplete(this.props.todoIndex)
}
}
Also, you're mutating the todo object in your toggleComplete
function, instead of:
todo.isCompleted = todo.isCompleted ? false : true;
Better do this:
const todos[index] = {...todo, isCompleted: !todo.isCompleted }
Or with Object.assign
:
const todos[index] = Object.assign({}, todo, {isCompleted: !isCompleted})
I think Max Kurtz answer is also correct, the this
binding seems to be problematic. Bind the toggleComplete
function in the constructor or use arrow functions to make sure this
doesn't bite you.
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
has been added.
– cjl85
Nov 25 '18 at 2:10
Thanks! So it seems you're already passingthis.props.todoIndex
in the ToDo component when you callthis.props.toggleComplete
. But you're not specifying thetodoIndex
prop when you're rendering those ToDo components in the App component. On this line<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
|
show 3 more comments
To pass the index to the ToDo
component add another prop:
<ToDo key={index} todoIndex={index} ... />
and make sure that the component calls toggleComplete
with that index prop, i.e.
class ToDo extends React.Component {
toggleComplete() {
this.props.toggleComplete(this.props.todoIndex)
}
}
Also, you're mutating the todo object in your toggleComplete
function, instead of:
todo.isCompleted = todo.isCompleted ? false : true;
Better do this:
const todos[index] = {...todo, isCompleted: !todo.isCompleted }
Or with Object.assign
:
const todos[index] = Object.assign({}, todo, {isCompleted: !isCompleted})
I think Max Kurtz answer is also correct, the this
binding seems to be problematic. Bind the toggleComplete
function in the constructor or use arrow functions to make sure this
doesn't bite you.
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
has been added.
– cjl85
Nov 25 '18 at 2:10
Thanks! So it seems you're already passingthis.props.todoIndex
in the ToDo component when you callthis.props.toggleComplete
. But you're not specifying thetodoIndex
prop when you're rendering those ToDo components in the App component. On this line<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
|
show 3 more comments
To pass the index to the ToDo
component add another prop:
<ToDo key={index} todoIndex={index} ... />
and make sure that the component calls toggleComplete
with that index prop, i.e.
class ToDo extends React.Component {
toggleComplete() {
this.props.toggleComplete(this.props.todoIndex)
}
}
Also, you're mutating the todo object in your toggleComplete
function, instead of:
todo.isCompleted = todo.isCompleted ? false : true;
Better do this:
const todos[index] = {...todo, isCompleted: !todo.isCompleted }
Or with Object.assign
:
const todos[index] = Object.assign({}, todo, {isCompleted: !isCompleted})
I think Max Kurtz answer is also correct, the this
binding seems to be problematic. Bind the toggleComplete
function in the constructor or use arrow functions to make sure this
doesn't bite you.
To pass the index to the ToDo
component add another prop:
<ToDo key={index} todoIndex={index} ... />
and make sure that the component calls toggleComplete
with that index prop, i.e.
class ToDo extends React.Component {
toggleComplete() {
this.props.toggleComplete(this.props.todoIndex)
}
}
Also, you're mutating the todo object in your toggleComplete
function, instead of:
todo.isCompleted = todo.isCompleted ? false : true;
Better do this:
const todos[index] = {...todo, isCompleted: !todo.isCompleted }
Or with Object.assign
:
const todos[index] = Object.assign({}, todo, {isCompleted: !isCompleted})
I think Max Kurtz answer is also correct, the this
binding seems to be problematic. Bind the toggleComplete
function in the constructor or use arrow functions to make sure this
doesn't bite you.
edited Nov 25 '18 at 0:18
answered Nov 24 '18 at 23:55
StefanStefan
60028
60028
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
has been added.
– cjl85
Nov 25 '18 at 2:10
Thanks! So it seems you're already passingthis.props.todoIndex
in the ToDo component when you callthis.props.toggleComplete
. But you're not specifying thetodoIndex
prop when you're rendering those ToDo components in the App component. On this line<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
|
show 3 more comments
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
has been added.
– cjl85
Nov 25 '18 at 2:10
Thanks! So it seems you're already passingthis.props.todoIndex
in the ToDo component when you callthis.props.toggleComplete
. But you're not specifying thetodoIndex
prop when you're rendering those ToDo components in the App component. On this line<ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
appreciate the help. I understand changing the ternary expression, but it gives me an error saying that index is an fatal unexpected token.
– cjl85
Nov 25 '18 at 1:47
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
for your last comment about this, are you saying that I should avoid using it whenever possible and what would be an example of using an arrow function instead? Would replacing the this binding with arrow functions throughout the program be considered better practice or convention?
– cjl85
Nov 25 '18 at 1:52
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
You can use the ternary, that's fine. You are not showing the ToDo component implementation, so I can only guess. I assume that it is calling the toggleComplete function. But that function needs the index of the todo and the ToDo component doesn't know that index because you're not passing it as a prop.
– Stefan
Nov 25 '18 at 2:06
has been added.
– cjl85
Nov 25 '18 at 2:10
has been added.
– cjl85
Nov 25 '18 at 2:10
Thanks! So it seems you're already passing
this.props.todoIndex
in the ToDo component when you call this.props.toggleComplete
. But you're not specifying the todoIndex
prop when you're rendering those ToDo components in the App component. On this line <ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
Thanks! So it seems you're already passing
this.props.todoIndex
in the ToDo component when you call this.props.toggleComplete
. But you're not specifying the todoIndex
prop when you're rendering those ToDo components in the App component. On this line <ToDo key={ index } description={ todo.description } isCompleted={ todo.isCompleted }... />
– Stefan
Nov 25 '18 at 2:15
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463391%2freact-passing-props%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OASmFN nw,XlLXMSDO