What are the first 4 bytes of a std::vector?
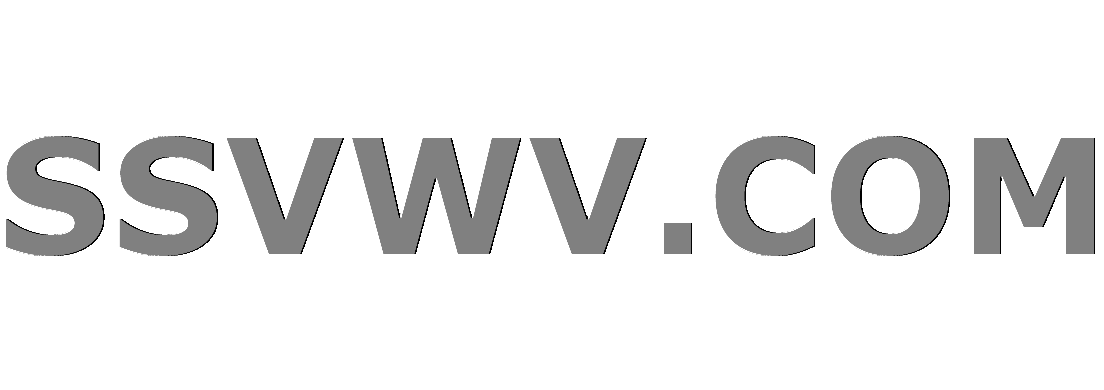
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I would like to manually read the values, number of values, and capacity allotted to the vector.
From reading this, I believed that a std::vector could be manually accessed via the following struct
struct _vector{
DWORD* begin;
DWORD* end;
DWORD* tail;
};
and that I could access the first value in the vector by doing the following.
_vector *vec = (_vector*)vectorAddress;
DWORD first_value = vec->begin[0];
However instead I found that this is not the case. I needed to add another 4 byte value at the top of the struct in order to properly do access addresses for begin, end, and tail. Below is the working version of what I would like to do.
#include <iostream>
#include <windows.h>
#include <vector>
std::vector<DWORD> vectorData;
void readVector(DWORD vectorAddress){
struct _vector{
DWORD* WHATISTHIS;
DWORD* begin;
DWORD* end;
DWORD* tail;
};
_vector* vec = (_vector*)vectorAddress;
DWORD count = ((DWORD)vec->end - (DWORD)vec->begin) / sizeof(DWORD);
DWORD capacity = ((DWORD)vec->tail - (DWORD)vec->begin) / sizeof(DWORD);
printf("Vector has %d items and %d capacityn", count, capacity);
for (int i = 0; i < count; i++)
printf("tValue at %d is 0x%xn", i, vec->begin[i]);
}
int main(void){
vectorData.reserve(3);
vectorData.push_back(0x123456);
vectorData.push_back(0x654321);
while (true){
readVector((DWORD)&vectorData);
system("pause");
}
}
Output of program:
Vector has 2 items and 3 capacity
Value at 0 is 0x123456
Value at 1 is 0x654321
Press any key to continue . . .
Given this what does the first 4 bytes of my _vector struct actually represent?
c++ vector
add a comment |
I would like to manually read the values, number of values, and capacity allotted to the vector.
From reading this, I believed that a std::vector could be manually accessed via the following struct
struct _vector{
DWORD* begin;
DWORD* end;
DWORD* tail;
};
and that I could access the first value in the vector by doing the following.
_vector *vec = (_vector*)vectorAddress;
DWORD first_value = vec->begin[0];
However instead I found that this is not the case. I needed to add another 4 byte value at the top of the struct in order to properly do access addresses for begin, end, and tail. Below is the working version of what I would like to do.
#include <iostream>
#include <windows.h>
#include <vector>
std::vector<DWORD> vectorData;
void readVector(DWORD vectorAddress){
struct _vector{
DWORD* WHATISTHIS;
DWORD* begin;
DWORD* end;
DWORD* tail;
};
_vector* vec = (_vector*)vectorAddress;
DWORD count = ((DWORD)vec->end - (DWORD)vec->begin) / sizeof(DWORD);
DWORD capacity = ((DWORD)vec->tail - (DWORD)vec->begin) / sizeof(DWORD);
printf("Vector has %d items and %d capacityn", count, capacity);
for (int i = 0; i < count; i++)
printf("tValue at %d is 0x%xn", i, vec->begin[i]);
}
int main(void){
vectorData.reserve(3);
vectorData.push_back(0x123456);
vectorData.push_back(0x654321);
while (true){
readVector((DWORD)&vectorData);
system("pause");
}
}
Output of program:
Vector has 2 items and 3 capacity
Value at 0 is 0x123456
Value at 1 is 0x654321
Press any key to continue . . .
Given this what does the first 4 bytes of my _vector struct actually represent?
c++ vector
5
No, the article is explaining a possible implementation ofstd::vector
(actually from a quick look I think only the standard-demanded interface). In no way is the layout guaranteed and you are certainly not allowed to just reinterpret it into some custom structure. Whatever result you are getting this way it is just undefined behavior.
– user10605163
Nov 24 '18 at 20:54
6
vector
's internals are implementation specific. The "fields" you believevector
has are just accessor functions which are mandated by the standard. If you absolutely need low-level access, usevector::data()
. Its only other state variables are given bysize()
andcapacity()
.
– meowgoesthedog
Nov 24 '18 at 20:54
2
The only way to know is to look at the source code for your implementation. All the standard guarantees is the interface and complexity, the actual implementation is left to the library implementor.
– NathanOliver
Nov 24 '18 at 21:08
I see, thank you all for your input on the issue.
– a_user
Nov 24 '18 at 21:08
add a comment |
I would like to manually read the values, number of values, and capacity allotted to the vector.
From reading this, I believed that a std::vector could be manually accessed via the following struct
struct _vector{
DWORD* begin;
DWORD* end;
DWORD* tail;
};
and that I could access the first value in the vector by doing the following.
_vector *vec = (_vector*)vectorAddress;
DWORD first_value = vec->begin[0];
However instead I found that this is not the case. I needed to add another 4 byte value at the top of the struct in order to properly do access addresses for begin, end, and tail. Below is the working version of what I would like to do.
#include <iostream>
#include <windows.h>
#include <vector>
std::vector<DWORD> vectorData;
void readVector(DWORD vectorAddress){
struct _vector{
DWORD* WHATISTHIS;
DWORD* begin;
DWORD* end;
DWORD* tail;
};
_vector* vec = (_vector*)vectorAddress;
DWORD count = ((DWORD)vec->end - (DWORD)vec->begin) / sizeof(DWORD);
DWORD capacity = ((DWORD)vec->tail - (DWORD)vec->begin) / sizeof(DWORD);
printf("Vector has %d items and %d capacityn", count, capacity);
for (int i = 0; i < count; i++)
printf("tValue at %d is 0x%xn", i, vec->begin[i]);
}
int main(void){
vectorData.reserve(3);
vectorData.push_back(0x123456);
vectorData.push_back(0x654321);
while (true){
readVector((DWORD)&vectorData);
system("pause");
}
}
Output of program:
Vector has 2 items and 3 capacity
Value at 0 is 0x123456
Value at 1 is 0x654321
Press any key to continue . . .
Given this what does the first 4 bytes of my _vector struct actually represent?
c++ vector
I would like to manually read the values, number of values, and capacity allotted to the vector.
From reading this, I believed that a std::vector could be manually accessed via the following struct
struct _vector{
DWORD* begin;
DWORD* end;
DWORD* tail;
};
and that I could access the first value in the vector by doing the following.
_vector *vec = (_vector*)vectorAddress;
DWORD first_value = vec->begin[0];
However instead I found that this is not the case. I needed to add another 4 byte value at the top of the struct in order to properly do access addresses for begin, end, and tail. Below is the working version of what I would like to do.
#include <iostream>
#include <windows.h>
#include <vector>
std::vector<DWORD> vectorData;
void readVector(DWORD vectorAddress){
struct _vector{
DWORD* WHATISTHIS;
DWORD* begin;
DWORD* end;
DWORD* tail;
};
_vector* vec = (_vector*)vectorAddress;
DWORD count = ((DWORD)vec->end - (DWORD)vec->begin) / sizeof(DWORD);
DWORD capacity = ((DWORD)vec->tail - (DWORD)vec->begin) / sizeof(DWORD);
printf("Vector has %d items and %d capacityn", count, capacity);
for (int i = 0; i < count; i++)
printf("tValue at %d is 0x%xn", i, vec->begin[i]);
}
int main(void){
vectorData.reserve(3);
vectorData.push_back(0x123456);
vectorData.push_back(0x654321);
while (true){
readVector((DWORD)&vectorData);
system("pause");
}
}
Output of program:
Vector has 2 items and 3 capacity
Value at 0 is 0x123456
Value at 1 is 0x654321
Press any key to continue . . .
Given this what does the first 4 bytes of my _vector struct actually represent?
c++ vector
c++ vector
asked Nov 24 '18 at 20:45
a_usera_user
42
42
5
No, the article is explaining a possible implementation ofstd::vector
(actually from a quick look I think only the standard-demanded interface). In no way is the layout guaranteed and you are certainly not allowed to just reinterpret it into some custom structure. Whatever result you are getting this way it is just undefined behavior.
– user10605163
Nov 24 '18 at 20:54
6
vector
's internals are implementation specific. The "fields" you believevector
has are just accessor functions which are mandated by the standard. If you absolutely need low-level access, usevector::data()
. Its only other state variables are given bysize()
andcapacity()
.
– meowgoesthedog
Nov 24 '18 at 20:54
2
The only way to know is to look at the source code for your implementation. All the standard guarantees is the interface and complexity, the actual implementation is left to the library implementor.
– NathanOliver
Nov 24 '18 at 21:08
I see, thank you all for your input on the issue.
– a_user
Nov 24 '18 at 21:08
add a comment |
5
No, the article is explaining a possible implementation ofstd::vector
(actually from a quick look I think only the standard-demanded interface). In no way is the layout guaranteed and you are certainly not allowed to just reinterpret it into some custom structure. Whatever result you are getting this way it is just undefined behavior.
– user10605163
Nov 24 '18 at 20:54
6
vector
's internals are implementation specific. The "fields" you believevector
has are just accessor functions which are mandated by the standard. If you absolutely need low-level access, usevector::data()
. Its only other state variables are given bysize()
andcapacity()
.
– meowgoesthedog
Nov 24 '18 at 20:54
2
The only way to know is to look at the source code for your implementation. All the standard guarantees is the interface and complexity, the actual implementation is left to the library implementor.
– NathanOliver
Nov 24 '18 at 21:08
I see, thank you all for your input on the issue.
– a_user
Nov 24 '18 at 21:08
5
5
No, the article is explaining a possible implementation of
std::vector
(actually from a quick look I think only the standard-demanded interface). In no way is the layout guaranteed and you are certainly not allowed to just reinterpret it into some custom structure. Whatever result you are getting this way it is just undefined behavior.– user10605163
Nov 24 '18 at 20:54
No, the article is explaining a possible implementation of
std::vector
(actually from a quick look I think only the standard-demanded interface). In no way is the layout guaranteed and you are certainly not allowed to just reinterpret it into some custom structure. Whatever result you are getting this way it is just undefined behavior.– user10605163
Nov 24 '18 at 20:54
6
6
vector
's internals are implementation specific. The "fields" you believe vector
has are just accessor functions which are mandated by the standard. If you absolutely need low-level access, use vector::data()
. Its only other state variables are given by size()
and capacity()
.– meowgoesthedog
Nov 24 '18 at 20:54
vector
's internals are implementation specific. The "fields" you believe vector
has are just accessor functions which are mandated by the standard. If you absolutely need low-level access, use vector::data()
. Its only other state variables are given by size()
and capacity()
.– meowgoesthedog
Nov 24 '18 at 20:54
2
2
The only way to know is to look at the source code for your implementation. All the standard guarantees is the interface and complexity, the actual implementation is left to the library implementor.
– NathanOliver
Nov 24 '18 at 21:08
The only way to know is to look at the source code for your implementation. All the standard guarantees is the interface and complexity, the actual implementation is left to the library implementor.
– NathanOliver
Nov 24 '18 at 21:08
I see, thank you all for your input on the issue.
– a_user
Nov 24 '18 at 21:08
I see, thank you all for your input on the issue.
– a_user
Nov 24 '18 at 21:08
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462216%2fwhat-are-the-first-4-bytes-of-a-stdvector%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462216%2fwhat-are-the-first-4-bytes-of-a-stdvector%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AhPAdKVxSQWWwTKB9fP02MLphijXn6px,AUsrxHXv0hW4,Lkzd3eLWmVvq3ixdHS9VY,EP MEMI,bUmo2i,B5dnIgg cS,Vt3X
5
No, the article is explaining a possible implementation of
std::vector
(actually from a quick look I think only the standard-demanded interface). In no way is the layout guaranteed and you are certainly not allowed to just reinterpret it into some custom structure. Whatever result you are getting this way it is just undefined behavior.– user10605163
Nov 24 '18 at 20:54
6
vector
's internals are implementation specific. The "fields" you believevector
has are just accessor functions which are mandated by the standard. If you absolutely need low-level access, usevector::data()
. Its only other state variables are given bysize()
andcapacity()
.– meowgoesthedog
Nov 24 '18 at 20:54
2
The only way to know is to look at the source code for your implementation. All the standard guarantees is the interface and complexity, the actual implementation is left to the library implementor.
– NathanOliver
Nov 24 '18 at 21:08
I see, thank you all for your input on the issue.
– a_user
Nov 24 '18 at 21:08